ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
zbar.h File Reference
ZBar Barcode Reader C API definition. More...
Go to the source code of this file.
Namespaces | |
namespace | zbar |
C++ namespace for library interfaces. | |
Global library interfaces | |
enum | zbar_color_e { ZBAR_SPACE = 0, ZBAR_BAR = 1 } |
"color" of element: bar or space. More... | |
enum | zbar_symbol_type_e { ZBAR_NONE = 0, ZBAR_PARTIAL = 1, ZBAR_EAN8 = 8, ZBAR_UPCE = 9, ZBAR_ISBN10 = 10, ZBAR_UPCA = 12, ZBAR_EAN13 = 13, ZBAR_ISBN13 = 14, ZBAR_I25 = 25, ZBAR_CODE39 = 39, ZBAR_PDF417 = 57, ZBAR_QRCODE = 64, ZBAR_CODE128 = 128, ZBAR_SYMBOL = 0x00ff, ZBAR_ADDON2 = 0x0200, ZBAR_ADDON5 = 0x0500, ZBAR_ADDON = 0x0700 } |
decoded symbol type. More... | |
enum | zbar_error_e { ZBAR_OK = 0, ZBAR_ERR_NOMEM, ZBAR_ERR_INTERNAL, ZBAR_ERR_UNSUPPORTED, ZBAR_ERR_INVALID, ZBAR_ERR_SYSTEM, ZBAR_ERR_LOCKING, ZBAR_ERR_BUSY, ZBAR_ERR_XDISPLAY, ZBAR_ERR_XPROTO, ZBAR_ERR_CLOSED, ZBAR_ERR_WINAPI, ZBAR_ERR_NUM } |
error codes. More... | |
enum | zbar_config_e { ZBAR_CFG_ENABLE = 0, ZBAR_CFG_ADD_CHECK, ZBAR_CFG_EMIT_CHECK, ZBAR_CFG_ASCII, ZBAR_CFG_NUM, ZBAR_CFG_MIN_LEN = 0x20, ZBAR_CFG_MAX_LEN, ZBAR_CFG_POSITION = 0x80, ZBAR_CFG_X_DENSITY = 0x100, ZBAR_CFG_Y_DENSITY } |
decoder configuration options. More... | |
typedef enum zbar::zbar_color_e | zbar_color_t |
"color" of element: bar or space. | |
typedef enum zbar::zbar_symbol_type_e | zbar_symbol_type_t |
decoded symbol type. | |
typedef enum zbar::zbar_error_e | zbar_error_t |
error codes. | |
typedef enum zbar::zbar_config_e | zbar_config_t |
decoder configuration options. | |
int | zbar_version (unsigned *major, unsigned *minor) |
retrieve runtime library version information. | |
void | zbar_set_verbosity (int verbosity) |
set global library debug level. | |
void | zbar_increase_verbosity (void) |
increase global library debug level. | |
const char * | zbar_get_symbol_name (zbar_symbol_type_t sym) |
retrieve string name for symbol encoding. | |
const char * | zbar_get_addon_name (zbar_symbol_type_t sym) |
retrieve string name for addon encoding. | |
int | zbar_parse_config (const char *config_string, zbar_symbol_type_t *symbology, zbar_config_t *config, int *value) |
parse a configuration string of the form "[symbology.]config[=value]". | |
int | _zbar_error_spew (const void *object, int verbosity) |
const char * | _zbar_error_string (const void *object, int verbosity) |
zbar_error_t | _zbar_get_error_code (const void *object) |
Symbol interface | |
decoded barcode symbol result object. stores type, data, and image location of decoded symbol. all memory is owned by the library | |
typedef struct zbar_symbol_s | zbar_symbol_t |
opaque decoded symbol object. | |
void | zbar_symbol_ref (const zbar_symbol_t *symbol, int refs) |
symbol reference count manipulation. | |
zbar_symbol_type_t | zbar_symbol_get_type (const zbar_symbol_t *symbol) |
retrieve type of decoded symbol. | |
const char * | zbar_symbol_get_data (const zbar_symbol_t *symbol) |
retrieve data decoded from symbol. | |
unsigned int | zbar_symbol_get_data_length (const zbar_symbol_t *symbol) |
retrieve length of binary data. | |
int | zbar_symbol_get_quality (const zbar_symbol_t *symbol) |
retrieve a symbol confidence metric. | |
int | zbar_symbol_get_count (const zbar_symbol_t *symbol) |
retrieve current cache count. | |
unsigned | zbar_symbol_get_loc_size (const zbar_symbol_t *symbol) |
retrieve the number of points in the location polygon. | |
int | zbar_symbol_get_loc_x (const zbar_symbol_t *symbol, unsigned index) |
retrieve location polygon x-coordinates. | |
int | zbar_symbol_get_loc_y (const zbar_symbol_t *symbol, unsigned index) |
retrieve location polygon y-coordinates. | |
const zbar_symbol_t * | zbar_symbol_next (const zbar_symbol_t *symbol) |
iterate the set to which this symbol belongs (there can be only one). | |
const zbar_symbol_set_t * | zbar_symbol_get_components (const zbar_symbol_t *symbol) |
retrieve components of a composite result. | |
