ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
zbar Namespace Reference
C++ namespace for library interfaces. More...
Global library interfaces | |
enum | zbar_color_e { ZBAR_SPACE = 0, ZBAR_BAR = 1 } |
"color" of element: bar or space. More... | |
enum | zbar_symbol_type_e { ZBAR_NONE = 0, ZBAR_PARTIAL = 1, ZBAR_EAN8 = 8, ZBAR_UPCE = 9, ZBAR_ISBN10 = 10, ZBAR_UPCA = 12, ZBAR_EAN13 = 13, ZBAR_ISBN13 = 14, ZBAR_I25 = 25, ZBAR_CODE39 = 39, ZBAR_PDF417 = 57, ZBAR_QRCODE = 64, ZBAR_CODE128 = 128, ZBAR_SYMBOL = 0x00ff, ZBAR_ADDON2 = 0x0200, ZBAR_ADDON5 = 0x0500, ZBAR_ADDON = 0x0700 } |
decoded symbol type. More... | |
enum | zbar_error_e { ZBAR_OK = 0, ZBAR_ERR_NOMEM, ZBAR_ERR_INTERNAL, ZBAR_ERR_UNSUPPORTED, ZBAR_ERR_INVALID, ZBAR_ERR_SYSTEM, ZBAR_ERR_LOCKING, ZBAR_ERR_BUSY, ZBAR_ERR_XDISPLAY, ZBAR_ERR_XPROTO, ZBAR_ERR_CLOSED, ZBAR_ERR_WINAPI, ZBAR_ERR_NUM } |
error codes. More... | |
enum | zbar_config_e { ZBAR_CFG_ENABLE = 0, ZBAR_CFG_ADD_CHECK, ZBAR_CFG_EMIT_CHECK, ZBAR_CFG_ASCII, ZBAR_CFG_NUM, ZBAR_CFG_MIN_LEN = 0x20, ZBAR_CFG_MAX_LEN, ZBAR_CFG_POSITION = 0x80, ZBAR_CFG_X_DENSITY = 0x100, ZBAR_CFG_Y_DENSITY } |
decoder configuration options. More... | |
typedef enum zbar::zbar_color_e | zbar_color_t |
"color" of element: bar or space. | |
typedef enum zbar::zbar_symbol_type_e | zbar_symbol_type_t |
decoded symbol type. | |
typedef enum zbar::zbar_error_e | zbar_error_t |
error codes. | |
typedef enum zbar::zbar_config_e | zbar_config_t |
decoder configuration options. | |
int | zbar_version (unsigned *major, unsigned *minor) |
retrieve runtime library version information. | |
void | zbar_set_verbosity (int verbosity) |
set global library debug level. | |
void | zbar_increase_verbosity (void) |
increase global library debug level. | |
const char * | zbar_get_symbol_name (zbar_symbol_type_t sym) |
retrieve string name for symbol encoding. | |
const char * | zbar_get_addon_name (zbar_symbol_type_t sym) |
retrieve string name for addon encoding. | |
int | zbar_parse_config (const char *config_string, zbar_symbol_type_t *symbology, zbar_config_t *config, int *value) |
parse a configuration string of the form "[symbology.]config[=value]". | |
int | _zbar_error_spew (const void *object, int verbosity) |
const char * | _zbar_error_string (const void *object, int verbosity) |
zbar_error_t | _zbar_get_error_code (const void *object) |
Symbol interface | |
decoded barcode symbol result object. stores type, data, and image location of decoded symbol. all memory is owned by the library | |
typedef struct zbar_symbol_s | zbar_symbol_t |
opaque decoded symbol object. | |
void | zbar_symbol_ref (const zbar_symbol_t *symbol, int refs) |
symbol reference count manipulation. | |
zbar_symbol_type_t | zbar_symbol_get_type (const zbar_symbol_t *symbol) |
retrieve type of decoded symbol. | |
const char * | zbar_symbol_get_data (const zbar_symbol_t *symbol) |
retrieve data decoded from symbol. | |
unsigned int | zbar_symbol_get_data_length (const zbar_symbol_t *symbol) |
retrieve length of binary data. | |
int | zbar_symbol_get_quality (const zbar_symbol_t *symbol) |
retrieve a symbol confidence metric. | |
int | zbar_symbol_get_count (const zbar_symbol_t *symbol) |
retrieve current cache count. | |
unsigned | zbar_symbol_get_loc_size (const zbar_symbol_t *symbol) |
retrieve the number of points in the location polygon. | |
int | zbar_symbol_get_loc_x (const zbar_symbol_t *symbol, unsigned index) |
retrieve location polygon x-coordinates. | |
int | zbar_symbol_get_loc_y (const zbar_symbol_t *symbol, unsigned index) |
retrieve location polygon y-coordinates. | |
const zbar_symbol_t * | zbar_symbol_next (const zbar_symbol_t *symbol) |
iterate the set to which this symbol belongs (there can be only one). | |
const zbar_symbol_set_t * | zbar_symbol_get_components (const zbar_symbol_t *symbol) |
retrieve components of a composite result. | |
const zbar_symbol_t * | zbar_symbol_first_component (const zbar_symbol_t *symbol) |
iterate components of a composite result. | |
char * | zbar_symbol_xml (const zbar_symbol_t *symbol, char **buffer, unsigned *buflen) |
print XML symbol element representation to user result buffer. | |
Symbol Set interface | |
container for decoded result symbols associated with an image or a composite symbol.
