mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
asn1write.h File Reference
ASN.1 buffer writing functionality. More...
Go to the source code of this file.
Functions | |
int | asn1_write_len (unsigned char **p, unsigned char *start, size_t len) |
Write a length field in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_tag (unsigned char **p, unsigned char *start, unsigned char tag) |
Write a ASN.1 tag in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_raw_buffer (unsigned char **p, unsigned char *start, const unsigned char *buf, size_t size) |
Write raw buffer data Note: function works backwards in data buffer. | |
int | asn1_write_mpi (unsigned char **p, unsigned char *start, mpi *X) |
Write a big number (ASN1_INTEGER) in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_null (unsigned char **p, unsigned char *start) |
Write a NULL tag (ASN1_NULL) with zero data in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_oid (unsigned char **p, unsigned char *start, const char *oid, size_t oid_len) |
Write an OID tag (ASN1_OID) and data in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_algorithm_identifier (unsigned char **p, unsigned char *start, const char *oid, size_t oid_len, size_t par_len) |
Write an AlgorithmIdentifier sequence in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_bool (unsigned char **p, unsigned char *start, int boolean) |
Write a boolean tag (ASN1_BOOLEAN) and value in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_int (unsigned char **p, unsigned char *start, int val) |
Write an int tag (ASN1_INTEGER) and value in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_printable_string (unsigned char **p, unsigned char *start, const char *text, size_t text_len) |
Write a printable string tag (ASN1_PRINTABLE_STRING) and value in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_ia5_string (unsigned char **p, unsigned char *start, const char *text, size_t text_len) |
Write an IA5 string tag (ASN1_IA5_STRING) and value in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_bitstring (unsigned char **p, unsigned char *start, const unsigned char *buf, size_t bits) |
Write a bitstring tag (ASN1_BIT_STRING) and value in ASN.1 format Note: function works backwards in data buffer. | |
int | asn1_write_octet_string (unsigned char **p, unsigned char *start, const unsigned char *buf, size_t size) |
Write an octet string tag (ASN1_OCTET_STRING) and value in ASN.1 format Note: function works backwards in data buffer. | |
asn1_named_data * | asn1_store_named_data (asn1_named_data **list, const char *oid, size_t oid_len, const unsigned char *val, size_t val_len) |
Create or find a specific named_data entry for writing in a sequence or list based on the OID. |
Detailed Description
ASN.1 buffer writing functionality.
Copyright (C) 2006-2014, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file asn1write.h.
Function Documentation
asn1_named_data* asn1_store_named_data | ( | asn1_named_data ** | list, |
const char * | oid, | ||
size_t | oid_len, | ||
const unsigned char * | val, | ||
size_t | val_len | ||
) |
Create or find a specific named_data entry for writing in a sequence or list based on the OID.
If not already in there, a new entry is added to the head of the list. Warning: Destructive behaviour for the val data!
- Parameters:
-
list Pointer to the location of the head of the list to seek through (will be updated in case of a new entry) oid The OID to look for oid_len Size of the OID val Data to store (can be NULL if you want to fill it by hand) val_len Minimum length of the data buffer needed
- Returns:
- NULL if if there was a memory allocation error, or a pointer to the new / existing entry.
Definition at line 305 of file asn1write.c.
int asn1_write_algorithm_identifier | ( | unsigned char ** | p, |
unsigned char * | start, | ||
const char * | oid, | ||
size_t | oid_len, | ||
size_t | par_len | ||
) |
Write an AlgorithmIdentifier sequence in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) oid the OID of the algorithm oid_len length of the OID par_len length of parameters, which must be already written. If 0, NULL parameters are added
- Returns:
- the length written or a negative error code
Definition at line 166 of file asn1write.c.
