ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
zbar.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _ZBAR_H_ 00024 #define _ZBAR_H_ 00025 00026 /** @file 00027 * ZBar Barcode Reader C API definition 00028 */ 00029 00030 /** @mainpage 00031 * 00032 * interface to the barcode reader is available at several levels. 00033 * most applications will want to use the high-level interfaces: 00034 * 00035 * @section high-level High-Level Interfaces 00036 * 00037 * these interfaces wrap all library functionality into an easy-to-use 00038 * package for a specific toolkit: 00039 * - the "GTK+ 2.x widget" may be used with GTK GUI applications. a 00040 * Python wrapper is included for PyGtk 00041 * - the @ref zbar::QZBar "Qt4 widget" may be used with Qt GUI 00042 * applications 00043 * - the Processor interface (in @ref c-processor "C" or @ref 00044 * zbar::Processor "C++") adds a scanning window to an application 00045 * with no GUI. 00046 * 00047 * @section mid-level Intermediate Interfaces 00048 * 00049 * building blocks used to construct high-level interfaces: 00050 * - the ImageScanner (in @ref c-imagescanner "C" or @ref 00051 * zbar::ImageScanner "C++") looks for barcodes in a library defined 00052 * image object 00053 * - the Window abstraction (in @ref c-window "C" or @ref 00054 * zbar::Window "C++") sinks library images, displaying them on the 00055 * platform display 00056 * - the Video abstraction (in @ref c-video "C" or @ref zbar::Video 00057 * "C++") sources library images from a video device 00058 * 00059 * @section low-level Low-Level Interfaces 00060 * 00061 * direct interaction with barcode scanning and decoding: 00062 * - the Scanner (in @ref c-scanner "C" or @ref zbar::Scanner "C++") 00063 * looks for barcodes in a linear intensity sample stream 00064 * - the Decoder (in @ref c-decoder "C" or @ref zbar::Decoder "C++") 00065 * extracts barcodes from a stream of bar and space widths 00066 */ 00067 00068 #ifdef __cplusplus 00069 00070 /** C++ namespace for library interfaces */ 00071 namespace zbar { 00072 extern "C" { 00073 #endif 00074 00075 00076 /** @name Global library interfaces */ 00077 /*@{*/ 00078 00079 /** "color" of element: bar or space. */ 00080 typedef enum zbar_color_e { 00081 ZBAR_SPACE = 0, /**< light area or space between bars */ 00082 ZBAR_BAR = 1, /**< dark area or colored bar segment */ 00083 } zbar_color_t; 00084 00085 /** decoded symbol type. */ 00086 typedef enum zbar_symbol_type_e { 00087 ZBAR_NONE = 0, /**< no symbol decoded */ 00088 ZBAR_PARTIAL = 1, /**< intermediate status */ 00089 ZBAR_EAN8 = 8, /**< EAN-8 */ 00090 ZBAR_UPCE = 9, /**< UPC-E */ 00091 ZBAR_ISBN10 = 10, /**< ISBN-10 (from EAN-13). @since 0.4 */ 00092 ZBAR_UPCA = 12, /**< UPC-A */ 00093 ZBAR_EAN13 = 13, /**< EAN-13 */ 00094 ZBAR_ISBN13 = 14, /**< ISBN-13 (from EAN-13). @since 0.4 */ 00095 ZBAR_I25 = 25, /**< Interleaved 2 of 5. @since 0.4 */ 00096 ZBAR_CODE39 = 39, /**< Code 39. @since 0.4 */ 00097 ZBAR_PDF417 = 57, /**< PDF417. @since 0.6 */ 00098 ZBAR_QRCODE = 64, /**< QR Code. @since 0.10 */ 00099 ZBAR_CODE128 = 128, /**< Code 128 */ 00100 ZBAR_SYMBOL = 0x00ff, /**< mask for base symbol type */ 00101 ZBAR_ADDON2 = 0x0200, /**< 2-digit add-on flag */ 00102 ZBAR_ADDON5 = 0x0500, /**< 5-digit add-on flag */ 00103 ZBAR_ADDON = 0x0700, /**< add-on flag mask */ 00104 } zbar_symbol_type_t; 00105 00106 /** error codes. */ 00107 typedef enum zbar_error_e { 00108 ZBAR_OK = 0, /**< no error */ 00109 ZBAR_ERR_NOMEM, /**< out of memory */ 00110 ZBAR_ERR_INTERNAL, /**< internal library error */ 00111 ZBAR_ERR_UNSUPPORTED, /**< unsupported request */ 00112 ZBAR_ERR_INVALID, /**< invalid request */ 00113 ZBAR_ERR_SYSTEM, /**< system error */ 00114 ZBAR_ERR_LOCKING, /**< locking error */ 00115 ZBAR_ERR_BUSY, /**< all resources busy */ 00116 ZBAR_ERR_XDISPLAY, /**< X11 display error */ 00117 ZBAR_ERR_XPROTO, /**< X11 protocol error */ 00118 ZBAR_ERR_CLOSED, /**< output window is closed */ 00119 ZBAR_ERR_WINAPI, /**< windows system error */ 00120 ZBAR_ERR_NUM /**< number of error codes */ 00121 } zbar_error_t; 00122 00123 /** decoder configuration options. 00124 * @since 0.4 00125 */ 00126 typedef enum zbar_config_e { 00127 ZBAR_CFG_ENABLE = 0, /**< enable symbology/feature */ 00128 ZBAR_CFG_ADD_CHECK, /**< enable check digit when optional */ 00129 ZBAR_CFG_EMIT_CHECK, /**< return check digit when present */ 00130 ZBAR_CFG_ASCII, /**< enable full ASCII character set */ 00131 ZBAR_CFG_NUM, /**< number of boolean decoder configs */ 00132 00133 ZBAR_CFG_MIN_LEN = 0x20, /**< minimum data length for valid decode */ 00134 ZBAR_CFG_MAX_LEN, /**< maximum data length for valid decode */ 00135 00136 ZBAR_CFG_POSITION = 0x80, /**< enable scanner to collect position data */ 00137 00138 ZBAR_CFG_X_DENSITY = 0x100, /**< image scanner vertical scan density */ 00139 ZBAR_CFG_Y_DENSITY, /**< image scanner horizontal scan density */ 00140 } zbar_config_t; 00141 00142 /** retrieve runtime library version information. 00143 * @param major set to the running major version (unless NULL) 00144 * @param minor set to the running minor version (unless NULL) 00145 * @returns 0 00146 */ 00147 extern int zbar_version(unsigned *major, 00148 unsigned *minor); 00149 00150 /** set global library debug level. 00151 * @param verbosity desired debug level. higher values create more spew 00152 */ 00153 extern void zbar_set_verbosity(int verbosity); 00154 00155 /** increase global library debug level. 00156 * eg, for -vvvv 00157 */ 00158 extern void zbar_increase_verbosity(void); 00159 00160 /** retrieve string name for symbol encoding. 00161 * @param sym symbol type encoding 00162 * @returns the static string name for the specified symbol type, 00163 * or "UNKNOWN" if the encoding is not recognized 00164 */ 00165 extern const char *zbar_get_symbol_name(zbar_symbol_type_t sym); 00166 00167 /** retrieve string name for addon encoding. 00168 * @param sym symbol type encoding 00169 * @returns static string name for any addon, or the empty string 00170 * if no addons were decoded 00171 */ 00172 extern const char *zbar_get_addon_name(zbar_symbol_type_t sym); 00173 00174 /** parse a configuration string of the form "[symbology.]config[=value]". 