ros_serial for mbed updated to work with ROS Hydro. Library still needs to be debugged
Embed:
(wiki syntax)
Show/hide line numbers
Logging.cpp
00001 //#define COMPILE_LOGGING_CODE_ROSSERIAL 00002 #ifdef COMPILE_LOGGING_CODE_ROSSERIAL 00003 00004 /* 00005 * rosserial PubSub Example 00006 * Prints "hello world!" and toggles led 00007 */ 00008 00009 #include <ros.h> 00010 #include <std_msgs/String.h> 00011 #include <std_msgs/Empty.h> 00012 00013 ros::NodeHandle nh; 00014 00015 std_msgs::String str_msg; 00016 ros::Publisher chatter("chatter", &str_msg); 00017 00018 char hello[13] = "hello world!"; 00019 00020 char debug[]= "debug statements"; 00021 char info[] = "infos"; 00022 char warn[] = "warnings"; 00023 char error[] = "errors"; 00024 char fatal[] = "fatalities"; 00025 00026 int main() { 00027 nh.initNode(); 00028 nh.advertise(chatter); 00029 00030 while (1) { 00031 str_msg.data = hello; 00032 chatter.publish( &str_msg ); 00033 00034 nh.logdebug(debug); 00035 nh.loginfo(info); 00036 nh.logwarn(warn); 00037 nh.logerror(error); 00038 nh.logfatal(fatal); 00039 00040 nh.spinOnce(); 00041 wait_ms(500); 00042 } 00043 } 00044 00045 #endif
Generated on Sun Jul 17 2022 00:31:54 by
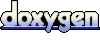