const zbar_symbol_t * | zbar_symbol_first_component (const zbar_symbol_t *symbol) |
iterate components of a composite result. | |
char * | zbar_symbol_xml (const zbar_symbol_t *symbol, char **buffer, unsigned *buflen) |
print XML symbol element representation to user result buffer. | |
Symbol Set interface | |
container for decoded result symbols associated with an image or a composite symbol.
| |
typedef struct zbar_symbol_set_s | zbar_symbol_set_t |
opaque symbol iterator object. | |
void | zbar_symbol_set_ref (const zbar_symbol_set_t *symbols, int refs) |
reference count manipulation. | |
int | zbar_symbol_set_get_size (const zbar_symbol_set_t *symbols) |
retrieve set size. | |
const zbar_symbol_t * | zbar_symbol_set_first_symbol (const zbar_symbol_set_t *symbols) |
set iterator. | |
Image interface | |
stores image data samples along with associated format and size metadata | |
typedef struct zbar_image_s | zbar_image_t |
opaque image object. | |
typedef void( | zbar_image_cleanup_handler_t )(zbar_image_t *image) |
cleanup handler callback function. | |
typedef void( | zbar_image_data_handler_t )(zbar_image_t *image, const void *userdata) |
data handler callback function. | |
zbar_image_t * | zbar_image_create (void) |
new image constructor. | |
void | zbar_image_destroy (zbar_image_t *image) |
image destructor. | |
void | zbar_image_ref (zbar_image_t *image, int refs) |
image reference count manipulation. | |
zbar_image_t * | zbar_image_convert (const zbar_image_t *image, unsigned long format) |
image format conversion. | |
zbar_image_t * | zbar_image_convert_resize (const zbar_image_t *image, unsigned long format, unsigned width, unsigned height) |
image format conversion with crop/pad. | |
unsigned long | zbar_image_get_format (const zbar_image_t *image) |
retrieve the image format. | |
unsigned | zbar_image_get_sequence (const zbar_image_t *image) |
retrieve a "sequence" (page/frame) number associated with this image. | |
unsigned | zbar_image_get_width (const zbar_image_t *image) |
retrieve the width of the image. | |
unsigned | zbar_image_get_height (const zbar_image_t *image) |
retrieve the height of the image. | |
const void * | zbar_image_get_data (const zbar_image_t *image) |
return the image sample data. | |
unsigned long | zbar_image_get_data_length (const zbar_image_t *img) |
return the size of image data. | |
const zbar_symbol_set_t * | zbar_image_get_symbols (const zbar_image_t *image) |
retrieve the decoded results. | |
void | zbar_image_set_symbols (zbar_image_t *image, const zbar_symbol_set_t *symbols) |
associate the specified symbol set with the image, replacing any existing results. | |
const zbar_symbol_t * | zbar_image_first_symbol (const zbar_image_t *image) |
image_scanner decode result iterator. | |
void | zbar_image_set_format (zbar_image_t *image, unsigned long format) |
specify the fourcc image format code for image sample data. | |
void | zbar_image_set_sequence (zbar_image_t *image, unsigned sequence_num) |
associate a "sequence" (page/frame) number with this image. | |
void | zbar_image_set_size (zbar_image_t *image, unsigned width, unsigned height) |
specify the pixel size of the image. | |
void | zbar_image_set_data (zbar_image_t *image, const void *data, unsigned long data_byte_length, zbar_image_cleanup_handler_t *cleanup_hndlr) |
specify image sample data. | |
void | zbar_image_free_data (zbar_image_t *image) |
built-in cleanup handler. | |
void | zbar_image_set_userdata (zbar_image_t *image, void *userdata) |
associate user specified data value with an image. | |
void * | zbar_image_get_userdata (const zbar_image_t *image) |
return user specified data value associated with the image. | |
int | zbar_image_write (const zbar_image_t *image, const char *filebase) |
dump raw image data to a file for debug. | |
zbar_image_t * | zbar_image_read (char *filename) |
read back an image in the format written by zbar_image_write() | |
Processor interface | |
high-level self-contained image processor. processes video and images for barcodes, optionally displaying images to a library owned output window | |
