mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
rsa.h File Reference
The RSA public-key cryptosystem. More...
Go to the source code of this file.
Data Structures | |
struct | rsa_context |
RSA context structure. More... | |
Functions | |
void | rsa_init (rsa_context *ctx, int padding, int hash_id) |
Initialize an RSA context. | |
void | rsa_set_padding (rsa_context *ctx, int padding, int hash_id) |
Set padding for an already initialized RSA context See rsa_init() for details. | |
int | rsa_gen_key (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, unsigned int nbits, int exponent) |
Generate an RSA keypair. | |
int | rsa_check_pubkey (const rsa_context *ctx) |
Check a public RSA key. | |
int | rsa_check_privkey (const rsa_context *ctx) |
Check a private RSA key. | |
int | rsa_check_pub_priv (const rsa_context *pub, const rsa_context *prv) |
Check a public-private RSA key pair. | |
int | rsa_public (rsa_context *ctx, const unsigned char *input, unsigned char *output) |
Do an RSA public key operation. | |
int | rsa_private (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, const unsigned char *input, unsigned char *output) |
Do an RSA private key operation. | |
int | rsa_pkcs1_encrypt (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t ilen, const unsigned char *input, unsigned char *output) |
Generic wrapper to perform a PKCS#1 encryption using the mode from the context. | |
int | rsa_rsaes_pkcs1_v15_encrypt (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t ilen, const unsigned char *input, unsigned char *output) |
Perform a PKCS#1 v1.5 encryption (RSAES-PKCS1-v1_5-ENCRYPT) | |
int | rsa_rsaes_oaep_encrypt (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, const unsigned char *label, size_t label_len, size_t ilen, const unsigned char *input, unsigned char *output) |
Perform a PKCS#1 v2.1 OAEP encryption (RSAES-OAEP-ENCRYPT) | |
int | rsa_pkcs1_decrypt (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t *olen, const unsigned char *input, unsigned char *output, size_t output_max_len) |
Generic wrapper to perform a PKCS#1 decryption using the mode from the context. | |
int | rsa_rsaes_pkcs1_v15_decrypt (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t *olen, const unsigned char *input, unsigned char *output, size_t output_max_len) |
Perform a PKCS#1 v1.5 decryption (RSAES-PKCS1-v1_5-DECRYPT) | |
int | rsa_rsaes_oaep_decrypt (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, const unsigned char *label, size_t label_len, size_t *olen, const unsigned char *input, unsigned char *output, size_t output_max_len) |
Perform a PKCS#1 v2.1 OAEP decryption (RSAES-OAEP-DECRYPT) | |
int | rsa_pkcs1_sign (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, unsigned char *sig) |
Generic wrapper to perform a PKCS#1 signature using the mode from the context. | |
int | rsa_rsassa_pkcs1_v15_sign (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, unsigned char *sig) |
Perform a PKCS#1 v1.5 signature (RSASSA-PKCS1-v1_5-SIGN) | |
int | rsa_rsassa_pss_sign (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, unsigned char *sig) |
Perform a PKCS#1 v2.1 PSS signature (RSASSA-PSS-SIGN) | |
int | rsa_pkcs1_verify (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, const unsigned char *sig) |
Generic wrapper to perform a PKCS#1 verification using the mode from the context. | |
int | rsa_rsassa_pkcs1_v15_verify (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, const unsigned char *sig) |
Perform a PKCS#1 v1.5 verification (RSASSA-PKCS1-v1_5-VERIFY) | |
int | rsa_rsassa_pss_verify (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, const unsigned char *sig) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the "simple" version.) | |
int | rsa_rsassa_pss_verify_ext (rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, md_type_t mgf1_hash_id, int expected_salt_len, const unsigned char *sig) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the version with "full" options.) | |
int | rsa_copy (rsa_context *dst, const rsa_context *src) |
Copy the components of an RSA context. | |
void | rsa_free (rsa_context *ctx) |
Free the components of an RSA key. | |
int | rsa_self_test (int verbose) |
Checkup routine. |
Detailed Description
The RSA public-key cryptosystem.
Copyright (C) 2006-2014, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file rsa.h.
