ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
window.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _WINDOW_H_ 00024 #define _WINDOW_H_ 00025 00026 #include <config.h> 00027 #ifdef HAVE_INTTYPES_H 00028 # include <inttypes.h> 00029 #endif 00030 #include <stdlib.h> 00031 00032 #include <zbar.h> 00033 #include "symbol.h" 00034 #include "error.h" 00035 #include "mutex.h" 00036 00037 typedef struct window_state_s window_state_t; 00038 00039 struct zbar_window_s { 00040 errinfo_t err; /* error reporting */ 00041 zbar_image_t *image; /* last displayed image 00042 * NB image access must be locked! 00043 */ 00044 unsigned overlay; /* user set overlay level */ 00045 00046 uint32_t format; /* output format */ 00047 unsigned width, height; /* current output size */ 00048 unsigned max_width, max_height; 00049 00050 uint32_t src_format; /* current input format */ 00051 unsigned src_width; /* last displayed image size */ 00052 unsigned src_height; 00053 00054 unsigned dst_width; /* conversion target */ 00055 unsigned dst_height; 00056 00057 unsigned scale_num; /* output scaling */ 00058 unsigned scale_den; 00059 00060 point_t scaled_offset; /* output position and size */ 00061 point_t scaled_size; 00062 00063 uint32_t *formats; /* supported formats (zero terminated) */ 00064 00065 zbar_mutex_t imglock; /* lock displayed image */ 00066 00067 void *display; 00068 unsigned long xwin; 00069 unsigned long time; /* last image display in milliseconds */ 00070 unsigned long time_avg; /* average of inter-frame times */ 00071 00072 window_state_t *state; /* platform/interface specific state */ 00073 00074 /* interface dependent methods */ 00075 int (*init)(zbar_window_t*, zbar_image_t*, int); 00076 int (*draw_image)(zbar_window_t*, zbar_image_t*); 00077 int (*cleanup)(zbar_window_t*); 00078 }; 00079 00080 /* window.draw has to be thread safe wrt/other apis 00081 * FIXME should be a semaphore 00082 */ 00083 static inline int window_lock (zbar_window_t *w) 00084 { 00085 int rc = 0; 00086 if((rc = _zbar_mutex_lock(&w->imglock))) { 00087 err_capture(w, SEV_FATAL, ZBAR_ERR_LOCKING, __func__, 00088 "unable to acquire lock"); 00089 w->err.errnum = rc; 00090 return(-1); 00091 } 00092 return(0); 00093 } 00094 00095 static inline int window_unlock (zbar_window_t *w) 00096 { 00097 int rc = 0; 00098 if((rc = _zbar_mutex_unlock(&w->imglock))) { 00099 err_capture(w, SEV_FATAL, ZBAR_ERR_LOCKING, __func__, 00100 "unable to release lock"); 00101 w->err.errnum = rc; 00102 return(-1); 00103 } 00104 return(0); 00105 } 00106 00107 static inline int _zbar_window_add_format (zbar_window_t *w, 00108 uint32_t fmt) 00109 { 00110 int i; 00111 for(i = 0; w->formats && w->formats[i]; i++) 00112 if(w->formats[i] == fmt) 00113 return(i); 00114 00115 w->formats = realloc(w->formats, (i + 2) * sizeof(uint32_t)); 00116 w->formats[i] = fmt; 00117 w->formats[i + 1] = 0; 00118 return(i); 00119 } 00120 00121 static inline point_t window_scale_pt (zbar_window_t *w, 00122 point_t p) 00123 { 00124 p.x = ((long)p.x * w->scale_num + w->scale_den - 1) / w->scale_den; 00125 p.y = ((long)p.y * w->scale_num + w->scale_den - 1) / w->scale_den; 00126 return(p); 00127 } 00128 00129 00130 /* PAL interface */ 00131 extern int _zbar_window_attach(zbar_window_t*, void*, unsigned long); 00132 extern int _zbar_window_expose(zbar_window_t*, int, int, int, int); 00133 extern int _zbar_window_resize(zbar_window_t*); 00134 extern int _zbar_window_clear(zbar_window_t*); 00135 extern int _zbar_window_begin(zbar_window_t*); 00136 extern int _zbar_window_end(zbar_window_t*); 00137 extern int _zbar_window_draw_marker(zbar_window_t*, uint32_t, point_t); 00138 extern int _zbar_window_draw_polygon(zbar_window_t*, uint32_t, const point_t*, int); 00139 extern int _zbar_window_draw_text(zbar_window_t*, uint32_t, 00140 point_t, const char*); 00141 extern int _zbar_window_fill_rect(zbar_window_t*, uint32_t, point_t, point_t); 00142 extern int _zbar_window_draw_logo(zbar_window_t*); 00143 00144 #endif 00145
Generated on Tue Jul 12 2022 18:54:12 by
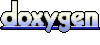