Added ability to use printf directly
Fork of LCD_DISCO_F429ZI by
Embed:
(wiki syntax)
Show/hide line numbers
LCD_DISCO_F429ZI.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef __LCD_DISCO_F429ZI_H 00020 #define __LCD_DISCO_F429ZI_H 00021 00022 #ifdef TARGET_DISCO_F429ZI 00023 00024 #include "mbed.h" 00025 #include "stm32f429i_discovery_lcd.h" 00026 00027 /* 00028 This class drives the LCD display (ILI9341 240x320) present on DISCO_F429ZI board. 00029 00030 Usage: 00031 00032 #include "mbed.h" 00033 #include "LCD_DISCO_F429ZI.h" 00034 00035 LCD_DISCO_F429ZI lcd; 00036 00037 int main() 00038 { 00039 lcd.Clear(LCD_COLOR_BLUE); 00040 lcd.SetBackColor(LCD_COLOR_BLUE); 00041 lcd.SetTextColor(LCD_COLOR_WHITE); 00042 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"HELLO", CENTER_MODE); 00043 while(1) 00044 { 00045 } 00046 } 00047 */ 00048 class LCD_DISCO_F429ZI : public Stream //use Stream base class 00049 { 00050 00051 public: 00052 //! Constructor 00053 LCD_DISCO_F429ZI(); 00054 00055 //! Destructor 00056 ~LCD_DISCO_F429ZI(); 00057 00058 /** 00059 * @brief Initializes the LCD. 00060 * @param None 00061 * @retval LCD state 00062 */ 00063 uint8_t Init(void); 00064 00065 /** 00066 * @brief Gets the LCD X size. 00067 * @param None 00068 * @retval The used LCD X size 00069 */ 00070 uint32_t GetXSize(void); 00071 00072 /** 00073 * @brief Gets the LCD Y size. 00074 * @param None 00075 * @retval The used LCD Y size 00076 */ 00077 uint32_t GetYSize(void); 00078 00079 /** 00080 * @brief Initializes the LCD layers. 00081 * @param LayerIndex: the layer foreground or background. 00082 * @param FB_Address: the layer frame buffer. 00083 * @retval None 00084 */ 00085 void LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address); 00086 00087 /** 00088 * @brief Selects the LCD Layer. 00089 * @param LayerIndex: the Layer foreground or background. 00090 * @retval None 00091 */ 00092 void SelectLayer(uint32_t LayerIndex); 00093 00094 /** 00095 * @brief Sets a LCD Layer visible. 00096 * @param LayerIndex: the visible Layer. 00097 * @param state: new state of the specified layer. 00098 * This parameter can be: ENABLE or DISABLE. 00099 * @retval None 00100 */ 00101 void SetLayerVisible(uint32_t LayerIndex, FunctionalState state); 00102 00103 /** 00104 * @brief Configures the Transparency. 00105 * @param LayerIndex: the Layer foreground or background. 00106 * @param Transparency: the Transparency, 00107 * This parameter must range from 0x00 to 0xFF. 00108 * @retval None 00109 */ 00110 void SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00111 00112 /** 00113 * @brief Sets a LCD layer frame buffer address. 00114 * @param LayerIndex: specifies the Layer foreground or background 00115 * @param Address: new LCD frame buffer value 00116 * @retval None 00117 */ 00118 void SetLayerAddress(uint32_t LayerIndex, uint32_t Address); 00119 00120 /** 00121 * @brief Sets the Display window. 00122 * @param LayerIndex: layer index 00123 * @param Xpos: LCD X position 00124 * @param Ypos: LCD Y position 00125 * @param Width: LCD window width 00126 * @param Height: LCD window height 00127 * @retval None 00128 */ 00129 void SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00130 00131 /** 00132 * @brief Configures and sets the color Keying. 00133 * @param LayerIndex: the Layer foreground or background 00134 * @param RGBValue: the Color reference 00135 * @retval None 00136 */ 00137 void SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00138 00139 /** 00140 * @brief Disables the color Keying. 00141 * @param LayerIndex: the Layer foreground or background 00142 * @retval None 00143 */ 00144 void ResetColorKeying(uint32_t LayerIndex); 00145 00146 /** 00147 * @brief Gets the LCD Text color. 00148 * @param None 00149 * @retval Text color 00150 */ 00151 uint32_t GetTextColor(void); 00152 00153 /** 00154 * @brief Gets the LCD Background color. 