| |
typedef struct zbar_symbol_set_s | zbar_symbol_set_t |
opaque symbol iterator object. | |
void | zbar_symbol_set_ref (const zbar_symbol_set_t *symbols, int refs) |
reference count manipulation. | |
int | zbar_symbol_set_get_size (const zbar_symbol_set_t *symbols) |
retrieve set size. | |
const zbar_symbol_t * | zbar_symbol_set_first_symbol (const zbar_symbol_set_t *symbols) |
set iterator. | |
Image interface | |
stores image data samples along with associated format and size metadata | |
typedef struct zbar_image_s | zbar_image_t |
opaque image object. | |
typedef void( | zbar_image_cleanup_handler_t )(zbar_image_t *image) |
cleanup handler callback function. | |
typedef void( | zbar_image_data_handler_t )(zbar_image_t *image, const void *userdata) |
data handler callback function. | |
zbar_image_t * | zbar_image_create (void) |
new image constructor. | |
void | zbar_image_destroy (zbar_image_t *image) |
image destructor. | |
void | zbar_image_ref (zbar_image_t *image, int refs) |
image reference count manipulation. | |
zbar_image_t * | zbar_image_convert (const zbar_image_t *image, unsigned long format) |
image format conversion. | |
zbar_image_t * | zbar_image_convert_resize (const zbar_image_t *image, unsigned long format, unsigned width, unsigned height) |
image format conversion with crop/pad. | |
unsigned long | zbar_image_get_format (const zbar_image_t *image) |
retrieve the image format. | |
unsigned | zbar_image_get_sequence (const zbar_image_t *image) |
retrieve a "sequence" (page/frame) number associated with this image. | |
unsigned | zbar_image_get_width (const zbar_image_t *image) |
retrieve the width of the image. | |
unsigned | zbar_image_get_height (const zbar_image_t *image) |
retrieve the height of the image. | |
const void * | zbar_image_get_data (const zbar_image_t *image) |
return the image sample data. | |
unsigned long | zbar_image_get_data_length (const zbar_image_t *img) |
return the size of image data. | |
const zbar_symbol_set_t * | zbar_image_get_symbols (const zbar_image_t *image) |
retrieve the decoded results. | |
void | zbar_image_set_symbols (zbar_image_t *image, const zbar_symbol_set_t *symbols) |
associate the specified symbol set with the image, replacing any existing results. | |
const zbar_symbol_t * | zbar_image_first_symbol (const zbar_image_t *image) |
image_scanner decode result iterator. | |
void | zbar_image_set_format (zbar_image_t *image, unsigned long format) |
specify the fourcc image format code for image sample data. | |
void | zbar_image_set_sequence (zbar_image_t *image, unsigned sequence_num) |
associate a "sequence" (page/frame) number with this image. | |
void | zbar_image_set_size (zbar_image_t *image, unsigned width, unsigned height) |
specify the pixel size of the image. | |
void | zbar_image_set_data (zbar_image_t *image, const void *data, unsigned long data_byte_length, zbar_image_cleanup_handler_t *cleanup_hndlr) |
specify image sample data. | |
void | zbar_image_free_data (zbar_image_t *image) |
built-in cleanup handler. | |
void | zbar_image_set_userdata (zbar_image_t *image, void *userdata) |
associate user specified data value with an image. | |
void * | zbar_image_get_userdata (const zbar_image_t *image) |
return user specified data value associated with the image. | |
int | zbar_image_write (const zbar_image_t *image, const char *filebase) |
dump raw image data to a file for debug. | |
zbar_image_t * | zbar_image_read (char *filename) |
read back an image in the format written by zbar_image_write() | |
Processor interface | |
high-level self-contained image processor. processes video and images for barcodes, optionally displaying images to a library owned output window | |
typedef struct zbar_processor_s | zbar_processor_t |
opaque standalone processor object. | |
zbar_processor_t * | zbar_processor_create (int threaded) |
constructor. | |
void | zbar_processor_destroy (zbar_processor_t *processor) |
destructor. | |
int | zbar_processor_init (zbar_processor_t *processor, const char *video_device, int enable_display) |
(re)initialization. | |
int | zbar_processor_request_size (zbar_processor_t *processor, unsigned width, unsigned height) |
request a preferred size for the video image from the device. | |
int | zbar_processor_request_interface (zbar_processor_t *processor, int version) |
request a preferred video driver interface version for debug/testing. | |
int | zbar_processor_request_iomode (zbar_processor_t *video, int iomode) |
request a preferred video I/O mode for debug/testing. | |
int | zbar_processor_force_format (zbar_processor_t *processor, unsigned long input_format, unsigned long output_format) |
force specific input and output formats for debug/testing. | |
zbar_image_data_handler_t * | zbar_processor_set_data_handler (zbar_processor_t *processor, zbar_image_data_handler_t *handler, const void *userdata) |
setup result handler callback. | |
void | zbar_processor_set_userdata (zbar_processor_t *processor, void *userdata) |
associate user specified data value with the processor. | |
void * | zbar_processor_get_userdata (const zbar_processor_t *processor) |
return user specified data value associated with the processor. | |
int | zbar_processor_set_config (zbar_processor_t *processor, zbar_symbol_type_t symbology, zbar_config_t config, int value) |
set config for indicated symbology (0 for all) to specified value. | |
static int | zbar_processor_parse_config (zbar_processor_t *processor, const char *config_string) |
parse configuration string using zbar_parse_config() and apply to processor using zbar_processor_set_config(). | |
int | zbar_processor_is_visible (zbar_processor_t *processor) |
retrieve the current state of the ouput window. | |
int | zbar_processor_set_visible (zbar_processor_t *processor, int visible) |
show or hide the display window owned by the library. | |
int | zbar_processor_set_active (zbar_processor_t *processor, int active) |
control the processor in free running video mode. | |
const zbar_symbol_set_t * | zbar_processor_get_results (const zbar_processor_t *processor) |
retrieve decode results for last scanned image/frame. | |
int | zbar_processor_user_wait (zbar_processor_t *processor, int timeout) |
wait for input to the display window from the user (via mouse or keyboard). | |
int | zbar_process_one (zbar_processor_t *processor, int timeout) |
process from the video stream until a result is available, or the timeout (in milliseconds) expires. | |
int | zbar_process_image (zbar_processor_t *processor, zbar_image_t *image) |
process the provided image for barcodes. | |
static int | zbar_processor_error_spew (const zbar_processor_t *processor, int verbosity) |
display detail for last processor error to stderr. | |
static const char * | zbar_processor_error_string (const zbar_processor_t *processor, int verbosity) |
retrieve the detail string for the last processor error. | |
static zbar_error_t | zbar_processor_get_error_code (const zbar_processor_t *processor) |
retrieve the type code for the last processor error. | |
Video interface | |
mid-level video source abstraction. captures images from a video device | |
typedef struct zbar_video_s | zbar_video_t |
opaque video object. | |
zbar_video_t * | zbar_video_create (void) |
constructor. | |
void | zbar_video_destroy (zbar_video_t *video) |
destructor. | |
int | zbar_video_open (zbar_video_t *video, const char *device) |
open and probe a video device. | |
int | zbar_video_get_fd (const zbar_video_t *video) |
retrieve file descriptor associated with open *nix video device useful for using select()/poll() to tell when new images are available (NB v4l2 only!!). | |
int | zbar_video_request_size (zbar_video_t *video, unsigned width, unsigned height) |
request a preferred size for the video image from the device. | |
