Library for using the AMS TSL45315 Ambient Light Sensor
Embed:
(wiki syntax)
Show/hide line numbers
tsl45315.hpp
00001 00002 00003 #ifndef _TSL45315_HPP_ 00004 #define _TSL45315_HPP_ 00005 00006 #include "mbed.h" 00007 00008 #define I2C_ADDR (0x52) 00009 // Register definition 00010 #define CONTROL_REG 0x00 00011 #define CONFIG_REG 0x01 00012 #define DATALOW_REG 0x04 00013 #define DATAHIGH_REG 0x05 00014 #define ID_REG 0x0A 00015 //Control Register 00016 #define POWER_DOWN 0X00 00017 #define SINGLE_ADC 0x02 00018 #define NORMAL_OP 0x03 00019 //Config Register 00020 #define M1 0x00 00021 #define M2 0x01 00022 #define M4 0x02 00023 00024 #define I2C_FREQ 400000 00025 00026 namespace TSL45x 00027 { 00028 /** Class: TSL45315 00029 * Digital Ambient Light Sensor (ALS) - TSL45315 00030 * Illumination values are directly presented in lux 00031 * without calculation. 00032 * 00033 * Example: 00034 * @code 00035 * #include "mbed.h" 00036 * #include "tsl45315.hpp" 00037 00038 * Serial pc(USBTX, USBRX); 00039 * //TSL45x::TSL45315 sensor(p9, p10); 00040 * //TSL45x::TSL45315 sensor(p9, p10, M1); 00041 * TSL45x::TSL45315 sensor(p9, p10, I2C_FREQ, 0.5); 00042 * //TSL45x::TSL45315 sensor(p9, p10, I2C_FREQ, 0.5, M1); 00043 * 00044 * int main( void ) 00045 * { 00046 * pc.printf("TSL45315 Illumination Sensor\n"); 00047 * 00048 * pc.printf("ID:\t%x\n", sensor.getID()); 00049 * pc.printf("Multiplier:\t%x\n", sensor.getMultiplier()); 00050 * 00051 * while(1) { 00052 * //sensor.getLuxData(); 00053 * pc.printf("Illumination: %u lux\n",sensor.getLux()); 00054 * 00055 * wait(1); 00056 * } 00057 * } 00058 * @endcode 00059 */ 00060 class TSL45315 00061 { 00062 00063 private: 00064 00065 I2C _i2c; 00066 Ticker _luxTicker; 00067 00068 uint32_t lux; 00069 uint8_t devID; 00070 uint8_t multiplier; 00071 00072 public: 00073 /** Create a TSL45315 instance without variable integration time ( set to M1) 00074 * with integrated ticker to repeatedly update the illumination value. 00075 * @param sda - I2C Dataline pin 00076 * @param scl - I2C Clockline pin 00077 * @param i2c_freq - choose the i2c frequency 00078 * @param t - ticker interval in seconds 00079 */ 00080 TSL45315(PinName sda, PinName scl, int i2c_freq, float t); 00081 00082 /** Create a TSL45315 instance with variable integration time (M1,M2,M4) 00083 * and with integrated ticker to repeatedly update the illumination value. 00084 * @param sda - I2C Dataline pin 00085 * @param scl - I2C Clockline pin 00086 * @param i2c_freq - choose the i2c frequency 00087 * @param mult - Multiplier with corresponding integration time (M1 = 400ms, M2 = 200ms, M4 = 100ms) 00088 * @param t - ticker interval in seconds 00089 */ 00090 TSL45315(PinName sda, PinName scl, int i2c_freq, float t, uint8_t mult); 00091 00092 /** Create a TSL45315 instance with variable integration time (M1,M2,M4) 00093 * without integrated ticker to repeatedly update the illumination value. 00094 * For use with seperate ticker or RtosTimer. 00095 * @param sda - I2C Dataline pin 00096 * @param scl - I2C Clockline pin 00097 * @param mult - Multiplier with corresponding integration time (M1 = 400ms, M2 = 200ms, M4 = 100ms) 00098 */ 00099 TSL45315(PinName sda, PinName scl, uint8_t mult); 00100 00101 /** Create a TSL45315 instance without variable integration time ( set to M1) 00102 * and without integrated ticker to repeatedly update the illumination value. 00103 * For use with seperate ticker or RtosTimer. 00104 * @param sda - I2C Dataline pin 00105 * @param scl - I2C Clockline pin 00106 */ 00107 TSL45315(PinName sda, PinName scl); 00108 00109 /** sets the address in the command register of the 00110 * target register for future write operations 00111 * @param reg - specific register to write to (control or config) 00112 * @param arg - argument of function in the control or config register 00113 */ 00114 void setReg( int reg, int arg); 00115 00116 00117 00118 /** reads out datalow and datahigh registers and 00119 * calculates the resulting illumination value 00120 * in lux which is saved in the variable lux 00121 * @param reg - specific register to write to 00122 * @param arg - argument of function in the control or config register 00123 */ 00124 void getLuxData( ); 00125 00126 /** @returns returns the actual illumination value in lux which was previously calculated in the getLuxData routine 00127 */ 00128 uint32_t getLux ( ) { 00129 return lux; 00130 }; 00131 00132 /** reads out the ID register and saves the id in the variable devID 00133 */ 00134 void getIDdata( ); 00135 00136 /** @returns the ID of the device 00137 */ 00138 uint8_t getID ( ) { 00139 return devID; 00140 }; 00141 00142 /** reads out datalow and datahigh registers and 00143 * calculates the resulting illumination value 00144 * in lux which is saved in the variable lux 00145 * @param mult - Multiplier (1,2,4) defined by M1, M2, M4 00146 */ 00147 void setMultiplier( uint8_t mult); 00148 00149 /** @returns the multiplier according to the choosen integration time 00150 */ 00151 int getMultiplier ( ) { 00152 return multiplier; 00153 }; 00154 00155 }; 00156 } 00157 00158 00159 /* !_TSL45315_HPP_ */ 00160 #endif
Generated on Wed Jul 13 2022 02:27:10 by
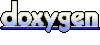