This library provides a class to generate differents signale wave form. Note that the maximum update rate of 1 MHz, so Fmax = 1MHz / _num_pixels (see UM10360 - Chapter 30: LPC17xx Digital-to-Analog Converter (DAC).
SignalGenerator Class Reference
This class provides methods to generate differents signale wave form. More...
#include <SignalGenerator.h>
Public Types | |
enum | SignalGeneratorType |
List of supported signals. More... | |
Public Member Functions | |
SignalGenerator (PinName p_outPort) | |
Default ctor. | |
virtual | ~SignalGenerator () |
Default dtor. | |
void | SetSignalFrequency (SignalGenerator::SignalGeneratorType p_signalType=SignalGenerator::SquareSignal, int p_frequency=1000, int p_num_pixels=64) |
Set the frequency of the signal. | |
void | Run () |
Generate the signal in synchrnous mode. | |
void | Stop () |
Method called to terminate synchronous running mode. | |
int | BeginRunAsync () |
Method called to prepare an asynchronous running mode. | |
void | RunAsync () |
Method called to launch the asynchronous running mode. | |
void | EndRunAsync () |
Method called to terminate asynchronous running mode. |
Detailed Description
This class provides methods to generate differents signale wave form.
V0.0.0.2
Note that the maximum update rate of 1 MHz, so Fmax = 1MHz / _num_pixels
Note that Vout is given by the relation below (UM10360 - Table 537. D/A Pin Description): Vout = VALUE * ((Vrefp - Vrefn) / 1024) + Vrefn in which:
- Vrefp: tied to VDD (e.g. 3.3V)
- Vrefn: tied to Vss
- See also:
- UM10360 - Chapter 30: LPC17xx Digital-to-Analog Converter (DAC)
- Remarks:
- This class was validated with Tektronix TDS2014 oscilloscope
http://fabrice.sincere.pagesperso-orange.fr/cm_electronique/projet_pic/gbf/gbf.htm
Definition at line 44 of file SignalGenerator.h.
Member Enumeration Documentation
enum SignalGeneratorType |
List of supported signals.
Definition at line 49 of file SignalGenerator.h.
Constructor & Destructor Documentation
SignalGenerator | ( | PinName | p_outPort ) |
Default ctor.
Initialize the internal datas. Default values are:
- signal type: SquareSignal
- frequency: 1000Hz
- # of pixels is fixed, _twait is adjusted
- Parameters:
-
p_outPort,: Pin name of the Analog out port (e.g. p18 for LPC1768)
Definition at line 5 of file SignalGenerator.cpp.
~SignalGenerator | ( | ) | [virtual] |
Member Function Documentation
int BeginRunAsync | ( | ) |
Method called to prepare an asynchronous running mode.
- Returns:
- 0 on success, -1 if _twait less that 7us. On error, you shall use synchronous method
Definition at line 83 of file SignalGenerator.cpp.
void EndRunAsync | ( | ) |
Method called to terminate asynchronous running mode.
Definition at line 103 of file SignalGenerator.cpp.
void Run | ( | ) |
Generate the signal in synchrnous mode.
- See also:
- Stop() method
Definition at line 64 of file SignalGenerator.cpp.
void RunAsync | ( | ) |
Method called to launch the asynchronous running mode.
Definition at line 95 of file SignalGenerator.cpp.
void SetSignalFrequency | ( | SignalGenerator::SignalGeneratorType | p_signalType = SignalGenerator::SquareSignal , |
int | p_frequency = 1000 , |
||
int | p_num_pixels = 64 |
||
) |
Set the frequency of the signal.
- Parameters:
-
p_signalType,: Signal type. Default value is square signal p_frequency,: Signal frequency. Default value is 1000Hz p_num_pixels,: Number of pixels ber period. Default value is 64
- See also:
- UM10360 - Chapter 30: LPC17xx Digital-to-Analog Converter (DAC)
// Prepare a sinus signal at 500Hz signal.SetSignalFrequency(SignalGenerator::SinusSignal,500); // Launch execution signal.BeginRunAsync(); // Here signal generation is running. Use Led or oscilloscope to vizualize output printf("Press any key to terminate"); getchar(); // Terminate execution signal.EndRunAsync();
Definition at line 19 of file SignalGenerator.cpp.
void Stop | ( | ) |
Method called to terminate synchronous running mode.
Definition at line 77 of file SignalGenerator.cpp.
Generated on Tue Jul 19 2022 21:58:57 by
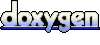