mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
dhm.h
00001 /** 00002 * \file dhm.h 00003 * 00004 * \brief Diffie-Hellman-Merkle key exchange 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_DHM_H 00025 #define POLARSSL_DHM_H 00026 00027 #include "bignum.h" 00028 00029 /* 00030 * DHM Error codes 00031 */ 00032 #define POLARSSL_ERR_DHM_BAD_INPUT_DATA -0x3080 /**< Bad input parameters to function. */ 00033 #define POLARSSL_ERR_DHM_READ_PARAMS_FAILED -0x3100 /**< Reading of the DHM parameters failed. */ 00034 #define POLARSSL_ERR_DHM_MAKE_PARAMS_FAILED -0x3180 /**< Making of the DHM parameters failed. */ 00035 #define POLARSSL_ERR_DHM_READ_PUBLIC_FAILED -0x3200 /**< Reading of the public values failed. */ 00036 #define POLARSSL_ERR_DHM_MAKE_PUBLIC_FAILED -0x3280 /**< Making of the public value failed. */ 00037 #define POLARSSL_ERR_DHM_CALC_SECRET_FAILED -0x3300 /**< Calculation of the DHM secret failed. */ 00038 #define POLARSSL_ERR_DHM_INVALID_FORMAT -0x3380 /**< The ASN.1 data is not formatted correctly. */ 00039 #define POLARSSL_ERR_DHM_MALLOC_FAILED -0x3400 /**< Allocation of memory failed. */ 00040 #define POLARSSL_ERR_DHM_FILE_IO_ERROR -0x3480 /**< Read/write of file failed. */ 00041 00042 /** 00043 * RFC 2409 defines a number of standardized Diffie-Hellman groups 00044 * that can be used. 00045 * RFC 3526 defines a number of standardized Diffie-Hellman groups 00046 * for IKE. 00047 * RFC 5114 defines a number of standardized Diffie-Hellman groups 00048 * that can be used. 00049 * 00050 * Some are included here for convenience. 00051 * 00052 * Included are: 00053 * RFC 2409 6.2. 1024-bit MODP Group (Second Oakley Group) 00054 * RFC 3526 3. 2048-bit MODP Group 00055 * RFC 3526 4. 3072-bit MODP Group 00056 * RFC 5114 2.1. 1024-bit MODP Group with 160-bit Prime Order Subgroup 00057 * RFC 5114 2.2. 2048-bit MODP Group with 224-bit Prime Order Subgroup 00058 */ 00059 #define POLARSSL_DHM_RFC2409_MODP_1024_P \ 00060 "FFFFFFFFFFFFFFFFC90FDAA22168C234C4C6628B80DC1CD1" \ 00061 "29024E088A67CC74020BBEA63B139B22514A08798E3404DD" \ 00062 "EF9519B3CD3A431B302B0A6DF25F14374FE1356D6D51C245" \ 00063 "E485B576625E7EC6F44C42E9A637ED6B0BFF5CB6F406B7ED" \ 00064 "EE386BFB5A899FA5AE9F24117C4B1FE649286651ECE65381" \ 00065 "FFFFFFFFFFFFFFFF" 00066 00067 #define POLARSSL_DHM_RFC2409_MODP_1024_G "02" 00068 00069 #define POLARSSL_DHM_RFC3526_MODP_2048_P \ 00070 "FFFFFFFFFFFFFFFFC90FDAA22168C234C4C6628B80DC1CD1" \ 00071 "29024E088A67CC74020BBEA63B139B22514A08798E3404DD" \ 00072 "EF9519B3CD3A431B302B0A6DF25F14374FE1356D6D51C245" \ 00073 "E485B576625E7EC6F44C42E9A637ED6B0BFF5CB6F406B7ED" \ 00074 "EE386BFB5A899FA5AE9F24117C4B1FE649286651ECE45B3D" \ 00075 "C2007CB8A163BF0598DA48361C55D39A69163FA8FD24CF5F" \ 00076 "83655D23DCA3AD961C62F356208552BB9ED529077096966D" \ 00077 "670C354E4ABC9804F1746C08CA18217C32905E462E36CE3B" \ 00078 "E39E772C180E86039B2783A2EC07A28FB5C55DF06F4C52C9" \ 00079 "DE2BCBF6955817183995497CEA956AE515D2261898FA0510" \ 00080 "15728E5A8AACAA68FFFFFFFFFFFFFFFF" 00081 00082 #define POLARSSL_DHM_RFC3526_MODP_2048_G "02" 00083 00084 #define POLARSSL_DHM_RFC3526_MODP_3072_P \ 00085 "FFFFFFFFFFFFFFFFC90FDAA22168C234C4C6628B80DC1CD1" \ 00086 "29024E088A67CC74020BBEA63B139B22514A08798E3404DD" \ 00087 "EF9519B3CD3A431B302B0A6DF25F14374FE1356D6D51C245" \ 00088 "E485B576625E7EC6F44C42E9A637ED6B0BFF5CB6F406B7ED" \ 00089 "EE386BFB5A899FA5AE9F24117C4B1FE649286651ECE45B3D" \ 00090 "C2007CB8A163BF0598DA48361C55D39A69163FA8FD24CF5F" \ 00091 "83655D23DCA3AD961C62F356208552BB9ED529077096966D" \ 00092 "670C354E4ABC9804F1746C08CA18217C32905E462E36CE3B" \ 00093 "E39E772C180E86039B2783A2EC07A28FB5C55DF06F4C52C9" \ 00094 "DE2BCBF6955817183995497CEA956AE515D2261898FA0510" \ 00095 "15728E5A8AAAC42DAD33170D04507A33A85521ABDF1CBA64" \ 00096 "ECFB850458DBEF0A8AEA71575D060C7DB3970F85A6E1E4C7" \ 00097 "ABF5AE8CDB0933D71E8C94E04A25619DCEE3D2261AD2EE6B" \ 00098 "F12FFA06D98A0864D87602733EC86A64521F2B18177B200C" \ 00099 "BBE117577A615D6C770988C0BAD946E208E24FA074E5AB31" \ 00100 "43DB5BFCE0FD108E4B82D120A93AD2CAFFFFFFFFFFFFFFFF" 00101 00102 #define POLARSSL_DHM_RFC3526_MODP_3072_G "02" 00103 00104 #define POLARSSL_DHM_RFC5114_MODP_1024_P \ 00105 "B10B8F96A080E01DDE92DE5EAE5D54EC52C99FBCFB06A3C6" \ 00106 "9A6A9DCA52D23B616073E28675A23D189838EF1E2EE652C0" \ 00107 "13ECB4AEA906112324975C3CD49B83BFACCBDD7D90C4BD70" \ 00108 "98488E9C219A73724EFFD6FAE5644738FAA31A4FF55BCCC0" \ 00109 "A151AF5F0DC8B4BD45BF37DF365C1A65E68CFDA76D4DA708" \ 00110 "DF1FB2BC2E4A4371" 00111 00112 #define POLARSSL_DHM_RFC5114_MODP_1024_G \ 00113 "A4D1CBD5C3FD34126765A442EFB99905F8104DD258AC507F" \ 00114 "D6406CFF14266D31266FEA1E5C41564B777E690F5504F213" \ 00115 "160217B4B01B886A5E91547F9E2749F4D7FBD7D3B9A92EE1" \ 00116 "909D0D2263F80A76A6A24C087A091F531DBF0A0169B6A28A" \ 00117 "D662A4D18E73AFA32D779D5918D08BC8858F4DCEF97C2A24" \ 00118 "855E6EEB22B3B2E5" 00119 00120 #define POLARSSL_DHM_RFC5114_MODP_2048_P \ 00121 "AD107E1E9123A9D0D660FAA79559C51FA20D64E5683B9FD1" \ 00122 "B54B1597B61D0A75E6FA141DF95A56DBAF9A3C407BA1DF15" \ 00123 "EB3D688A309C180E1DE6B85A1274A0A66D3F8152AD6AC212" \ 00124 "9037C9EDEFDA4DF8D91E8FEF55B7394B7AD5B7D0B6C12207" \ 00125 "C9F98D11ED34DBF6C6BA0B2C8BBC27BE6A00E0A0B9C49708" \ 00126 "B3BF8A317091883681286130BC8985DB1602E714415D9330" \ 00127 "278273C7DE31EFDC7310F7121FD5A07415987D9ADC0A486D" \ 00128 "CDF93ACC44328387315D75E198C641A480CD86A1B9E587E8" \ 00129 "BE60E69CC928B2B9C52172E413042E9B23F10B0E16E79763" \ 00130 "C9B53DCF4BA80A29E3FB73C16B8E75B97EF363E2FFA31F71" \ 00131 "CF9DE5384E71B81C0AC4DFFE0C10E64F" 00132 00133 #define POLARSSL_DHM_RFC5114_MODP_2048_G \ 00134 "AC4032EF4F2D9AE39DF30B5C8FFDAC506CDEBE7B89998CAF"\ 00135 "74866A08CFE4FFE3A6824A4E10B9A6F0DD921F01A70C4AFA"\ 