int | zbar_video_request_interface (zbar_video_t *video, int version) |
request a preferred driver interface version for debug/testing. | |
int | zbar_video_request_iomode (zbar_video_t *video, int iomode) |
request a preferred I/O mode for debug/testing. | |
int | zbar_video_get_width (const zbar_video_t *video) |
retrieve current output image width. | |
int | zbar_video_get_height (const zbar_video_t *video) |
retrieve current output image height. | |
int | zbar_video_init (zbar_video_t *video, unsigned long format) |
initialize video using a specific format for debug. | |
int | zbar_video_enable (zbar_video_t *video, int enable) |
start/stop video capture. | |
zbar_image_t * | zbar_video_next_image (zbar_video_t *video) |
retrieve next captured image. | |
static int | zbar_video_error_spew (const zbar_video_t *video, int verbosity) |
display detail for last video error to stderr. | |
static const char * | zbar_video_error_string (const zbar_video_t *video, int verbosity) |
retrieve the detail string for the last video error. | |
static zbar_error_t | zbar_video_get_error_code (const zbar_video_t *video) |
retrieve the type code for the last video error. | |
Window interface | |
mid-level output window abstraction. displays images to user-specified platform specific output window | |
typedef struct zbar_window_s | zbar_window_t |
opaque window object. | |
zbar_window_t * | zbar_window_create (void) |
constructor. | |
void | zbar_window_destroy (zbar_window_t *window) |
destructor. | |
int | zbar_window_attach (zbar_window_t *window, void *x11_display_w32_hwnd, unsigned long x11_drawable) |
associate reader with an existing platform window. | |
void | zbar_window_set_overlay (zbar_window_t *window, int level) |
control content level of the reader overlay. | |
int | zbar_window_get_overlay (const zbar_window_t *window) |
retrieve current content level of reader overlay. | |
int | zbar_window_draw (zbar_window_t *window, zbar_image_t *image) |
draw a new image into the output window. | |
int | zbar_window_redraw (zbar_window_t *window) |
redraw the last image (exposure handler). | |
int | zbar_window_resize (zbar_window_t *window, unsigned width, unsigned height) |
resize the image window (reconfigure handler). | |
static int | zbar_window_error_spew (const zbar_window_t *window, int verbosity) |
display detail for last window error to stderr. | |
static const char * | zbar_window_error_string (const zbar_window_t *window, int verbosity) |
retrieve the detail string for the last window error. | |
static zbar_error_t | zbar_window_get_error_code (const zbar_window_t *window) |
retrieve the type code for the last window error. | |
int | zbar_negotiate_format (zbar_video_t *video, zbar_window_t *window) |
select a compatible format between video input and output window. | |
Image Scanner interface | |
mid-level image scanner interface. reads barcodes from 2-D images | |
typedef struct zbar_image_scanner_s | zbar_image_scanner_t |
opaque image scanner object. | |
zbar_image_scanner_t * | zbar_image_scanner_create (void) |
constructor. | |
void | zbar_image_scanner_destroy (zbar_image_scanner_t *scanner) |
destructor. | |
zbar_image_data_handler_t * | zbar_image_scanner_set_data_handler (zbar_image_scanner_t *scanner, zbar_image_data_handler_t *handler, const void *userdata) |
setup result handler callback. | |
int | zbar_image_scanner_set_config (zbar_image_scanner_t *scanner, zbar_symbol_type_t symbology, zbar_config_t config, int value) |
set config for indicated symbology (0 for all) to specified value. | |
static int | zbar_image_scanner_parse_config (zbar_image_scanner_t *scanner, const char *config_string) |
parse configuration string using zbar_parse_config() and apply to image scanner using zbar_image_scanner_set_config(). | |
void | zbar_image_scanner_enable_cache (zbar_image_scanner_t *scanner, int enable) |
enable or disable the inter-image result cache (default disabled). | |
void | zbar_image_scanner_recycle_image (zbar_image_scanner_t *scanner, zbar_image_t *image) |
remove any previously decoded results from the image scanner and the specified image. | |
const zbar_symbol_set_t * | zbar_image_scanner_get_results (const zbar_image_scanner_t *scanner) |
retrieve decode results for last scanned image. | |
int | zbar_scan_image (zbar_image_scanner_t *scanner, zbar_image_t *image) |
scan for symbols in provided image. | |
Decoder interface | |
low-level bar width stream decoder interface. identifies symbols and extracts encoded data | |
typedef struct zbar_decoder_s | zbar_decoder_t |
opaque decoder object. | |
typedef void( | zbar_decoder_handler_t )(zbar_decoder_t *decoder) |
decoder data handler callback function. | |
zbar_decoder_t * | zbar_decoder_create (void) |
constructor. | |
void | zbar_decoder_destroy (zbar_decoder_t *decoder) |
destructor. | |
int | zbar_decoder_set_config (zbar_decoder_t *decoder, zbar_symbol_type_t symbology, zbar_config_t config, int value) |
set config for indicated symbology (0 for all) to specified value. | |
static int | zbar_decoder_parse_config (zbar_decoder_t *decoder, const char *config_string) |
parse configuration string using zbar_parse_config() and apply to decoder using zbar_decoder_set_config(). | |
void | zbar_decoder_reset (zbar_decoder_t *decoder) |
clear all decoder state. | |
void | zbar_decoder_new_scan (zbar_decoder_t *decoder) |
mark start of a new scan pass. | |
zbar_symbol_type_t | zbar_decode_width (zbar_decoder_t *decoder, unsigned width) |
process next bar/space width from input stream. | |
zbar_color_t | zbar_decoder_get_color (const zbar_decoder_t *decoder) |
retrieve color of next element passed to zbar_decode_width(). | |
const char * | zbar_decoder_get_data (const zbar_decoder_t *decoder) |
retrieve last decoded data. | |
unsigned int | zbar_decoder_get_data_length (const zbar_decoder_t *decoder) |
retrieve length of binary data. | |
zbar_symbol_type_t | zbar_decoder_get_type (const zbar_decoder_t *decoder) |
retrieve last decoded symbol type. | |
zbar_decoder_handler_t * | zbar_decoder_set_handler (zbar_decoder_t *decoder, zbar_decoder_handler_t *handler) |
setup data handler callback. | |
void | zbar_decoder_set_userdata (zbar_decoder_t *decoder, void *userdata) |
associate user specified data value with the decoder. | |
void * | zbar_decoder_get_userdata (const zbar_decoder_t *decoder) |
return user specified data value associated with the decoder. | |
Scanner interface | |
low-level linear intensity sample stream scanner interface. identifies "bar" edges and measures width between them. optionally passes to bar width decoder | |
typedef struct zbar_scanner_s | zbar_scanner_t |
opaque scanner object. | |
zbar_scanner_t * | zbar_scanner_create (zbar_decoder_t *decoder) |
constructor. | |
void | zbar_scanner_destroy (zbar_scanner_t *scanner) |
destructor. | |
zbar_symbol_type_t | zbar_scanner_reset (zbar_scanner_t *scanner) |
clear all scanner state. | |
zbar_symbol_type_t | zbar_scanner_new_scan (zbar_scanner_t *scanner) |
mark start of a new scan pass. | |
zbar_symbol_type_t | zbar_scanner_flush (zbar_scanner_t *scanner) |
flush scanner processing pipeline. | |
zbar_symbol_type_t | zbar_scan_y (zbar_scanner_t *scanner, int y) |
process next sample intensity value. | |
static zbar_symbol_type_t | zbar_scan_rgb24 (zbar_scanner_t *scanner, unsigned char *rgb) |
process next sample from RGB (or BGR) triple. | |
unsigned | zbar_scanner_get_width (const zbar_scanner_t *scanner) |
retrieve last scanned width. | |
unsigned | zbar_scanner_get_edge (const zbar_scanner_t *scn, unsigned offset, int prec) |
retrieve sample position of last edge. | |
zbar_color_t | zbar_scanner_get_color (const zbar_scanner_t *scanner) |
retrieve last scanned color. |
Detailed Description
C++ namespace for library interfaces.