00175 * the config must match one of the recognized names. 00176 * the symbology, if present, must match one of the recognized names. 00177 * if symbology is unspecified, it will be set to 0. 00178 * if value is unspecified it will be set to 1. 00179 * @returns 0 if the config is parsed successfully, 1 otherwise 00180 * @since 0.4 00181 */ 00182 extern int zbar_parse_config(const char *config_string, 00183 zbar_symbol_type_t *symbology, 00184 zbar_config_t *config, 00185 int *value); 00186 00187 /** @internal type unsafe error API (don't use) */ 00188 extern int _zbar_error_spew(const void *object, 00189 int verbosity); 00190 extern const char *_zbar_error_string(const void *object, 00191 int verbosity); 00192 extern zbar_error_t _zbar_get_error_code(const void *object); 00193 00194 /*@}*/ 00195 00196 struct zbar_symbol_s; 00197 typedef struct zbar_symbol_s zbar_symbol_t; 00198 00199 struct zbar_symbol_set_s; 00200 typedef struct zbar_symbol_set_s zbar_symbol_set_t; 00201 00202 00203 /*------------------------------------------------------------*/ 00204 /** @name Symbol interface 00205 * decoded barcode symbol result object. stores type, data, and image 00206 * location of decoded symbol. all memory is owned by the library 00207 */ 00208 /*@{*/ 00209 00210 /** @typedef zbar_symbol_t 00211 * opaque decoded symbol object. 00212 */ 00213 00214 /** symbol reference count manipulation. 00215 * increment the reference count when you store a new reference to the 00216 * symbol. decrement when the reference is no longer used. do not 00217 * refer to the symbol once the count is decremented and the 00218 * containing image has been recycled or destroyed. 00219 * @note the containing image holds a reference to the symbol, so you 00220 * only need to use this if you keep a symbol after the image has been 00221 * destroyed or reused. 00222 * @since 0.9 00223 */ 00224 extern void zbar_symbol_ref(const zbar_symbol_t *symbol, 00225 int refs); 00226 00227 /** retrieve type of decoded symbol. 00228 * @returns the symbol type 00229 */ 00230 extern zbar_symbol_type_t zbar_symbol_get_type(const zbar_symbol_t *symbol); 00231 00232 /** retrieve data decoded from symbol. 00233 * @returns the data string 00234 */ 00235 extern const char *zbar_symbol_get_data(const zbar_symbol_t *symbol); 00236 00237 /** retrieve length of binary data. 00238 * @returns the length of the decoded data 00239 */ 00240 extern unsigned int zbar_symbol_get_data_length(const zbar_symbol_t *symbol); 00241 00242 /** retrieve a symbol confidence metric. 00243 * @returns an unscaled, relative quantity: larger values are better 00244 * than smaller values, where "large" and "small" are application 00245 * dependent. 00246 * @note expect the exact definition of this quantity to change as the 00247 * metric is refined. currently, only the ordered relationship 00248 * between two values is defined and will remain stable in the future 00249 * @since 0.9 00250 */ 00251 extern int zbar_symbol_get_quality(const zbar_symbol_t *symbol); 00252 00253 /** retrieve current cache count. when the cache is enabled for the 00254 * image_scanner this provides inter-frame reliability and redundancy 00255 * information for video streams. 00256 * @returns < 0 if symbol is still uncertain. 00257 * @returns 0 if symbol is newly verified. 00258 * @returns > 0 for duplicate symbols 00259 */ 00260 extern int zbar_symbol_get_count(const zbar_symbol_t *symbol); 00261 00262 /** retrieve the number of points in the location polygon. the 00263 * location polygon defines the image area that the symbol was 00264 * extracted from. 00265 * @returns the number of points in the location polygon 00266 * @note this is currently not a polygon, but the scan locations 00267 * where the symbol was decoded 00268 */ 00269 extern unsigned zbar_symbol_get_loc_size(const zbar_symbol_t *symbol); 00270 00271 /** retrieve location polygon x-coordinates. 00272 * points are specified by 0-based index. 00273 * @returns the x-coordinate for a point in the location polygon. 00274 * @returns -1 if index is out of range 00275 */ 00276 extern int zbar_symbol_get_loc_x(const zbar_symbol_t *symbol, 00277 unsigned index); 00278 00279 /** retrieve location polygon y-coordinates. 00280 * points are specified by 0-based index. 00281 * @returns the y-coordinate for a point in the location polygon. 00282 * @returns -1 if index is out of range 00283 */ 00284 extern int zbar_symbol_get_loc_y(const zbar_symbol_t *symbol, 00285 unsigned index); 00286 00287 /** iterate the set to which this symbol belongs (there can be only one). 00288 * @returns the next symbol in the set, or 00289 * @returns NULL when no more results are available 00290 */ 00291 extern const zbar_symbol_t *zbar_symbol_next(const zbar_symbol_t *symbol); 00292 00293 /** retrieve components of a composite result. 00294 * @returns the symbol set containing the components 00295 * @returns NULL if the symbol is already a physical symbol 00296 * @since 0.10 00297 */ 00298 extern const zbar_symbol_set_t* 00299 zbar_symbol_get_components(const zbar_symbol_t *symbol); 00300 00301 /** iterate components of a composite result. 00302 * @returns the first physical component symbol of a composite result 00303 * @returns NULL if the symbol is already a physical symbol 00304 * @since 0.10 00305 */ 00306 extern const zbar_symbol_t* 00307 zbar_symbol_first_component(const zbar_symbol_t *symbol); 00308 00309 /** print XML symbol element representation to user result buffer. 00310 * @see http://zbar.sourceforge.net/2008/barcode.xsd for the schema. 00311 * @param symbol is the symbol to print 00312 * @param buffer is the inout result pointer, it will be reallocated 00313 * with a larger size if necessary. 00314 * @param buflen is inout length of the result buffer. 00315 * @returns the buffer pointer 00316 * @since 0.6 00317 */ 00318 extern char *zbar_symbol_xml(const zbar_symbol_t *symbol, 00319 char **buffer, 00320 unsigned *buflen); 00321 00322 /*@}*/ 00323 00324 /*------------------------------------------------------------*/ 00325 /** @name Symbol Set interface 00326 * container for decoded result symbols associated with an image 00327 * or a composite symbol. 