mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
bignum.h File Reference
Multi-precision integer library. More...
Go to the source code of this file.
Data Structures | |
struct | mpi |
MPI structure. More... | |
Functions | |
void | mpi_init (mpi *X) |
Initialize one MPI (make internal references valid) This just makes it ready to be set or freed, but does not define a value for the MPI. | |
void | mpi_free (mpi *X) |
Unallocate one MPI. | |
int | mpi_grow (mpi *X, size_t nblimbs) |
Enlarge to the specified number of limbs. | |
int | mpi_shrink (mpi *X, size_t nblimbs) |
Resize down, keeping at least the specified number of limbs. | |
int | mpi_copy (mpi *X, const mpi *Y) |
Copy the contents of Y into X. | |
void | mpi_swap (mpi *X, mpi *Y) |
Swap the contents of X and Y. | |
int | mpi_safe_cond_assign (mpi *X, const mpi *Y, unsigned char assign) |
Safe conditional assignement X = Y if assign is 1. | |
int | mpi_safe_cond_swap (mpi *X, mpi *Y, unsigned char assign) |
Safe conditional swap X <-> Y if swap is 1. | |
int | mpi_lset (mpi *X, t_sint z) |
Set value from integer. | |
int | mpi_get_bit (const mpi *X, size_t pos) |
Get a specific bit from X. | |
int | mpi_set_bit (mpi *X, size_t pos, unsigned char val) |
Set a bit of X to a specific value of 0 or 1. | |
size_t | mpi_lsb (const mpi *X) |
Return the number of zero-bits before the least significant '1' bit. | |
size_t | mpi_msb (const mpi *X) |
Return the number of bits up to and including the most significant '1' bit'. | |
size_t | mpi_size (const mpi *X) |
Return the total size in bytes. | |
int | mpi_read_string (mpi *X, int radix, const char *s) |
Import from an ASCII string. | |
int | mpi_write_string (const mpi *X, int radix, char *s, size_t *slen) |
Export into an ASCII string. | |
int | mpi_read_file (mpi *X, int radix, FILE *fin) |
Read X from an opened file. | |
int | mpi_write_file (const char *p, const mpi *X, int radix, FILE *fout) |
Write X into an opened file, or stdout if fout is NULL. | |
int | mpi_read_binary (mpi *X, const unsigned char *buf, size_t buflen) |
Import X from unsigned binary data, big endian. | |
int | mpi_write_binary (const mpi *X, unsigned char *buf, size_t buflen) |
Export X into unsigned binary data, big endian. | |
int | mpi_shift_l (mpi *X, size_t count) |
Left-shift: X <<= count. | |
int | mpi_shift_r (mpi *X, size_t count) |
Right-shift: X >>= count. | |
int | mpi_cmp_abs (const mpi *X, const mpi *Y) |
Compare unsigned values. | |
int | mpi_cmp_mpi (const mpi *X, const mpi *Y) |
Compare signed values. | |
int | mpi_cmp_int (const mpi *X, t_sint z) |
Compare signed values. | |
int | mpi_add_abs (mpi *X, const mpi *A, const mpi *B) |
Unsigned addition: X = |A| + |B|. | |
int | mpi_sub_abs (mpi *X, const mpi *A, const mpi *B) |
Unsigned subtraction: X = |A| - |B|. | |
int | mpi_add_mpi (mpi *X, const mpi *A, const mpi *B) |
Signed addition: X = A + B. | |
int | mpi_sub_mpi (mpi *X, const mpi *A, const mpi *B) |
Signed subtraction: X = A - B. | |
int | mpi_add_int (mpi *X, const mpi *A, t_sint b) |
Signed addition: X = A + b. | |
int | mpi_sub_int (mpi *X, const mpi *A, t_sint b) |
Signed subtraction: X = A - b. | |
int | mpi_mul_mpi (mpi *X, const mpi *A, const mpi *B) |
Baseline multiplication: X = A * B. | |
int | mpi_mul_int (mpi *X, const mpi *A, t_sint b) |
Baseline multiplication: X = A * b Note: despite the functon signature, b is treated as a t_uint. | |
int | mpi_div_mpi (mpi *Q, mpi *R, const mpi *A, const mpi *B) |
Division by mpi: A = Q * B + R. | |
int | mpi_div_int (mpi *Q, mpi *R, const mpi *A, t_sint b) |
Division by int: A = Q * b + R. | |
