MODSERIAL with support for more devices
Dependents: 1D-Pong BMT-K9_encoder BMT-K9-Regelaar programma_filter ... more
The MODSERIAL API
Functions | |
int | rxDiscardLastChar (void) |
rxDiscardLastChar() | |
void | attach (void(*fptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
Function: attach. | |
template<typename T > | |
void | attach (T *tptr, void(T::*mptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
Function: attach. | |
void | connect (void(*fptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
template<typename T > | |
void | connect (T *tptr, void(T::*mptr)(MODSERIAL_IRQ_INFO *), IrqType type=RxIrq) |
int | writeable () |
Function: writeable. | |
int | readable () |
Function: readable. | |
bool | txBufferSane (void) |
Function: txBufferSane. | |
bool | rxBufferSane (void) |
Function: rxBufferSane. | |
int | txBufferGetCount (void) |
Function: txBufferGetCount. | |
int | rxBufferGetCount (void) |
Function: rxBufferGetCount. | |
int | txBufferGetSize (int size) |
Function: txBufferGetSize. | |
int | rxBufferGetSize (int size) |
Function: rxBufferGetSize. | |
bool | txBufferFull (void) |
Function: txBufferFull. | |
bool | rxBufferFull (void) |
Function: rxBufferFull. | |
bool | txBufferEmpty (void) |
Function: txBufferEmpty. | |
bool | rxBufferEmpty (void) |
Function: rxBufferEmpty. | |
int | txBufferSetSize (int size, bool m) |
Function: txBufferSetSize. | |
int | rxBufferSetSize (int size, bool m) |
Function: rxBufferSetSize. | |
int | txBufferSetSize (int size) |
Function: txBufferSetSize. | |
int | rxBufferSetSize (int size) |
Function: rxBufferSetSize. | |
void | txBufferFlush (void) |
Function: txBufferFlush. | |
void | rxBufferFlush (void) |
Function: rxBufferFlush. | |
int | getcNb () |
Function: getcNb. | |
int | getc () |
Function: getc. | |
void | autoDetectChar (char c) |
Function: autoDetectChar. | |
int | move (char *s, int max, char end) |
Function: move. | |
int | move (char *s, int max) |
Function: move (overloaded) | |
bool | claim (FILE *stream=stdout) |
Function: claim. | |
int | putc (int c) |
Function: putc. | |
int | printf (const char *format,...) |
Function: printf. | |
int | scanf (const char *format,...) |
Function: scanf. | |
bool | txIsBusy (void) |
Function: txIsBusy. |
Function Documentation
void attach | ( | void(*)(MODSERIAL_IRQ_INFO *) | fptr, |
IrqType | type = RxIrq |
||
) | [inherited] |
Function: attach.
The Mbed standard Serial library object allows an interrupt callback to be made when a byte is received by the TX or RX UART hardware. MODSERIAL traps these interrupts to enable it's buffering system. However, after the byte has been received/sent under interrupt control, MODSERIAL can callback a user function as a notification of the interrupt. Note, user code should not directly interact with the Uart hardware, MODSERIAL does that, instead, MODSERIAL API functions should be used.
Note, a character is written out then, if there is room in the TX FIFO and the TX buffer is empty, putc() will put the character directly into THR (the output holding register). If the TX FIFO is full and cannot accept the character, it is placed into the TX output buffer. The TX interrupts are then enabled so that when the TX FIFO empties, the TX buffer is then transferred to the THR FIFO. The TxIrq will ONLY be activated when this transfer of a character from BUFFER to THR FIFO takes place. If your character throughput is not high bandwidth, then the 16 byte TX FIFO may be enough and the TX output buffer may never come into play.
#include "mbed.h" #include "MODSERIAL.h" DigitalOut led1(LED1); DigitalOut led2(LED2); DigitalOut led3(LED3); // To test, connect p9 to p10 as a loopback. MODSERIAL pc(p9, p10); // This function is called when a character goes into the TX buffer. void txCallback(void) { led2 = !led2; } // This function is called when a character goes into the RX buffer. void rxCallback(void) { led3 = !led3; } int main() { pc.baud(115200); pc.attach(&txCallback, MODSERIAL::TxIrq); pc.attach(&rxCallback, MODSERIAL::RxIrq); while(1) { led1 = !led1; wait(0.5); pc.putc('A'); wait(0.5); } ]
- Parameters:
-
fptr A pointer to a void function, or 0 to set as none type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
Definition at line 367 of file MODSERIAL.h.
void attach | ( | T * | tptr, |
void(T::*)(MODSERIAL_IRQ_INFO *) | mptr, | ||
IrqType | type = RxIrq |
||
) | [inherited] |
Function: attach.
The Mbed standard Serial library object allows an interrupt callback to be made when a byte is received by the TX or RX UART hardware. MODSERIAL traps these interrupts to enable it's buffering system. However, after the byte has been received/sent under interrupt control, MODSERIAL can callback a user function as a notification of the interrupt. Note, user code should not directly interact with the Uart hardware, MODSERIAL does that, instead, MODSERIAL API functions should be used.
