Software serial, for when you are out of serial pins
Dependents: BufferedSoftSerial neurGAI_Seeed_BLUETOOTH LPC-SD-35 ESP-WROOM-02_test ... more
SoftSerial Class Reference
A software serial implementation. More...
#include <SoftSerial.h>
Public Member Functions | |
SoftSerial (PinName TX, PinName RX, const char *name=NULL) | |
Constructor. | |
void | baud (int baudrate) |
Set the baud rate of the serial port. | |
void | format (int bits=8, Parity parity=SoftSerial::None, int stop_bits=1) |
Set the transmission format used by the serial port. | |
int | readable () |
Determine if there is a character available to read. | |
int | writeable () |
Determine if there is space available to write a character. | |
void | attach (void(*fptr)(void), IrqType type=RxIrq) |
Attach a function to call whenever a serial interrupt is generated. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(void), IrqType type=RxIrq) |
Attach a member function to call whenever a serial interrupt is generated. | |
void | send_break () |
Generate a break condition on the serial line. |
Detailed Description
A software serial implementation.
Definition at line 9 of file SoftSerial.h.
Constructor & Destructor Documentation
SoftSerial | ( | PinName | TX, |
PinName | RX, | ||
const char * | name = NULL |
||
) |
Constructor.
- Parameters:
-
TX Name of the TX pin, NC for not connected RX Name of the RX pin, NC for not connected, must be capable of being InterruptIn name Name of the connection
Definition at line 3 of file SoftSerial.cpp.
Member Function Documentation
void attach | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
IrqType | type = RxIrq |
||
) |
Attach a member function to call whenever a serial interrupt is generated.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
Definition at line 81 of file SoftSerial.h.
void attach | ( | void(*)(void) | fptr, |
IrqType | type = RxIrq |
||
) |
Attach a function to call whenever a serial interrupt is generated.
- Parameters:
-
fptr A pointer to a void function, or 0 to set as none type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
Definition at line 70 of file SoftSerial.h.
void baud | ( | int | baudrate ) |
Set the baud rate of the serial port.
- Parameters:
-
baudrate The baudrate of the serial port (default = 9600).
Definition at line 32 of file SoftSerial.cpp.
void format | ( | int | bits = 8 , |
Parity | parity = SoftSerial::None , |
||
int | stop_bits = 1 |
||
) |
Set the transmission format used by the serial port.
- Parameters:
-
bits The number of bits in a word (default = 8) parity The parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) stop The number of stop bits (default = 1)
Definition at line 36 of file SoftSerial.cpp.
int readable | ( | void | ) |
Determine if there is a character available to read.
- Returns:
- 1 if there is a character available to read, 0 otherwise
Definition at line 11 of file SoftSerial_rx.cpp.
void send_break | ( | void | ) |
Generate a break condition on the serial line.
Definition at line 13 of file SoftSerial_tx.cpp.
int writeable | ( | void | ) |
Determine if there is space available to write a character.
- Returns:
- 1 if there is space to write a character, 0 otherwise
Definition at line 22 of file SoftSerial_tx.cpp.
Generated on Wed Jul 13 2022 05:29:29 by
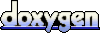