int | mpi_mod_mpi (mpi *R, const mpi *A, const mpi *B) |
Modulo: R = A mod B. | |
int | mpi_mod_int (t_uint *r, const mpi *A, t_sint b) |
Modulo: r = A mod b. | |
int | mpi_exp_mod (mpi *X, const mpi *A, const mpi *E, const mpi *N, mpi *_RR) |
Sliding-window exponentiation: X = A^E mod N. | |
int | mpi_fill_random (mpi *X, size_t size, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Fill an MPI X with size bytes of random. | |
int | mpi_gcd (mpi *G, const mpi *A, const mpi *B) |
Greatest common divisor: G = gcd(A, B) | |
int | mpi_inv_mod (mpi *X, const mpi *A, const mpi *N) |
Modular inverse: X = A^-1 mod N. | |
int | mpi_is_prime (mpi *X, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Miller-Rabin primality test. | |
int | mpi_gen_prime (mpi *X, size_t nbits, int dh_flag, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Prime number generation. | |
int | mpi_self_test (int verbose) |
Checkup routine. |
Detailed Description
Multi-precision integer library.
Copyright (C) 2006-2014, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file bignum.h.
Function Documentation
int mpi_cmp_int | ( | const mpi * | X, |
t_sint | z | ||
) |
Division by int: A = Q * b + R.
- Parameters:
-
Q Destination MPI for the quotient R Destination MPI for the rest value A Left-hand MPI b Integer to divide by
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_DIVISION_BY_ZERO if b == 0
- Note:
- Either Q or R can be NULL.
Division by mpi: A = Q * B + R.
- Parameters:
-
Q Destination MPI for the quotient R Destination MPI for the rest value A Left-hand MPI B Right-hand MPI
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_DIVISION_BY_ZERO if B == 0
- Note:
- Either Q or R can be NULL.
Sliding-window exponentiation: X = A^E mod N.
- Parameters:
-
X Destination MPI A Left-hand MPI E Exponent MPI N Modular MPI _RR Speed-up MPI used for recalculations
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_BAD_INPUT_DATA if N is negative or even or if E is negative
- Note:
- _RR is used to avoid re-computing R*R mod N across multiple calls, which speeds up things a bit. It can be set to NULL if the extra performance is unneeded.
int mpi_fill_random | ( | mpi * | X, |
size_t | size, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
void mpi_free | ( | mpi * | X ) |
int mpi_gen_prime | ( | mpi * | X, |
size_t | nbits, | ||
int | dh_flag, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Prime number generation.
- Parameters:
-
X Destination MPI nbits Required size of X in bits ( 3 <= nbits <= POLARSSL_MPI_MAX_BITS ) dh_flag If 1, then (X-1)/2 will be prime too f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful (probably prime), POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_BAD_INPUT_DATA if nbits is < 3
int mpi_get_bit | ( | const mpi * | X, |
size_t | pos | ||
) |
int mpi_grow | ( | mpi * | X, |
size_t | nblimbs | ||
) |
void mpi_init | ( | mpi * | X ) |
Modular inverse: X = A^-1 mod N.
- Parameters:
-
X Destination MPI A Left-hand MPI N Right-hand MPI
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_BAD_INPUT_DATA if N is negative or nil POLARSSL_ERR_MPI_NOT_ACCEPTABLE if A has no inverse mod N
int mpi_is_prime | ( | mpi * | X, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
size_t mpi_lsb | ( | const mpi * | X ) |
int mpi_lset | ( | mpi * | X, |
t_sint | z | ||
) |
int mpi_mod_int | ( | t_uint * | r, |
const mpi * | A, | ||
t_sint | b | ||
) |
Modulo: r = A mod b.
- Parameters:
-
r Destination t_uint A Left-hand MPI b Integer to divide by
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_DIVISION_BY_ZERO if b == 0, POLARSSL_ERR_MPI_NEGATIVE_VALUE if b < 0
Modulo: R = A mod B.
