mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
asn1.h
00001 /** 00002 * \file asn1.h 00003 * 00004 * \brief Generic ASN.1 parsing 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_ASN1_H 00025 #define POLARSSL_ASN1_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(POLARSSL_BIGNUM_C) 00036 #include "bignum.h" 00037 #endif 00038 00039 /** 00040 * \addtogroup asn1_module 00041 * \{ 00042 */ 00043 00044 /** 00045 * \name ASN1 Error codes 00046 * These error codes are OR'ed to X509 error codes for 00047 * higher error granularity. 00048 * ASN1 is a standard to specify data structures. 00049 * \{ 00050 */ 00051 #define POLARSSL_ERR_ASN1_OUT_OF_DATA -0x0060 /**< Out of data when parsing an ASN1 data structure. */ 00052 #define POLARSSL_ERR_ASN1_UNEXPECTED_TAG -0x0062 /**< ASN1 tag was of an unexpected value. */ 00053 #define POLARSSL_ERR_ASN1_INVALID_LENGTH -0x0064 /**< Error when trying to determine the length or invalid length. */ 00054 #define POLARSSL_ERR_ASN1_LENGTH_MISMATCH -0x0066 /**< Actual length differs from expected length. */ 00055 #define POLARSSL_ERR_ASN1_INVALID_DATA -0x0068 /**< Data is invalid. (not used) */ 00056 #define POLARSSL_ERR_ASN1_MALLOC_FAILED -0x006A /**< Memory allocation failed */ 00057 #define POLARSSL_ERR_ASN1_BUF_TOO_SMALL -0x006C /**< Buffer too small when writing ASN.1 data structure. */ 00058 00059 /* \} name */ 00060 00061 /** 00062 * \name DER constants 00063 * These constants comply with DER encoded the ANS1 type tags. 00064 * DER encoding uses hexadecimal representation. 00065 * An example DER sequence is:\n 00066 * - 0x02 -- tag indicating INTEGER 00067 * - 0x01 -- length in octets 00068 * - 0x05 -- value 00069 * Such sequences are typically read into \c ::x509_buf. 00070 * \{ 00071 */ 00072 #define ASN1_BOOLEAN 0x01 00073 #define ASN1_INTEGER 0x02 00074 #define ASN1_BIT_STRING 0x03 00075 #define ASN1_OCTET_STRING 0x04 00076 #define ASN1_NULL 0x05 00077 #define ASN1_OID 0x06 00078 #define ASN1_UTF8_STRING 0x0C 00079 #define ASN1_SEQUENCE 0x10 00080 #define ASN1_SET 0x11 00081 #define ASN1_PRINTABLE_STRING 0x13 00082 #define ASN1_T61_STRING 0x14 00083 #define ASN1_IA5_STRING 0x16 00084 #define ASN1_UTC_TIME 0x17 00085 #define ASN1_GENERALIZED_TIME 0x18 00086 #define ASN1_UNIVERSAL_STRING 0x1C 00087 #define ASN1_BMP_STRING 0x1E 00088 #define ASN1_PRIMITIVE 0x00 00089 #define ASN1_CONSTRUCTED 0x20 00090 #define ASN1_CONTEXT_SPECIFIC 0x80 00091 /* \} name */ 00092 /* \} addtogroup asn1_module */ 00093 00094 /** Returns the size of the binary string, without the trailing \\0 */ 00095 #define OID_SIZE(x) (sizeof(x) - 1) 00096 00097 /** 00098 * Compares an asn1_buf structure to a reference OID. 00099 * 00100 * Only works for 'defined' oid_str values (OID_HMAC_SHA1), you cannot use a 00101 * 'unsigned char *oid' here! 00102 * 00103 * Warning: returns true when the OIDs are equal (unlike memcmp)! 00104 */ 00105 #define OID_CMP(oid_str, oid_buf) \ 00106 ( ( OID_SIZE(oid_str) == (oid_buf)->len ) && \ 00107 memcmp( (oid_str), (oid_buf)->p, (oid_buf)->len) == 0 ) 00108 00109 #ifdef __cplusplus 00110 extern "C" { 00111 #endif 00112 00113 /** 00114 * \name Functions to parse ASN.1 data structures 00115 * \{ 00116 */ 00117 00118 /** 00119 * Type-length-value structure that allows for ASN1 using DER. 00120 */ 00121 typedef struct _asn1_buf 00122 { 00123 int tag; /**< ASN1 type, e.g. ASN1_UTF8_STRING. */ 00124 size_t len; /**< ASN1 length, e.g. in octets. */ 00125 unsigned char *p; /**< ASN1 data, e.g. in ASCII. */ 00126 } 00127 asn1_buf; 00128 00129 /** 00130 * Container for ASN1 bit strings. 