
ガイガーカウンタのカウントアップに合わせて表示内容を更新する仕様にしました
Dependencies: Terminal TextLCD mbed SDFileSystem
geiger.cpp
00001 #include "geiger.h" 00002 #include "Terminal.h" 00003 #include "TextLCD.h" 00004 #include "SDFileSystem.h" 00005 00006 00007 //debug use 00008 //#define DEBUG 00009 00010 //avarage range minutes 00011 #define AVARAGE_MIN 5 00012 00013 //sleeptime after a minute cout 00014 #define SLEEP_SEC 30 00015 00016 //if sdcard not use commentout #define SD_USE 00017 #define SD_USE 00018 00019 #ifdef DEBUG 00020 Terminal output(USBTX, USBRX); // tx, rx 00021 #else 00022 TextLCD output( p24, p26, p27, p28, p29, p30 ); // rs, e, d0-d3 00023 #endif 00024 00025 //Trans contorol pin 00026 #define CTL_TRANS_PIN p21 00027 00028 //Trans params 00029 #define TRANS_PERIOD (0.16/1000.0) 00030 #define TRANS_DUTY (0.83) 00031 00032 //Giger Counter Pin 00033 #define GIGER_PIN p18 00034 00035 #ifdef SD_USE 00036 //SD File system setup 00037 SDFileSystem sd(p5, p6, p7, p8, "sd"); // mosi, miso, sclk, cs 00038 #endif 00039 00040 DigitalOut waiting(LED1); 00041 DigitalOut working(LED2); 00042 00043 PwmOut trans_ctl(CTL_TRANS_PIN); 00044 InterruptIn gigerin(GIGER_PIN); 00045 Ticker show; 00046 //for timedata 00047 char buf[40]; 00048 time_t sec; 00049 00050 //counter 00051 int cnt; 00052 //array first touch index chk 00053 int first_touch_index; 00054 //micro sv value 00055 float usv; 00056 //peak sv value; 00057 float peak_usv; 00058 00059 00060 //for avarage calculate arrays 00061 float cpm_array[AVARAGE_MIN]; 00062 //current index for array 00063 int cpm_index; 00064 00065 00066 ///////////////////////////////// 00067 //trans contorol for 400v make // 00068 ///////////////////////////////// 00069 00070 //make 400v 00071 void transon() 00072 { 00073 //6khz puluse 00074 trans_ctl.period(TRANS_PERIOD); 00075 trans_ctl=TRANS_DUTY; 00076 } 00077 00078 //trans sleep! 00079 void transoff() 00080 { 00081 trans_ctl=0.0; 00082 } 00083 00084 00085 //print lcd for 1sec 00086 void show_result() 00087 { 00088 output.cls(); 00089 output.printf("NOW%fuSv/h",usv); 00090 output.printf("PEK%fuSv/h",peak_usv); 00091 00092 } 00093 //sv value calc 00094 void calculate_sv() 00095 { 00096 float cpm=0; 00097 for(int i=0;i<=first_touch_index;i++) 00098 { 00099 cpm+=cpm_array[i]; 00100 } 00101 cpm/=(first_touch_index+1); 00102 //si-3bg is maybe 200 cps/R/h = 200 cps/ 10000uSv/h = 0.02 cps/uSv/h 00103 usv=cpm/60.0*0.02; 00104 00105 //peak usv set 00106 if(peak_usv<usv) 00107 { 00108 peak_usv=usv; 00109 } 00110 } 00111 00112 00113 void debug_print() 00114 { 00115 00116 //printf("\r\n\r\nresult_str=%s\r\n",result_str); 00117 00118 float cpm=0; 00119 for(int i=0;i<=first_touch_index;i++) 00120 { 00121 cpm+=cpm_array[i]; 00122 } 00123 cpm/=(first_touch_index+1); 00124 printf("%fuSv/h\r\n",cpm/20.0); 00125 for(int i=0;i<AVARAGE_MIN;i++) 00126 { 00127 printf("[%d]=%f\r\n",i,cpm_array[i]); 00128 } 00129 printf("first_touch_index=%d\r\n",first_touch_index); 00130 printf("cpm_index=%d\r\n",cpm_index); 00131 00132 00133 } 00134 //interuput giger pin 00135 void geiger_countup() 00136 { 00137 //if sleeptime countup stoped. 