typedef struct zbar_processor_s | zbar_processor_t |
opaque standalone processor object. | |
zbar_processor_t * | zbar_processor_create (int threaded) |
constructor. | |
void | zbar_processor_destroy (zbar_processor_t *processor) |
destructor. | |
int | zbar_processor_init (zbar_processor_t *processor, const char *video_device, int enable_display) |
(re)initialization. | |
int | zbar_processor_request_size (zbar_processor_t *processor, unsigned width, unsigned height) |
request a preferred size for the video image from the device. | |
int | zbar_processor_request_interface (zbar_processor_t *processor, int version) |
request a preferred video driver interface version for debug/testing. | |
int | zbar_processor_request_iomode (zbar_processor_t *video, int iomode) |
request a preferred video I/O mode for debug/testing. | |
int | zbar_processor_force_format (zbar_processor_t *processor, unsigned long input_format, unsigned long output_format) |
force specific input and output formats for debug/testing. | |
zbar_image_data_handler_t * | zbar_processor_set_data_handler (zbar_processor_t *processor, zbar_image_data_handler_t *handler, const void *userdata) |
setup result handler callback. | |
void | zbar_processor_set_userdata (zbar_processor_t *processor, void *userdata) |
associate user specified data value with the processor. | |
void * | zbar_processor_get_userdata (const zbar_processor_t *processor) |
return user specified data value associated with the processor. | |
int | zbar_processor_set_config (zbar_processor_t *processor, zbar_symbol_type_t symbology, zbar_config_t config, int value) |
set config for indicated symbology (0 for all) to specified value. | |
static int | zbar_processor_parse_config (zbar_processor_t *processor, const char *config_string) |
parse configuration string using zbar_parse_config() and apply to processor using zbar_processor_set_config(). | |
int | zbar_processor_is_visible (zbar_processor_t *processor) |
retrieve the current state of the ouput window. | |
int | zbar_processor_set_visible (zbar_processor_t *processor, int visible) |
show or hide the display window owned by the library. | |
int | zbar_processor_set_active (zbar_processor_t *processor, int active) |
control the processor in free running video mode. | |
const zbar_symbol_set_t * | zbar_processor_get_results (const zbar_processor_t *processor) |
retrieve decode results for last scanned image/frame. | |
int | zbar_processor_user_wait (zbar_processor_t *processor, int timeout) |
wait for input to the display window from the user (via mouse or keyboard). | |
int | zbar_process_one (zbar_processor_t *processor, int timeout) |
process from the video stream until a result is available, or the timeout (in milliseconds) expires. | |
int | zbar_process_image (zbar_processor_t *processor, zbar_image_t *image) |
process the provided image for barcodes. | |
static int | zbar_processor_error_spew (const zbar_processor_t *processor, int verbosity) |
display detail for last processor error to stderr. | |
static const char * | zbar_processor_error_string (const zbar_processor_t *processor, int verbosity) |
retrieve the detail string for the last processor error. | |
static zbar_error_t | zbar_processor_get_error_code (const zbar_processor_t *processor) |
retrieve the type code for the last processor error. | |
Video interface | |
mid-level video source abstraction. captures images from a video device | |
typedef struct zbar_video_s | zbar_video_t |
opaque video object. | |
zbar_video_t * | zbar_video_create (void) |
constructor. | |
void | zbar_video_destroy (zbar_video_t *video) |
destructor. | |
int | zbar_video_open (zbar_video_t *video, const char *device) |
open and probe a video device. | |
int | zbar_video_get_fd (const zbar_video_t *video) |
retrieve file descriptor associated with open *nix video device useful for using select()/poll() to tell when new images are available (NB v4l2 only!!). | |