Typedef Documentation
typedef enum zbar::zbar_color_e zbar_color_t |
"color" of element: bar or space.
typedef enum zbar::zbar_config_e zbar_config_t |
decoder configuration options.
- Since:
- 0.4
typedef void( zbar_decoder_handler_t)(zbar_decoder_t *decoder) |
typedef struct zbar_decoder_s zbar_decoder_t |
typedef enum zbar::zbar_error_e zbar_error_t |
error codes.
typedef void( zbar_image_cleanup_handler_t)(zbar_image_t *image) |
typedef void( zbar_image_data_handler_t)(zbar_image_t *image, const void *userdata) |
typedef struct zbar_image_scanner_s zbar_image_scanner_t |
typedef struct zbar_image_s zbar_image_t |
typedef struct zbar_processor_s zbar_processor_t |
typedef struct zbar_scanner_s zbar_scanner_t |
typedef enum zbar::zbar_symbol_type_e zbar_symbol_type_t |
decoded symbol type.
typedef struct zbar_video_s zbar_video_t |
typedef struct zbar_window_s zbar_window_t |
Enumeration Type Documentation
enum zbar_color_e |
enum zbar_config_e |
decoder configuration options.
- Since:
- 0.4
- Enumerator:
enum zbar_error_e |
error codes.
- Enumerator:
enum zbar_symbol_type_e |
decoded symbol type.
- Enumerator:
Function Documentation
zbar_symbol_type_t zbar::zbar_decode_width | ( | zbar_decoder_t * | decoder, |
unsigned | width | ||
) |
process next bar/space width from input stream.
the width is in arbitrary relative units. first value of a scan is ZBAR_SPACE width, alternating from there.
- Returns:
- appropriate symbol type if width completes decode of a symbol (data is available for retrieval)
- ZBAR_PARTIAL as a hint if part of a symbol was decoded
- ZBAR_NONE (0) if no new symbol data is available
zbar_decoder_t* zbar::zbar_decoder_create | ( | void | ) |
void zbar::zbar_decoder_destroy | ( | zbar_decoder_t * | decoder ) |
destructor.
zbar_color_t zbar::zbar_decoder_get_color | ( | const zbar_decoder_t * | decoder ) |
retrieve color of next element passed to zbar_decode_width().
const char* zbar::zbar_decoder_get_data | ( | const zbar_decoder_t * | decoder ) |
retrieve last decoded data.
- Returns:
- the data string or NULL if no new data available. the returned data buffer is owned by library, contents are only valid between non-0 return from zbar_decode_width and next library call
unsigned int zbar::zbar_decoder_get_data_length | ( | const zbar_decoder_t * | decoder ) |
retrieve length of binary data.
- Returns:
- the length of the decoded data or 0 if no new data available.
zbar_symbol_type_t zbar::zbar_decoder_get_type | ( | const zbar_decoder_t * | decoder ) |
retrieve last decoded symbol type.
- Returns:
- the type or ZBAR_NONE if no new data available
void* zbar::zbar_decoder_get_userdata | ( | const zbar_decoder_t * | decoder ) |
return user specified data value associated with the decoder.
void zbar::zbar_decoder_new_scan | ( | zbar_decoder_t * | decoder ) |
mark start of a new scan pass.
clears any intra-symbol state and resets color to ZBAR_SPACE. any partially decoded symbol state is retained
static int zbar::zbar_decoder_parse_config | ( | zbar_decoder_t * | decoder, |
const char * | config_string | ||
) | [static] |
parse configuration string using zbar_parse_config() and apply to decoder using zbar_decoder_set_config().
- Returns:
- 0 for success, non-0 for failure
- Since:
- 0.4
void zbar::zbar_decoder_reset | ( | zbar_decoder_t * | decoder ) |
clear all decoder state.
any partial symbols are flushed
int zbar::zbar_decoder_set_config | ( | zbar_decoder_t * | decoder, |
zbar_symbol_type_t | symbology, | ||
zbar_config_t | config, | ||
int | value | ||
) |
set config for indicated symbology (0 for all) to specified value.
- Returns:
- 0 for success, non-0 for failure (config does not apply to specified symbology, or value out of range)
- Since:
- 0.4
zbar_decoder_handler_t* zbar::zbar_decoder_set_handler | ( | zbar_decoder_t * | decoder, |
zbar_decoder_handler_t * | handler | ||
) |
setup data handler callback.
the registered function will be called by the decoder just before zbar_decode_width() returns a non-zero value. pass a NULL value to disable callbacks.
- Returns:
- the previously registered handler
void zbar::zbar_decoder_set_userdata | ( | zbar_decoder_t * | decoder, |
void * | userdata | ||
) |
associate user specified data value with the decoder.
const char* zbar::zbar_get_addon_name | ( | zbar_symbol_type_t | sym ) |
retrieve string name for addon encoding.
- Parameters:
-
sym symbol type encoding
- Returns:
- static string name for any addon, or the empty string if no addons were decoded
const char* zbar::zbar_get_symbol_name | ( | zbar_symbol_type_t | sym ) |
retrieve string name for symbol encoding.
- Parameters:
-
sym symbol type encoding
- Returns:
- the static string name for the specified symbol type, or "UNKNOWN" if the encoding is not recognized
zbar_image_t* zbar::zbar_image_convert | ( | const zbar_image_t * | image, |
unsigned long | format | ||
) |
image format conversion.
refer to the documentation for supported image formats
- Returns:
- a new image with the sample data from the original image converted to the requested format. the original image is unaffected.
- Note:
- the converted image size may be rounded (up) due to format constraints
zbar_image_t* zbar::zbar_image_convert_resize | ( | const zbar_image_t * | image, |
unsigned long | format, | ||
unsigned | width, | ||
unsigned | height | ||
) |
image format conversion with crop/pad.
if the requested size is larger than the image, the last row/column are duplicated to cover the difference. if the requested size is smaller than the image, the extra rows/columns are dropped from the right/bottom.
- Returns:
- a new image with the sample data from the original image converted to the requested format and size.
- Note:
- the image is not scaled
- See also:
- zbar_image_convert()
- Since:
- 0.4
zbar_image_t* zbar::zbar_image_create | ( | void | ) |
new image constructor.