00136 "AB739D7700C29F52C57DB17C620A8652BE5E9001A8D66AD7"\ 00137 "C17669101999024AF4D027275AC1348BB8A762D0521BC98A"\ 00138 "E247150422EA1ED409939D54DA7460CDB5F6C6B250717CBE"\ 00139 "F180EB34118E98D119529A45D6F834566E3025E316A330EF"\ 00140 "BB77A86F0C1AB15B051AE3D428C8F8ACB70A8137150B8EEB"\ 00141 "10E183EDD19963DDD9E263E4770589EF6AA21E7F5F2FF381"\ 00142 "B539CCE3409D13CD566AFBB48D6C019181E1BCFE94B30269"\ 00143 "EDFE72FE9B6AA4BD7B5A0F1C71CFFF4C19C418E1F6EC0179"\ 00144 "81BC087F2A7065B384B890D3191F2BFA" 00145 00146 #ifdef __cplusplus 00147 extern "C" { 00148 #endif 00149 00150 /** 00151 * \brief DHM context structure 00152 */ 00153 typedef struct 00154 { 00155 size_t len ; /*!< size(P) in chars */ 00156 mpi P ; /*!< prime modulus */ 00157 mpi G ; /*!< generator */ 00158 mpi X ; /*!< secret value */ 00159 mpi GX ; /*!< self = G^X mod P */ 00160 mpi GY ; /*!< peer = G^Y mod P */ 00161 mpi K ; /*!< key = GY^X mod P */ 00162 mpi RP ; /*!< cached R^2 mod P */ 00163 mpi Vi ; /*!< blinding value */ 00164 mpi Vf ; /*!< un-blinding value */ 00165 mpi pX ; /*!< previous X */ 00166 } 00167 dhm_context; 00168 00169 /** 00170 * \brief Initialize DHM context 00171 * 00172 * \param ctx DHM context to be initialized 00173 */ 00174 void dhm_init( dhm_context *ctx ); 00175 00176 /** 00177 * \brief Parse the ServerKeyExchange parameters 00178 * 00179 * \param ctx DHM context 00180 * \param p &(start of input buffer) 00181 * \param end end of buffer 00182 * 00183 * \return 0 if successful, or an POLARSSL_ERR_DHM_XXX error code 00184 */ 00185 int dhm_read_params( dhm_context *ctx, 00186 unsigned char **p, 00187 const unsigned char *end ); 00188 00189 /** 00190 * \brief Setup and write the ServerKeyExchange parameters 00191 * 00192 * \param ctx DHM context 00193 * \param x_size private value size in bytes 00194 * \param output destination buffer 00195 * \param olen number of chars written 00196 * \param f_rng RNG function 00197 * \param p_rng RNG parameter 00198 * 00199 * \note This function assumes that ctx->P and ctx->G 00200 * have already been properly set (for example 00201 * using mpi_read_string or mpi_read_binary). 00202 * 00203 * \return 0 if successful, or an POLARSSL_ERR_DHM_XXX error code 00204 */ 00205 int dhm_make_params( dhm_context *ctx, int x_size, 00206 unsigned char *output, size_t *olen, 00207 int (*f_rng)(void *, unsigned char *, size_t), 00208 void *p_rng ); 00209 00210 /** 00211 * \brief Import the peer's public value G^Y 00212 * 00213 * \param ctx DHM context 00214 * \param input input buffer 00215 * \param ilen size of buffer 00216 * 00217 * \return 0 if successful, or an POLARSSL_ERR_DHM_XXX error code 00218 */ 00219 int dhm_read_public( dhm_context *ctx, 00220 const unsigned char *input, size_t ilen ); 00221 00222 /** 00223 * \brief Create own private value X and export G^X 00224 * 00225 * \param