Note, a character is written out then, if there is room in the TX FIFO and the TX buffer is empty, putc() will put the character directly into THR (the output holding register). If the TX FIFO is full and cannot accept the character, it is placed into the TX output buffer. The TX interrupts are then enabled so that when the TX FIFO empties, the TX buffer is then transferred to the THR FIFO. The TxIrq will ONLY be activated when this transfer of a character from BUFFER to THR FIFO takes place. If your character throughput is not high bandwidth, then the 16 byte TX FIFO may be enough and the TX output buffer may never come into play.
#include "mbed.h" #include "MODSERIAL.h" DigitalOut led1(LED1); DigitalOut led2(LED2); DigitalOut led3(LED3); // To test, connect p9 to p10 as a loopback. MODSERIAL pc(p9, p10); class Foo { public: // This method is called when a character goes into the TX buffer. void txCallback(void) { led2 = !led2; } // This method is called when a character goes into the RX buffer. void rxCallback(void) { led3 = !led3; } }; Foo foo; int main() { pc.baud(115200); pc.attach(&foo, &Foo::txCallback, MODSERIAL::TxIrq); pc.attach(&foo, &Foo::rxCallback, MODSERIAL::RxIrq); while(1) { led1 = !led1; wait(0.5); pc.putc('A'); wait(0.5); } ]
- Parameters:
-
tptr A pointer to the object to call the member function on mptr A pointer to the member function to be called type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
Definition at line 429 of file MODSERIAL.h.
void autoDetectChar | ( | char | c ) | [inherited] |
Function: autoDetectChar.
Set the char that, if seen incoming, invokes the AutoDetectChar callback.
- Parameters:
-
int c The character to detect.
Definition at line 694 of file MODSERIAL.h.
bool claim | ( | FILE * | stream = stdout ) |
[inherited] |
Function: claim.
Redirect a stream to this MODSERIAL object
Important: A name parameter must have been added when creating the MODSERIAL object:
#include "MODSERIAL.h" ... MODSERIAL pc(USBTX, USBRX, "modser"); int main() { pc.claim() // capture <stdout> pc.printf("Uses the MODSERIAL library\r\n"); printf("So does this!\r\n"); }
- Parameters:
-
FILE *stream The stream to redirect (default = stdout)
- Returns:
- true if succeeded, else false
Definition at line 96 of file MODSERIAL.cpp.
void connect | ( | void(*)(MODSERIAL_IRQ_INFO *) | fptr, |
IrqType | type = RxIrq |
||
) | [inherited] |
- See also:
- attach
Definition at line 439 of file MODSERIAL.h.
void connect | ( | T * | tptr, |
void(T::*)(MODSERIAL_IRQ_INFO *) | mptr, | ||
IrqType | type = RxIrq |
||
) | [inherited] |
- See also:
- attach
Definition at line 446 of file MODSERIAL.h.
int getc | ( | ) | [inherited] |
Function: getc.
Overloaded version of Serial::getc()
This function blocks (if the RX buffer is empty the function will wait for a character to arrive and then return that character).
- Returns:
- A byte from the RX buffer
Definition at line 662 of file MODSERIAL.h.
int getcNb | ( | ) | [inherited] |
Function: getcNb.
Like getc() but is non-blocking. If no bytes are in the RX buffer this function returns Result::NoChar (-1)
- Returns:
- A byte from the RX buffer or Result::NoChar (-1) if bufer empty.
Definition at line 649 of file MODSERIAL.h.
int move | ( | char * | s, |
int | max, | ||
char | end | ||
) | [inherited] |
Function: move.
Move contents of RX buffer to external buffer. Stops if "end" detected.
- Parameters:
-
char *s The destination buffer address int max The maximum number of chars to move. char end If this char is detected stop moving.
Definition at line 706 of file MODSERIAL.h.
int move | ( | char * | s, |
int | max | ||
) | [inherited] |
Function: move (overloaded)
Move contents of RX buffer to external buffer. Stops if auto_detect_char detected.
- Parameters:
-
int max The maximum number of chars to move. char *s The destination buffer address
Definition at line 728 of file MODSERIAL.h.
int printf | ( | const char * | format, |
... | |||
) | [inherited] |
Function: printf.
Write a formated string Inhereted from Serial/Stream
- Parameters:
-
format A printf-style format string, followed by the variables to use in formating the string.
int putc | ( | int | c ) | [inherited] |
Function: putc.
Write a character Inhereted from Serial/Stream
- Parameters:
-
c The character to write to the serial port
int readable | ( | ) | [inherited] |
Function: readable.
Determine if there is a byte available to read
- Returns:
- 1 if there is a character available to read, else 0
Definition at line 470 of file MODSERIAL.h.
bool rxBufferEmpty | ( | void | ) | [inherited] |
Function: rxBufferEmpty.
Is the RX buffer empty?
- Returns:
- true if the RX buffer is empty, otherwise false
Definition at line 71 of file MODSERIAL.cpp.
void rxBufferFlush | ( | void | ) | [inherited] |
Function: rxBufferFlush.