00155 * @param None 00156 * @retval Background color 00157 */ 00158 uint32_t GetBackColor(void); 00159 00160 /** 00161 * @brief Sets the Text color. 00162 * @param Color: the Text color code ARGB(8-8-8-8); 00163 * @retval None 00164 */ 00165 void SetTextColor(uint32_t Color); 00166 00167 /** 00168 * @brief Sets the Background color. 00169 * @param Color: the layer Background color code ARGB(8-8-8-8); 00170 * @retval None 00171 */ 00172 void SetBackColor(uint32_t Color); 00173 00174 /** 00175 * @brief Sets the Text Font. 00176 * @param pFonts: the layer font to be used 00177 * @retval None 00178 */ 00179 void SetFont(sFONT *pFonts); 00180 00181 /** 00182 * @brief Gets the Text Font. 00183 * @param None 00184 * @retval Layer font 00185 */ 00186 sFONT *GetFont(void); 00187 00188 /** 00189 * @brief Reads Pixel. 00190 * @param Xpos: the X position 00191 * @param Ypos: the Y position 00192 * @retval RGB pixel color 00193 */ 00194 uint32_t ReadPixel(uint16_t Xpos, uint16_t Ypos); 00195 00196 /** 00197 * @brief Clears the hole LCD. 00198 * @param Color: the color of the background 00199 * @retval None 00200 */ 00201 void Clear(uint32_t Color); 00202 00203 /** 00204 * @brief Clears the selected line. 00205 * @param Line: the line to be cleared 00206 * @retval None 00207 */ 00208 void ClearStringLine(uint32_t Line); 00209 00210 /** 00211 * @brief Displays one character. 00212 * @param Xpos: start column address 00213 * @param Ypos: the Line where to display the character shape 00214 * @param Ascii: character ascii code, must be between 0x20 and 0x7E 00215 * @retval None 00216 */ 00217 void DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00218 00219 /** 00220 * @brief Moves cursor. 00221 * @param Xpos: start column address 00222 * @param Ypos: the Line where to display the character shape 00223 * @retval None 00224 */ 00225 void Cursor(uint16_t Xpos, uint16_t Ypos); 00226 00227 /** 00228 * @brief Displays a maximum of 60 char on the LCD. 00229 * @param X: pointer to x position (in pixel); 00230 * @param Y: pointer to y position (in pixel); 00231 * @param pText: pointer to string to display on LCD 00232 * @param mode: The display mode 00233 * This parameter can be one of the following values: 00234 * @arg CENTER_MODE 00235 * @arg RIGHT_MODE 00236 * @arg LEFT_MODE 00237 * @retval None 00238 */ 00239 void DisplayStringAt(uint16_t X, uint16_t Y, uint8_t *pText, Text_AlignModeTypdef mode); 00240 00241 /** 00242 * @brief Displays a maximum of 20 char on the LCD. 00243 * @param Line: the Line where to display the character shape 00244 * @param ptr: pointer to string to display on LCD 00245 * @retval None 00246 */ 00247 void DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00248 00249 /** 00250 * @brief Displays an horizontal line. 00251 * @param Xpos: the X position 00252 * @param Ypos: the Y position 00253 * @param Length: line length 00254 * @retval None 00255 */ 00256 void DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00257 00258 /** 00259 * @brief Displays a vertical line. 00260 * @param Xpos: the X position 00261 * @param Ypos: the Y position 00262 * @param Length: line length 00263 * @retval None 00264 */ 00265 void DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00266 00267 /** 00268 * @brief Displays an uni-line (between two points);. 00269 * @param X1: the point 1 X position 00270 * @param Y1: the point 1 Y position 00271 * @param X2: the point 2 X position 00272 * @param Y2: the point 2 Y position 00273 * @retval None 00274 */ 00275 void DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2); 00276 00277 /** 00278 * @brief Displays a rectangle. 00279 * @param Xpos: the X position 00280 * @param Ypos: the Y position 00281 * @param Height: display rectangle height 00282 * @param Width: display rectangle width 00283 * @retval None 00284 */ 00285 void DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00286 00287 /** 00288 * @brief Displays a circle. 