Basic library for interfacing the AK8975 using I2C. It does not include more advanced functions. The datasheet does not include what the self-test should return for example, so this library does not include the self-test function.
AK8975.h
00001 /* Library for AK8975 digital compass IC, for use with I2C 00002 00003 This library contains basic functionality, if you want more, make it ;) 00004 00005 */ 00006 00007 #ifndef AK8975_H 00008 #define AK8975_H 00009 00010 /** 00011 * Includes 00012 */ 00013 #include "mbed.h" 00014 00015 /** 00016 * Registers 00017 */ 00018 #define AK8975_ID_REG 0x00 00019 #define AK8975_ST1_REG 0x02 00020 #define AK8975_X_REG 0x03 00021 #define AK8975_Y_REG 0x05 00022 #define AK8975_Z_REG 0x07 00023 #define AK8975_ST2_REG 0x09 00024 #define AK8975_CONTROL_REG 0x0A 00025 #define AK8975_SELFTEST_REG 0x0C 00026 00027 /** 00028 * Bits 00029 */ 00030 #define AK8975_DRDY_BIT 0 00031 #define AK8975_DERROR_BIT 2 00032 #define AK8975_OFLOW_BIT 3 00033 00034 #define AK8975_SINGLE_MEASUREMENT 1 00035 00036 #define AK8975_SENSITIVITY 0.3 00037 00038 /** AK8975 magnetometer/digital compass simple library. 00039 * 00040 * Example: 00041 * @code 00042 * #include "mbed.h" 00043 * #include "AK8975.h" 00044 * 00045 * DigitalOut led1(LED1); 00046 * Serial pc(USBTX, USBRX); // tx, rx 00047 * AK8975 mag(p9,p10,0x0E); 00048 * 00049 * 00050 * int main() { 00051 * int data[3]; 00052 * if (mag.testConnection()) 00053 * pc.printf("Connection succeeded. \n"); 00054 * else 00055 * pc.printf("Connection failed. \n"); 00056 * 00057 * while(1) { 00058 * led1=!led1; 00059 * mag.startMeasurement(); 00060 * wait(0.5); //(or use the isReady() function) 00061 * mag.getAll(data); 00062 * pc.printf("X: %d", data[0]); 00063 * pc.putc('\n'); 00064 * pc.printf("Y: %d", data[1]); 00065 * pc.putc('\n'); 00066 * pc.printf("Z: %d", data[2]); 00067 * pc.putc('\n'); 00068 * } 00069 * } 00070 * @endcode 00071 */ 00072 class AK8975 { 00073 public: 00074 /** 00075 * Constructor. 00076 * 00077 * @param sda - mbed pin to use for the SDA I2C line. 00078 * @param scl - mbed pin to use for the SCL I2C line. 00079 * @param I2Caddress - the I2C address of the device (0x0C - 0x0F) 00080 */ 00081 AK8975(PinName sda, PinName scl, char address); 00082 00083 /** 00084 * Checks connection with the device. 00085 * 00086 * @return - true if working connection, otherwise false 00087 */ 00088 bool testConnection( void ); 00089 00090 /** 00091 * Checks if measurement is ready 00092 * 00093 * @return - true if available measurement, otherwise false 00094 */ 00095 bool isReady( void ); 00096 00097 /** 00098 * Gets the X data 00099 * 00100 * @return - signed integer containing the raw data 00101 */ 00102 int getX( void ); 00103 00104 /** 00105 * Gets the Y data 00106 * 00107 * @return - signed integer containing the raw data 00108 */ 00109 int getY( void ); 00110 00111 /** 00112 * Gets the Z data 00113 * 00114 * @return - signed integer containing the raw data 00115 */ 00116 int getZ( void ); 00117 00118 /** 00119 * Gets all the data, this is more efficient than calling the functions individually 00120 * 00121 * @param data - pointer to integer array with length 3 where data is stored (data[0]=X - data[1]=Y - data[2]=Z) 00122 */ 00123 void getAll( int *data ); 00124 00125 00126 00127 /** 00128 * Checks if there is a data error due to reading at wrong moment 00129 * 00130 * @return - true for error, false for no error 00131 */ 00132 bool getDataError( void ); 00133 00134 /** 00135 * Checks if a magnetic overflow happened (sensor saturation) 00136 * 00137 * @return - true for error, false for no error 00138 */ 00139 bool getOverflow( void ); 00140 00141 /** 00142 * Starts a measurement cycle 00143 */ 00144 void startMeasurement( void ); 00145 00146 00147 00148 private: 00149 I2C connection; 00150 char deviceAddress; 00151 00152 /** 00153 * Writes data to the device 00154 * 00155 * @param adress - register address to write to 00156 * @param data - data to write 00157 */ 00158 void write( char address, char data); 00159 00160 /** 00161 * Read data from the device 00162 * 00163 * @param adress - register address to write to 00164 * @return - data from the register specified by RA 00165 */ 00166 char read( char adress); 00167 00168 /** 00169 * Read multtiple regigsters from the device, more efficient than using multiple normal reads. 00170 * 00171 * @param adress - register address to write to 00172 * @param length - number of bytes to read 00173 * @param data - pointer where the data needs to be written to 00174 */ 00175 void read( char adress, char *data, int length); 00176 }; 00177 #endif
Generated on Wed Jul 13 2022 13:24:50 by
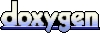