Function Documentation
int rsa_check_privkey | ( | const rsa_context * | ctx ) |
int rsa_check_pub_priv | ( | const rsa_context * | pub, |
const rsa_context * | prv | ||
) |
int rsa_check_pubkey | ( | const rsa_context * | ctx ) |
int rsa_copy | ( | rsa_context * | dst, |
const rsa_context * | src | ||
) |
void rsa_free | ( | rsa_context * | ctx ) |
int rsa_gen_key | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
unsigned int | nbits, | ||
int | exponent | ||
) |
Generate an RSA keypair.
- Parameters:
-
ctx RSA context that will hold the key f_rng RNG function p_rng RNG parameter nbits size of the public key in bits exponent public exponent (e.g., 65537)
- Note:
- rsa_init() must be called beforehand to setup the RSA context.
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
void rsa_init | ( | rsa_context * | ctx, |
int | padding, | ||
int | hash_id | ||
) |
Initialize an RSA context.
Note: Set padding to RSA_PKCS_V21 for the RSAES-OAEP encryption scheme and the RSASSA-PSS signature scheme.
- Parameters:
-
ctx RSA context to be initialized padding RSA_PKCS_V15 or RSA_PKCS_V21 hash_id RSA_PKCS_V21 hash identifier
- Note:
- The hash_id parameter is actually ignored when using RSA_PKCS_V15 padding.
- Choice of padding mode is strictly enforced for private key operations, since there might be security concerns in mixing padding modes. For public key operations it's merely a default value, which can be overriden by calling specific rsa_rsaes_xxx or rsa_rsassa_xxx functions.
- The chosen hash is always used for OEAP encryption. For PSS signatures, it's always used for making signatures, but can be overriden (and always is, if set to POLARSSL_MD_NONE) for verifying them.
int rsa_pkcs1_decrypt | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t * | olen, | ||
const unsigned char * | input, | ||
unsigned char * | output, | ||
size_t | output_max_len | ||
) |
Generic wrapper to perform a PKCS#1 decryption using the mode from the context.
Do an RSA operation, then remove the message padding
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE olen will contain the plaintext length input buffer holding the encrypted data output buffer that will hold the plaintext output_max_len maximum length of the output buffer
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise an error is thrown.
int rsa_pkcs1_encrypt | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t | ilen, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Generic wrapper to perform a PKCS#1 encryption using the mode from the context.
Add the message padding, then do an RSA operation.
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding and RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE ilen contains the plaintext length input buffer holding the data to be encrypted output buffer that will hold the ciphertext
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int rsa_pkcs1_sign | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
unsigned char * | sig | ||
) |
Generic wrapper to perform a PKCS#1 signature using the mode from the context.
Do a private RSA operation to sign a message digest
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) hashlen message digest length (for POLARSSL_MD_NONE only) hash buffer holding the message digest sig buffer that will hold the ciphertext
- Returns:
- 0 if the signing operation was successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- In case of PKCS#1 v2.1 encoding, see comments on
-
rsa_rsassa_pss_sign()
for details on md_alg and hash_id.
int rsa_pkcs1_verify | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
const unsigned char * | sig | ||
) |
Generic wrapper to perform a PKCS#1 verification using the mode from the context.
Do a public RSA operation and check the message digest
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) hashlen message digest length (for POLARSSL_MD_NONE only) hash buffer holding the message digest sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
-
In case of PKCS#1 v2.1 encoding, see comments on
rsa_rsassa_pss_verify()
about md_alg and hash_id.
int rsa_private | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Do an RSA private key operation.
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for blinding) p_rng RNG parameter input input buffer output output buffer
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The input and output buffers must be large enough (eg. 128 bytes if RSA-1024 is used).
int rsa_public | ( | rsa_context * | ctx, |
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Do an RSA public key operation.
- Parameters:
-
ctx RSA context input input buffer output output buffer
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- This function does NOT take care of message padding. Also, be sure to set input[0] = 0 or assure that input is smaller than N.
