
a
BezierCurve< T > Class Template Reference
It implements the functionality of a Bezier curve. More...
#include <beziercurve.hpp>
Public Member Functions | |
BezierCurve () | |
Construct a new Bezier Curve< T>:: Bezier Curve object. | |
BezierCurve (std::complex< T > points[BEZIER_ORDER+1]) | |
Construct a new Bezier Curve< T>:: Bezier Curve object. | |
BezierCurve (std::complex< T > a, std::complex< T > b, std::complex< T > c, std::complex< T > d) | |
Construct a new Bezier Curve< T>:: Bezier Curve object. | |
virtual | ~BezierCurve () |
Destroy the Bezier Curve< T>:: Bezier Curve object. | |
void | setBezierCurve (std::complex< T > a, std::complex< T > b, std::complex< T > c, std::complex< T > d) |
Set the points for creating a new Bezier curve. | |
std::complex< T > | getValue (float input_value) |
Get the point on the bezier curve. | |
std::complex< T > | get_FO_DerivateValue (float input_value) |
Get the value of the first order derivative of Bezier curve. | |
std::complex< T > | get_SO_DerivateValue (float input_value) |
Get the value of the second order derivative of Bezier Curve. | |
math::PolynomialFunction < std::complex< T > , BEZIER_ORDER > | getBezierCurve () |
Get the polynomial function, which respresents the Bezier curve. | |
math::PolynomialFunction < std::complex< T > , BEZIER_ORDER-1 > | getFODerivate () |
Get resulted polynomial function of the first order derivative. | |
math::PolynomialFunction < std::complex< T > , BEZIER_ORDER-2 > | getSODerivate () |
Get resulted polynomial function of the second order derivative. |
Detailed Description
template<class T>
class math::BezierCurve< T >
It implements the functionality of a Bezier curve.
The points on the curve are represented by complex numbers and the input value of the curve have to belong to the interval [0,1].
- Template Parameters:
-
T The type of the Bezier curve.
Definition at line 29 of file beziercurve.hpp.
Constructor & Destructor Documentation
BezierCurve | ( | ) |
Construct a new Bezier Curve< T>:: Bezier Curve object.
Definition at line 9 of file beziercurve.inl.
BezierCurve | ( | std::complex< T > | points[BEZIER_ORDER+1] ) |
Construct a new Bezier Curve< T>:: Bezier Curve object.
- Parameters:
-
points Array of points
Definition at line 38 of file beziercurve.inl.
BezierCurve | ( | std::complex< T > | a, |
std::complex< T > | b, | ||
std::complex< T > | c, | ||
std::complex< T > | d | ||
) |
Construct a new Bezier Curve< T>:: Bezier Curve object.
- Parameters:
-
a point A b point B c point C d point D
Definition at line 21 of file beziercurve.inl.
~BezierCurve | ( | ) | [virtual] |
Destroy the Bezier Curve< T>:: Bezier Curve object.
Definition at line 53 of file beziercurve.inl.
Member Function Documentation
std::complex< T > get_FO_DerivateValue | ( | float | input_value ) |
Get the value of the first order derivative of Bezier curve.
- Parameters:
-
input_value The input value [0,1]
- Returns:
- The point as a complex number.
Definition at line 100 of file beziercurve.inl.
std::complex< T > get_SO_DerivateValue | ( | float | input_value ) |
Get the value of the second order derivative of Bezier Curve.
- Parameters:
-
input_value The input value of the function have to belong to interval [0,1]
- Returns:
- The resulted value as a complex number.
Definition at line 112 of file beziercurve.inl.
math::PolynomialFunction< std::complex< T >, BEZIER_ORDER > getBezierCurve | ( | ) |
Get the polynomial function, which respresents the Bezier curve.
Definition at line 123 of file beziercurve.inl.
math::PolynomialFunction< std::complex< T >, BEZIER_ORDER-1 > getFODerivate | ( | ) |
Get resulted polynomial function of the first order derivative.
- Returns:
- math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-1>
Definition at line 133 of file beziercurve.inl.
math::PolynomialFunction< std::complex< T >, BEZIER_ORDER-2 > getSODerivate | ( | ) |
Get resulted polynomial function of the second order derivative.
- Returns:
- math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-2>
Definition at line 143 of file beziercurve.inl.
std::complex< T > getValue | ( | float | input_value ) |
Get the point on the bezier curve.
- Parameters:
-
input_value The input value, it must belong to interval [0,1].
- Returns:
- The point as complex number
Definition at line 87 of file beziercurve.inl.
void setBezierCurve | ( | std::complex< T > | a, |
std::complex< T > | b, | ||
std::complex< T > | c, | ||
std::complex< T > | d | ||
) |
Set the points for creating a new Bezier curve.
- Parameters:
-
a point A b point B c point C d point D
Definition at line 156 of file beziercurve.inl.
Generated on Tue Jul 12 2022 22:40:51 by
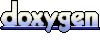