This library provides a class to generate differents signale wave form. Note that the maximum update rate of 1 MHz, so Fmax = 1MHz / _num_pixels (see UM10360 - Chapter 30: LPC17xx Digital-to-Analog Converter (DAC).
SignalGenerator.cpp
00001 #include "SignalGenerator.h" 00002 00003 #define PI 3.1415 00004 00005 SignalGenerator::SignalGenerator(PinName p_outPort) : _out(p_outPort), _asyncTicker(), _debugLed(LED4) { 00006 _debugLed = 0; 00007 _mode = true; // _num_pixels is fixed, _twait is computed 00008 _stop = false; 00009 _values = NULL; 00010 SetSignalFrequency(); 00011 } // End of SignalGenerator::SignalGenerator 00012 00013 SignalGenerator::~SignalGenerator() { 00014 if (_values != NULL) { 00015 delete [] _values; 00016 } 00017 } // End of SignalGenerator::~SignalGenerator 00018 00019 void SignalGenerator::SetSignalFrequency(SignalGenerator::SignalGeneratorType p_signalType, int p_frequency, int p_num_pixels) { 00020 DEBUG_ENTER("SignalGenerator::SetSignalFrequency") 00021 if (_values != NULL) { 00022 DEBUG("SignalGenerator::SetSignalFrequency: Delete _values"); 00023 delete [] _values; 00024 } 00025 00026 _signalType = p_signalType; 00027 _frequency = p_frequency; 00028 _num_pixels = p_num_pixels; 00029 DEBUG("SignalGenerator::SetSignalFrequency: _frequency=%d", _frequency) 00030 if (_mode) { 00031 //_twait = 1000000.0f / (_frequency * _num_pixels); // _num_pixels is set to 1000 pixels/T 00032 _twait = 1.0f / (_frequency * _num_pixels); // _num_pixels is set to 1000 pixels/T 00033 } else { 00034 _twait = 0.000001f; // 1us 00035 _num_pixels = (int)(1.0f / (_frequency * _twait)); // t_w is set to 1us and we fix at least 1000 pixels per period (T >> t_w): T >= 1000 * t_w ==> F < 1KHz 00036 } 00037 DEBUG("SignalGenerator::SetSignalFrequency: _twait=%f", _twait) 00038 DEBUG("SignalGenerator::SetSignalFrequency: _num_pixels=%d", _num_pixels) 00039 _values = new float[_num_pixels]; 00040 PrepareSignal(); 00041 DEBUG_LEAVE("SignalGenerator::SetSignalFrequency") 00042 } // End of SignalGenerator::SetSignalFrequency 00043 00044 void SignalGenerator::PrepareSignal() { 00045 switch (_signalType) { 00046 case SquareSignal: 00047 PrepareSquareSignal(); 00048 break; 00049 case TriangleSignal: 00050 PrepareTriangleSignal(); 00051 break; 00052 case SawtoothSignal: 00053 PrepareSawtoothSignal(); 00054 break; 00055 case ReverseSawtoothSignal: 00056 PrepareReverseSawtoothSignal(); 00057 break; 00058 case SinusSignal: 00059 PrepareSinusSignal(); 00060 break; 00061 } // End of 'switch' statement 00062 } // End of SignalGenerator::PrepareSignal 00063 00064 void SignalGenerator::Run() { 00065 DEBUG_ENTER("SignalGenerator::Run") 00066 do { 00067 for (int i = 0; i < _num_pixels; i++) { 00068 _out = *(_values + i); 00069 //DEBUG_1f("SignalGenerator::Run: Current=", (float)_out) 00070 wait(_twait); 00071 } 00072 } while (!_stop); 00073 _out = 0.0f; // Reset output 00074 DEBUG_LEAVE("SignalGenerator::Run") 00075 } // End of SignalGenerator::Run 00076 00077 void SignalGenerator::Stop() { 00078 DEBUG_ENTER("SignalGenerator::Stop") 00079 _stop = true; 00080 DEBUG_LEAVE("SignalGenerator::Stop") 00081 } // End of SignalGenerator::Stop 00082 00083 int SignalGenerator::BeginRunAsync() { 00084 DEBUG_ENTER("SignalGenerator::BeginRunAsync") 00085 if (_twait < 0.000007f) { 00086 return -1; 00087 } else { 00088 _asynckCounter = 0; 00089 _asyncTicker.attach(this, &SignalGenerator::RunAsync, _twait); 00090 } 00091 DEBUG_LEAVE("SignalGenerator::BeginRunAsync") 00092 return 0; 00093 } // End of SignalGenerator::BeginRunAsync 00094 00095 void SignalGenerator::RunAsync() { 00096 //DEBUG_ENTER("SignalGenerator::RunAsync") 00097 _debugLed = !