Fixed custom headers and Basic authorization, added support for redirection, functional file download interface can be used for SW updates and more.
Dependents: Sample_HTTPClient Sample_HTTPClient LWM2M_NanoService_Ethernet LWM2M_NanoService_Ethernet ... more
Fork of HTTPClient by
HTTPiCal Class Reference
An iCal handling mechanism - downloads and parses calendar events. More...
#include <HTTPiCal.h>
Inherits IHTTPDataIn.
Data Structures | |
struct | Event_T |
A single event consists of quite a number of attributes. More... | |
Public Types | |
enum | RepeatFreq_t { rptfNone, rptfDaily, rptfWeekly, rptfMonthly, rptfYearly } |
The repeat attribute for an event. More... | |
Public Member Functions | |
HTTPiCal (int count) | |
Instantiate HTTPiCal. | |
~HTTPiCal () | |
Destructor to free memory. | |
void | SetTimeWindow (time_t StartTime=0, time_t EndTime=0) |
Set the time window of interest, for which to retain events. | |
int | GetEventCount (void) |
Get the count of Events currently available. | |
bool | GetEvent (unsigned int i, Event_T *event) |
Get a copy of the specified event. | |
bool | RepeatIntersects (time_t *start1, time_t *end1, time_t *start2, time_t *end2, Event_T *Event=NULL) |
Compute the intersection of two time ranges, and evaluate the recurringing events. | |
bool | TimeIntersects (time_t *start1, time_t *end1, time_t *start2, time_t *end2) |
Compute the intersection of two time ranges, and returns that intersection. | |
Protected Member Functions | |
virtual void | writeReset () |
Reset stream to its beginning Called by the HTTPClient on each new request. | |
virtual int | write (const char *buf, size_t len) |
Write a piece of data transmitted by the server. | |
virtual void | setDataType (const char *type) |
Set MIME type. | |
virtual void | setIsChunked (bool chunked) |
Determine whether the data is chunked Recovered from Transfer-Encoding header. | |
virtual void | setDataLen (size_t len) |
If the data is not chunked, set its size From Content-Length header. | |
Friends | |
class | HTTPClient |
Detailed Description
An iCal handling mechanism - downloads and parses calendar events.
Definition at line 8 of file HTTPiCal.h.
Member Enumeration Documentation
enum RepeatFreq_t |
The repeat attribute for an event.
- Enumerator:
rptfNone no repeat for this event
rptfDaily daily repeat
rptfWeekly weekly repeat
rptfMonthly monthly repeat
rptfYearly yearly repeat
Definition at line 17 of file HTTPiCal.h.
Constructor & Destructor Documentation
HTTPiCal | ( | int | count ) |
Instantiate HTTPiCal.
HTTPClient http; HTTPiCal iCal(10); // define a limit of 10 events to be held http.basicAuth(calInfo.user, calInfo.pass); time_t now = t.timelocal(); // Set a 4-hour window from now time_t nxt = now + 4 * 3600; ical.SetTimeWindow(now, nxt); HTTPErrorCode = http.get(calInfo.url, &iCal); if (HTTPErrorCode == HTTP_OK) { // calendar successfully downloaded for (int i=0; i<iCal.GetEventCount(); i++) { HTTPiCal::Event_T event; if (ical.GetEvent(i, &event)) { printf("Event %d\r\n", i); printf("Summary : %s\r\n", event.Summary); printf("Location: %s\r\n", event.Location); printf("Category: %s\r\n", event.Category); } } }
- Parameters:
-
count is the number of Event_T entries to reserve space for.
Definition at line 23 of file HTTPiCal.cpp.
~HTTPiCal | ( | ) |
Destructor to free memory.
Definition at line 34 of file HTTPiCal.cpp.
Member Function Documentation
bool GetEvent | ( | unsigned int | i, |
Event_T * | event | ||
) |
Get a copy of the specified event.
- Parameters:
-
i is the event number, ranging from 0 to GetEventCount() event is a pointer to where the event will be copied.
- Returns:
- true if the event was available and copied.
Definition at line 44 of file HTTPiCal.cpp.
int GetEventCount | ( | void | ) |
Get the count of Events currently available.