Remove all bytes from the RX buffer.
Definition at line 638 of file MODSERIAL.h.
bool rxBufferFull | ( | void | ) | [inherited] |
Function: rxBufferFull.
Is the RX buffer full?
- Returns:
- true if the RX buffer is full, otherwise false
Definition at line 61 of file MODSERIAL.cpp.
int rxBufferGetCount | ( | void | ) | [inherited] |
Function: rxBufferGetCount.
Returns how many bytes are in the RX buffer
- Returns:
- The number of bytes in the RX buffer
Definition at line 510 of file MODSERIAL.h.
int rxBufferGetSize | ( | int | size ) | [inherited] |
Function: rxBufferGetSize.
Returns the current size of the RX buffer
- Returns:
- The length iof the RX buffer in bytes
Definition at line 530 of file MODSERIAL.h.
bool rxBufferSane | ( | void | ) | [inherited] |
Function: rxBufferSane.
Determine if the RX buffer has been initialized.
- Returns:
- true if the buffer is initialized, else false
Definition at line 490 of file MODSERIAL.h.
int rxBufferSetSize | ( | int | size, |
bool | m | ||
) | [inherited] |
Function: rxBufferSetSize.
Change the RX buffer size.
- See also:
- Result
- Parameters:
-
size The new RX buffer size in bytes. m Perform a memory sanity check. Errs the Mbed if memory alloc fails.
- Returns:
- Result Ok on success.
Definition at line 596 of file MODSERIAL.h.
int rxBufferSetSize | ( | int | size ) | [inherited] |
Function: rxBufferSetSize.
Change the RX buffer size. Always performs a memory sanity check, halting the Mbed on failure.
- See also:
- Result
- Parameters:
-
size The new RX buffer size in bytes.
- Returns:
- Result Ok on success.
Definition at line 622 of file MODSERIAL.h.
int rxDiscardLastChar | ( | void | ) | [inherited] |
rxDiscardLastChar()
Remove the last char placed into the rx buffer. This is an operation that can only be performed by an rxCallback function.
- Returns:
- The byte removed from the buffer.
Definition at line 31 of file MODSERIAL_IRQ_INFO.cpp.
int scanf | ( | const char * | format, |
... | |||
) | [inherited] |
Function: scanf.
Read a formated string Inhereted from Serial/Stream
- Parameters:
-
format - A scanf-style format string, followed by the pointers to variables to store the results.
bool txBufferEmpty | ( | void | ) | [inherited] |
Function: txBufferEmpty.
Is the TX buffer empty?
- Returns:
- true if the TX buffer is empty, otherwise false
Definition at line 66 of file MODSERIAL.cpp.
void txBufferFlush | ( | void | ) | [inherited] |
Function: txBufferFlush.
Remove all bytes from the TX buffer.
Definition at line 630 of file MODSERIAL.h.
bool txBufferFull | ( | void | ) | [inherited] |
Function: txBufferFull.
Is the TX buffer full?
- Returns:
- true if the TX buffer is full, otherwise false
Definition at line 56 of file MODSERIAL.cpp.
int txBufferGetCount | ( | void | ) | [inherited] |
Function: txBufferGetCount.
Returns how many bytes are in the TX buffer
- Returns:
- The number of bytes in the TX buffer
Definition at line 500 of file MODSERIAL.h.
int txBufferGetSize | ( | int | size ) | [inherited] |
Function: txBufferGetSize.
Returns the current size of the TX buffer
- Returns:
- The length iof the TX buffer in bytes
Definition at line 520 of file MODSERIAL.h.
bool txBufferSane | ( | void | ) | [inherited] |
Function: txBufferSane.
Determine if the TX buffer has been initialized.
- Returns:
- true if the buffer is initialized, else false
Definition at line 480 of file MODSERIAL.h.
int txBufferSetSize | ( | int | size ) | [inherited] |
Function: txBufferSetSize.
Change the TX buffer size. Always performs a memory sanity check, halting the Mbed on failure.
- See also:
- Result
- Parameters:
-
size The new TX buffer size in bytes.
- Returns:
- Result Ok on success.
Definition at line 609 of file MODSERIAL.h.
int txBufferSetSize | ( | int | size, |
bool | m | ||
) | [inherited] |
Function: txBufferSetSize.
Change the TX buffer size.
- See also:
- Result
- Parameters:
-
size The new TX buffer size in bytes. m Perform a memory sanity check. Errs the Mbed if memory alloc fails.
- Returns:
- Result Ok on success.
Definition at line 583 of file MODSERIAL.h.
bool txIsBusy | ( | void | ) | [inherited] |
Function: txIsBusy.
If the Uart is still actively sending characters this function will return true.
- Returns:
- bool
int writeable | ( | ) | [inherited] |
Function: writeable.
Determine if there is space available to write a byte
- Returns:
- 1 if there is space to write a character, else 0
Definition at line 460 of file MODSERIAL.h.
Generated on Wed Jul 13 2022 20:00:12 by
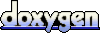