00131 */ 00132 typedef struct _asn1_bitstring 00133 { 00134 size_t len; /**< ASN1 length, e.g. in octets. */ 00135 unsigned char unused_bits; /**< Number of unused bits at the end of the string */ 00136 unsigned char *p; /**< Raw ASN1 data for the bit string */ 00137 } 00138 asn1_bitstring; 00139 00140 /** 00141 * Container for a sequence of ASN.1 items 00142 */ 00143 typedef struct _asn1_sequence 00144 { 00145 asn1_buf buf; /**< Buffer containing the given ASN.1 item. */ 00146 struct _asn1_sequence *next; /**< The next entry in the sequence. */ 00147 } 00148 asn1_sequence; 00149 00150 /** 00151 * Container for a sequence or list of 'named' ASN.1 data items 00152 */ 00153 typedef struct _asn1_named_data 00154 { 00155 asn1_buf oid; /**< The object identifier. */ 00156 asn1_buf val; /**< The named value. */ 00157 struct _asn1_named_data *next; /**< The next entry in the sequence. */ 00158 unsigned char next_merged; /**< Merge next item into the current one? */ 00159 } 00160 asn1_named_data; 00161 00162 /** 00163 * \brief Get the length of an ASN.1 element. 00164 * Updates the pointer to immediately behind the length. 00165 * 00166 * \param p The position in the ASN.1 data 00167 * \param end End of data 00168 * \param len The variable that will receive the value 00169 * 00170 * \return 0 if successful, POLARSSL_ERR_ASN1_OUT_OF_DATA on reaching 00171 * end of data, POLARSSL_ERR_ASN1_INVALID_LENGTH if length is 00172 * unparseable. 00173 */ 00174 int asn1_get_len( unsigned char **p, 00175 const unsigned char *end, 00176 size_t *len ); 00177 00178 /** 00179 * \brief Get the tag and length of the tag. Check for the requested tag. 00180 * Updates the pointer to immediately behind the tag and length. 00181 * 00182 * \param p The position in the ASN.1 data 00183 * \param end End of data 00184 * \param len The variable that will receive the length 00185 * \param tag The expected tag 00186 * 00187 * \return 0 if successful, POLARSSL_ERR_ASN1_UNEXPECTED_TAG if tag did 00188 * not match requested tag, or another specific ASN.1 error code. 00189 */ 00190 int asn1_get_tag( unsigned char **p, 00191 const unsigned char *end, 00192 size_t *len, int tag ); 00193 00194 /** 00195 * \brief Retrieve a boolean ASN.1 tag and its value. 00196 * Updates the pointer to immediately behind the full tag. 00197 * 00198 * \param p The position in the ASN.1 data 00199 * \param end End of data 00200 * \param val The variable that will receive the value 00201 * 00202 * \return 0 if successful or a specific ASN.1 error code. 00203 */ 00204 int asn1_get_bool( unsigned char **p, 00205 const unsigned char *end, 00206 int *val ); 00207 00208 /** 00209 * \brief Retrieve an integer ASN.1 tag and its value. 00210 * Updates the pointer to immediately behind the full tag. 00211 * 00212 * \param p The position in the ASN.1 data 00213 * \param end End of data 00214 * \param val The variable that will receive the value 00215 * 00216 * \return 0 if successful or a specific ASN.1 error code. 00217 */ 00218 int asn1_get_int( unsigned char **p, 00219 const unsigned char *end, 00220 int *val ); 00221 00222 /** 00223 * \brief Retrieve a bitstring ASN.1 tag and its value. 00224 * Updates the pointer to immediately behind the full tag. 00225 * 00226 * \param p The position in the ASN.1 data 00227 * \param end End of data 00228 * \param bs The variable that will receive the value 00229 * 00230 * \return 0 if successful or a specific ASN.1 error code. 00231 */ 00232 int asn1_get_bitstring( unsigned char **p, const unsigned char *end, 00233 asn1_bitstring *bs); 00234 00235 /** 00236 * \brief Retrieve a bitstring ASN.1 tag without unused bits and its 00237 * value. 