- The input and output buffers must be large enough (eg. 128 bytes if RSA-1024 is used).
int rsa_rsaes_oaep_decrypt | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
const unsigned char * | label, | ||
size_t | label_len, | ||
size_t * | olen, | ||
const unsigned char * | input, | ||
unsigned char * | output, | ||
size_t | output_max_len | ||
) |
Perform a PKCS#1 v2.1 OAEP decryption (RSAES-OAEP-DECRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE label buffer holding the custom label to use label_len contains the label length olen will contain the plaintext length input buffer holding the encrypted data output buffer that will hold the plaintext output_max_len maximum length of the output buffer
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise an error is thrown.
int rsa_rsaes_oaep_encrypt | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
const unsigned char * | label, | ||
size_t | label_len, | ||
size_t | ilen, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Perform a PKCS#1 v2.1 OAEP encryption (RSAES-OAEP-ENCRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding and RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE label buffer holding the custom label to use label_len contains the label length ilen contains the plaintext length input buffer holding the data to be encrypted output buffer that will hold the ciphertext
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int rsa_rsaes_pkcs1_v15_decrypt | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t * | olen, | ||
const unsigned char * | input, | ||
unsigned char * | output, | ||
size_t | output_max_len | ||
) |
Perform a PKCS#1 v1.5 decryption (RSAES-PKCS1-v1_5-DECRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE olen will contain the plaintext length input buffer holding the encrypted data output buffer that will hold the plaintext output_max_len maximum length of the output buffer
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise an error is thrown.
int rsa_rsaes_pkcs1_v15_encrypt | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t | ilen, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Perform a PKCS#1 v1.5 encryption (RSAES-PKCS1-v1_5-ENCRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for padding and RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE ilen contains the plaintext length input buffer holding the data to be encrypted output buffer that will hold the ciphertext
- Returns:
- 0 if successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int rsa_rsassa_pkcs1_v15_sign | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
unsigned char * | sig | ||
) |
Perform a PKCS#1 v1.5 signature (RSASSA-PKCS1-v1_5-SIGN)
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) hashlen message digest length (for POLARSSL_MD_NONE only) hash buffer holding the message digest sig buffer that will hold the ciphertext
- Returns:
- 0 if the signing operation was successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int rsa_rsassa_pkcs1_v15_verify | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
const unsigned char * | sig | ||
) |
Perform a PKCS#1 v1.5 verification (RSASSA-PKCS1-v1_5-VERIFY)
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) hashlen message digest length (for POLARSSL_MD_NONE only) hash buffer holding the message digest sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int rsa_rsassa_pss_sign | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
unsigned char * | sig | ||
) |
Perform a PKCS#1 v2.1 PSS signature (RSASSA-PSS-SIGN)
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) hashlen message digest length (for POLARSSL_MD_NONE only) hash buffer holding the message digest sig buffer that will hold the ciphertext
- Returns:
- 0 if the signing operation was successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- The hash_id in the RSA context is the one used for the encoding. md_alg in the function call is the type of hash that is encoded. According to RFC 3447 it is advised to keep both hashes the same.
int rsa_rsassa_pss_verify | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
const unsigned char * | sig | ||
) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the "simple" version.)
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) hashlen message digest length (for POLARSSL_MD_NONE only) hash buffer holding the message digest sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- The hash_id in the RSA context is the one used for the verification. md_alg in the function call is the type of hash that is verified. According to RFC 3447 it is advised to keep both hashes the same. If hash_id in the RSA context is unset, the md_alg from the function call is used.
int rsa_rsassa_pss_verify_ext | ( | rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
md_type_t | mgf1_hash_id, | ||
int | expected_salt_len, | ||
const unsigned char * | sig | ||
) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the version with "full" options.)
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for RSA_PRIVATE) p_rng RNG parameter mode RSA_PUBLIC or RSA_PRIVATE md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) hashlen message digest length (for POLARSSL_MD_NONE only) hash buffer holding the message digest mgf1_hash_id message digest used for mask generation expected_salt_len Length of the salt used in padding, use RSA_SALT_LEN_ANY to accept any salt length sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an POLARSSL_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- The hash_id in the RSA context is ignored.
int rsa_self_test | ( | int | verbose ) |
void rsa_set_padding | ( | rsa_context * | ctx, |
int | padding, | ||
int | hash_id | ||
) |
Set padding for an already initialized RSA context See rsa_init()
for details.
- Parameters:
-
ctx RSA context to be set padding RSA_PKCS_V15 or RSA_PKCS_V21 hash_id RSA_PKCS_V21 hash identifier
Generated on Tue Jul 12 2022 13:50:40 by
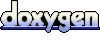