int asn1_write_bitstring | ( | unsigned char ** | p, |
unsigned char * | start, | ||
const unsigned char * | buf, | ||
size_t | bits | ||
) |
Write a bitstring tag (ASN1_BIT_STRING) and value in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) buf the bitstring bits the total number of bits in the bitstring
- Returns:
- the length written or a negative error code
Definition at line 264 of file asn1write.c.
int asn1_write_bool | ( | unsigned char ** | p, |
unsigned char * | start, | ||
int | boolean | ||
) |
Write a boolean tag (ASN1_BOOLEAN) and value in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) boolean 0 or 1
- Returns:
- the length written or a negative error code
Definition at line 187 of file asn1write.c.
int asn1_write_ia5_string | ( | unsigned char ** | p, |
unsigned char * | start, | ||
const char * | text, | ||
size_t | text_len | ||
) |
Write an IA5 string tag (ASN1_IA5_STRING) and value in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) text the text to write text_len length of the text
- Returns:
- the length written or a negative error code
Definition at line 249 of file asn1write.c.
int asn1_write_int | ( | unsigned char ** | p, |
unsigned char * | start, | ||
int | val | ||
) |
Write an int tag (ASN1_INTEGER) and value in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) val the integer value
- Returns:
- the length written or a negative error code
Definition at line 204 of file asn1write.c.
int asn1_write_len | ( | unsigned char ** | p, |
unsigned char * | start, | ||
size_t | len | ||
) |
Write a length field in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) len the length to write
- Returns:
- the length written or a negative error code
Definition at line 43 of file asn1write.c.
int asn1_write_mpi | ( | unsigned char ** | p, |
unsigned char * | start, | ||
mpi * | X | ||
) |
Write a big number (ASN1_INTEGER) in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) X the MPI to write
- Returns:
- the length written or a negative error code
Definition at line 102 of file asn1write.c.
int asn1_write_null | ( | unsigned char ** | p, |
unsigned char * | start | ||
) |
Write a NULL tag (ASN1_NULL) with zero data in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking)
- Returns:
- the length written or a negative error code
Definition at line 139 of file asn1write.c.
int asn1_write_octet_string | ( | unsigned char ** | p, |
unsigned char * | start, | ||
const unsigned char * | buf, | ||
size_t | size | ||
) |
Write an octet string tag (ASN1_OCTET_STRING) and value in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) buf data buffer to write size length of the data buffer
- Returns:
- the length written or a negative error code
Definition at line 291 of file asn1write.c.
int asn1_write_oid | ( | unsigned char ** | p, |
unsigned char * | start, | ||
const char * | oid, | ||
size_t | oid_len | ||
) |
Write an OID tag (ASN1_OID) and data in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) oid the OID to write oid_len length of the OID
- Returns:
- the length written or a negative error code
Definition at line 152 of file asn1write.c.
int asn1_write_printable_string | ( | unsigned char ** | p, |
unsigned char * | start, | ||
const char * | text, | ||
size_t | text_len | ||
) |
Write a printable string tag (ASN1_PRINTABLE_STRING) and value in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) text the text to write text_len length of the text
- Returns:
- the length written or a negative error code
Definition at line 234 of file asn1write.c.
int asn1_write_raw_buffer | ( | unsigned char ** | p, |
unsigned char * | start, | ||
const unsigned char * | buf, | ||
size_t | size | ||
) |
Write raw buffer data Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) buf data buffer to write size length of the data buffer
- Returns:
- the length written or a negative error code
Definition at line 86 of file asn1write.c.
int asn1_write_tag | ( | unsigned char ** | p, |
unsigned char * | start, | ||
unsigned char | tag | ||
) |
Write a ASN.1 tag in ASN.1 format Note: function works backwards in data buffer.
- Parameters:
-
p reference to current position pointer start start of the buffer (for bounds-checking) tag the tag to write
- Returns:
- the length written or a negative error code
Definition at line 76 of file asn1write.c.
Generated on Tue Jul 12 2022 13:50:39 by
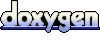