mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
asn1.h File Reference
Generic ASN.1 parsing. More...
Go to the source code of this file.
Data Structures | |
struct | _asn1_buf |
Type-length-value structure that allows for ASN1 using DER. More... | |
struct | _asn1_bitstring |
Container for ASN1 bit strings. More... | |
struct | _asn1_sequence |
Container for a sequence of ASN.1 items. More... | |
struct | _asn1_named_data |
Container for a sequence or list of 'named' ASN.1 data items. More... | |
Functions to parse ASN.1 data structures | |
typedef struct _asn1_buf | asn1_buf |
Type-length-value structure that allows for ASN1 using DER. | |
typedef struct _asn1_bitstring | asn1_bitstring |
Container for ASN1 bit strings. | |
typedef struct _asn1_sequence | asn1_sequence |
Container for a sequence of ASN.1 items. | |
typedef struct _asn1_named_data | asn1_named_data |
Container for a sequence or list of 'named' ASN.1 data items. | |
int | asn1_get_len (unsigned char **p, const unsigned char *end, size_t *len) |
Get the length of an ASN.1 element. | |
int | asn1_get_tag (unsigned char **p, const unsigned char *end, size_t *len, int tag) |
Get the tag and length of the tag. | |
int | asn1_get_bool (unsigned char **p, const unsigned char *end, int *val) |
Retrieve a boolean ASN.1 tag and its value. | |
int | asn1_get_int (unsigned char **p, const unsigned char *end, int *val) |
Retrieve an integer ASN.1 tag and its value. | |
int | asn1_get_bitstring (unsigned char **p, const unsigned char *end, asn1_bitstring *bs) |
Retrieve a bitstring ASN.1 tag and its value. | |
int | asn1_get_bitstring_null (unsigned char **p, const unsigned char *end, size_t *len) |
Retrieve a bitstring ASN.1 tag without unused bits and its value. | |
int | asn1_get_sequence_of (unsigned char **p, const unsigned char *end, asn1_sequence *cur, int tag) |
Parses and splits an ASN.1 "SEQUENCE OF <tag>" Updated the pointer to immediately behind the full sequence tag. | |
int | asn1_get_mpi (unsigned char **p, const unsigned char *end, mpi *X) |
Retrieve a MPI value from an integer ASN.1 tag. | |
int | asn1_get_alg (unsigned char **p, const unsigned char *end, asn1_buf *alg, asn1_buf *params) |
Retrieve an AlgorithmIdentifier ASN.1 sequence. | |
int | asn1_get_alg_null (unsigned char **p, const unsigned char *end, asn1_buf *alg) |
Retrieve an AlgorithmIdentifier ASN.1 sequence with NULL or no params. | |
asn1_named_data * | asn1_find_named_data (asn1_named_data *list, const char *oid, size_t len) |
Find a specific named_data entry in a sequence or list based on the OID. | |
void | asn1_free_named_data (asn1_named_data *entry) |
Free a asn1_named_data entry. | |
void | asn1_free_named_data_list (asn1_named_data **head) |
Free all entries in a asn1_named_data list Head will be set to NULL. |
Detailed Description
Generic ASN.1 parsing.
Copyright (C) 2006-2013, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file asn1.h.
Typedef Documentation
typedef struct _asn1_bitstring asn1_bitstring |
Container for ASN1 bit strings.
typedef struct _asn1_named_data asn1_named_data |
Container for a sequence or list of 'named' ASN.1 data items.
typedef struct _asn1_sequence asn1_sequence |
Container for a sequence of ASN.1 items.
Function Documentation
asn1_named_data* asn1_find_named_data | ( | asn1_named_data * | list, |
const char * | oid, | ||
size_t | len | ||
) |
Find a specific named_data entry in a sequence or list based on the OID.
- Parameters:
-
list The list to seek through oid The OID to look for len Size of the OID
- Returns:
- NULL if not found, or a pointer to the existing entry.
Definition at line 378 of file asn1parse.c.
void asn1_free_named_data | ( | asn1_named_data * | entry ) |
Free a asn1_named_data entry.
- Parameters:
-
entry The named data entry to free
Definition at line 355 of file asn1parse.c.
void asn1_free_named_data_list | ( | asn1_named_data ** | head ) |
Free all entries in a asn1_named_data list Head will be set to NULL.
- Parameters:
-
head Pointer to the head of the list of named data entries to free
Definition at line 366 of file asn1parse.c.
int asn1_get_alg | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
asn1_buf * | alg, | ||
asn1_buf * | params | ||
) |
Retrieve an AlgorithmIdentifier ASN.1 sequence.
