This library contains a simple device driver for the X10 CM17a module. It also contains a simple X10Server, which listens for network commands to be forwarded to the module.
X10Server.h
00001 00002 #ifndef X10SERVER_H 00003 #define X10SERVER_H 00004 00005 #include "mbed.h" 00006 #include "rtos.h" 00007 #include "EthernetInterface.h" 00008 00009 /// The X10Server class, which will listen for X10 commands from 00010 /// the network. 00011 /// 00012 class X10Server 00013 { 00014 public: 00015 /// X10Server Constructor, which also assigns the port. 00016 /// 00017 /// This constructs the X10Server object, and sets it to 00018 /// listen for incoming messages. 00019 /// 00020 /// @param[in] fptr is an optional pointer to a function that can 00021 /// accept and process the received message. 00022 /// This function is called with a pointer to the message and 00023 /// the size of the message as parameters. 00024 /// @param[in] port is an optional parameter to set the port 00025 /// to listen to. The default is 10630. 00026 /// 00027 X10Server(void(*fptr)(char * buffer, int size) = NULL, uint16_t port = 10630); 00028 00029 /// The process to call where there is free time, to see 00030 /// if there is a request, and to handle it. 00031 /// 00032 void poll(void); 00033 00034 private: 00035 void (*callback)(char * buffer, int size); 00036 uint16_t listenPort; 00037 TCPSocketServer svr; 00038 TCPSocketConnection client; 00039 bool serverIsListening; 00040 bool clientIsConnected; 00041 }; 00042 00043 #endif // X10SERVER_H
Generated on Mon Jul 25 2022 09:04:25 by
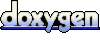