fork of ReportDB customized and renamed as a trivial support personnel database
Fork of ReportDB by
SupportPersonnelDB.cpp
00001 /* Copyright C2014 ARM, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files the "Software", to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "SupportPersonnelDB.h" 00020 00021 // min function 00022 extern "C" int min(int val1,int val2) { 00023 if (val1 < val2) return val1; 00024 return val2; 00025 } 00026 00027 // constructor 00028 SupportPersonnelDB::SupportPersonnelDB() { 00029 this->initDB(); 00030 } 00031 00032 // destructor 00033 SupportPersonnelDB::~SupportPersonnelDB() { 00034 } 00035 00036 // lookup and return a support person 00037 SupportPersonEntry *SupportPersonnelDB::lookup(int rfid) { 00038 SupportPersonEntry *entry = NULL; 00039 bool found = false; 00040 00041 // linear search through the list until we end or find something... 00042 for(int i=0;i<DB_MAX_NUM_SUPPORT_PERSONS &&!found;++i) { 00043 if (this->m_db[i].rfid == rfid) { 00044 found = true; 00045 entry = &(this->m_db[i]); 00046 } 00047 } 00048 00049 return entry; 00050 } 00051 00052 // lookup a support person and return the name 00053 char *SupportPersonnelDB::lookupName(int rfid) { 00054 SupportPersonEntry *entry = this->lookup(rfid); 00055 if (entry != NULL) return entry->name; 00056 return NULL; 00057 } 00058 00059 // lookup a support person and return the description 00060 char *SupportPersonnelDB::lookupDescription(int rfid) { 00061 SupportPersonEntry *entry = this->lookup(rfid); 00062 if (entry != NULL) return entry->description; 00063 return NULL; 00064 } 00065 00066 // lookup a support person and return the current status 00067 char *SupportPersonnelDB::lookupStatus(int rfid) { 00068 SupportPersonEntry *entry = this->lookup(rfid); 00069 if (entry != NULL) return entry->status; 00070 return NULL; 00071 } 00072 00073 // lookup a support person and return the current location(latitude) 00074 char *SupportPersonnelDB::lookupLocationLatitude(int rfid) { 00075 SupportPersonEntry *entry = this->lookup(rfid); 00076 if (entry != NULL) return entry->latitude; 00077 return NULL; 00078 } 00079 00080 // lookup a support person and return the current location(longitude) 00081 char *SupportPersonnelDB::lookupLocationLongitude(int rfid) { 00082 SupportPersonEntry *entry = this->lookup(rfid); 00083 if (entry != NULL) return entry->longitude; 00084 return NULL; 00085 } 00086 00087 // initialize the simple support personnel DB 00088 void SupportPersonnelDB::initDB() { 00089 // First Support Person 00090 memset((void *)&(this->m_db[FIRST_SUPPORT_PERSON]),0,sizeof(SupportPersonEntry)); 00091 this->m_db[FIRST_SUPPORT_PERSON].rfid = FIRST_SUPPORT_PERSON_RFID; 00092 