BLE mbed Endpoint network stack for mbedConnectorInterface. The stack makes use of a special BLE Socket abstraction to create socket() semantics over BLE.
Dependencies: libnsdl_m0 BLE_API Base64 nRF51822 SplitterAssembler
BLELocation.cpp
00001 /** 00002 * @file BLEBLEBLELocation.cpp 00003 * @brief mbed CoAP Endpoint BLE BLELocation class 00004 * @author Doug Anson/Chris Paola 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #include "BLELocation.h" 00024 00025 // UartRPCFunctions 00026 #include "UartRPCFunctions.h" 00027 00028 #ifdef DBG 00029 #undef DBG 00030 #endif 00031 #define DBG std::printf 00032 00033 // Constructor 00034 BLELocation::BLELocation(const RawSerial *pc) : Connector::Location(pc) { 00035 } 00036 00037 // Destructor 00038 BLELocation::~BLELocation() { 00039 } 00040 00041 // dispatch our location update request 00042 void BLELocation::dispatchUpdateRequest() { 00043 //this->m_pc->printf("BLELocation:dispatchUpdateRequest: dispatching location update request...\r\n"); 00044 ble_rpc_get_location(this); 00045 } 00046 00047 // set the default location 00048 void BLELocation::setDefault(float latitude,float longitude,float altitude,float speed) { 00049 sprintf(this->m_latitude,"%.5f",latitude); 00050 sprintf(this->m_longitude,"%.5f",longitude); 00051 sprintf(this->m_msl_altitude_m,"%.1f",altitude); 00052 sprintf(this->m_speed,"%.1f",speed); 00053 } 00054 00055 // process the location update response 00056 void BLELocation::processLocationUpdateResponse(char *response,int response_length) { 00057 float latitude = 0.0; 00058 float longitude = 0.0; 00059 float altitude = 0.0; 00060 float speed = 0.0; 00061 bool present = false; 00062 00063 if (response != NULL && response_length > 0) { 00064 //DBG("BLELocation:processLocationUpdateResponse: data(%d): %s\r\n",response_length,response); 00065 for(int i=0;i<response_length;++i) { 00066 if (response[i] == ':') { 00067 response[i] = ' '; 00068 present = true; 00069 } 00070 } 00071 00072 // fill with defaults if not presented with new ones... 00073 if (!present) { 00074 sprintf(response,"%s %s %s %s",this->m_latitude,this->m_longitude,this->m_msl_altitude_m,this->m_speed); 00075 } 00076 00077 // parse 00078 sscanf((char *)response,"%f%f%f%f",&latitude,&longitude,&altitude,&speed); 00079 this->setDefault(latitude,longitude,altitude,speed); 00080 00081 // check for disabled location 00082 if (latitude < 1.0 && longitude < 1.0 && speed < 0.0 && altitude < 0) { 00083 DBG("BLELocation: Location reporting is DISABLED in UART_PROXY...\r\n"); 00084 ::Connector::Location::initBuffers(); 00085 } 00086 else if (!present) { 00087 // using cached values 00088 DBG("BLELocation (CACHED): latitude=%s longtiude=%s altitude=%s speed=%s\r\n", 00089 this->m_latitude,this->m_longitude,this->m_msl_altitude_m,this->m_speed); 00090 } 00091 else { 00092 // latest values 00093 DBG("BLELocation (CURRENT): latitude=%s longtiude=%s altitude=%s speed=%s\r\n", 00094 this->m_latitude,this->m_longitude,this->m_msl_altitude_m,this->m_speed); 00095 } 00096 } 00097 else { 00098 // no reponse 00099 DBG("BLELocation: EMPTY response received! Assuming DISABLED.\r\n"); 00100 ::Connector::Location::initBuffers(); 00101 } 00102 } 00103 00104 // update our location 00105 void BLELocation::updateLocation() { 00106 // dispatch an update request 00107 this->dispatchUpdateRequest(); 00108 }
Generated on Sun Jul 17 2022 05:11:06 by
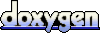