
ECE 4180 Lab 3 Part 1 Extra Credit
Dependencies: HC_SR04_Ultrasonic_Library mbed
Fork of LPC1768_HCSR04_HelloWorld by
main.cpp
00001 //Theremin style demo using HC-SR04 Sonar and a speaker 00002 // moving a hand away/towards sonar changes audio frequency 00003 #include "mbed.h" 00004 #include "ultrasonic.h" 00005 00006 DigitalOut audio(p26); //output to speaker amp or audio jack 00007 DigitalOut led(LED1); 00008 DigitalOut led2(LED2); 00009 00010 Timeout cycle; 00011 00012 volatile int half_cycle_time = 1; 00013 00014 //two calls to this interrupt routine generates a square wave 00015 void toggle_interrupt() 00016 { 00017 if (half_cycle_time>22000) audio=0; //mute if nothing in range 00018 else audio = !audio; //toggle to make half a square wave 00019 led = !led; 00020 cycle.detach(); 00021 //update time for interrupt activation -change frequency of square wave 00022 cycle.attach_us(&toggle_interrupt, half_cycle_time); 00023 } 00024 void newdist(int distance) 00025 { 00026 //update frequency based on new sonar data 00027 led2 = !led2; 00028 half_cycle_time = distance<<3; 00029 } 00030 //HC-SR04 Sonar module 00031 ultrasonic mu(p6, p7, .07, 1, &newdist); 00032 //Set the trigger pin to p6 and the echo pin to p7 00033 //have updates every .07 seconds and a timeout after 1 00034 //second, and call newdist when the distance changes 00035 00036 int main() 00037 { 00038 audio = 0; 00039 led = 0; 00040 cycle.attach(&toggle_interrupt, half_cycle_time); 00041 mu.startUpdates();//start measuring the distance with the sonar 00042 while(1) { 00043 //Do something else here 00044 mu.checkDistance(); 00045 //call checkDistance() as much as possible, as this is where 00046 //the class checks if dist needs to be called. 00047 } 00048 }
Generated on Sat Jul 23 2022 12:07:23 by
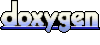