00289 * @param Xpos: the X position 00290 * @param Ypos: the Y position 00291 * @param Radius: the circle radius 00292 * @retval None 00293 */ 00294 void DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00295 00296 /** 00297 * @brief Displays an poly-line (between many points);. 00298 * @param Points: pointer to the points array 00299 * @param PointCount: Number of points 00300 * @retval None 00301 */ 00302 void DrawPolygon(pPoint Points, uint16_t PointCount); 00303 00304 /** 00305 * @brief Displays an Ellipse. 00306 * @param Xpos: the X position 00307 * @param Ypos: the Y position 00308 * @param XRadius: the X radius of ellipse 00309 * @param YRadius: the Y radius of ellipse 00310 * @retval None 00311 */ 00312 void DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00313 00314 /** 00315 * @brief Displays a bitmap picture loaded in the internal Flash (32 bpp);. 00316 * @param X: the bmp x position in the LCD 00317 * @param Y: the bmp Y position in the LCD 00318 * @param pBmp: Bmp picture address in the internal Flash 00319 * @retval None 00320 */ 00321 void DrawBitmap(uint32_t X, uint32_t Y, uint8_t *pBmp); 00322 00323 /** 00324 * @brief Displays a full rectangle. 00325 * @param Xpos: the X position 00326 * @param Ypos: the Y position 00327 * @param Height: rectangle height 00328 * @param Width: rectangle width 00329 * @retval None 00330 */ 00331 void FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00332 00333 /** 00334 * @brief Displays a full circle. 00335 * @param Xpos: the X position 00336 * @param Ypos: the Y position 00337 * @param Radius: the circle radius 00338 * @retval None 00339 */ 00340 void FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00341 00342 /** 00343 * @brief Fill triangle. 00344 * @param X1: the point 1 x position 00345 * @param Y1: the point 1 y position 00346 * @param X2: the point 2 x position 00347 * @param Y2: the point 2 y position 00348 * @param X3: the point 3 x position 00349 * @param Y3: the point 3 y position 00350 * @retval None 00351 */ 00352 void FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3); 00353 00354 /** 00355 * @brief Displays a full poly-line (between many points);. 00356 * @param Points: pointer to the points array 00357 * @param PointCount: Number of points 00358 * @retval None 00359 */ 00360 void FillPolygon(pPoint Points, uint16_t PointCount); 00361 00362 /** 00363 * @brief Draw a full ellipse. 00364 * @param Xpos: the X position 00365 * @param Ypos: the Y position 00366 * @param XRadius: X radius of ellipse 00367 * @param YRadius: Y radius of ellipse. 00368 * @retval None 00369 */ 00370 void FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00371 00372 /** 00373 * @brief Enables the Display. 00374 * @param None 00375 * @retval None 00376 */ 00377 void DisplayOn(void); 00378 00379 /** 00380 * @brief Disables the Display. 00381 * @param None 00382 * @retval None 00383 */ 00384 void DisplayOff(void); 00385 00386 /** 00387 * @brief Writes Pixel. 00388 * @param Xpos: the X position 00389 * @param Ypos: the Y position 00390 * @param RGB_Code: the pixel color in ARGB mode (8-8-8-8); 00391 * @retval None 00392 */ 00393 void DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code); 00394 00395 private: 00396 uint16_t cursorX; 00397 uint16_t cursorY; 00398 00399 protected: 00400 //used by printf 00401 virtual int _putc(int c) { 00402 if( COLUMN(cursorX+1) >= BSP_LCD_GetXSize() ) { 00403 cursorX = 0; 00404 cursorY++; 00405 } 00406 if( c > 31 && c <127 ) { 00407 BSP_LCD_DisplayChar(COLUMN(cursorX), LINE(cursorY), c); 00408 cursorX++; 00409 } 00410 // TODO: Handle wraparound based on font size 00411 if( c == '\n' ) cursorX = 0; 00412 if( c == '\r' ) cursorY++; 00413 return 0; 00414 }; 00415 //assuming no reads from LCD 00416 virtual int _getc() { 00417 return -1; 00418 } 00419 }; 00420 00421 #else 00422 #error "This class must be used with DISCO_F429ZI board only." 00423 #endif // TARGET_DISCO_F429ZI 00424 00425 #endif
Generated on Fri Jul 22 2022 18:00:16 by
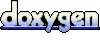