- Returns:
- the count of events.
Definition at line 97 of file HTTPiCal.h.
bool RepeatIntersects | ( | time_t * | start1, |
time_t * | end1, | ||
time_t * | start2, | ||
time_t * | end2, | ||
Event_T * | Event = NULL |
||
) |
Compute the intersection of two time ranges, and evaluate the recurringing events.
This compares a pair of time ranges, each by a start and end time. If they overlap it then computes the intersection of those two ranges. Additionally, for a specified Event, it will evaluate the recurring events that may also fall into the target time range.
- Note:
- This is usually the only API you need, as this will first call the TimeIntersects function, and if that fails, then it will evaluate repeat information.
- Parameters:
-
[in,out] start1 is the starting time of range 1. [in,out] end1 is the ending time of range 1. [in] start2 is the starting time of range 2. [in] end2 is the ending time of range 2. [in] Event is a pointer to the event of interest that may have recurring series.
- Returns:
- true if the ranges overlap, and then start1 and end1 are set to the intersection.
Definition at line 351 of file HTTPiCal.cpp.
void setDataLen | ( | size_t | len ) | [protected, virtual] |
If the data is not chunked, set its size From Content-Length header.
- Parameters:
-
[in] len defines the size of the non-chunked transfer.
Implements IHTTPDataIn.
Definition at line 111 of file HTTPiCal.cpp.
void setDataType | ( | const char * | type ) | [protected, virtual] |
Set MIME type.
- Parameters:
-
[in] type Internet media type from Content-Type header
Implements IHTTPDataIn.
Definition at line 98 of file HTTPiCal.cpp.
void setIsChunked | ( | bool | chunked ) | [protected, virtual] |
Determine whether the data is chunked Recovered from Transfer-Encoding header.
- Parameters:
-
[in] chunked indicates the transfer is chunked.
Implements IHTTPDataIn.
Definition at line 106 of file HTTPiCal.cpp.
void SetTimeWindow | ( | time_t | StartTime = 0 , |
time_t | EndTime = 0 |
||
) |
Set the time window of interest, for which to retain events.
This sets the time window of interest. Any event, whether directly scheduled in this window, or indirectly via repeat attributes, will be retained in the list of available events. Any event not in this window will be ignored.
- Parameters:
-
StartTime is the optional time value for the beginning of interest. If gridStartTime is zero, no filtering is performed. EndTime is the optional time value ending the period of interest.
Definition at line 39 of file HTTPiCal.cpp.
bool TimeIntersects | ( | time_t * | start1, |
time_t * | end1, | ||
time_t * | start2, | ||
time_t * | end2 | ||
) |
Compute the intersection of two time ranges, and returns that intersection.
Computes the intersection of time1 and time2 ranges, and modifies time1 range to represent the intersection.
This compares a pair of time ranges, each by a start and end time. If they overlap it then computes the intersection of those two ranges.
- Parameters:
-
[in,out] start1 is the starting time of range 1. [in,out] end1 is the ending time of range 1. [in] start2 is the starting time of range 2. [in] end2 is the ending time of range 2.
- Returns:
- true if the ranges overlap, and then start1 and end1 are set to the intersection.
start1 is input as the start of the time1 range, and is written to represent the intersection of the two ranges. end1 is input as the end of the time1 range and is written to represent the intersection of the two ranges. start2 is the start of the time2 range. end2 is the end of the time2 range. returns true if the ranges have an intersection, and the time1 range values have been modified.
Definition at line 255 of file HTTPiCal.cpp.
int write | ( | const char * | buf, |
size_t | len | ||
) | [protected, virtual] |
Write a piece of data transmitted by the server.
- Parameters:
-
[in] buf Pointer to the buffer from which to copy the data [in] len Length of the buffer
- Returns:
- number of bytes written.
Implements IHTTPDataIn.
Definition at line 60 of file HTTPiCal.cpp.
void writeReset | ( | ) | [protected, virtual] |
Reset stream to its beginning Called by the HTTPClient on each new request.
Implements IHTTPDataIn.
Definition at line 53 of file HTTPiCal.cpp.
Generated on Tue Jul 12 2022 17:30:36 by
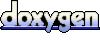