Basic library for interfacing the AK8975 using I2C. It does not include more advanced functions. The datasheet does not include what the self-test should return for example, so this library does not include the self-test function.
AK8975 Class Reference
AK8975 magnetometer/digital compass simple library. More...
#include <AK8975.h>
Public Member Functions | |
AK8975 (PinName sda, PinName scl, char address) | |
Constructor. | |
bool | testConnection (void) |
Checks connection with the device. | |
bool | isReady (void) |
Checks if measurement is ready. | |
int | getX (void) |
Gets the X data. | |
int | getY (void) |
Gets the Y data. | |
int | getZ (void) |
Gets the Z data. | |
void | getAll (int *data) |
Gets all the data, this is more efficient than calling the functions individually. | |
bool | getDataError (void) |
Checks if there is a data error due to reading at wrong moment. | |
bool | getOverflow (void) |
Checks if a magnetic overflow happened (sensor saturation) | |
void | startMeasurement (void) |
Starts a measurement cycle. |
Detailed Description
AK8975 magnetometer/digital compass simple library.
Example:
#include "mbed.h" #include "AK8975.h" DigitalOut led1(LED1); Serial pc(USBTX, USBRX); // tx, rx AK8975 mag(p9,p10,0x0E); int main() { int data[3]; if (mag.testConnection()) pc.printf("Connection succeeded. \n"); else pc.printf("Connection failed. \n"); while(1) { led1=!led1; mag.startMeasurement(); wait(0.5); //(or use the isReady() function) mag.getAll(data); pc.printf("X: %d", data[0]); pc.putc('\n'); pc.printf("Y: %d", data[1]); pc.putc('\n'); pc.printf("Z: %d", data[2]); pc.putc('\n'); } }
Definition at line 72 of file AK8975.h.
Constructor & Destructor Documentation
AK8975 | ( | PinName | sda, |
PinName | scl, | ||
char | address | ||
) |
Constructor.
Includes.
- Parameters:
-
sda - mbed pin to use for the SDA I2C line. scl - mbed pin to use for the SCL I2C line. I2Caddress - the I2C address of the device (0x0C - 0x0F)
Definition at line 6 of file AK8975.cpp.
Member Function Documentation
void getAll | ( | int * | data ) |
Gets all the data, this is more efficient than calling the functions individually.
- Parameters:
-
data - pointer to integer array with length 3 where data is stored (data[0]=X - data[1]=Y - data[2]=Z)
Definition at line 48 of file AK8975.cpp.
bool getDataError | ( | void | ) |
Checks if there is a data error due to reading at wrong moment.
- Returns:
- - true for error, false for no error
Definition at line 56 of file AK8975.cpp.
bool getOverflow | ( | void | ) |
Checks if a magnetic overflow happened (sensor saturation)
- Returns:
- - true for error, false for no error
Definition at line 63 of file AK8975.cpp.
int getX | ( | void | ) |
Gets the X data.
- Returns:
- - signed integer containing the raw data
Definition at line 23 of file AK8975.cpp.
int getY | ( | void | ) |
Gets the Y data.
- Returns:
- - signed integer containing the raw data
Definition at line 31 of file AK8975.cpp.
int getZ | ( | void | ) |
Gets the Z data.
- Returns:
- - signed integer containing the raw data
Definition at line 39 of file AK8975.cpp.
bool isReady | ( | void | ) |
Checks if measurement is ready.
- Returns:
- - true if available measurement, otherwise false
Definition at line 16 of file AK8975.cpp.
void startMeasurement | ( | void | ) |
Starts a measurement cycle.
Definition at line 70 of file AK8975.cpp.
bool testConnection | ( | void | ) |
Checks connection with the device.
- Returns:
- - true if working connection, otherwise false
Definition at line 10 of file AK8975.cpp.
Generated on Wed Jul 13 2022 13:24:50 by
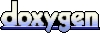