00328 * @since 0.10 00329 */ 00330 /*@{*/ 00331 00332 /** @typedef zbar_symbol_set_t 00333 * opaque symbol iterator object. 00334 * @since 0.10 00335 */ 00336 00337 /** reference count manipulation. 00338 * increment the reference count when you store a new reference. 00339 * decrement when the reference is no longer used. do not refer to 00340 * the object any longer once references have been released. 00341 * @since 0.10 00342 */ 00343 extern void zbar_symbol_set_ref(const zbar_symbol_set_t *symbols, 00344 int refs); 00345 00346 /** retrieve set size. 00347 * @returns the number of symbols in the set. 00348 * @since 0.10 00349 */ 00350 extern int zbar_symbol_set_get_size(const zbar_symbol_set_t *symbols); 00351 00352 /** set iterator. 00353 * @returns the first decoded symbol result in a set 00354 * @returns NULL if the set is empty 00355 * @since 0.10 00356 */ 00357 extern const zbar_symbol_t* 00358 zbar_symbol_set_first_symbol(const zbar_symbol_set_t *symbols); 00359 00360 /*@}*/ 00361 00362 /*------------------------------------------------------------*/ 00363 /** @name Image interface 00364 * stores image data samples along with associated format and size 00365 * metadata 00366 */ 00367 /*@{*/ 00368 00369 struct zbar_image_s; 00370 /** opaque image object. */ 00371 typedef struct zbar_image_s zbar_image_t; 00372 00373 /** cleanup handler callback function. 00374 * called to free sample data when an image is destroyed. 00375 */ 00376 typedef void (zbar_image_cleanup_handler_t)(zbar_image_t *image); 00377 00378 /** data handler callback function. 00379 * called when decoded symbol results are available for an image 00380 */ 00381 typedef void (zbar_image_data_handler_t)(zbar_image_t *image, 00382 const void *userdata); 00383 00384 /** new image constructor. 00385 * @returns a new image object with uninitialized data and format. 00386 * this image should be destroyed (using zbar_image_destroy()) as 00387 * soon as the application is finished with it 00388 */ 00389 extern zbar_image_t *zbar_image_create(void); 00390 00391 /** image destructor. all images created by or returned to the 00392 * application should be destroyed using this function. when an image 00393 * is destroyed, the associated data cleanup handler will be invoked 00394 * if available 00395 * @note make no assumptions about the image or the data buffer. 00396 * they may not be destroyed/cleaned immediately if the library 00397 * is still using them. if necessary, use the cleanup handler hook 00398 * to keep track of image data buffers 00399 */ 00400 extern void zbar_image_destroy(zbar_image_t *image); 00401 00402 /** image reference count manipulation. 00403 * increment the reference count when you store a new reference to the 00404 * image. decrement when the reference is no longer used. do not 00405 * refer to the image any longer once the count is decremented. 00406 * zbar_image_ref(image, -1) is the same as zbar_image_destroy(image) 00407 * @since 0.5 00408 */ 00409 extern void zbar_image_ref(zbar_image_t *image, 00410 int refs); 00411 00412 /** image format conversion. refer to the documentation for supported 00413 * image formats 00414 * @returns a @em new image with the sample data from the original image 00415 * converted to the requested format. the original image is 00416 * unaffected. 00417 * @note the converted image size may be rounded (up) due to format 00418 * constraints 00419 */ 00420 extern zbar_image_t *zbar_image_convert(const zbar_image_t *image, 00421 unsigned long format); 00422 00423 /** image format conversion with crop/pad. 00424 * if the requested size is larger than the image, the last row/column 00425 * are duplicated to cover the difference. if the requested size is 00426 * smaller than the image, the extra rows/columns are dropped from the 00427 * right/bottom. 00428 * @returns a @em new image with the sample data from the original 00429 * image converted to the requested format and size. 00430 * @note the image is @em not scaled 00431 * @see zbar_image_convert() 00432 * @since 0.4 00433 */ 00434 extern zbar_image_t *zbar_image_convert_resize(const zbar_image_t *image, 00435 unsigned long format, 00436 unsigned width, 00437 unsigned height); 00438 00439 /** retrieve the image format. 00440 * @returns the fourcc describing the format of the image sample data 00441 */ 00442 extern unsigned long zbar_image_get_format(const zbar_image_t *image); 00443 00444 /** retrieve a "sequence" (page/frame) number associated with this image. 00445 * @since 0.6 00446 */ 00447 extern unsigned zbar_image_get_sequence(const zbar_image_t *image); 00448 00449 /** retrieve the width of the image. 00450 * @returns the width in sample columns 00451 */ 00452 extern unsigned zbar_image_get_width(const zbar_image_t *image); 00453 00454 /** retrieve the height of the image. 00455 * @returns the height in sample rows 00456 */ 00457 extern unsigned zbar_image_get_height(const zbar_image_t *image); 00458 00459 /** return the image sample data. the returned data buffer is only 00460 * valid until zbar_image_destroy() is called 00461 */ 00462 extern const void *zbar_image_get_data(const zbar_image_t *image); 00463 00464 /** return the size of image data. 00465 * @since 0.6 00466 */ 00467 extern unsigned long zbar_image_get_data_length(const zbar_image_t *img); 00468 00469 /** retrieve the decoded results. 00470 * @returns the (possibly empty) set of decoded symbols 00471 * @returns NULL if the image has not been scanned 00472 * @since 0.10 00473 */ 00474 extern const zbar_symbol_set_t* 00475 zbar_image_get_symbols(const zbar_image_t *image); 00476 00477 /** associate the specified symbol set with the image, replacing any 00478 * existing results. use NULL to release the current results from the 00479 * image. 00480 * @see zbar_image_scanner_recycle_image() 00481 * @since 0.10 00482 */ 00483 extern void zbar_image_set_symbols(zbar_image_t *image, 00484 const zbar_symbol_set_t *symbols); 00485 00486 /** image_scanner decode result iterator. 