
Program an AVR microcontroller using mbed.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Program an AVR with an mbed. 00003 */ 00004 00005 #include "AVR910.h" 00006 00007 LocalFileSystem local("local"); 00008 Serial pc(USBTX, USBRX); 00009 AVR910 mAVRISP(p5, p6, p7, p8); //mosi, miso, sclk, nreset. 00010 00011 int main() { 00012 00013 int success = -1; 00014 int response = 0; 00015 00016 //Read the vendor code [0x1E == Atmel]. 00017 response = mAVRISP.readVendorCode(); 00018 00019 if (response == ATMEL_VENDOR_CODE) { 00020 pc.printf("Microcontroller is an Atmel [0x%02x]\n", response); 00021 } else if (response == DEVICE_LOCKED) { 00022 pc.printf("Device is locked\n"); 00023 return -1; 00024 } else { 00025 pc.printf("Microcontroller is not an Atmel\n"); 00026 return -1; 00027 } 00028 00029 //Read part family and flash size - see datasheet for code meaning. 00030 response = mAVRISP.readPartFamilyAndFlashSize(); 00031 00032 if (response == 0xFF) { 00033 pc.printf("Device code erased or target missing\n"); 00034 } else if (response == 0x01) { 00035 pc.printf("Device locked\n"); 00036 return -1; 00037 } else { 00038 pc.printf("Part family and flash size code is: 0x%02x\n", response); 00039 } 00040 00041 //Read part number. 00042 response = mAVRISP.readPartNumber(); 00043 00044 if (response == 0xFF) { 00045 pc.printf("Device code erased or target missing\n"); 00046 } else if (response == 0x02) { 00047 pc.printf("Device locked\n"); 00048 return -1; 00049 } else { 00050 pc.printf("Part number code is: 0x%02x\n", response); 00051 } 00052 00053 //Open binary file to write to AVR. 00054 FILE *fp = fopen(PATH_TO_BINARY, "rb"); 00055 00056 if (fp == NULL) { 00057 pc.printf("Failed to open binary. Please check the file path\n"); 00058 return -1; 00059 } else { 00060 //Program it! 00061 pc.printf("Binary file opened successfully\n"); 00062 success = mAVRISP.program(fp, 00063 ATMEGA328P_PAGESIZE, 00064 ATMEGA328P_NUM_PAGES); 00065 fclose(fp); 00066 } 00067 00068 if (success < 0) { 00069 printf("Programming failed.\n"); 00070 } else { 00071 printf("Programming was successful!\n"); 00072 } 00073 00074 }
Generated on Tue Jul 12 2022 14:07:14 by
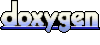