
a
Embed:
(wiki syntax)
Show/hide line numbers
beziercurve.inl
00001 00002 namespace math{ 00003 00004 /** 00005 * @brief Construct a new Bezier Curve< T>:: Bezier Curve object 00006 * 00007 */ 00008 template<class T> 00009 BezierCurve<T>::BezierCurve(){ 00010 } 00011 00012 /** 00013 * @brief Construct a new Bezier Curve< T>:: Bezier Curve object 00014 * 00015 * @param a point A 00016 * @param b point B 00017 * @param c point C 00018 * @param d point D 00019 */ 00020 template<class T> 00021 BezierCurve<T>::BezierCurve(std::complex<T> a,std::complex<T> b,std::complex<T> c,std::complex<T> d){ 00022 this->points[0]=a; 00023 this->points[1]=b; 00024 this->points[2]=c; 00025 this->points[3]=d; 00026 00027 this->bezierCurve=this->CP2PF(); 00028 this->FOder_bezierCurve=this->bezierCurve.derivateFO(); 00029 this->SOder_bezierCurve=this->FOder_bezierCurve.derivateFO(); 00030 } 00031 00032 /** 00033 * @brief Construct a new Bezier Curve< T>:: Bezier Curve object 00034 * 00035 * @param points Array of points 00036 */ 00037 template<class T> 00038 BezierCurve<T>::BezierCurve(std::complex<T> points[BEZIER_ORDER+1]) 00039 { 00040 for(int32_t i=0;i<=BEZIER_ORDER;++i){ 00041 this->points[i]=points[i]; 00042 } 00043 this->bezierCurve=this->CP2PF(); 00044 this->FOder_bezierCurve=this->bezierCurve.derivateFO(); 00045 this->SOder_bezierCurve=this->FOder_bezierCurve.derivateFO(); 00046 } 00047 00048 /** 00049 * @brief Destroy the Bezier Curve< T>:: Bezier Curve object 00050 * 00051 */ 00052 template<class T> 00053 BezierCurve<T>::~BezierCurve() 00054 { 00055 //dtor 00056 } 00057 00058 /** 00059 * @brief This metohd create a new polynomial function based on the complex points. 00060 * 00061 * @return The result polynomial function represent the Bezier curve. 00062 */ 00063 template<class T> 00064 math::PolynomialFunction<std::complex<float>,BEZIER_ORDER> BezierCurve<T>::CP2PF(){ 00065 math::PolynomialFunction<std::complex<float>,BEZIER_ORDER> pf; 00066 00067 const std::complex<T> temp_cst_3(3,0); 00068 const std::complex<T> temp_cst_2(2,0); 00069 00070 std::complex<T> coef1=temp_cst_3*(this->points[1]-this->points[0]); 00071 std::complex<T> coef2=temp_cst_3*(this->points[0]-temp_cst_2*this->points[1]+this->points[2]); 00072 std::complex<T> coef3=temp_cst_3*(this->points[1]-this->points[2])+this->points[3]-this->points[0]; 00073 pf.setCoefficientValue(0,this->points[0]); 00074 pf.setCoefficientValue(1,coef1); 00075 pf.setCoefficientValue(2,coef2); 00076 pf.setCoefficientValue(3,coef3); 00077 return pf; 00078 } 00079 00080 /** 00081 * @brief Get the point on the bezier curve. 00082 * 00083 * @param input_value The input value, it must belong to interval [0,1]. 00084 * @return The point as complex number 00085 */ 00086 template<class T> 00087 std::complex<T> BezierCurve<T>::getValue(float input_value){ 00088 T input_value_T=static_cast<T>(input_value); 00089 return this->bezierCurve.calculateValue(input_value); 00090 } 00091 00092 template<class T> 00093 /** 00094 * @brief Get the value of the first order derivative of Bezier curve 00095 * 00096 * @param input_value The input value [0,1] 00097 * @return The point as a complex number. 00098 * 00099 */ 00100 std::complex<T> BezierCurve<T>::get_FO_DerivateValue(float input_value){ 00101 T input_value_T=static_cast<T>(input_value); 00102 return this->FOder_bezierCurve.calculateValue(input_value_T); 00103 } 00104 00105 /** 00106 * @brief Get the value of the second order derivative of Bezier Curve. 00107 * 00108 * @param input_value The input value of the function have to belong to interval [0,1] 00109 * @return The resulted value as a complex number. 00110 */ 00111 template<class T> 00112 std::complex<T> BezierCurve<T>::get_SO_DerivateValue(float input_value){ 00113 T input_value_T=static_cast<T>(input_value); 00114 00115 return this->SOder_bezierCurve.calculateValue(input_value_T); 00116 } 00117 00118 /** 00119 * @brief Get the polynomial function, which respresents the Bezier curve. 00120 * 00121 */ 00122 template<class T> 00123 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER> BezierCurve<T>::getBezierCurve(){ 00124 return this->bezierCurve; 00125 } 00126 00127 /** 00128 * @brief Get resulted polynomial function of the first order derivative 00129 * 00130 * @return math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-1> 00131 */ 00132 template<class T> 00133 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-1> BezierCurve<T>::getFODerivate(){ 00134 return this->FOder_bezierCurve; 00135 } 00136 00137 template<class T> 00138 /** 00139 * @brief Get resulted polynomial function of the second order derivative 00140 * 00141 * @return math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-2> 00142 */ 00143 math::PolynomialFunction<std::complex<T>,BEZIER_ORDER-2> BezierCurve<T>::getSODerivate(){ 00144 return this->SOder_bezierCurve; 00145 } 00146 00147 template<class T> 00148 /** 00149 * @brief Set the points for creating a new Bezier curve 00150 * 00151 * @param a point A 00152 * @param b point B 00153 * @param c point C 00154 * @param d point D 00155 */ 00156 void BezierCurve<T>::setBezierCurve(std::complex<T> a,std::complex<T> b,std::complex<T> c,std::complex<T> d){ 00157 this->points[0]=a; 00158 this->points[1]=b; 00159 this->points[2]=c; 00160 this->points[3]=d; 00161 00162 this->bezierCurve=this->CP2PF(); 00163 this->FOder_bezierCurve=this->bezierCurve.derivateFO(); 00164 this->SOder_bezierCurve=this->FOder_bezierCurve.derivateFO(); 00165 } 00166 00167 }; // namespace math
Generated on Tue Jul 12 2022 22:40:50 by
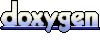