Library for using the AMS TSL45315 Ambient Light Sensor
TSL45315 Class Reference
Class: TSL45315 Digital Ambient Light Sensor (ALS) - TSL45315 Illumination values are directly presented in lux without calculation. More...
#include <tsl45315.hpp>
Public Member Functions | |
TSL45315 (PinName sda, PinName scl, int i2c_freq, float t) | |
Create a TSL45315 instance without variable integration time ( set to M1) with integrated ticker to repeatedly update the illumination value. | |
TSL45315 (PinName sda, PinName scl, int i2c_freq, float t, uint8_t mult) | |
Create a TSL45315 instance with variable integration time (M1,M2,M4) and with integrated ticker to repeatedly update the illumination value. | |
TSL45315 (PinName sda, PinName scl, uint8_t mult) | |
Create a TSL45315 instance with variable integration time (M1,M2,M4) without integrated ticker to repeatedly update the illumination value. | |
TSL45315 (PinName sda, PinName scl) | |
Create a TSL45315 instance without variable integration time ( set to M1) and without integrated ticker to repeatedly update the illumination value. | |
void | setReg (int reg, int arg) |
sets the address in the command register of the target register for future write operations | |
void | getLuxData () |
reads out datalow and datahigh registers and calculates the resulting illumination value in lux which is saved in the variable lux | |
uint32_t | getLux () |
void | getIDdata () |
reads out the ID register and saves the id in the variable devID | |
uint8_t | getID () |
void | setMultiplier (uint8_t mult) |
reads out datalow and datahigh registers and calculates the resulting illumination value in lux which is saved in the variable lux | |
int | getMultiplier () |
Detailed Description
Class: TSL45315 Digital Ambient Light Sensor (ALS) - TSL45315 Illumination values are directly presented in lux without calculation.
Example:
#include "mbed.h" #include "tsl45315.hpp" Serial pc(USBTX, USBRX); //TSL45x::TSL45315 sensor(p9, p10); //TSL45x::TSL45315 sensor(p9, p10, M1); TSL45x::TSL45315 sensor(p9, p10, I2C_FREQ, 0.5); //TSL45x::TSL45315 sensor(p9, p10, I2C_FREQ, 0.5, M1); int main( void ) { pc.printf("TSL45315 Illumination Sensor\n"); pc.printf("ID:\t%x\n", sensor.getID()); pc.printf("Multiplier:\t%x\n", sensor.getMultiplier()); while(1) { //sensor.getLuxData(); pc.printf("Illumination: %u lux\n",sensor.getLux()); wait(1); } }
Definition at line 60 of file tsl45315.hpp.
Constructor & Destructor Documentation
TSL45315 | ( | PinName | sda, |
PinName | scl, | ||
int | i2c_freq, | ||
float | t | ||
) |
Create a TSL45315 instance without variable integration time ( set to M1) with integrated ticker to repeatedly update the illumination value.
- Parameters:
-
sda - I2C Dataline pin scl - I2C Clockline pin i2c_freq - choose the i2c frequency t - ticker interval in seconds
Definition at line 7 of file tsl45315.cpp.
TSL45315 | ( | PinName | sda, |
PinName | scl, | ||
int | i2c_freq, | ||
float | t, | ||
uint8_t | mult | ||
) |
Create a TSL45315 instance with variable integration time (M1,M2,M4) and with integrated ticker to repeatedly update the illumination value.
- Parameters:
-
sda - I2C Dataline pin scl - I2C Clockline pin i2c_freq - choose the i2c frequency mult - Multiplier with corresponding integration time (M1 = 400ms, M2 = 200ms, M4 = 100ms) t - ticker interval in seconds
Definition at line 17 of file tsl45315.cpp.
TSL45315 | ( | PinName | sda, |
PinName | scl, | ||
uint8_t | mult | ||
) |
Create a TSL45315 instance with variable integration time (M1,M2,M4) without integrated ticker to repeatedly update the illumination value.
For use with seperate ticker or RtosTimer.
- Parameters:
-
sda - I2C Dataline pin scl - I2C Clockline pin mult - Multiplier with corresponding integration time (M1 = 400ms, M2 = 200ms, M4 = 100ms)
Definition at line 27 of file tsl45315.cpp.
TSL45315 | ( | PinName | sda, |
PinName | scl | ||
) |
Create a TSL45315 instance without variable integration time ( set to M1) and without integrated ticker to repeatedly update the illumination value.
For use with seperate ticker or RtosTimer.
- Parameters:
-
sda - I2C Dataline pin scl - I2C Clockline pin
Definition at line 36 of file tsl45315.cpp.
Member Function Documentation
uint8_t getID | ( | ) |
- Returns:
- the ID of the device
Definition at line 138 of file tsl45315.hpp.
void getIDdata | ( | ) |
reads out the ID register and saves the id in the variable devID
Definition at line 72 of file tsl45315.cpp.
uint32_t getLux | ( | ) |
- Returns:
- returns the actual illumination value in lux which was previously calculated in the getLuxData routine
Definition at line 128 of file tsl45315.hpp.
void getLuxData | ( | ) |
reads out datalow and datahigh registers and calculates the resulting illumination value in lux which is saved in the variable lux
- Parameters:
-
reg - specific register to write to arg - argument of function in the control or config register
Definition at line 85 of file tsl45315.cpp.
int getMultiplier | ( | ) |
- Returns:
- the multiplier according to the choosen integration time
Definition at line 151 of file tsl45315.hpp.
void setMultiplier | ( | uint8_t | mult ) |
reads out datalow and datahigh registers and calculates the resulting illumination value in lux which is saved in the variable lux
- Parameters:
-
mult - Multiplier (1,2,4) defined by M1, M2, M4
Definition at line 45 of file tsl45315.cpp.
void setReg | ( | int | reg, |
int | arg | ||
) |
sets the address in the command register of the target register for future write operations
- Parameters:
-
reg - specific register to write to (control or config) arg - argument of function in the control or config register
Definition at line 63 of file tsl45315.cpp.
Generated on Wed Jul 13 2022 02:27:10 by
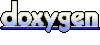