- Returns:
- a new image object with uninitialized data and format. this image should be destroyed (using zbar_image_destroy()) as soon as the application is finished with it
void zbar::zbar_image_destroy | ( | zbar_image_t * | image ) |
image destructor.
all images created by or returned to the application should be destroyed using this function. when an image is destroyed, the associated data cleanup handler will be invoked if available
- Note:
- make no assumptions about the image or the data buffer. they may not be destroyed/cleaned immediately if the library is still using them. if necessary, use the cleanup handler hook to keep track of image data buffers
const zbar_symbol_t* zbar::zbar_image_first_symbol | ( | const zbar_image_t * | image ) |
image_scanner decode result iterator.
- Returns:
- the first decoded symbol result for an image or NULL if no results are available
void zbar::zbar_image_free_data | ( | zbar_image_t * | image ) |
built-in cleanup handler.
passes the image data buffer to free()
const void* zbar::zbar_image_get_data | ( | const zbar_image_t * | image ) |
return the image sample data.
the returned data buffer is only valid until zbar_image_destroy() is called
unsigned long zbar::zbar_image_get_data_length | ( | const zbar_image_t * | img ) |
return the size of image data.
- Since:
- 0.6
unsigned long zbar::zbar_image_get_format | ( | const zbar_image_t * | image ) |
retrieve the image format.
- Returns:
- the fourcc describing the format of the image sample data
unsigned zbar::zbar_image_get_height | ( | const zbar_image_t * | image ) |
retrieve the height of the image.
- Returns:
- the height in sample rows
unsigned zbar::zbar_image_get_sequence | ( | const zbar_image_t * | image ) |
retrieve a "sequence" (page/frame) number associated with this image.
- Since:
- 0.6
const zbar_symbol_set_t* zbar::zbar_image_get_symbols | ( | const zbar_image_t * | image ) |
retrieve the decoded results.
- Returns:
- the (possibly empty) set of decoded symbols
- NULL if the image has not been scanned
- Since:
- 0.10
void* zbar::zbar_image_get_userdata | ( | const zbar_image_t * | image ) |
return user specified data value associated with the image.
- Since:
- 0.5
unsigned zbar::zbar_image_get_width | ( | const zbar_image_t * | image ) |
retrieve the width of the image.
- Returns:
- the width in sample columns
zbar_image_t* zbar::zbar_image_read | ( | char * | filename ) |
read back an image in the format written by zbar_image_write()
- Note:
- TBD
void zbar::zbar_image_ref | ( | zbar_image_t * | image, |
int | refs | ||
) |
image reference count manipulation.
increment the reference count when you store a new reference to the image. decrement when the reference is no longer used. do not refer to the image any longer once the count is decremented. zbar_image_ref(image, -1) is the same as zbar_image_destroy(image)
- Since:
- 0.5
zbar_image_scanner_t* zbar::zbar_image_scanner_create | ( | void | ) |
constructor.
Definition at line 453 of file img_scanner.c.
void zbar::zbar_image_scanner_destroy | ( | zbar_image_scanner_t * | scanner ) |
destructor.
void zbar::zbar_image_scanner_enable_cache | ( | zbar_image_scanner_t * | scanner, |
int | enable | ||
) |
enable or disable the inter-image result cache (default disabled).
mostly useful for scanning video frames, the cache filters duplicate results from consecutive images, while adding some consistency checking and hysteresis to the results. this interface also clears the cache
const zbar_symbol_set_t* zbar::zbar_image_scanner_get_results | ( | const zbar_image_scanner_t * | scanner ) |
retrieve decode results for last scanned image.
- Returns:
- the symbol set result container or NULL if no results are available
- Note:
- the symbol set does not have its reference count adjusted; ensure that the count is incremented if the results may be kept after the next image is scanned
- Since:
- 0.10
static int zbar::zbar_image_scanner_parse_config | ( | zbar_image_scanner_t * | scanner, |
const char * | config_string | ||
) | [static] |
parse configuration string using zbar_parse_config() and apply to image scanner using zbar_image_scanner_set_config().
- Returns:
- 0 for success, non-0 for failure
- Since:
- 0.4
void zbar::zbar_image_scanner_recycle_image | ( | zbar_image_scanner_t * | scanner, |
zbar_image_t * | image | ||
) |
remove any previously decoded results from the image scanner and the specified image.
somewhat more efficient version of zbar_image_set_symbols(image, NULL) which may retain memory for subsequent decodes
- Since:
- 0.10
int zbar::zbar_image_scanner_set_config | ( | zbar_image_scanner_t * | scanner, |
zbar_symbol_type_t | symbology, | ||
zbar_config_t | config, | ||
int | value | ||
) |
set config for indicated symbology (0 for all) to specified value.
- Returns:
- 0 for success, non-0 for failure (config does not apply to specified symbology, or value out of range)
- See also:
- zbar_decoder_set_config()
- Since:
- 0.4
zbar_image_data_handler_t* zbar::zbar_image_scanner_set_data_handler | ( | zbar_image_scanner_t * | scanner, |
zbar_image_data_handler_t * | handler, | ||
const void * | userdata | ||
) |
setup result handler callback.
the specified function will be called by the scanner whenever new results are available from a decoded image. pass a NULL value to disable callbacks.
- Returns:
- the previously registered handler
void zbar::zbar_image_set_data | ( | zbar_image_t * | image, |
const void * | data, | ||
unsigned long | data_byte_length, | ||
zbar_image_cleanup_handler_t * | cleanup_hndlr | ||
) |
specify image sample data.
when image data is no longer needed by the library the specific data cleanup handler will be called (unless NULL)
- Note:
- application image data will not be modified by the library
void zbar::zbar_image_set_format | ( | zbar_image_t * | image, |
unsigned long | format | ||
) |
specify the fourcc image format code for image sample data.
refer to the documentation for supported formats.
- Note:
- this does not convert the data! (see zbar_image_convert() for that)
void zbar::zbar_image_set_sequence | ( | zbar_image_t * | image, |
unsigned | sequence_num | ||
) |
associate a "sequence" (page/frame) number with this image.
- Since:
- 0.6
void zbar::zbar_image_set_size | ( | zbar_image_t * | image, |
unsigned | width, | ||
unsigned | height | ||
) |
specify the pixel size of the image.
- Note:
- this does not affect the data!
void zbar::zbar_image_set_symbols | ( | zbar_image_t * | image, |
const zbar_symbol_set_t * | symbols | ||
) |
associate the specified symbol set with the image, replacing any existing results.
use NULL to release the current results from the image.
- See also:
- zbar_image_scanner_recycle_image()
- Since:
- 0.10
void zbar::zbar_image_set_userdata | ( | zbar_image_t * | image, |
void * | userdata | ||
) |
associate user specified data value with an image.