00487 * @returns the first decoded symbol result for an image 00488 * or NULL if no results are available 00489 */ 00490 extern const zbar_symbol_t* 00491 zbar_image_first_symbol(const zbar_image_t *image); 00492 00493 /** specify the fourcc image format code for image sample data. 00494 * refer to the documentation for supported formats. 00495 * @note this does not convert the data! 00496 * (see zbar_image_convert() for that) 00497 */ 00498 extern void zbar_image_set_format(zbar_image_t *image, 00499 unsigned long format); 00500 00501 /** associate a "sequence" (page/frame) number with this image. 00502 * @since 0.6 00503 */ 00504 extern void zbar_image_set_sequence(zbar_image_t *image, 00505 unsigned sequence_num); 00506 00507 /** specify the pixel size of the image. 00508 * @note this does not affect the data! 00509 */ 00510 extern void zbar_image_set_size(zbar_image_t *image, 00511 unsigned width, 00512 unsigned height); 00513 00514 /** specify image sample data. when image data is no longer needed by 00515 * the library the specific data cleanup handler will be called 00516 * (unless NULL) 00517 * @note application image data will not be modified by the library 00518 */ 00519 extern void zbar_image_set_data(zbar_image_t *image, 00520 const void *data, 00521 unsigned long data_byte_length, 00522 zbar_image_cleanup_handler_t *cleanup_hndlr); 00523 00524 /** built-in cleanup handler. 00525 * passes the image data buffer to free() 00526 */ 00527 extern void zbar_image_free_data(zbar_image_t *image); 00528 00529 /** associate user specified data value with an image. 00530 * @since 0.5 00531 */ 00532 extern void zbar_image_set_userdata(zbar_image_t *image, 00533 void *userdata); 00534 00535 /** return user specified data value associated with the image. 00536 * @since 0.5 00537 */ 00538 extern void *zbar_image_get_userdata(const zbar_image_t *image); 00539 00540 /** dump raw image data to a file for debug. 00541 * the data will be prefixed with a 16 byte header consisting of: 00542 * - 4 bytes uint = 0x676d697a ("zimg") 00543 * - 4 bytes format fourcc 00544 * - 2 bytes width 00545 * - 2 bytes height 00546 * - 4 bytes size of following image data in bytes 00547 * this header can be dumped w/eg: 00548 * @verbatim 00549 od -Ax -tx1z -N16 -w4 [file] 00550 @endverbatim 00551 * for some formats the image can be displayed/converted using 00552 * ImageMagick, eg: 00553 * @verbatim 00554 display -size 640x480+16 [-depth ?] [-sampling-factor ?x?] \ 00555 {GRAY,RGB,UYVY,YUV}:[file] 00556 @endverbatim 00557 * 00558 * @param image the image object to dump 00559 * @param filebase base filename, appended with ".XXXX.zimg" where 00560 * XXXX is the format fourcc 00561 * @returns 0 on success or a system error code on failure 00562 */ 00563 extern int zbar_image_write(const zbar_image_t *image, 00564 const char *filebase); 00565 00566 /** read back an image in the format written by zbar_image_write() 00567 * @note TBD 00568 */ 00569 extern zbar_image_t *zbar_image_read(char *filename); 00570 00571 /*@}*/ 00572 00573 /*------------------------------------------------------------*/ 00574 /** @name Processor interface 00575 * @anchor c-processor 00576 * high-level self-contained image processor. 00577 * processes video and images for barcodes, optionally displaying 00578 * images to a library owned output window 00579 */ 00580 /*@{*/ 00581 00582 struct zbar_processor_s; 00583 /** opaque standalone processor object. */ 00584 typedef struct zbar_processor_s zbar_processor_t; 00585 00586 /** constructor. 00587 * if threaded is set and threading is available the processor 00588 * will spawn threads where appropriate to avoid blocking and 00589 * improve responsiveness 00590 */ 00591 extern zbar_processor_t *zbar_processor_create(int threaded); 00592 00593 /** destructor. cleans up all resources associated with the processor 00594 */ 00595 extern void zbar_processor_destroy(zbar_processor_t *processor); 00596 00597 /** (re)initialization. 00598 * opens a video input device and/or prepares to display output 00599 */ 00600 extern int zbar_processor_init(zbar_processor_t *processor, 00601 const char *video_device, 00602 int enable_display); 00603 00604 /** request a preferred size for the video image from the device. 00605 * the request may be adjusted or completely ignored by the driver. 00606 * @note must be called before zbar_processor_init() 00607 * @since 0.6 00608 */ 00609 extern int zbar_processor_request_size(zbar_processor_t *processor, 00610 unsigned width, 00611 unsigned height); 00612 00613 /** request a preferred video driver interface version for 00614 * debug/testing. 00615 * @note must be called before zbar_processor_init() 00616 * @since 0.6 00617 */ 00618 extern int zbar_processor_request_interface(zbar_processor_t *processor, 00619 int version); 00620 00621 /** request a preferred video I/O mode for debug/testing. You will 00622 * get errors if the driver does not support the specified mode. 00623 * @verbatim 00624 0 = auto-detect 00625 1 = force I/O using read() 00626 2 = force memory mapped I/O using mmap() 00627 3 = force USERPTR I/O (v4l2 only) 00628 @endverbatim 00629 * @note must be called before zbar_processor_init() 00630 * @since 0.7 00631 */ 00632 extern int zbar_processor_request_iomode(zbar_processor_t *video, 00633 int iomode); 00634 00635 /** force specific input and output formats for debug/testing. 00636 * @note must be called before zbar_processor_init() 00637 */ 00638 extern int zbar_processor_force_format(zbar_processor_t *processor, 00639 unsigned long input_format, 00640 unsigned long output_format); 00641 00642 /** setup result handler callback. 00643 * the specified function will be called by the processor whenever 00644 * new results are available from the video stream or a static image. 00645 * pass a NULL value to disable callbacks. 00646 * @param processor the object on which to set the handler. 00647 * @param handler the function to call when new results are available. 00648 * @param userdata is set as with zbar_processor_set_userdata(). 00649 * @returns the previously registered handler 00650 */ 00651 extern zbar_image_data_handler_t* 00652 zbar_processor_set_data_handler(zbar_processor_t *processor, 00653 zbar_image_data_handler_t *handler, 00654 const void *userdata); 00655 00656 /** associate user specified data value with the processor. 