ctx DHM context 00226 * \param x_size private value size in bytes 00227 * \param output destination buffer 00228 * \param olen must be equal to ctx->P.len 00229 * \param f_rng RNG function 00230 * \param p_rng RNG parameter 00231 * 00232 * \return 0 if successful, or an POLARSSL_ERR_DHM_XXX error code 00233 */ 00234 int dhm_make_public( dhm_context *ctx, int x_size, 00235 unsigned char *output, size_t olen, 00236 int (*f_rng)(void *, unsigned char *, size_t), 00237 void *p_rng ); 00238 00239 /** 00240 * \brief Derive and export the shared secret (G^Y)^X mod P 00241 * 00242 * \param ctx DHM context 00243 * \param output destination buffer 00244 * \param olen on entry, must hold the size of the destination buffer 00245 * on exit, holds the actual number of bytes written 00246 * \param f_rng RNG function, for blinding purposes 00247 * \param p_rng RNG parameter 00248 * 00249 * \return 0 if successful, or an POLARSSL_ERR_DHM_XXX error code 00250 * 00251 * \note If non-NULL, f_rng is used to blind the input as 00252 * countermeasure against timing attacks. Blinding is 00253 * automatically used if and only if our secret value X is 00254 * re-used and costs nothing otherwise, so it is recommended 00255 * to always pass a non-NULL f_rng argument. 00256 */ 00257 int dhm_calc_secret( dhm_context *ctx, 00258 unsigned char *output, size_t *olen, 00259 int (*f_rng)(void *, unsigned char *, size_t), 00260 void *p_rng ); 00261 00262 /** 00263 * \brief Free and clear the components of a DHM key 00264 * 00265 * \param ctx DHM context to free and clear 00266 */ 00267 void dhm_free( dhm_context *ctx ); 00268 00269 #if defined(POLARSSL_ASN1_PARSE_C) 00270 /** \ingroup x509_module */ 00271 /** 00272 * \brief Parse DHM parameters 00273 * 00274 * \param dhm DHM context to be initialized 00275 * \param dhmin input buffer 00276 * \param dhminlen size of the buffer 00277 * 00278 * \return 0 if successful, or a specific DHM or PEM error code 00279 */ 00280 int dhm_parse_dhm( dhm_context *dhm, const unsigned char *dhmin, 00281 size_t dhminlen ); 00282 00283 #if defined(POLARSSL_FS_IO) 00284 /** \ingroup x509_module */ 00285 /** 00286 * \brief Load and parse DHM parameters 00287 * 00288 * \param dhm DHM context to be initialized 00289 * \param path filename to read the DHM Parameters from 00290 * 00291 * \return 0 if successful, or a specific DHM or PEM error code 00292 */ 00293 int dhm_parse_dhmfile( dhm_context *dhm, const char *path ); 00294 #endif /* POLARSSL_FS_IO */ 00295 #endif /* POLARSSL_ASN1_PARSE_C */ 00296 00297 /** 00298 * \brief Checkup routine 00299 * 00300 * \return 0 if successful, or 1 if the test failed 00301 */ 00302 int dhm_self_test( int verbose ); 00303 00304 #ifdef __cplusplus 00305 } 00306 #endif 00307 00308 #endif /* dhm.h */ 00309
Generated on Tue Jul 12 2022 13:50:37 by
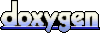