- Since:
- 0.5
int zbar::zbar_image_write | ( | const zbar_image_t * | image, |
const char * | filebase | ||
) |
dump raw image data to a file for debug.
the data will be prefixed with a 16 byte header consisting of:
- 4 bytes uint = 0x676d697a ("zimg")
- 4 bytes format fourcc
- 2 bytes width
- 2 bytes height
- 4 bytes size of following image data in bytes this header can be dumped w/eg: for some formats the image can be displayed/converted using ImageMagick, eg:
od -Ax -tx1z -N16 -w4 [file]
display -size 640x480+16 [-depth ?] [-sampling-factor ?x?] \ {GRAY,RGB,UYVY,YUV}:[file]
- Parameters:
-
image the image object to dump filebase base filename, appended with ".XXXX.zimg" where XXXX is the format fourcc
- Returns:
- 0 on success or a system error code on failure
void zbar::zbar_increase_verbosity | ( | void | ) |
int zbar::zbar_negotiate_format | ( | zbar_video_t * | video, |
zbar_window_t * | window | ||
) |
select a compatible format between video input and output window.
the selection algorithm attempts to use a format shared by video input and window output which is also most useful for barcode scanning. if a format conversion is necessary, it will heuristically attempt to minimize the cost of the conversion
int zbar::zbar_parse_config | ( | const char * | config_string, |
zbar_symbol_type_t * | symbology, | ||
zbar_config_t * | config, | ||
int * | value | ||
) |
parse a configuration string of the form "[symbology.]config[=value]".
the config must match one of the recognized names. the symbology, if present, must match one of the recognized names. if symbology is unspecified, it will be set to 0. if value is unspecified it will be set to 1.
- Returns:
- 0 if the config is parsed successfully, 1 otherwise
- Since:
- 0.4
int zbar::zbar_process_image | ( | zbar_processor_t * | processor, |
zbar_image_t * | image | ||
) |
process the provided image for barcodes.
if the library window is visible, the image will be displayed.
- Returns:
- >0 if symbols were successfully decoded, 0 if no symbols were found or -1 if an error occurs
int zbar::zbar_process_one | ( | zbar_processor_t * | processor, |
int | timeout | ||
) |
process from the video stream until a result is available, or the timeout (in milliseconds) expires.
specify a timeout of -1 to scan indefinitely (zbar_processor_set_active() may still be used to abort the scan from another thread). if the library window is visible, video display will be enabled.
- Note:
- that multiple results may still be returned (despite the name).
- Returns:
- >0 if symbols were successfully decoded, 0 if no symbols were found (ie, the timeout expired) or -1 if an error occurs
zbar_processor_t* zbar::zbar_processor_create | ( | int | threaded ) |
constructor.
if threaded is set and threading is available the processor will spawn threads where appropriate to avoid blocking and improve responsiveness
void zbar::zbar_processor_destroy | ( | zbar_processor_t * | processor ) |
destructor.
cleans up all resources associated with the processor
static int zbar::zbar_processor_error_spew | ( | const zbar_processor_t * | processor, |
int | verbosity | ||
) | [static] |
static const char* zbar::zbar_processor_error_string | ( | const zbar_processor_t * | processor, |
int | verbosity | ||
) | [static] |
int zbar::zbar_processor_force_format | ( | zbar_processor_t * | processor, |
unsigned long | input_format, | ||
unsigned long | output_format | ||
) |
force specific input and output formats for debug/testing.
- Note:
- must be called before zbar_processor_init()
static zbar_error_t zbar::zbar_processor_get_error_code | ( | const zbar_processor_t * | processor ) | [static] |
const zbar_symbol_set_t* zbar::zbar_processor_get_results | ( | const zbar_processor_t * | processor ) |
retrieve decode results for last scanned image/frame.
- Returns:
- the symbol set result container or NULL if no results are available
- Note:
- the returned symbol set has its reference count incremented; ensure that the count is decremented after use
- Since:
- 0.10
void* zbar::zbar_processor_get_userdata | ( | const zbar_processor_t * | processor ) |
return user specified data value associated with the processor.
- Since:
- 0.6
int zbar::zbar_processor_init | ( | zbar_processor_t * | processor, |
const char * | video_device, | ||
int | enable_display | ||
) |
(re)initialization.
opens a video input device and/or prepares to display output
int zbar::zbar_processor_is_visible | ( | zbar_processor_t * | processor ) |
retrieve the current state of the ouput window.
- Returns:
- 1 if the output window is currently displayed, 0 if not.
- -1 if an error occurs
static int zbar::zbar_processor_parse_config | ( | zbar_processor_t * | processor, |
const char * | config_string | ||
) | [static] |
parse configuration string using zbar_parse_config() and apply to processor using zbar_processor_set_config().
- Returns:
- 0 for success, non-0 for failure
- Since:
- 0.4
int zbar::zbar_processor_request_interface | ( | zbar_processor_t * | processor, |
int | version | ||
) |
request a preferred video driver interface version for debug/testing.
- Note:
- must be called before zbar_processor_init()
- Since:
- 0.6
int zbar::zbar_processor_request_iomode | ( | zbar_processor_t * | video, |
int | iomode | ||
) |
request a preferred video I/O mode for debug/testing.
You will get errors if the driver does not support the specified mode.
0 = auto-detect 1 = force I/O using read() 2 = force memory mapped I/O using mmap() 3 = force USERPTR I/O (v4l2 only)
- Note:
- must be called before zbar_processor_init()
- Since:
- 0.7
int zbar::zbar_processor_request_size | ( | zbar_processor_t * | processor, |
unsigned | width, | ||
unsigned | height | ||
) |
request a preferred size for the video image from the device.
the request may be adjusted or completely ignored by the driver.
- Note:
- must be called before zbar_processor_init()
- Since:
- 0.6
int zbar::zbar_processor_set_active | ( | zbar_processor_t * | processor, |
int | active | ||
) |
control the processor in free running video mode.
only works if video input is initialized. if threading is in use, scanning will occur in the background, otherwise this is only useful wrapping calls to zbar_processor_user_wait(). if the library output window is visible, video display will be enabled.
int zbar::zbar_processor_set_config | ( | zbar_processor_t * | processor, |
zbar_symbol_type_t | symbology, | ||
zbar_config_t | config, | ||
int | value | ||
) |
set config for indicated symbology (0 for all) to specified value.
- Returns:
- 0 for success, non-0 for failure (config does not apply to specified symbology, or value out of range)
- See also:
- zbar_decoder_set_config()
- Since:
- 0.4
zbar_image_data_handler_t* zbar::zbar_processor_set_data_handler | ( | zbar_processor_t * | processor, |
zbar_image_data_handler_t * | handler, | ||
const void * | userdata | ||
) |
setup result handler callback.
the specified function will be called by the processor whenever new results are available from the video stream or a static image. pass a NULL value to disable callbacks.
