
a
Embed:
(wiki syntax)
Show/hide line numbers
beziercurve.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file BezierCurve.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class declaration for the Bezier Curve 00008 * methods. 00009 ****************************************************************************** 00010 */ 00011 00012 /* include guard */ 00013 #ifndef BEZIERCURVE_HPP 00014 #define BEZIERCURVE_HPP 00015 00016 /* complex numbers in cartesian form and several functions and overloads to operate with them */ 00017 #include <complex> 00018 #include <BezierCurve/PolynomialFunction.hpp> 00019 00020 #define BEZIER_ORDER 3 00021 00022 namespace math{ 00023 /** 00024 * @brief It implements the functionality of a Bezier curve. The points on the curve are represented by complex numbers and the input value of the curve have to belong to the interval [0,1]. 00025 * 00026 * @tparam T The type of the Bezier curve. 00027 */ 00028 template<class T> 00029 class BezierCurve 00030 { 00031 public: 00032 /* Constructors */ 00033 BezierCurve(); 00034 BezierCurve(std::complex<T> points[BEZIER_ORDER+1]); 00035 BezierCurve(std::complex<T> a,std::complex<T> b,std::complex<T> c,std::complex<T> d); 00036 /* Destructor */ 00037 virtual ~BezierCurve(); 00038 /* Set curve */ 00039 void setBezierCurve(std::complex<T> a,std::complex<T> b,std::complex<T> c,std::complex<T> d); 00040 /* Get value */ 00041 std::complex<T> getValue(float input_value); 00042 /* First order derivate value */ 00043 std::complex<T> get_FO_DerivateValue(float input_value); 00044 /* Second order derivate value */ 00045 std::complex<T> get_SO_DerivateValue(float input_value); 00046 00047 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER > getBezierCurve(); 00048 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-1> getFODerivate(); 00049 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-2> getSODerivate(); 00050 00051 protected: 00052 00053 private: 00054 // PRIVATE FUNCTIONS 00055 // Convert the complex points to polynomial function 00056 math::PolynomialFunction<std::complex<float>,BEZIER_ORDER> CP2PF(); 00057 00058 // PRIVATE PARAMETERS 00059 std::complex<T> points[BEZIER_ORDER+1]; 00060 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER> bezierCurve; 00061 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-1> FOder_bezierCurve; 00062 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-2> SOder_bezierCurve; 00063 }; 00064 };//namespace math 00065 #include "beziercurve.inl" 00066 00067 #endif // BEZIERCURVE_HPP 00068
Generated on Tue Jul 12 2022 22:40:50 by
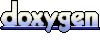