00657 * @since 0.6 00658 */ 00659 extern void zbar_processor_set_userdata(zbar_processor_t *processor, 00660 void *userdata); 00661 00662 /** return user specified data value associated with the processor. 00663 * @since 0.6 00664 */ 00665 extern void *zbar_processor_get_userdata(const zbar_processor_t *processor); 00666 00667 /** set config for indicated symbology (0 for all) to specified value. 00668 * @returns 0 for success, non-0 for failure (config does not apply to 00669 * specified symbology, or value out of range) 00670 * @see zbar_decoder_set_config() 00671 * @since 0.4 00672 */ 00673 extern int zbar_processor_set_config(zbar_processor_t *processor, 00674 zbar_symbol_type_t symbology, 00675 zbar_config_t config, 00676 int value); 00677 00678 /** parse configuration string using zbar_parse_config() 00679 * and apply to processor using zbar_processor_set_config(). 00680 * @returns 0 for success, non-0 for failure 00681 * @see zbar_parse_config() 00682 * @see zbar_processor_set_config() 00683 * @since 0.4 00684 */ 00685 static inline int zbar_processor_parse_config (zbar_processor_t *processor, 00686 const char *config_string) 00687 { 00688 zbar_symbol_type_t sym; 00689 zbar_config_t cfg; 00690 int val; 00691 return(zbar_parse_config(config_string, &sym, &cfg, &val) || 00692 zbar_processor_set_config(processor, sym, cfg, val)); 00693 } 00694 00695 /** retrieve the current state of the ouput window. 00696 * @returns 1 if the output window is currently displayed, 0 if not. 00697 * @returns -1 if an error occurs 00698 */ 00699 extern int zbar_processor_is_visible(zbar_processor_t *processor); 00700 00701 /** show or hide the display window owned by the library. 00702 * the size will be adjusted to the input size 00703 */ 00704 extern int zbar_processor_set_visible(zbar_processor_t *processor, 00705 int visible); 00706 00707 /** control the processor in free running video mode. 00708 * only works if video input is initialized. if threading is in use, 00709 * scanning will occur in the background, otherwise this is only 00710 * useful wrapping calls to zbar_processor_user_wait(). if the 00711 * library output window is visible, video display will be enabled. 00712 */ 00713 extern int zbar_processor_set_active(zbar_processor_t *processor, 00714 int active); 00715 00716 /** retrieve decode results for last scanned image/frame. 00717 * @returns the symbol set result container or NULL if no results are 00718 * available 00719 * @note the returned symbol set has its reference count incremented; 00720 * ensure that the count is decremented after use 00721 * @since 0.10 00722 */ 00723 extern const zbar_symbol_set_t* 00724 zbar_processor_get_results(const zbar_processor_t *processor); 00725 00726 /** wait for input to the display window from the user 00727 * (via mouse or keyboard). 00728 * @returns >0 when input is received, 0 if timeout ms expired 00729 * with no input or -1 in case of an error 00730 */ 00731 extern int zbar_processor_user_wait(zbar_processor_t *processor, 00732 int timeout); 00733 00734 /** process from the video stream until a result is available, 00735 * or the timeout (in milliseconds) expires. 00736 * specify a timeout of -1 to scan indefinitely 00737 * (zbar_processor_set_active() may still be used to abort the scan 00738 * from another thread). 00739 * if the library window is visible, video display will be enabled. 00740 * @note that multiple results may still be returned (despite the 00741 * name). 00742 * @returns >0 if symbols were successfully decoded, 00743 * 0 if no symbols were found (ie, the timeout expired) 00744 * or -1 if an error occurs 00745 */ 00746 extern int zbar_process_one(zbar_processor_t *processor, 00747 int timeout); 00748 00749 /** process the provided image for barcodes. 00750 * if the library window is visible, the image will be displayed. 00751 * @returns >0 if symbols were successfully decoded, 00752 * 0 if no symbols were found or -1 if an error occurs 00753 */ 00754 extern int zbar_process_image(zbar_processor_t *processor, 00755 zbar_image_t *image); 00756 00757 /** display detail for last processor error to stderr. 00758 * @returns a non-zero value suitable for passing to exit() 00759 */ 00760 static inline int 00761 zbar_processor_error_spew (const zbar_processor_t *processor, 00762 int verbosity) 00763 { 00764 return(_zbar_error_spew(processor, verbosity)); 00765 } 00766 00767 /** retrieve the detail string for the last processor error. */ 00768 static inline const char* 00769 zbar_processor_error_string (const zbar_processor_t *processor, 00770 int verbosity) 00771 { 00772 return(_zbar_error_string(processor, verbosity)); 00773 } 00774 00775 /** retrieve the type code for the last processor error. */ 00776 static inline zbar_error_t 00777 zbar_processor_get_error_code (const zbar_processor_t *processor) 00778 { 00779 return(_zbar_get_error_code(processor)); 00780 } 00781 00782 /*@}*/ 00783 00784 /*------------------------------------------------------------*/ 00785 /** @name Video interface 00786 * @anchor c-video 00787 * mid-level video source abstraction. 00788 * captures images from a video device 00789 */ 00790 /*@{*/ 00791 00792 struct zbar_video_s; 00793 /** opaque video object. */ 00794 typedef struct zbar_video_s zbar_video_t; 00795 00796 /** constructor. */ 00797 extern zbar_video_t *zbar_video_create(void); 00798 00799 /** destructor. */ 00800 extern void zbar_video_destroy(zbar_video_t *video); 00801 00802 /** open and probe a video device. 00803 * the device specified by platform specific unique name 00804 * (v4l device node path in *nix eg "/dev/video", 00805 * DirectShow DevicePath property in windows). 00806 * @returns 0 if successful or -1 if an error occurs 00807 */ 00808 extern int zbar_video_open(zbar_video_t *video, 00809 const char *device); 00810 00811 /** retrieve file descriptor associated with open *nix video device 00812 * useful for using select()/poll() to tell when new images are 00813 * available (NB v4l2 only!!). 00814 * @returns the file descriptor or -1 if the video device is not open 00815 * or the driver only supports v4l1 00816 */ 00817 extern int zbar_video_get_fd(const zbar_video_t *video); 00818 00819 /** request a preferred size for the video image from the device. 