- Parameters:
-
processor the object on which to set the handler. handler the function to call when new results are available. userdata is set as with zbar_processor_set_userdata().
- Returns:
- the previously registered handler
void zbar::zbar_processor_set_userdata | ( | zbar_processor_t * | processor, |
void * | userdata | ||
) |
associate user specified data value with the processor.
- Since:
- 0.6
int zbar::zbar_processor_set_visible | ( | zbar_processor_t * | processor, |
int | visible | ||
) |
show or hide the display window owned by the library.
the size will be adjusted to the input size
int zbar::zbar_processor_user_wait | ( | zbar_processor_t * | processor, |
int | timeout | ||
) |
wait for input to the display window from the user (via mouse or keyboard).
- Returns:
- >0 when input is received, 0 if timeout ms expired with no input or -1 in case of an error
int zbar::zbar_scan_image | ( | zbar_image_scanner_t * | scanner, |
zbar_image_t * | image | ||
) |
scan for symbols in provided image.
The image format must be "Y800" or "GRAY".
- Returns:
- >0 if symbols were successfully decoded from the image, 0 if no symbols were found or -1 if an error occurs
- See also:
- zbar_image_convert()
- Since:
- 0.9 - changed to only accept grayscale images
static zbar_symbol_type_t zbar::zbar_scan_rgb24 | ( | zbar_scanner_t * | scanner, |
unsigned char * | rgb | ||
) | [static] |
zbar_symbol_type_t zbar::zbar_scan_y | ( | zbar_scanner_t * | scanner, |
int | y | ||
) |
process next sample intensity value.
intensity (y) is in arbitrary relative units.
- Returns:
- result of zbar_decode_width() if a decoder is attached, otherwise
- (ZBAR_PARTIAL) when new edge is detected or 0 (ZBAR_NONE) if no new edge is detected
zbar_scanner_t* zbar::zbar_scanner_create | ( | zbar_decoder_t * | decoder ) |
constructor.
if decoder is non-NULL it will be attached to scanner and called automatically at each new edge current color is initialized to ZBAR_SPACE (so an initial BAR->SPACE transition may be discarded)
void zbar::zbar_scanner_destroy | ( | zbar_scanner_t * | scanner ) |
destructor.
zbar_symbol_type_t zbar::zbar_scanner_flush | ( | zbar_scanner_t * | scanner ) |
flush scanner processing pipeline.
forces current scanner position to be a scan boundary. call multiple times (max 3) to completely flush decoder.
- Returns:
- any decode/scan results flushed from the pipeline
- Note:
- when not using callback handlers, the return value should be checked the same as zbar_scan_y()
- Since:
- 0.9
zbar_color_t zbar::zbar_scanner_get_color | ( | const zbar_scanner_t * | scanner ) |
retrieve last scanned color.
unsigned zbar::zbar_scanner_get_edge | ( | const zbar_scanner_t * | scn, |
unsigned | offset, | ||
int | prec | ||
) |
retrieve sample position of last edge.
- Since:
- 0.10
unsigned zbar::zbar_scanner_get_width | ( | const zbar_scanner_t * | scanner ) |
retrieve last scanned width.
zbar_symbol_type_t zbar::zbar_scanner_new_scan | ( | zbar_scanner_t * | scanner ) |
mark start of a new scan pass.
resets color to ZBAR_SPACE. also updates an associated decoder.
- Returns:
- any decode results flushed from the pipeline
- Note:
- when not using callback handlers, the return value should be checked the same as zbar_scan_y()
- call zbar_scanner_flush() at least twice before calling this method to ensure no decode results are lost
zbar_symbol_type_t zbar::zbar_scanner_reset | ( | zbar_scanner_t * | scanner ) |
clear all scanner state.
also resets an associated decoder
void zbar::zbar_set_verbosity | ( | int | verbosity ) |
const zbar_symbol_t* zbar::zbar_symbol_first_component | ( | const zbar_symbol_t * | symbol ) |
iterate components of a composite result.
- Returns:
- the first physical component symbol of a composite result
- NULL if the symbol is already a physical symbol
- Since:
- 0.10
const zbar_symbol_set_t* zbar::zbar_symbol_get_components | ( | const zbar_symbol_t * | symbol ) |
retrieve components of a composite result.
- Returns:
- the symbol set containing the components
- NULL if the symbol is already a physical symbol
- Since:
- 0.10
int zbar::zbar_symbol_get_count | ( | const zbar_symbol_t * | symbol ) |
retrieve current cache count.
when the cache is enabled for the image_scanner this provides inter-frame reliability and redundancy information for video streams.
- Returns:
- < 0 if symbol is still uncertain.
- 0 if symbol is newly verified.
- > 0 for duplicate symbols
const char* zbar::zbar_symbol_get_data | ( | const zbar_symbol_t * | symbol ) |
retrieve data decoded from symbol.
- Returns:
- the data string
unsigned int zbar::zbar_symbol_get_data_length | ( | const zbar_symbol_t * | symbol ) |
retrieve length of binary data.
- Returns:
- the length of the decoded data
unsigned zbar::zbar_symbol_get_loc_size | ( | const zbar_symbol_t * | symbol ) |
retrieve the number of points in the location polygon.
the location polygon defines the image area that the symbol was extracted from.
- Returns:
- the number of points in the location polygon
- Note:
- this is currently not a polygon, but the scan locations where the symbol was decoded
int zbar::zbar_symbol_get_loc_x | ( | const zbar_symbol_t * | symbol, |
unsigned | index | ||
) |
retrieve location polygon x-coordinates.
points are specified by 0-based index.
- Returns:
- the x-coordinate for a point in the location polygon.
- -1 if index is out of range
int zbar::zbar_symbol_get_loc_y | ( | const zbar_symbol_t * | symbol, |
unsigned | index | ||
) |
retrieve location polygon y-coordinates.
points are specified by 0-based index.
- Returns:
- the y-coordinate for a point in the location polygon.
- -1 if index is out of range
int zbar::zbar_symbol_get_quality | ( | const zbar_symbol_t * | symbol ) |
retrieve a symbol confidence metric.
- Returns:
- an unscaled, relative quantity: larger values are better than smaller values, where "large" and "small" are application dependent.
- Note:
- expect the exact definition of this quantity to change as the metric is refined. currently, only the ordered relationship between two values is defined and will remain stable in the future
- Since:
- 0.9
zbar_symbol_type_t zbar::zbar_symbol_get_type | ( | const zbar_symbol_t * | symbol ) |
retrieve type of decoded symbol.
- Returns:
- the symbol type
const zbar_symbol_t* zbar::zbar_symbol_next | ( | const zbar_symbol_t * | symbol ) |
iterate the set to which this symbol belongs (there can be only one).