int | zbar_video_request_size (zbar_video_t *video, unsigned width, unsigned height) |
request a preferred size for the video image from the device. | |
int | zbar_video_request_interface (zbar_video_t *video, int version) |
request a preferred driver interface version for debug/testing. | |
int | zbar_video_request_iomode (zbar_video_t *video, int iomode) |
request a preferred I/O mode for debug/testing. | |
int | zbar_video_get_width (const zbar_video_t *video) |
retrieve current output image width. | |
int | zbar_video_get_height (const zbar_video_t *video) |
retrieve current output image height. | |
int | zbar_video_init (zbar_video_t *video, unsigned long format) |
initialize video using a specific format for debug. | |
int | zbar_video_enable (zbar_video_t *video, int enable) |
start/stop video capture. | |
zbar_image_t * | zbar_video_next_image (zbar_video_t *video) |
retrieve next captured image. | |
static int | zbar_video_error_spew (const zbar_video_t *video, int verbosity) |
display detail for last video error to stderr. | |
static const char * | zbar_video_error_string (const zbar_video_t *video, int verbosity) |
retrieve the detail string for the last video error. | |
static zbar_error_t | zbar_video_get_error_code (const zbar_video_t *video) |
retrieve the type code for the last video error. | |
Window interface | |
mid-level output window abstraction. displays images to user-specified platform specific output window | |
typedef struct zbar_window_s | zbar_window_t |
opaque window object. | |
zbar_window_t * | zbar_window_create (void) |
constructor. | |
void | zbar_window_destroy (zbar_window_t *window) |
destructor. | |
int | zbar_window_attach (zbar_window_t *window, void *x11_display_w32_hwnd, unsigned long x11_drawable) |
associate reader with an existing platform window. | |
void | zbar_window_set_overlay (zbar_window_t *window, int level) |
control content level of the reader overlay. | |
int | zbar_window_get_overlay (const zbar_window_t *window) |
retrieve current content level of reader overlay. | |
int | zbar_window_draw (zbar_window_t *window, zbar_image_t *image) |
draw a new image into the output window. | |
int | zbar_window_redraw (zbar_window_t *window) |
redraw the last image (exposure handler). | |
int | zbar_window_resize (zbar_window_t *window, unsigned width, unsigned height) |
resize the image window (reconfigure handler). | |
static int | zbar_window_error_spew (const zbar_window_t *window, int verbosity) |
display detail for last window error to stderr. | |
static const char * | zbar_window_error_string (const zbar_window_t *window, int verbosity) |
retrieve the detail string for the last window error. | |
static zbar_error_t | zbar_window_get_error_code (const zbar_window_t *window) |
retrieve the type code for the last window error. | |
int | zbar_negotiate_format (zbar_video_t *video, zbar_window_t *window) |
select a compatible format between video input and output window. | |
Image Scanner interface | |
mid-level image scanner interface. reads barcodes from 2-D images | |
typedef struct zbar_image_scanner_s | zbar_image_scanner_t |
opaque image scanner object. | |
zbar_image_scanner_t * | zbar_image_scanner_create (void) |
constructor. | |
void | zbar_image_scanner_destroy (zbar_image_scanner_t *scanner) |
destructor. | |
zbar_image_data_handler_t * | zbar_image_scanner_set_data_handler (zbar_image_scanner_t *scanner, zbar_image_data_handler_t *handler, const void *userdata) |
setup result handler callback. | |
int | zbar_image_scanner_set_config (zbar_image_scanner_t *scanner, zbar_symbol_type_t symbology, zbar_config_t config, int value) |
set config for indicated symbology (0 for all) to specified value. | |
static int | zbar_image_scanner_parse_config (zbar_image_scanner_t *scanner, const char *config_string) |
parse configuration string using zbar_parse_config() and apply to image scanner using zbar_image_scanner_set_config(). | |
void | zbar_image_scanner_enable_cache (zbar_image_scanner_t *scanner, int enable) |
