mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ecp.h File Reference
Elliptic curves over GF(p) More...
Go to the source code of this file.
Data Structures | |
struct | ecp_curve_info |
Curve information for use by other modules. More... | |
struct | ecp_point |
ECP point structure (jacobian coordinates) More... | |
struct | ecp_group |
ECP group structure. More... | |
struct | ecp_keypair |
ECP key pair structure. More... | |
Enumerations | |
enum | ecp_group_id { , POLARSSL_ECP_DP_SECP192R1, POLARSSL_ECP_DP_SECP224R1, POLARSSL_ECP_DP_SECP256R1, POLARSSL_ECP_DP_SECP384R1, POLARSSL_ECP_DP_SECP521R1, POLARSSL_ECP_DP_BP256R1, POLARSSL_ECP_DP_BP384R1, POLARSSL_ECP_DP_BP512R1, POLARSSL_ECP_DP_M221, POLARSSL_ECP_DP_M255, POLARSSL_ECP_DP_M383, POLARSSL_ECP_DP_M511, POLARSSL_ECP_DP_SECP192K1, POLARSSL_ECP_DP_SECP224K1, POLARSSL_ECP_DP_SECP256K1 } |
Domain parameters (curve, subgroup and generator) identifiers. More... | |
Functions | |
const ecp_curve_info * | ecp_curve_list (void) |
Get the list of supported curves in order of preferrence (full information) | |
const ecp_group_id * | ecp_grp_id_list (void) |
Get the list of supported curves in order of preferrence (grp_id only) | |
const ecp_curve_info * | ecp_curve_info_from_grp_id (ecp_group_id grp_id) |
Get curve information from an internal group identifier. | |
const ecp_curve_info * | ecp_curve_info_from_tls_id (uint16_t tls_id) |
Get curve information from a TLS NamedCurve value. | |
const ecp_curve_info * | ecp_curve_info_from_name (const char *name) |
Get curve information from a human-readable name. | |
void | ecp_point_init (ecp_point *pt) |
Initialize a point (as zero) | |
void | ecp_group_init (ecp_group *grp) |
Initialize a group (to something meaningless) | |
void | ecp_keypair_init (ecp_keypair *key) |
Initialize a key pair (as an invalid one) | |
void | ecp_point_free (ecp_point *pt) |
Free the components of a point. | |
void | ecp_group_free (ecp_group *grp) |
Free the components of an ECP group. | |
void | ecp_keypair_free (ecp_keypair *key) |
Free the components of a key pair. | |
int | ecp_copy (ecp_point *P, const ecp_point *Q) |
Copy the contents of point Q into P. | |
int | ecp_group_copy (ecp_group *dst, const ecp_group *src) |
Copy the contents of a group object. | |
int | ecp_set_zero (ecp_point *pt) |
Set a point to zero. | |
int | ecp_is_zero (ecp_point *pt) |
Tell if a point is zero. | |
int | ecp_point_read_string (ecp_point *P, int radix, const char *x, const char *y) |
Import a non-zero point from two ASCII strings. | |
int | ecp_point_write_binary (const ecp_group *grp, const ecp_point *P, int format, size_t *olen, unsigned char *buf, size_t buflen) |
Export a point into unsigned binary data. | |
int | ecp_point_read_binary (const ecp_group *grp, ecp_point *P, const unsigned char *buf, size_t ilen) |
Import a point from unsigned binary data. | |
int | ecp_tls_read_point (const ecp_group *grp, ecp_point *pt, const unsigned char **buf, size_t len) |
Import a point from a TLS ECPoint record. | |
int | ecp_tls_write_point (const ecp_group *grp, const ecp_point *pt, int format, size_t *olen, unsigned char *buf, size_t blen) |
Export a point as a TLS ECPoint record. | |
int | ecp_group_read_string (ecp_group *grp, int radix, const char *p, const char *b, const char *gx, const char *gy, const char *n) |
Import an ECP group from null-terminated ASCII strings. | |
int | ecp_use_known_dp (ecp_group *grp, ecp_group_id index) |
Set a group using well-known domain parameters. | |
int | ecp_tls_read_group (ecp_group *grp, const unsigned char **buf, size_t len) |
Set a group from a TLS ECParameters record. | |
int | ecp_tls_write_group (const ecp_group *grp, size_t *olen, unsigned char *buf, size_t blen) |
Write the TLS ECParameters record for a group. | |
int | ecp_add (const ecp_group *grp, ecp_point *R, const ecp_point *P, const ecp_point *Q) |
Addition: R = P + Q. | |
int | ecp_sub (const ecp_group *grp, ecp_point *R, const ecp_point *P, const ecp_point *Q) |
Subtraction: R = P - Q. | |
int | ecp_mul (ecp_group *grp, ecp_point *R, const mpi *m, const ecp_point *P, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Multiplication by an integer: R = m * P (Not thread-safe to use same group in multiple threads) | |
int | ecp_check_pubkey (const ecp_group *grp, const ecp_point *pt) |
Check that a point is a valid public key on this curve. | |
int | ecp_check_privkey (const ecp_group *grp, const mpi *d) |
Check that an mpi is a valid private key for this curve. | |
int | ecp_gen_keypair (ecp_group *grp, mpi *d, ecp_point *Q, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a keypair. | |
int | ecp_gen_key (ecp_group_id grp_id, ecp_keypair *key, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a keypair. | |
int | ecp_check_pub_priv (const ecp_keypair *pub, const ecp_keypair *prv) |
Check a public-private key pair. | |
int | ecp_self_test (int verbose) |
Checkup routine. |
Detailed Description
Elliptic curves over GF(p)
Copyright (C) 2006-2013, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file ecp.h.
