mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
openssl.h
00001 /** 00002 * \file openssl.h 00003 * 00004 * \brief OpenSSL wrapper (definitions, inline functions). 00005 * 00006 * \deprecated Use native mbed TLS functions instead 00007 * 00008 * Copyright (C) 2006-2010, ARM Limited, All Rights Reserved 00009 * 00010 * This file is part of mbed TLS (https://tls.mbed.org) 00011 * 00012 * This program is free software; you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation; either version 2 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * This program is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License along 00023 * with this program; if not, write to the Free Software Foundation, Inc., 00024 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00025 */ 00026 00027 /* 00028 * OpenSSL wrapper contributed by David Barett 00029 */ 00030 00031 #if ! defined(POLARSSL_DEPRECATED_REMOVED) 00032 00033 #if defined(POLARSSL_DEPRECATED_WARNING) 00034 #warning "Including openssl.h is deprecated" 00035 #endif 00036 00037 #ifndef POLARSSL_OPENSSL_H 00038 #define POLARSSL_OPENSSL_H 00039 00040 #include "aes.h" 00041 #include "md5.h" 00042 #include "rsa.h" 00043 #include "sha1.h" 00044 00045 #define AES_SIZE 16 00046 #define AES_BLOCK_SIZE 16 00047 #define AES_KEY aes_context 00048 #define MD5_CTX md5_context 00049 #define SHA_CTX sha1_context 00050 00051 #define SHA1_Init( CTX ) \ 00052 sha1_starts( (CTX) ) 00053 #define SHA1_Update( CTX, BUF, LEN ) \ 00054 sha1_update( (CTX), (unsigned char *)(BUF), (LEN) ) 00055 #define SHA1_Final( OUT, CTX ) \ 00056 sha1_finish( (CTX), (OUT) ) 00057 00058 #define MD5_Init( CTX ) \ 00059 md5_starts( (CTX) ) 00060 #define MD5_Update( CTX, BUF, LEN ) \ 00061 md5_update( (CTX), (unsigned char *)(BUF), (LEN) ) 00062 #define MD5_Final( OUT, CTX ) \ 00063 md5_finish( (CTX), (OUT) ) 00064 00065 #define AES_set_encrypt_key( KEY, KEYSIZE, CTX ) \ 00066 aes_setkey_enc( (CTX), (KEY), (KEYSIZE) ) 00067 #define AES_set_decrypt_key( KEY, KEYSIZE, CTX ) \ 00068 aes_setkey_dec( (CTX), (KEY), (KEYSIZE) ) 00069 #define AES_cbc_encrypt( INPUT, OUTPUT, LEN, CTX, IV, MODE ) \ 00070 aes_crypt_cbc( (CTX), (MODE), (LEN), (IV), (INPUT), (OUTPUT) ) 00071 00072 #ifdef __cplusplus 00073 extern "C" { 00074 #endif 00075 00076 /* 00077 * RSA stuff follows. TODO: needs cleanup 00078 */ 00079 inline int __RSA_Passthrough( void *output, void *input, int size ) 00080 { 00081 memcpy( output, input, size ); 00082 return size; 00083 } 00084 00085 inline rsa_context* d2i_RSA_PUBKEY( void *ignore, unsigned char **bufptr, 00086 int len ) 00087 { 00088 unsigned char *buffer = *(unsigned char **) bufptr; 00089 rsa_context *rsa; 00090 00091 /* 00092 * Not a general-purpose parser: only parses public key from *exactly* 00093 * openssl genrsa -out privkey.pem 512 (or 1024) 00094 * openssl rsa -in privkey.pem -out privatekey.der -outform der 00095 * openssl rsa -in privkey.pem -out pubkey.der -outform der -pubout 00096 * 00097 * TODO: make a general-purpose parse 00098 */ 00099 if( ignore != 0 || ( len != 94 && len != 162 ) ) 00100 return( 0 ); 00101 00102 rsa = (rsa_context *) malloc( sizeof( rsa_rsa ) ); 00103 if( rsa == NULL ) 00104 return( 0 ); 00105 00106 memset( rsa, 0, sizeof( rsa_context ) ); 00107 00108 if( ( len == 94 && 00109 mpi_read_binary( &rsa->N , &buffer[ 25], 64 ) == 0 && 00110 mpi_read_binary( &rsa->E , &buffer[ 91], 3 ) == 0 ) || 00111 ( len == 162 && 00112 mpi_read_binary( &rsa->N , &buffer[ 29], 128 ) == 0 ) && 00113 mpi_read_binary( &rsa->E , &buffer[159], 3 ) == 0 ) 00114 { 00115 /* 00116 * key read successfully 00117 */ 00118 rsa->len = ( mpi_msb( &rsa->N ) + 7 ) >> 3; 00119 return( rsa ); 00120 } 00121 else 00122 { 00123 memset( rsa, 0, sizeof( rsa_context ) ); 00124 free( rsa ); 00125 return( 0 ); 00126 } 00127 } 00128 00129 #define RSA rsa_context 00130 #define RSA_PKCS1_PADDING 1 /* ignored; always encrypt with this */ 00131 #define RSA_size( CTX ) (CTX)->len 00132 #define RSA_free( CTX ) rsa_free( CTX ) 00133 #define ERR_get_error( ) "ERR_get_error() not supported" 00134 #define RSA_blinding_off( IGNORE ) 00135 00136 #define d2i_RSAPrivateKey( a, b, c ) new rsa_context /* TODO: C++ bleh */ 00137 00138 inline int RSA_public_decrypt ( int size, unsigned char* input, unsigned char* output, RSA* key, int ignore ) { int outsize=size; if( !rsa_pkcs1_decrypt( key, RSA_PUBLIC, &outsize, input, output ) ) return outsize; else return -1; } 00139 inline int RSA_private_decrypt( int size, unsigned char* input, unsigned char* output, RSA* key, int ignore ) { int outsize=size; if( !rsa_pkcs1_decrypt( key, RSA_PRIVATE, &outsize, input, output ) ) return outsize; else return -1; } 00140 inline int RSA_public_encrypt ( int size, unsigned char* input, unsigned char* output, RSA* key, int ignore ) { if( !rsa_pkcs1_encrypt( key, RSA_PUBLIC, size, input, output ) ) return RSA_size(key); else return -1; } 00141 inline int RSA_private_encrypt( int size, unsigned char* input, unsigned char* output, RSA* key, int ignore ) { if( !rsa_pkcs1_encrypt( key, RSA_PRIVATE, size, input, output ) ) return RSA_size(key); else return -1; } 00142 00143 #ifdef __cplusplus 00144 } 00145 #endif 00146 00147 #endif /* openssl.h */ 00148 #endif /* POLARSSL_DEPRECATED_REMOVED */ 00149
Generated on Tue Jul 12 2022 13:50:37 by
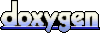