00820 * the request may be adjusted or completely ignored by the driver. 00821 * @returns 0 if successful or -1 if the video device is already 00822 * initialized 00823 * @since 0.6 00824 */ 00825 extern int zbar_video_request_size(zbar_video_t *video, 00826 unsigned width, 00827 unsigned height); 00828 00829 /** request a preferred driver interface version for debug/testing. 00830 * @note must be called before zbar_video_open() 00831 * @since 0.6 00832 */ 00833 extern int zbar_video_request_interface(zbar_video_t *video, 00834 int version); 00835 00836 /** request a preferred I/O mode for debug/testing. You will get 00837 * errors if the driver does not support the specified mode. 00838 * @verbatim 00839 0 = auto-detect 00840 1 = force I/O using read() 00841 2 = force memory mapped I/O using mmap() 00842 3 = force USERPTR I/O (v4l2 only) 00843 @endverbatim 00844 * @note must be called before zbar_video_open() 00845 * @since 0.7 00846 */ 00847 extern int zbar_video_request_iomode(zbar_video_t *video, 00848 int iomode); 00849 00850 /** retrieve current output image width. 00851 * @returns the width or 0 if the video device is not open 00852 */ 00853 extern int zbar_video_get_width(const zbar_video_t *video); 00854 00855 /** retrieve current output image height. 00856 * @returns the height or 0 if the video device is not open 00857 */ 00858 extern int zbar_video_get_height(const zbar_video_t *video); 00859 00860 /** initialize video using a specific format for debug. 00861 * use zbar_negotiate_format() to automatically select and initialize 00862 * the best available format 00863 */ 00864 extern int zbar_video_init(zbar_video_t *video, 00865 unsigned long format); 00866 00867 /** start/stop video capture. 00868 * all buffered images are retired when capture is disabled. 00869 * @returns 0 if successful or -1 if an error occurs 00870 */ 00871 extern int zbar_video_enable(zbar_video_t *video, 00872 int enable); 00873 00874 /** retrieve next captured image. blocks until an image is available. 00875 * @returns NULL if video is not enabled or an error occurs 00876 */ 00877 extern zbar_image_t *zbar_video_next_image(zbar_video_t *video); 00878 00879 /** display detail for last video error to stderr. 00880 * @returns a non-zero value suitable for passing to exit() 00881 */ 00882 static inline int zbar_video_error_spew (const zbar_video_t *video, 00883 int verbosity) 00884 { 00885 return(_zbar_error_spew(video, verbosity)); 00886 } 00887 00888 /** retrieve the detail string for the last video error. */ 00889 static inline const char *zbar_video_error_string (const zbar_video_t *video, 00890 int verbosity) 00891 { 00892 return(_zbar_error_string(video, verbosity)); 00893 } 00894 00895 /** retrieve the type code for the last video error. */ 00896 static inline zbar_error_t 00897 zbar_video_get_error_code (const zbar_video_t *video) 00898 { 00899 return(_zbar_get_error_code(video)); 00900 } 00901 00902 /*@}*/ 00903 00904 /*------------------------------------------------------------*/ 00905 /** @name Window interface 00906 * @anchor c-window 00907 * mid-level output window abstraction. 00908 * displays images to user-specified platform specific output window 00909 */ 00910 /*@{*/ 00911 00912 struct zbar_window_s; 00913 /** opaque window object. */ 00914 typedef struct zbar_window_s zbar_window_t; 00915 00916 /** constructor. */ 00917 extern zbar_window_t *zbar_window_create(void); 00918 00919 /** destructor. */ 00920 extern void zbar_window_destroy(zbar_window_t *window); 00921 00922 /** associate reader with an existing platform window. 00923 * This can be any "Drawable" for X Windows or a "HWND" for windows. 00924 * input images will be scaled into the output window. 00925 * pass NULL to detach from the resource, further input will be 00926 * ignored 00927 */ 00928 extern int zbar_window_attach(zbar_window_t *window, 00929 void *x11_display_w32_hwnd, 00930 unsigned long x11_drawable); 00931 00932 /** control content level of the reader overlay. 00933 * the overlay displays graphical data for informational or debug 00934 * purposes. higher values increase the level of annotation (possibly 00935 * decreasing performance). @verbatim 00936 0 = disable overlay 00937 1 = outline decoded symbols (default) 00938 2 = also track and display input frame rate 00939 @endverbatim 00940 */ 00941 extern void zbar_window_set_overlay(zbar_window_t *window, 00942 int level); 00943 00944 /** retrieve current content level of reader overlay. 00945 * @see zbar_window_set_overlay() 00946 * @since 0.10 00947 */ 00948 extern int zbar_window_get_overlay(const zbar_window_t *window); 00949 00950 /** draw a new image into the output window. */ 00951 extern int zbar_window_draw(zbar_window_t *window, 00952 zbar_image_t *image); 00953 00954 /** redraw the last image (exposure handler). */ 00955 extern int zbar_window_redraw(zbar_window_t *window); 00956 00957 /** resize the image window (reconfigure handler). 00958 * this does @em not update the contents of the window 00959 * @since 0.3, changed in 0.4 to not redraw window 00960 */ 00961 extern int zbar_window_resize(zbar_window_t *window, 00962 unsigned width, 00963 unsigned height); 00964 00965 /** display detail for last window error to stderr. 00966 * @returns a non-zero value suitable for passing to exit() 00967 */ 00968 static inline int zbar_window_error_spew (const zbar_window_t *window, 00969 int verbosity) 00970 { 00971 return(_zbar_error_spew(window, verbosity)); 00972 } 00973 00974 /** retrieve the detail string for the last window error. */ 00975 static inline const char* 00976 zbar_window_error_string (const zbar_window_t *window, 00977 int verbosity) 00978 { 00979 return(_zbar_error_string(window, verbosity)); 00980 } 00981 00982 /** retrieve the type code for the last window error. */ 00983 static inline zbar_error_t 00984 zbar_window_get_error_code (const zbar_window_t *window) 00985 { 00986 return(_zbar_get_error_code(window)); 00987 } 00988 00989 00990 /** select a compatible format between video input and output window. 