_debugLed; 00098 _out = *(_values + _asynckCounter++); 00099 _asynckCounter %= _num_pixels; 00100 //DEBUG_LEAVE("SignalGenerator::RunAsync") 00101 } // End of SignalGenerator::RunAsync 00102 00103 void SignalGenerator::EndRunAsync() { 00104 DEBUG_ENTER("SignalGenerator::EndRunAsync") 00105 _asyncTicker.detach(); 00106 _out = 0.0f; // Reset output 00107 DEBUG_LEAVE("SignalGenerator::EndRunAsync") 00108 } // End of SignalGenerator::EndRunAsync 00109 00110 SignalGenerator& SignalGenerator::operator =(const int& p_frequency) { 00111 DEBUG_ENTER("SignalGenerator::operator =") 00112 if (_frequency != p_frequency) { 00113 this->SetSignalFrequency(_signalType, p_frequency); 00114 } 00115 DEBUG_LEAVE("SignalGenerator::operator =") 00116 return *this; 00117 } // End of SignalGenerator::operator = 00118 00119 SignalGenerator& SignalGenerator::operator =(const bool& p_mode) { 00120 DEBUG_ENTER("SignalGenerator::operator =") 00121 if (_mode != p_mode) { 00122 _mode = p_mode; 00123 this->SetSignalFrequency(_signalType, _frequency); 00124 } 00125 DEBUG_LEAVE("SignalGenerator::operator =") 00126 return *this; 00127 } // End of SignalGenerator::operator = 00128 00129 SignalGenerator& SignalGenerator::operator =(const SignalGenerator::SignalGeneratorType& p_signalType) { 00130 DEBUG_ENTER("SignalGenerator::operator =") 00131 this->SetSignalFrequency(p_signalType, _frequency); 00132 DEBUG_LEAVE("SignalGenerator::operator =") 00133 return *this; 00134 00135 } // End of SignalGenerator::operator = 00136 00137 void SignalGenerator::PrepareSquareSignal() { 00138 DEBUG_ENTER("SignalGenerator::PrepareSquareSignal") 00139 int step = _num_pixels / 2; 00140 for (int i = 0; i < step; i += 1) { 00141 *(_values + i) = 1.0f; 00142 } // End of 'for' statement 00143 for (int i = step; i < _num_pixels; i += 1) { 00144 *(_values + i) = 0.0f; 00145 } // End of 'for' statement 00146 DEBUG_ENTER("SignalGenerator::PrepareSquareSignal") 00147 } // End of SignalGenerator::PrepareSquareSignal 00148 00149 void SignalGenerator::PrepareTriangleSignal() { 00150 float step = 1.0f / (_num_pixels / 2.0f); 00151 int counter = 0; 00152 float i = 0.0f; 00153 for ( ; i < 1.0f; i += step) { 00154 *(_values + counter++) = i; 00155 } // End of 'for' statement 00156 for ( ; i >= 0.0f; i -= step) { 00157 *(_values + counter++) = i; 00158 } // End of 'for' statement 00159 } // End of SignalGenerator::PrepareTriangleSignal 00160 00161 void SignalGenerator::PrepareSawtoothSignal() { 00162 float step = 1.0f / _num_pixels; 00163 int counter = 0; 00164 for (float i = 0.0f; i < 1.0f; i += step) { 00165 *(_values + counter++) = i; 00166 } // End of 'for' statement 00167 } // End of SignalGenerator::PrepareSawtoothSignal 00168 00169 void SignalGenerator::PrepareReverseSawtoothSignal() { 00170 float step = 1.0f / _num_pixels; 00171 int counter = 0; 00172 for (float i = 0.0f; i < 1.0f; i += step) { 00173 *(_values + counter++) = (1.0f - i); 00174 } // End of 'for' statement 00175 } // End of SignalGenerator::PrepareReverseSawtoothSignal 00176 00177 void SignalGenerator::PrepareSinusSignal() { 00178 float step = 1.0f / _num_pixels; 00179 int counter = 0; 00180 for (float i = 0.0f; i < 1.0f; i += step) { 00181 *(_values + counter++) = sin(2 * PI * i); 00182 } // End of 'for' statement 00183 } // End of SignalGenerator::PrepareSinusSignal
Generated on Tue Jul 19 2022 21:58:57 by
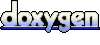