00138 if(!working) 00139 return; 00140 00141 cnt++; 00142 cpm_array[cpm_index]=cnt; 00143 calculate_sv(); 00144 // debug_print(); 00145 } 00146 00147 //geiger count after 1min 00148 void next_geiger_countup() 00149 { 00150 00151 cnt=0; 00152 cpm_index++; 00153 if(cpm_index >=AVARAGE_MIN) 00154 { 00155 cpm_index=0; 00156 } 00157 00158 //first touch index count up 00159 if(cpm_index>first_touch_index) 00160 { 00161 first_touch_index=cpm_index; 00162 } 00163 00164 cpm_array[cpm_index]=cnt; 00165 calculate_sv(); 00166 // debug_print(); 00167 } 00168 00169 00170 void setup_pinmode() 00171 { 00172 //p25 lowlevel = write mode? 00173 gigerin.mode(PullNone); 00174 gigerin.rise(&geiger_countup); 00175 } 00176 00177 00178 00179 //geiger system init 00180 void geiger_setup() 00181 { 00182 first_touch_index=0; 00183 cnt=0; 00184 setup_pinmode(); 00185 sec=time(NULL); 00186 strftime(buf,40, "%Y/%m/%d\n%X", localtime(&sec)); 00187 //fprintf(fp, "giger start %s \n",buf); 00188 output.cls(); 00189 output.printf("TIME%s",buf); 00190 wait(1); 00191 output.cls(); 00192 output.printf("please wait...."); 00193 //lcd show setup 1sec print 00194 show.attach(&show_result,1.0); 00195 } 00196 00197 00198 void geigerrun() 00199 { 00200 00201 00202 #ifdef SD_USE 00203 //SD card write 00204 { 00205 FILE *fp = fopen("/sd/giger.txt", "a"); 00206 if(fp == NULL) { 00207 // error("Could not open file for write\n"); 00208 printf("please insert SD"); 00209 } 00210 else 00211 { 00212 sec=time(NULL); 00213 strftime(buf,40, "%Y/%m/%d %X", localtime(&sec)); 00214 fprintf(fp, "giger start %s \n",buf); 00215 fclose(fp); 00216 } 00217 } 00218 #endif 00219 geiger_setup(); 00220 while(1) 00221 { 00222 waiting=1; 00223 transon(); 00224 //400v voltage set waiting 00225 wait(1); 00226 waiting=0; 00227 00228 // 00229 //count up start 00230 // 00231 working=1; 00232 //count start 00233 cnt=0; 00234 calculate_sv(); 00235 wait(60); 00236 working=0; 00237 waiting=1; 00238 // 00239 // trans cooldown time 00240 // 00241 transoff(); 00242 //next countup move index 00243 next_geiger_countup(); 00244 00245 #ifdef SD_USE 00246 //SD card write gigier.txt 00247 { 00248 FILE *fp = fopen("/sd/giger.txt", "a"); 00249 if(fp == NULL) { 00250 //error("Could not open file for write\n"); 00251 printf("please insert SD"); 00252 } 00253 else 00254 { 00255 sec=time(NULL); 00256 strftime(buf,40, "%Y/%m/%d %X", localtime(&sec)); 00257 fprintf(fp, "%s %fuSv/h\n",buf,usv); 00258 fclose(fp); 00259 } 00260 } 00261 00262 //SD Card Write raw,txt 00263 { 00264 FILE *fp = fopen("/sd/raw.txt", "a"); 00265 if(fp == NULL) { 00266 printf("please insert SD"); 00267 } 00268 else 00269 { 00270 00271 sec=time(NULL); 00272 strftime(buf,40, "%Y/%m/%d %X", localtime(&sec)); 00273 fprintf(fp, "%s cpm=%d\n",buf,cpm_array[cpm_index]); 00274 fclose(fp); 00275 } 00276 00277 } 00278 #endif 00279 00280 //Sleep time for geiger lifetime safe 00281 wait(SLEEP_SEC); 00282 waiting=0; 00283 } 00284 00285 00286 } 00287
Generated on Tue Aug 2 2022 15:26:00 by
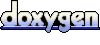