00991 * the selection algorithm attempts to use a format shared by 00992 * video input and window output which is also most useful for 00993 * barcode scanning. if a format conversion is necessary, it will 00994 * heuristically attempt to minimize the cost of the conversion 00995 */ 00996 extern int zbar_negotiate_format(zbar_video_t *video, 00997 zbar_window_t *window); 00998 00999 /*@}*/ 01000 01001 /*------------------------------------------------------------*/ 01002 /** @name Image Scanner interface 01003 * @anchor c-imagescanner 01004 * mid-level image scanner interface. 01005 * reads barcodes from 2-D images 01006 */ 01007 /*@{*/ 01008 01009 struct zbar_image_scanner_s; 01010 /** opaque image scanner object. */ 01011 typedef struct zbar_image_scanner_s zbar_image_scanner_t; 01012 01013 /** constructor. */ 01014 extern zbar_image_scanner_t *zbar_image_scanner_create(void); 01015 01016 /** destructor. */ 01017 extern void zbar_image_scanner_destroy(zbar_image_scanner_t *scanner); 01018 01019 /** setup result handler callback. 01020 * the specified function will be called by the scanner whenever 01021 * new results are available from a decoded image. 01022 * pass a NULL value to disable callbacks. 01023 * @returns the previously registered handler 01024 */ 01025 extern zbar_image_data_handler_t* 01026 zbar_image_scanner_set_data_handler(zbar_image_scanner_t *scanner, 01027 zbar_image_data_handler_t *handler, 01028 const void *userdata); 01029 01030 01031 /** set config for indicated symbology (0 for all) to specified value. 01032 * @returns 0 for success, non-0 for failure (config does not apply to 01033 * specified symbology, or value out of range) 01034 * @see zbar_decoder_set_config() 01035 * @since 0.4 01036 */ 01037 extern int zbar_image_scanner_set_config(zbar_image_scanner_t *scanner, 01038 zbar_symbol_type_t symbology, 01039 zbar_config_t config, 01040 int value); 01041 01042 /** parse configuration string using zbar_parse_config() 01043 * and apply to image scanner using zbar_image_scanner_set_config(). 01044 * @returns 0 for success, non-0 for failure 01045 * @see zbar_parse_config() 01046 * @see zbar_image_scanner_set_config() 01047 * @since 0.4 01048 */ 01049 static inline int 01050 zbar_image_scanner_parse_config (zbar_image_scanner_t *scanner, 01051 const char *config_string) 01052 { 01053 zbar_symbol_type_t sym; 01054 zbar_config_t cfg; 01055 int val; 01056 return(zbar_parse_config(config_string, &sym, &cfg, &val) || 01057 zbar_image_scanner_set_config(scanner, sym, cfg, val)); 01058 } 01059 01060 /** enable or disable the inter-image result cache (default disabled). 01061 * mostly useful for scanning video frames, the cache filters 01062 * duplicate results from consecutive images, while adding some 01063 * consistency checking and hysteresis to the results. 01064 * this interface also clears the cache 01065 */ 01066 extern void zbar_image_scanner_enable_cache(zbar_image_scanner_t *scanner, 01067 int enable); 01068 01069 /** remove any previously decoded results from the image scanner and the 01070 * specified image. somewhat more efficient version of 01071 * zbar_image_set_symbols(image, NULL) which may retain memory for 01072 * subsequent decodes 01073 * @since 0.10 01074 */ 01075 extern void zbar_image_scanner_recycle_image(zbar_image_scanner_t *scanner, 01076 zbar_image_t *image); 01077 01078 /** retrieve decode results for last scanned image. 01079 * @returns the symbol set result container or NULL if no results are 01080 * available 01081 * @note the symbol set does not have its reference count adjusted; 01082 * ensure that the count is incremented if the results may be kept 01083 * after the next image is scanned 01084 * @since 0.10 01085 */ 01086 extern const zbar_symbol_set_t* 01087 zbar_image_scanner_get_results(const zbar_image_scanner_t *scanner); 01088 01089 /** scan for symbols in provided image. The image format must be 01090 * "Y800" or "GRAY". 01091 * @returns >0 if symbols were successfully decoded from the image, 01092 * 0 if no symbols were found or -1 if an error occurs 01093 * @see zbar_image_convert() 01094 * @since 0.9 - changed to only accept grayscale images 01095 */ 01096 extern int zbar_scan_image(zbar_image_scanner_t *scanner, 01097 zbar_image_t *image); 01098 01099 /*@}*/ 01100 01101 /*------------------------------------------------------------*/ 01102 /** @name Decoder interface 01103 * @anchor c-decoder 01104 * low-level bar width stream decoder interface. 01105 * identifies symbols and extracts encoded data 01106 */ 01107 /*@{*/ 01108 01109 struct zbar_decoder_s; 01110 /** opaque decoder object. */ 01111 typedef struct zbar_decoder_s zbar_decoder_t; 01112 01113 /** decoder data handler callback function. 01114 * called by decoder when new data has just been decoded 01115 */ 01116 typedef void (zbar_decoder_handler_t)(zbar_decoder_t *decoder); 01117 01118 /** constructor. */ 01119 extern zbar_decoder_t *zbar_decoder_create(void); 01120 01121 /** destructor. */ 01122 extern void zbar_decoder_destroy(zbar_decoder_t *decoder); 01123 01124 /** set config for indicated symbology (0 for all) to specified value. 01125 * @returns 0 for success, non-0 for failure (config does not apply to 01126 * specified symbology, or value out of range) 01127 * @since 0.4 01128 */ 01129 extern int zbar_decoder_set_config(zbar_decoder_t *decoder, 01130 zbar_symbol_type_t symbology, 01131 zbar_config_t config, 01132 int value); 01133 01134 /** parse configuration string using zbar_parse_config() 01135 * and apply to decoder using zbar_decoder_set_config(). 01136 * @returns 0 for success, non-0 for failure 01137 * @see zbar_parse_config() 01138 * @see zbar_decoder_set_config() 01139 * @since 0.4 01140 */ 01141 static inline int zbar_decoder_parse_config (zbar_decoder_t *decoder, 01142 const char *config_string) 01143 { 01144 zbar_symbol_type_t sym; 01145 zbar_config_t cfg; 01146 int val; 01147 return(zbar_parse_config(config_string, &sym, &cfg, &val) || 01148 zbar_decoder_set_config(decoder, sym, cfg, val)); 01149 } 01150 01151 /** clear all decoder state. 