Updates the pointer to immediately behind the full AlgorithmIdentifier.
- Parameters:
-
p The position in the ASN.1 data end End of data alg The buffer to receive the OID params The buffer to receive the params (if any)
- Returns:
- 0 if successful or a specific ASN.1 or MPI error code.
Definition at line 293 of file asn1parse.c.
int asn1_get_alg_null | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
asn1_buf * | alg | ||
) |
Retrieve an AlgorithmIdentifier ASN.1 sequence with NULL or no params.
Updates the pointer to immediately behind the full AlgorithmIdentifier.
- Parameters:
-
p The position in the ASN.1 data end End of data alg The buffer to receive the OID
- Returns:
- 0 if successful or a specific ASN.1 or MPI error code.
Definition at line 337 of file asn1parse.c.
int asn1_get_bitstring | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
asn1_bitstring * | bs | ||
) |
Retrieve a bitstring ASN.1 tag and its value.
Updates the pointer to immediately behind the full tag.
- Parameters:
-
p The position in the ASN.1 data end End of data bs The variable that will receive the value
- Returns:
- 0 if successful or a specific ASN.1 error code.
Definition at line 190 of file asn1parse.c.
int asn1_get_bitstring_null | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
size_t * | len | ||
) |
Retrieve a bitstring ASN.1 tag without unused bits and its value.
Updates the pointer to the beginning of the bit/octet string.
- Parameters:
-
p The position in the ASN.1 data end End of data len Length of the actual bit/octect string in bytes
- Returns:
- 0 if successful or a specific ASN.1 error code.
Definition at line 223 of file asn1parse.c.
int asn1_get_bool | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
int * | val | ||
) |
Retrieve a boolean ASN.1 tag and its value.
Updates the pointer to immediately behind the full tag.
- Parameters:
-
p The position in the ASN.1 data end End of data val The variable that will receive the value
- Returns:
- 0 if successful or a specific ASN.1 error code.
Definition at line 128 of file asn1parse.c.
int asn1_get_int | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
int * | val | ||
) |
Retrieve an integer ASN.1 tag and its value.
Updates the pointer to immediately behind the full tag.
- Parameters:
-
p The position in the ASN.1 data end End of data val The variable that will receive the value
- Returns:
- 0 if successful or a specific ASN.1 error code.
Definition at line 147 of file asn1parse.c.
int asn1_get_len | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
size_t * | len | ||
) |
Get the length of an ASN.1 element.
Updates the pointer to immediately behind the length.
- Parameters:
-
p The position in the ASN.1 data end End of data len The variable that will receive the value
- Returns:
- 0 if successful, POLARSSL_ERR_ASN1_OUT_OF_DATA on reaching end of data, POLARSSL_ERR_ASN1_INVALID_LENGTH if length is unparseable.
Definition at line 55 of file asn1parse.c.
int asn1_get_mpi | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
mpi * | X | ||
) |
Retrieve a MPI value from an integer ASN.1 tag.
Updates the pointer to immediately behind the full tag.
- Parameters:
-
p The position in the ASN.1 data end End of data X The MPI that will receive the value
- Returns:
- 0 if successful or a specific ASN.1 or MPI error code.
Definition at line 172 of file asn1parse.c.
int asn1_get_sequence_of | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
asn1_sequence * | cur, | ||
int | tag | ||
) |
Parses and splits an ASN.1 "SEQUENCE OF <tag>" Updated the pointer to immediately behind the full sequence tag.
- Parameters:
-
p The position in the ASN.1 data end End of data cur First variable in the chain to fill tag Type of sequence
- Returns:
- 0 if successful or a specific ASN.1 error code.
Definition at line 242 of file asn1parse.c.
int asn1_get_tag | ( | unsigned char ** | p, |
const unsigned char * | end, | ||
size_t * | len, | ||
int | tag | ||
) |
Get the tag and length of the tag.
Check for the requested tag. Updates the pointer to immediately behind the tag and length.
- Parameters:
-
p The position in the ASN.1 data end End of data len The variable that will receive the length tag The expected tag
- Returns:
- 0 if successful, POLARSSL_ERR_ASN1_UNEXPECTED_TAG if tag did not match requested tag, or another specific ASN.1 error code.
Definition at line 113 of file asn1parse.c.
Generated on Tue Jul 12 2022 13:50:39 by
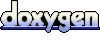