- Parameters:
-
R Destination MPI for the rest value A Left-hand MPI B Right-hand MPI
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_DIVISION_BY_ZERO if B == 0, POLARSSL_ERR_MPI_NEGATIVE_VALUE if B < 0
size_t mpi_msb | ( | const mpi * | X ) |
Baseline multiplication: X = A * b Note: despite the functon signature, b is treated as a t_uint.
Negative values of b are treated as large positive values.
- Parameters:
-
X Destination MPI A Left-hand MPI b The integer value to multiply with
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed
int mpi_read_binary | ( | mpi * | X, |
const unsigned char * | buf, | ||
size_t | buflen | ||
) |
int mpi_read_file | ( | mpi * | X, |
int | radix, | ||
FILE * | fin | ||
) |
int mpi_read_string | ( | mpi * | X, |
int | radix, | ||
const char * | s | ||
) |
Safe conditional assignement X = Y if assign is 1.
- Parameters:
-
X MPI to conditionally assign to Y Value to be assigned assign 1: perform the assignment, 0: keep X's original value
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed,
- Note:
- This function is equivalent to if( assign ) mpi_copy( X, Y ); except that it avoids leaking any information about whether the assignment was done or not (the above code may leak information through branch prediction and/or memory access patterns analysis).
Safe conditional swap X <-> Y if swap is 1.
- Parameters:
-
X First mpi value Y Second mpi value assign 1: perform the swap, 0: keep X and Y's original values
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed,
- Note:
- This function is equivalent to if( assign ) mpi_swap( X, Y ); except that it avoids leaking any information about whether the assignment was done or not (the above code may leak information through branch prediction and/or memory access patterns analysis).
int mpi_self_test | ( | int | verbose ) |
int mpi_set_bit | ( | mpi * | X, |
size_t | pos, | ||
unsigned char | val | ||
) |
Set a bit of X to a specific value of 0 or 1.
- Note:
- Will grow X if necessary to set a bit to 1 in a not yet existing limb. Will not grow if bit should be set to 0
- Parameters:
-
X MPI to use pos Zero-based index of the bit in X val The value to set the bit to (0 or 1)
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_MALLOC_FAILED if memory allocation failed, POLARSSL_ERR_MPI_BAD_INPUT_DATA if val is not 0 or 1
int mpi_shift_l | ( | mpi * | X, |
size_t | count | ||
) |
int mpi_shift_r | ( | mpi * | X, |
size_t | count | ||
) |
int mpi_shrink | ( | mpi * | X, |
size_t | nblimbs | ||
) |
size_t mpi_size | ( | const mpi * | X ) |
int mpi_write_binary | ( | const mpi * | X, |
unsigned char * | buf, | ||
size_t | buflen | ||
) |
Export X into unsigned binary data, big endian.
Always fills the whole buffer, which will start with zeros if the number is smaller.
- Parameters:
-
X Source MPI buf Output buffer buflen Output buffer size
- Returns:
- 0 if successful, POLARSSL_ERR_MPI_BUFFER_TOO_SMALL if buf isn't large enough
int mpi_write_file | ( | const char * | p, |
const mpi * | X, | ||
int | radix, | ||
FILE * | fout | ||
) |
Write X into an opened file, or stdout if fout is NULL.
- Parameters:
-
p Prefix, can be NULL X Source MPI radix Output numeric base fout Output file handle (can be NULL)
- Returns:
- 0 if successful, or a POLARSSL_ERR_MPI_XXX error code
- Note:
- Set fout == NULL to print X on the console.
int mpi_write_string | ( | const mpi * | X, |
int | radix, | ||
char * | s, | ||
size_t * | slen | ||
) |
Export into an ASCII string.
- Parameters:
-
X Source MPI radix Output numeric base s String buffer slen String buffer size
- Returns:
- 0 if successful, or a POLARSSL_ERR_MPI_XXX error code. *slen is always updated to reflect the amount of data that has (or would have) been written.
- Note:
- Call this function with *slen = 0 to obtain the minimum required buffer size in *slen.
Generated on Tue Jul 12 2022 13:50:39 by
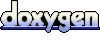