Enumeration Type Documentation
enum ecp_group_id |
Domain parameters (curve, subgroup and generator) identifiers.
Only curves over prime fields are supported.
- Warning:
- This library does not support validation of arbitrary domain parameters. Therefore, only well-known domain parameters from trusted sources should be used. See ecp_use_known_dp().
- Enumerator:
Function Documentation
Check that an mpi is a valid private key for this curve.
- Parameters:
-
grp Group used d Integer to check
- Returns:
- 0 if point is a valid private key, POLARSSL_ERR_ECP_INVALID_KEY otherwise.
- Note:
- Uses bare components rather than an ecp_keypair structure in order to ease use with other structures such as ecdh_context of ecdsa_context.
int ecp_check_pub_priv | ( | const ecp_keypair * | pub, |
const ecp_keypair * | prv | ||
) |
Check a public-private key pair.
- Parameters:
-
pub Keypair structure holding a public key prv Keypair structure holding a private (plus public) key
- Returns:
- 0 if successful (keys are valid and match), or POLARSSL_ERR_ECP_BAD_INPUT_DATA, or a POLARSSL_ERR_ECP_XXX or POLARSSL_ERR_MPI_XXX code.
Check that a point is a valid public key on this curve.
- Parameters:
-
grp Curve/group the point should belong to pt Point to check
- Returns:
- 0 if point is a valid public key, POLARSSL_ERR_ECP_INVALID_KEY otherwise.
- Note:
- This function only checks the point is non-zero, has valid coordinates and lies on the curve, but not that it is indeed a multiple of G. This is additional check is more expensive, isn't required by standards, and shouldn't be necessary if the group used has a small cofactor. In particular, it is useless for the NIST groups which all have a cofactor of 1.
- Uses bare components rather than an ecp_keypair structure in order to ease use with other structures such as ecdh_context of ecdsa_context.
const ecp_curve_info* ecp_curve_info_from_grp_id | ( | ecp_group_id | grp_id ) |
const ecp_curve_info* ecp_curve_info_from_name | ( | const char * | name ) |
const ecp_curve_info* ecp_curve_info_from_tls_id | ( | uint16_t | tls_id ) |
const ecp_curve_info* ecp_curve_list | ( | void | ) |
int ecp_gen_key | ( | ecp_group_id | grp_id, |
ecp_keypair * | key, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
int ecp_gen_keypair | ( | ecp_group * | grp, |
mpi * | d, | ||
ecp_point * | Q, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Generate a keypair.
- Parameters:
-
grp ECP group d Destination MPI (secret part) Q Destination point (public part) f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful, or a POLARSSL_ERR_ECP_XXX or POLARSSL_MPI_XXX error code
- Note:
- Uses bare components rather than an ecp_keypair structure in order to ease use with other structures such as ecdh_context of ecdsa_context.
void ecp_group_free | ( | ecp_group * | grp ) |
void ecp_group_init | ( | ecp_group * | grp ) |
int ecp_group_read_string | ( | ecp_group * | grp, |
int | radix, | ||
const char * | p, | ||
const char * | b, | ||
const char * | gx, | ||
const char * | gy, | ||
const char * | n | ||
) |
Import an ECP group from null-terminated ASCII strings.
- Parameters:
-
grp Destination group radix Input numeric base p Prime modulus of the base field b Constant term in the equation gx The generator's X coordinate gy The generator's Y coordinate n The generator's order
- Returns:
- 0 if successful, or a POLARSSL_ERR_MPI_XXX error code
- Note:
- Sets all fields except modp.
const ecp_group_id* ecp_grp_id_list | ( | void | ) |
int ecp_is_zero | ( | ecp_point * | pt ) |
void ecp_keypair_free | ( | ecp_keypair * | key ) |
void ecp_keypair_init | ( | ecp_keypair * | key ) |
int ecp_mul | ( | ecp_group * | grp, |
ecp_point * | R, | ||
const mpi * | m, | ||
const ecp_point * | P, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Multiplication by an integer: R = m * P (Not thread-safe to use same group in multiple threads)
- Parameters:
-
grp ECP group R Destination point m Integer by which to multiply P Point to multiply f_rng RNG function (see notes) p_rng RNG parameter
- Returns:
- 0 if successful, POLARSSL_ERR_ECP_INVALID_KEY if m is not a valid privkey or P is not a valid pubkey, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed
- Note:
- In order to prevent timing attacks, this function executes the exact same sequence of (base field) operations for any valid m. It avoids any if-branch or array index depending on the value of m.