- Returns:
- the next symbol in the set, or
- NULL when no more results are available
void zbar::zbar_symbol_ref | ( | const zbar_symbol_t * | symbol, |
int | refs | ||
) |
symbol reference count manipulation.
increment the reference count when you store a new reference to the symbol. decrement when the reference is no longer used. do not refer to the symbol once the count is decremented and the containing image has been recycled or destroyed.
- Note:
- the containing image holds a reference to the symbol, so you only need to use this if you keep a symbol after the image has been destroyed or reused.
- Since:
- 0.9
const zbar_symbol_t* zbar::zbar_symbol_set_first_symbol | ( | const zbar_symbol_set_t * | symbols ) |
set iterator.
- Returns:
- the first decoded symbol result in a set
- NULL if the set is empty
- Since:
- 0.10
int zbar::zbar_symbol_set_get_size | ( | const zbar_symbol_set_t * | symbols ) |
retrieve set size.
- Returns:
- the number of symbols in the set.
- Since:
- 0.10
void zbar::zbar_symbol_set_ref | ( | const zbar_symbol_set_t * | symbols, |
int | refs | ||
) |
reference count manipulation.
increment the reference count when you store a new reference. decrement when the reference is no longer used. do not refer to the object any longer once references have been released.
- Since:
- 0.10
char* zbar::zbar_symbol_xml | ( | const zbar_symbol_t * | symbol, |
char ** | buffer, | ||
unsigned * | buflen | ||
) |
print XML symbol element representation to user result buffer.
- See also:
- http://zbar.sourceforge.net/2008/barcode.xsd for the schema.
- Parameters:
-
symbol is the symbol to print buffer is the inout result pointer, it will be reallocated with a larger size if necessary. buflen is inout length of the result buffer.
- Returns:
- the buffer pointer
- Since:
- 0.6
int zbar::zbar_version | ( | unsigned * | major, |
unsigned * | minor | ||
) |
zbar_video_t* zbar::zbar_video_create | ( | void | ) |
constructor.
void zbar::zbar_video_destroy | ( | zbar_video_t * | video ) |
destructor.
int zbar::zbar_video_enable | ( | zbar_video_t * | video, |
int | enable | ||
) |
start/stop video capture.
all buffered images are retired when capture is disabled.
- Returns:
- 0 if successful or -1 if an error occurs
static int zbar::zbar_video_error_spew | ( | const zbar_video_t * | video, |
int | verbosity | ||
) | [static] |
static const char* zbar::zbar_video_error_string | ( | const zbar_video_t * | video, |
int | verbosity | ||
) | [static] |
static zbar_error_t zbar::zbar_video_get_error_code | ( | const zbar_video_t * | video ) | [static] |
int zbar::zbar_video_get_fd | ( | const zbar_video_t * | video ) |
retrieve file descriptor associated with open *nix video device useful for using select()/poll() to tell when new images are available (NB v4l2 only!!).
- Returns:
- the file descriptor or -1 if the video device is not open or the driver only supports v4l1
int zbar::zbar_video_get_height | ( | const zbar_video_t * | video ) |
retrieve current output image height.
- Returns:
- the height or 0 if the video device is not open
int zbar::zbar_video_get_width | ( | const zbar_video_t * | video ) |
retrieve current output image width.
- Returns:
- the width or 0 if the video device is not open
int zbar::zbar_video_init | ( | zbar_video_t * | video, |
unsigned long | format | ||
) |
initialize video using a specific format for debug.
use zbar_negotiate_format() to automatically select and initialize the best available format
zbar_image_t* zbar::zbar_video_next_image | ( | zbar_video_t * | video ) |
retrieve next captured image.
blocks until an image is available.
- Returns:
- NULL if video is not enabled or an error occurs
int zbar::zbar_video_open | ( | zbar_video_t * | video, |
const char * | device | ||
) |
open and probe a video device.
the device specified by platform specific unique name (v4l device node path in *nix eg "/dev/video", DirectShow DevicePath property in windows).
- Returns:
- 0 if successful or -1 if an error occurs
int zbar::zbar_video_request_interface | ( | zbar_video_t * | video, |
int | version | ||
) |
request a preferred driver interface version for debug/testing.
- Note:
- must be called before zbar_video_open()
- Since:
- 0.6
int zbar::zbar_video_request_iomode | ( | zbar_video_t * | video, |
int | iomode | ||
) |
request a preferred I/O mode for debug/testing.
You will get errors if the driver does not support the specified mode.
0 = auto-detect 1 = force I/O using read() 2 = force memory mapped I/O using mmap() 3 = force USERPTR I/O (v4l2 only)
- Note:
- must be called before zbar_video_open()
- Since:
- 0.7
int zbar::zbar_video_request_size | ( | zbar_video_t * | video, |
unsigned | width, | ||
unsigned | height | ||
) |
request a preferred size for the video image from the device.
the request may be adjusted or completely ignored by the driver.
- Returns:
- 0 if successful or -1 if the video device is already initialized
- Since:
- 0.6
int zbar::zbar_window_attach | ( | zbar_window_t * | window, |
void * | x11_display_w32_hwnd, | ||
unsigned long | x11_drawable | ||
) |
associate reader with an existing platform window.
This can be any "Drawable" for X Windows or a "HWND" for windows. input images will be scaled into the output window. pass NULL to detach from the resource, further input will be ignored
zbar_window_t* zbar::zbar_window_create | ( | void | ) |
constructor.
void zbar::zbar_window_destroy | ( | zbar_window_t * | window ) |
destructor.
int zbar::zbar_window_draw | ( | zbar_window_t * | window, |
zbar_image_t * | image | ||
) |
draw a new image into the output window.
static int zbar::zbar_window_error_spew | ( | const zbar_window_t * | window, |
int | verbosity | ||
) | [static] |
static const char* zbar::zbar_window_error_string | ( | const zbar_window_t * | window, |
int | verbosity | ||
) | [static] |
static zbar_error_t zbar::zbar_window_get_error_code | ( | const zbar_window_t * | window ) | [static] |
int zbar::zbar_window_get_overlay | ( | const zbar_window_t * | window ) |
int zbar::zbar_window_redraw | ( | zbar_window_t * | window ) |
redraw the last image (exposure handler).
int zbar::zbar_window_resize | ( | zbar_window_t * | window, |
unsigned | width, | ||
unsigned | height | ||
) |
resize the image window (reconfigure handler).
this does not update the contents of the window
- Since:
- 0.3, changed in 0.4 to not redraw window
void zbar::zbar_window_set_overlay | ( | zbar_window_t * | window, |
int | level | ||
) |
control content level of the reader overlay.
the overlay displays graphical data for informational or debug purposes. higher values increase the level of annotation (possibly decreasing performance).
0 = disable overlay 1 = outline decoded symbols (default) 2 = also track and display input frame rate
Generated on Tue Jul 12 2022 18:54:12 by
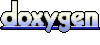