enable or disable the inter-image result cache (default disabled). | |
void | zbar_image_scanner_recycle_image (zbar_image_scanner_t *scanner, zbar_image_t *image) |
remove any previously decoded results from the image scanner and the specified image. | |
const zbar_symbol_set_t * | zbar_image_scanner_get_results (const zbar_image_scanner_t *scanner) |
retrieve decode results for last scanned image. | |
int | zbar_scan_image (zbar_image_scanner_t *scanner, zbar_image_t *image) |
scan for symbols in provided image. | |
Decoder interface | |
low-level bar width stream decoder interface. identifies symbols and extracts encoded data | |
typedef struct zbar_decoder_s | zbar_decoder_t |
opaque decoder object. | |
typedef void( | zbar_decoder_handler_t )(zbar_decoder_t *decoder) |
decoder data handler callback function. | |
zbar_decoder_t * | zbar_decoder_create (void) |
constructor. | |
void | zbar_decoder_destroy (zbar_decoder_t *decoder) |
destructor. | |
int | zbar_decoder_set_config (zbar_decoder_t *decoder, zbar_symbol_type_t symbology, zbar_config_t config, int value) |
set config for indicated symbology (0 for all) to specified value. | |
static int | zbar_decoder_parse_config (zbar_decoder_t *decoder, const char *config_string) |
parse configuration string using zbar_parse_config() and apply to decoder using zbar_decoder_set_config(). | |
void | zbar_decoder_reset (zbar_decoder_t *decoder) |
clear all decoder state. | |
void | zbar_decoder_new_scan (zbar_decoder_t *decoder) |
mark start of a new scan pass. | |
zbar_symbol_type_t | zbar_decode_width (zbar_decoder_t *decoder, unsigned width) |
process next bar/space width from input stream. | |
zbar_color_t | zbar_decoder_get_color (const zbar_decoder_t *decoder) |
retrieve color of next element passed to zbar_decode_width(). | |
const char * | zbar_decoder_get_data (const zbar_decoder_t *decoder) |
retrieve last decoded data. | |
unsigned int | zbar_decoder_get_data_length (const zbar_decoder_t *decoder) |
retrieve length of binary data. | |
zbar_symbol_type_t | zbar_decoder_get_type (const zbar_decoder_t *decoder) |
retrieve last decoded symbol type. | |
zbar_decoder_handler_t * | zbar_decoder_set_handler (zbar_decoder_t *decoder, zbar_decoder_handler_t *handler) |
setup data handler callback. | |
void | zbar_decoder_set_userdata (zbar_decoder_t *decoder, void *userdata) |
associate user specified data value with the decoder. | |
void * | zbar_decoder_get_userdata (const zbar_decoder_t *decoder) |
return user specified data value associated with the decoder. | |
Scanner interface | |
low-level linear intensity sample stream scanner interface. identifies "bar" edges and measures width between them. optionally passes to bar width decoder | |
typedef struct zbar_scanner_s | zbar_scanner_t |
opaque scanner object. | |
zbar_scanner_t * | zbar_scanner_create (zbar_decoder_t *decoder) |
constructor. | |
void | zbar_scanner_destroy (zbar_scanner_t *scanner) |
destructor. | |
zbar_symbol_type_t | zbar_scanner_reset (zbar_scanner_t *scanner) |
clear all scanner state. | |
zbar_symbol_type_t | zbar_scanner_new_scan (zbar_scanner_t *scanner) |
mark start of a new scan pass. | |
zbar_symbol_type_t | zbar_scanner_flush (zbar_scanner_t *scanner) |
flush scanner processing pipeline. | |
zbar_symbol_type_t | zbar_scan_y (zbar_scanner_t *scanner, int y) |
process next sample intensity value. | |
static zbar_symbol_type_t | zbar_scan_rgb24 (zbar_scanner_t *scanner, unsigned char *rgb) |
process next sample from RGB (or BGR) triple. | |
unsigned | zbar_scanner_get_width (const zbar_scanner_t *scanner) |
retrieve last scanned width. | |
unsigned | zbar_scanner_get_edge (const zbar_scanner_t *scn, unsigned offset, int prec) |
retrieve sample position of last edge. | |
zbar_color_t | zbar_scanner_get_color (const zbar_scanner_t *scanner) |
retrieve last scanned color. |
Detailed Description
ZBar Barcode Reader C API definition.
Definition in file zbar.h.
Generated on Tue Jul 12 2022 18:54:12 by
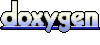