01152 * any partial symbols are flushed 01153 */ 01154 extern void zbar_decoder_reset(zbar_decoder_t *decoder); 01155 01156 /** mark start of a new scan pass. 01157 * clears any intra-symbol state and resets color to ::ZBAR_SPACE. 01158 * any partially decoded symbol state is retained 01159 */ 01160 extern void zbar_decoder_new_scan(zbar_decoder_t *decoder); 01161 01162 /** process next bar/space width from input stream. 01163 * the width is in arbitrary relative units. first value of a scan 01164 * is ::ZBAR_SPACE width, alternating from there. 01165 * @returns appropriate symbol type if width completes 01166 * decode of a symbol (data is available for retrieval) 01167 * @returns ::ZBAR_PARTIAL as a hint if part of a symbol was decoded 01168 * @returns ::ZBAR_NONE (0) if no new symbol data is available 01169 */ 01170 extern zbar_symbol_type_t zbar_decode_width(zbar_decoder_t *decoder, 01171 unsigned width); 01172 01173 /** retrieve color of @em next element passed to 01174 * zbar_decode_width(). */ 01175 extern zbar_color_t zbar_decoder_get_color(const zbar_decoder_t *decoder); 01176 01177 /** retrieve last decoded data. 01178 * @returns the data string or NULL if no new data available. 01179 * the returned data buffer is owned by library, contents are only 01180 * valid between non-0 return from zbar_decode_width and next library 01181 * call 01182 */ 01183 extern const char *zbar_decoder_get_data(const zbar_decoder_t *decoder); 01184 01185 /** retrieve length of binary data. 01186 * @returns the length of the decoded data or 0 if no new data 01187 * available. 01188 */ 01189 extern unsigned int 01190 zbar_decoder_get_data_length(const zbar_decoder_t *decoder); 01191 01192 /** retrieve last decoded symbol type. 01193 * @returns the type or ::ZBAR_NONE if no new data available 01194 */ 01195 extern zbar_symbol_type_t 01196 zbar_decoder_get_type(const zbar_decoder_t *decoder); 01197 01198 /** setup data handler callback. 01199 * the registered function will be called by the decoder 01200 * just before zbar_decode_width() returns a non-zero value. 01201 * pass a NULL value to disable callbacks. 01202 * @returns the previously registered handler 01203 */ 01204 extern zbar_decoder_handler_t* 01205 zbar_decoder_set_handler(zbar_decoder_t *decoder, 01206 zbar_decoder_handler_t *handler); 01207 01208 /** associate user specified data value with the decoder. */ 01209 extern void zbar_decoder_set_userdata(zbar_decoder_t *decoder, 01210 void *userdata); 01211 01212 /** return user specified data value associated with the decoder. */ 01213 extern void *zbar_decoder_get_userdata(const zbar_decoder_t *decoder); 01214 01215 /*@}*/ 01216 01217 /*------------------------------------------------------------*/ 01218 /** @name Scanner interface 01219 * @anchor c-scanner 01220 * low-level linear intensity sample stream scanner interface. 01221 * identifies "bar" edges and measures width between them. 01222 * optionally passes to bar width decoder 01223 */ 01224 /*@{*/ 01225 01226 struct zbar_scanner_s; 01227 /** opaque scanner object. */ 01228 typedef struct zbar_scanner_s zbar_scanner_t; 01229 01230 /** constructor. 01231 * if decoder is non-NULL it will be attached to scanner 01232 * and called automatically at each new edge 01233 * current color is initialized to ::ZBAR_SPACE 01234 * (so an initial BAR->SPACE transition may be discarded) 01235 */ 01236 extern zbar_scanner_t *zbar_scanner_create(zbar_decoder_t *decoder); 01237 01238 /** destructor. */ 01239 extern void zbar_scanner_destroy(zbar_scanner_t *scanner); 01240 01241 /** clear all scanner state. 01242 * also resets an associated decoder 01243 */ 01244 extern zbar_symbol_type_t zbar_scanner_reset(zbar_scanner_t *scanner); 01245 01246 /** mark start of a new scan pass. resets color to ::ZBAR_SPACE. 01247 * also updates an associated decoder. 01248 * @returns any decode results flushed from the pipeline 01249 * @note when not using callback handlers, the return value should 01250 * be checked the same as zbar_scan_y() 01251 * @note call zbar_scanner_flush() at least twice before calling this 01252 * method to ensure no decode results are lost 01253 */ 01254 extern zbar_symbol_type_t zbar_scanner_new_scan(zbar_scanner_t *scanner); 01255 01256 /** flush scanner processing pipeline. 01257 * forces current scanner position to be a scan boundary. 01258 * call multiple times (max 3) to completely flush decoder. 01259 * @returns any decode/scan results flushed from the pipeline 01260 * @note when not using callback handlers, the return value should 01261 * be checked the same as zbar_scan_y() 01262 * @since 0.9 01263 */ 01264 extern zbar_symbol_type_t zbar_scanner_flush(zbar_scanner_t *scanner); 01265 01266 /** process next sample intensity value. 01267 * intensity (y) is in arbitrary relative units. 01268 * @returns result of zbar_decode_width() if a decoder is attached, 01269 * otherwise @returns (::ZBAR_PARTIAL) when new edge is detected 01270 * or 0 (::ZBAR_NONE) if no new edge is detected 01271 */ 01272 extern zbar_symbol_type_t zbar_scan_y(zbar_scanner_t *scanner, 01273 int y); 01274 01275 /** process next sample from RGB (or BGR) triple. */ 01276 static inline zbar_symbol_type_t zbar_scan_rgb24 (zbar_scanner_t *scanner, 01277 unsigned char *rgb) 01278 { 01279 return(zbar_scan_y(scanner, rgb[0] + rgb[1] + rgb[2])); 01280 } 01281 01282 /** retrieve last scanned width. */ 01283 extern unsigned zbar_scanner_get_width(const zbar_scanner_t *scanner); 01284 01285 /** retrieve sample position of last edge. 01286 * @since 0.10 01287 */ 01288 extern unsigned zbar_scanner_get_edge(const zbar_scanner_t *scn, 01289 unsigned offset, 01290 int prec); 01291 01292 /** retrieve last scanned color. */ 01293 extern zbar_color_t zbar_scanner_get_color(const zbar_scanner_t *scanner); 01294 01295 /*@}*/ 01296 01297 #ifdef __cplusplus 01298 } 01299 } 01300 01301 # include "zbar/Exception.h" 01302 # include "zbar/Decoder.h" 01303 # include "zbar/Scanner.h" 01304 # include "zbar/Symbol.h" 01305 # include "zbar/Image.h" 01306 # include "zbar/ImageScanner.h" 01307 # include "zbar/Video.h" 01308 # include "zbar/Window.h" 01309 # include "zbar/Processor.h" 01310 #endif 01311 01312 #endif 01313
Generated on Tue Jul 12 2022 18:54:12 by
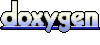