- If f_rng is not NULL, it is used to randomize intermediate results in order to prevent potential timing attacks targeting these results. It is recommended to always provide a non-NULL f_rng (the overhead is negligible).
void ecp_point_free | ( | ecp_point * | pt ) |
void ecp_point_init | ( | ecp_point * | pt ) |
int ecp_point_read_binary | ( | const ecp_group * | grp, |
ecp_point * | P, | ||
const unsigned char * | buf, | ||
size_t | ilen | ||
) |
Import a point from unsigned binary data.
- Parameters:
-
grp Group to which the point should belong P Point to import buf Input buffer ilen Actual length of input
- Returns:
- 0 if successful, POLARSSL_ERR_ECP_BAD_INPUT_DATA if input is invalid, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_ECP_FEATURE_UNAVAILABLE if the point format is not implemented.
- Note:
- This function does NOT check that the point actually belongs to the given group, see ecp_check_pubkey() for that.
int ecp_point_read_string | ( | ecp_point * | P, |
int | radix, | ||
const char * | x, | ||
const char * | y | ||
) |
Import a non-zero point from two ASCII strings.
- Parameters:
-
P Destination point radix Input numeric base x First affine coordinate as a null-terminated string y Second affine coordinate as a null-terminated string
- Returns:
- 0 if successful, or a POLARSSL_ERR_MPI_XXX error code
int ecp_point_write_binary | ( | const ecp_group * | grp, |
const ecp_point * | P, | ||
int | format, | ||
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | buflen | ||
) |
Export a point into unsigned binary data.
- Parameters:
-
grp Group to which the point should belong P Point to export format Point format, should be a POLARSSL_ECP_PF_XXX macro olen Length of the actual output buf Output buffer buflen Length of the output buffer
- Returns:
- 0 if successful, or POLARSSL_ERR_ECP_BAD_INPUT_DATA or POLARSSL_ERR_ECP_BUFFER_TOO_SMALL
int ecp_self_test | ( | int | verbose ) |
int ecp_set_zero | ( | ecp_point * | pt ) |
Subtraction: R = P - Q.
- Parameters:
-
grp ECP group R Destination point P Left-hand point Q Right-hand point
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed
- Note:
- This function does not support Montgomery curves, such as Curve25519.
int ecp_tls_read_group | ( | ecp_group * | grp, |
const unsigned char ** | buf, | ||
size_t | len | ||
) |
Set a group from a TLS ECParameters record.
- Parameters:
-
grp Destination group buf &(Start of input buffer) len Buffer length
- Note:
- buf is updated to point right after ECParameters on exit
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_XXX if initialization failed POLARSSL_ERR_ECP_BAD_INPUT_DATA if input is invalid
int ecp_tls_read_point | ( | const ecp_group * | grp, |
ecp_point * | pt, | ||
const unsigned char ** | buf, | ||
size_t | len | ||
) |
Import a point from a TLS ECPoint record.
- Parameters:
-
grp ECP group used pt Destination point buf $(Start of input buffer) len Buffer length
- Note:
- buf is updated to point right after the ECPoint on exit
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_XXX if initialization failed POLARSSL_ERR_ECP_BAD_INPUT_DATA if input is invalid
int ecp_tls_write_group | ( | const ecp_group * | grp, |
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | blen | ||
) |
int ecp_tls_write_point | ( | const ecp_group * | grp, |
const ecp_point * | pt, | ||
int | format, | ||
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | blen | ||
) |
Export a point as a TLS ECPoint record.
- Parameters:
-
grp ECP group used pt Point to export format Export format olen length of data written buf Buffer to write to blen Buffer length
- Returns:
- 0 if successful, or POLARSSL_ERR_ECP_BAD_INPUT_DATA or POLARSSL_ERR_ECP_BUFFER_TOO_SMALL
int ecp_use_known_dp | ( | ecp_group * | grp, |
ecp_group_id | index | ||
) |
Set a group using well-known domain parameters.
- Parameters:
-
grp Destination group index Index in the list of well-known domain parameters
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_XXX if initialization failed POLARSSL_ERR_ECP_FEATURE_UNAVAILABLE for unkownn groups
- Note:
- Index should be a value of RFC 4492's enum NamdeCurve, possibly in the form of a POLARSSL_ECP_DP_XXX macro.
Definition at line 722 of file ecp_curves.c.
Generated on Tue Jul 12 2022 13:50:39 by
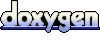