00238 * Updates the pointer to the beginning of the bit/octet string. 00239 * 00240 * \param p The position in the ASN.1 data 00241 * \param end End of data 00242 * \param len Length of the actual bit/octect string in bytes 00243 * 00244 * \return 0 if successful or a specific ASN.1 error code. 00245 */ 00246 int asn1_get_bitstring_null( unsigned char **p, const unsigned char *end, 00247 size_t *len ); 00248 00249 /** 00250 * \brief Parses and splits an ASN.1 "SEQUENCE OF <tag>" 00251 * Updated the pointer to immediately behind the full sequence tag. 00252 * 00253 * \param p The position in the ASN.1 data 00254 * \param end End of data 00255 * \param cur First variable in the chain to fill 00256 * \param tag Type of sequence 00257 * 00258 * \return 0 if successful or a specific ASN.1 error code. 00259 */ 00260 int asn1_get_sequence_of( unsigned char **p, 00261 const unsigned char *end, 00262 asn1_sequence *cur, 00263 int tag); 00264 00265 #if defined(POLARSSL_BIGNUM_C) 00266 /** 00267 * \brief Retrieve a MPI value from an integer ASN.1 tag. 00268 * Updates the pointer to immediately behind the full tag. 00269 * 00270 * \param p The position in the ASN.1 data 00271 * \param end End of data 00272 * \param X The MPI that will receive the value 00273 * 00274 * \return 0 if successful or a specific ASN.1 or MPI error code. 00275 */ 00276 int asn1_get_mpi( unsigned char **p, 00277 const unsigned char *end, 00278 mpi *X ); 00279 #endif /* POLARSSL_BIGNUM_C */ 00280 00281 /** 00282 * \brief Retrieve an AlgorithmIdentifier ASN.1 sequence. 00283 * Updates the pointer to immediately behind the full 00284 * AlgorithmIdentifier. 00285 * 00286 * \param p The position in the ASN.1 data 00287 * \param end End of data 00288 * \param alg The buffer to receive the OID 00289 * \param params The buffer to receive the params (if any) 00290 * 00291 * \return 0 if successful or a specific ASN.1 or MPI error code. 00292 */ 00293 int asn1_get_alg( unsigned char **p, 00294 const unsigned char *end, 00295 asn1_buf *alg, asn1_buf *params ); 00296 00297 /** 00298 * \brief Retrieve an AlgorithmIdentifier ASN.1 sequence with NULL or no 00299 * params. 00300 * Updates the pointer to immediately behind the full 00301 * AlgorithmIdentifier. 00302 * 00303 * \param p The position in the ASN.1 data 00304 * \param end End of data 00305 * \param alg The buffer to receive the OID 00306 * 00307 * \return 0 if successful or a specific ASN.1 or MPI error code. 00308 */ 00309 int asn1_get_alg_null( unsigned char **p, 00310 const unsigned char *end, 00311 asn1_buf *alg ); 00312 00313 /** 00314 * \brief Find a specific named_data entry in a sequence or list based on 00315 * the OID. 00316 * 00317 * \param list The list to seek through 00318 * \param oid The OID to look for 00319 * \param len Size of the OID 00320 * 00321 * \return NULL if not found, or a pointer to the existing entry. 00322 */ 00323 asn1_named_data *asn1_find_named_data( asn1_named_data *list, 00324 const char *oid, size_t len ); 00325 00326 /** 00327 * \brief Free a asn1_named_data entry 00328 * 00329 * \param entry The named data entry to free 00330 */ 00331 void asn1_free_named_data( asn1_named_data *entry ); 00332 00333 /** 00334 * \brief Free all entries in a asn1_named_data list 00335 * Head will be set to NULL 00336 * 00337 * \param head Pointer to the head of the list of named data entries to free 00338 */ 00339 void asn1_free_named_data_list( asn1_named_data **head ); 00340 00341 #ifdef __cplusplus 00342 } 00343 #endif 00344 00345 #endif /* asn1.h */ 00346
Generated on Tue Jul 12 2022 13:50:36 by
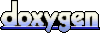