strncpy(this->m_db[FIRST_SUPPORT_PERSON].name,FIRST_SUPPORT_PERSON_NAME,min(strlen(FIRST_SUPPORT_PERSON_NAME),DB_MAX_NAME_LENGTH)); 00093 strncpy(this->m_db[FIRST_SUPPORT_PERSON].description,FIRST_SUPPORT_PERSON_DESCRIPTION,min(strlen(FIRST_SUPPORT_PERSON_DESCRIPTION),DB_MAX_DESCRIPTION_LENGTH)); 00094 strncpy(this->m_db[FIRST_SUPPORT_PERSON].status,FIRST_SUPPORT_PERSON_STATUS,min(strlen(FIRST_SUPPORT_PERSON_STATUS),DB_MAX_STATUS_LENGTH)); 00095 strncpy(this->m_db[FIRST_SUPPORT_PERSON].latitude,FIRST_SUPPORT_PERSON_LATITUDE,min(strlen(FIRST_SUPPORT_PERSON_LATITUDE),DB_MAX_LATLONG_LENGTH)); 00096 strncpy(this->m_db[FIRST_SUPPORT_PERSON].longitude,FIRST_SUPPORT_PERSON_LONGITUDE,min(strlen(FIRST_SUPPORT_PERSON_LONGITUDE),DB_MAX_LATLONG_LENGTH)); 00097 00098 // Second Support Person 00099 memset((void *)&(this->m_db[SECOND_SUPPORT_PERSON]),0,sizeof(SupportPersonEntry)); 00100 this->m_db[SECOND_SUPPORT_PERSON].rfid = SECOND_SUPPORT_PERSON_RFID; 00101 strncpy(this->m_db[SECOND_SUPPORT_PERSON].name,SECOND_SUPPORT_PERSON_NAME,min(strlen(SECOND_SUPPORT_PERSON_NAME),DB_MAX_NAME_LENGTH)); 00102 strncpy(this->m_db[SECOND_SUPPORT_PERSON].description,SECOND_SUPPORT_PERSON_DESCRIPTION,min(strlen(SECOND_SUPPORT_PERSON_DESCRIPTION),DB_MAX_DESCRIPTION_LENGTH)); 00103 strncpy(this->m_db[SECOND_SUPPORT_PERSON].status,SECOND_SUPPORT_PERSON_STATUS,min(strlen(SECOND_SUPPORT_PERSON_STATUS),DB_MAX_STATUS_LENGTH)); 00104 strncpy(this->m_db[SECOND_SUPPORT_PERSON].latitude,SECOND_SUPPORT_PERSON_LATITUDE,min(strlen(SECOND_SUPPORT_PERSON_LATITUDE),DB_MAX_LATLONG_LENGTH)); 00105 strncpy(this->m_db[SECOND_SUPPORT_PERSON].longitude,SECOND_SUPPORT_PERSON_LONGITUDE,min(strlen(SECOND_SUPPORT_PERSON_LONGITUDE),DB_MAX_LATLONG_LENGTH)); 00106 00107 // Third Support Person 00108 memset((void *)&(this->m_db[THIRD_SUPPORT_PERSON]),0,sizeof(SupportPersonEntry)); 00109 this->m_db[THIRD_SUPPORT_PERSON].rfid = THIRD_SUPPORT_PERSON_RFID; 00110 strncpy(this->m_db[THIRD_SUPPORT_PERSON].name,THIRD_SUPPORT_PERSON_NAME,min(strlen(THIRD_SUPPORT_PERSON_NAME),DB_MAX_NAME_LENGTH)); 00111 strncpy(this->m_db[THIRD_SUPPORT_PERSON].description,THIRD_SUPPORT_PERSON_DESCRIPTION,min(strlen(THIRD_SUPPORT_PERSON_DESCRIPTION),DB_MAX_DESCRIPTION_LENGTH)); 00112 strncpy(this->m_db[THIRD_SUPPORT_PERSON].status,THIRD_SUPPORT_PERSON_STATUS,min(strlen(THIRD_SUPPORT_PERSON_STATUS),DB_MAX_STATUS_LENGTH)); 00113 strncpy(this->m_db[THIRD_SUPPORT_PERSON].latitude,THIRD_SUPPORT_PERSON_LATITUDE,min(strlen(THIRD_SUPPORT_PERSON_LATITUDE),DB_MAX_LATLONG_LENGTH)); 00114 strncpy(this->m_db[THIRD_SUPPORT_PERSON].longitude,THIRD_SUPPORT_PERSON_LONGITUDE,min(strlen(THIRD_SUPPORT_PERSON_LONGITUDE),DB_MAX_LATLONG_LENGTH)); 00115 }
Generated on Thu Jul 28 2022 02:27:20 by
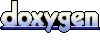