SocketIO is a compatibility library on top of the MBED Websockets API. Currently the broadcast paradigm is supported. There is much more to the SocketIO spec that could be built into this API. Have fun!
Dependents: df-2013-thermostat-handson df-2013-minihack-thermostat-complete df-2013-minihack-thermostat df-2013-thermostat-remotes ... more
SocketIO Class Reference
SocketIO client Class. More...
#include <SocketIO.h>
Public Member Functions | |
SocketIO (char *url) | |
Constructor. | |
SocketIO (char *url, int version) | |
Constructor. | |
bool | connect () |
Connect to the SocketIO url. | |
int | emit (char *name, char *args) |
Emit (Broadcast) a socket.io message to the SocketIO server. | |
bool | read (char *message) |
Read a SocketIO message. | |
bool | is_connected () |
To see if there is a SocketIO connection active. | |
bool | close () |
Close the SocketIO connection. |
Detailed Description
SocketIO client Class.
Example (ethernet network):
#include "mbed.h" #include "WiflyInterface.h" #include "SocketIO.h" // our wifi interface WiflyInterface wifly(p9, p10, p30, p29, "mysid", "mypw", WPA); int main() { wifly.init(); //Use DHCP wifly.connect(); printf("IP Address is %s\n\r", wifly.getIPAddress()); SocketIO socketio("myapp.herokuapp.com"); socketio.connect(); char recv[256]; while (1) { int res = socketio.emit("mesage_name","[\"SocketIO Hello World!\"]"); if (socketio.read(recv)) { printf("rcv: %s\r\n", recv); } wait(0.1); } }
Definition at line 79 of file SocketIO.h.
Constructor & Destructor Documentation
SocketIO | ( | char * | url ) |
Constructor.
- Parameters:
-
url The SocketIO url in the form "www.example.com:[port]" (by default: port = 80) - i.e. just the endpoint name
Definition at line 4 of file SocketIO.cpp.
SocketIO | ( | char * | url, |
int | version | ||
) |
Constructor.
- Parameters:
-
url The SocketIO url in the form "www.example.com:[port]" (by default: port = 80) - i.e. just the endpoint name version The SocketIO version for the session URL (by default version = 1)
Definition at line 10 of file SocketIO.cpp.
Member Function Documentation
bool close | ( | ) |
Close the SocketIO connection.
- Returns:
- true if the connection has been closed, false otherwise
Definition at line 42 of file SocketIO.cpp.
bool connect | ( | ) |
Connect to the SocketIO url.
- Returns:
- true if the connection is established, false otherwise
Definition at line 16 of file SocketIO.cpp.
int emit | ( | char * | name, |
char * | args | ||
) |
Emit (Broadcast) a socket.io message to the SocketIO server.
Socket.IO message Format (JSON): { "name": <name>, "args":<args> } name: the "name" of the socket.io message args: the argument(s) (must always be a JSON array) of the message. Example: "[ \"foo", {"bar": "none"}]"
- Parameters:
-
name "name" of the socket.io message to broadcast args argument string to be sent ( must be in a JSON array format. Example: "[ \"foo", {"bar": "none"}]" )
- Returns:
- the number of bytes sent
Definition at line 64 of file SocketIO.cpp.
bool is_connected | ( | ) |
To see if there is a SocketIO connection active.
- Returns:
- true if there is a connection active
Definition at line 98 of file SocketIO.cpp.
bool read | ( | char * | message ) |
Read a SocketIO message.
- Parameters:
-
message pointer to the string to be read (null if drop frame)
- Returns:
- true if a SocketIO frame has been read
Definition at line 84 of file SocketIO.cpp.
Generated on Tue Jul 12 2022 17:26:41 by
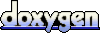