ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
symbol.c
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 00024 #include <config.h> 00025 #include <stdio.h> 00026 #include <string.h> 00027 #include <assert.h> 00028 00029 #include <zbar.h> 00030 #include "symbol.h" 00031 00032 const char *zbar_get_symbol_name (zbar_symbol_type_t sym) 00033 { 00034 switch(sym & ZBAR_SYMBOL) { 00035 case ZBAR_EAN8: return("EAN-8"); 00036 case ZBAR_UPCE: return("UPC-E"); 00037 case ZBAR_ISBN10: return("ISBN-10"); 00038 case ZBAR_UPCA: return("UPC-A"); 00039 case ZBAR_EAN13: return("EAN-13"); 00040 case ZBAR_ISBN13: return("ISBN-13"); 00041 case ZBAR_I25: return("I2/5"); 00042 case ZBAR_CODE39: return("CODE-39"); 00043 case ZBAR_CODE128: return("CODE-128"); 00044 case ZBAR_PDF417: return("PDF417"); 00045 case ZBAR_QRCODE: return("QR-Code"); 00046 default: return("UNKNOWN"); 00047 } 00048 } 00049 00050 const char *zbar_get_addon_name (zbar_symbol_type_t sym) 00051 { 00052 switch(sym & ZBAR_ADDON) { 00053 case ZBAR_ADDON2: return("+2"); 00054 case ZBAR_ADDON5: return("+5"); 00055 default: return(""); 00056 } 00057 } 00058 00059 00060 void _zbar_symbol_free (zbar_symbol_t *sym) 00061 { 00062 if(sym->syms) { 00063 zbar_symbol_set_ref(sym->syms, -1); 00064 sym->syms = NULL; 00065 } 00066 if(sym->pts) 00067 free(sym->pts); 00068 if(sym->data_alloc && sym->data) 00069 free(sym->data); 00070 free(sym); 00071 } 00072 00073 void zbar_symbol_ref (const zbar_symbol_t *sym, 00074 int refs) 00075 { 00076 zbar_symbol_t *ncsym = (zbar_symbol_t*)sym; 00077 _zbar_symbol_refcnt(ncsym, refs); 00078 } 00079 00080 zbar_symbol_type_t zbar_symbol_get_type (const zbar_symbol_t *sym) 00081 { 00082 return(sym->type); 00083 } 00084 00085 const char *zbar_symbol_get_data (const zbar_symbol_t *sym) 00086 { 00087 return(sym->data); 00088 } 00089 00090 unsigned int zbar_symbol_get_data_length (const zbar_symbol_t *sym) 00091 { 00092 return(sym->datalen); 00093 } 00094 00095 int zbar_symbol_get_count (const zbar_symbol_t *sym) 00096 { 00097 return(sym->cache_count); 00098 } 00099 00100 int zbar_symbol_get_quality (const zbar_symbol_t *sym) 00101 { 00102 return(sym->quality); 00103 } 00104 00105 unsigned zbar_symbol_get_loc_size (const zbar_symbol_t *sym) 00106 { 00107 return(sym->npts); 00108 } 00109 00110 int zbar_symbol_get_loc_x (const zbar_symbol_t *sym, 00111 unsigned idx) 00112 { 00113 if(idx < sym->npts) 00114 return(sym->pts[idx].x); 00115 else 00116 return(-1); 00117 } 00118 00119 int zbar_symbol_get_loc_y (const zbar_symbol_t *sym, 00120 unsigned idx) 00121 { 00122 if(idx < sym->npts) 00123 return(sym->pts[idx].y); 00124 else 00125 return(-1); 00126 } 00127 00128 const zbar_symbol_t *zbar_symbol_next (const zbar_symbol_t *sym) 00129 { 00130 return((sym) ? sym->next : NULL); 00131 } 00132 00133 const zbar_symbol_set_t* 00134 zbar_symbol_get_components (const zbar_symbol_t *sym) 00135 { 00136 return(sym->syms); 00137 } 00138 00139 const zbar_symbol_t *zbar_symbol_first_component (const zbar_symbol_t *sym) 00140 { 00141 return((sym && sym->syms) ? sym->syms->head : NULL); 00142 } 00143 00144 00145 static const char *xmlfmt[] = { 00146 "<symbol type='%s' quality='%d'", 00147 " count='%d'", 00148 "><data><![CDATA[", 00149 "]]></data></symbol>", 00150 }; 00151 00152 /* FIXME suspect... */ 00153 #define MAX_INT_DIGITS 10 00154 00155 char *zbar_symbol_xml (const zbar_symbol_t *sym, 00156 char **buf, 00157 unsigned *len) 00158 { 00159 const char *type = zbar_get_symbol_name(sym->type); 00160 /* FIXME binary data */ 00161 unsigned datalen = strlen(sym->data); 00162 unsigned maxlen = (strlen(xmlfmt[0]) + strlen(xmlfmt[1]) + 00163 strlen(xmlfmt[2]) + strlen(xmlfmt[3]) + 00164 strlen(type) + datalen + MAX_INT_DIGITS + 1); 00165 if(!*buf || (*len < maxlen)) { 00166 if(*buf) 00167 free(*buf); 00168 *buf = malloc(maxlen); 00169 /* FIXME check OOM */ 00170 *len = maxlen; 00171 } 00172 00173 int n = snprintf(*buf, maxlen, xmlfmt[0], type, sym->quality); 00174 assert(n > 0); 00175 assert(n <= maxlen); 00176 00177 if(sym->cache_count) { 00178 int i = snprintf(*buf + n, maxlen - n, xmlfmt[1], sym->cache_count); 00179 assert(i > 0); 00180 n += i; 00181 assert(n <= maxlen); 00182 } 00183 00184 int i = strlen(xmlfmt[2]); 00185 memcpy(*buf + n, xmlfmt[2], i + 1); 00186 n += i; 00187 assert(n <= maxlen); 00188 00189 /* FIXME binary data */ 00190 /* FIXME handle "]]>" */ 00191 strncpy(*buf + n, sym->data, datalen + 1); 00192 n += datalen; 00193 assert(n <= maxlen); 00194 00195 i = strlen(xmlfmt[3]); 00196 memcpy(*buf + n, xmlfmt[3], i + 1); 00197 n += i; 00198 assert(n <= maxlen); 00199 00200 *len = n; 00201 return(*buf); 00202 } 00203 00204 00205 zbar_symbol_set_t *_zbar_symbol_set_create () 00206 { 00207 zbar_symbol_set_t *syms = calloc(1, sizeof(*syms)); 00208 _zbar_refcnt(&syms->refcnt, 1); 00209 return(syms); 00210 } 00211 00212 inline void _zbar_symbol_set_free (zbar_symbol_set_t *syms) 00213 { 00214 zbar_symbol_t *sym, *next; 00215 for(sym = syms->head; sym; sym = next) { 00216 next = sym->next; 00217 sym->next = NULL; 00218 _zbar_symbol_refcnt(sym, -1); 00219 } 00220 syms->head = NULL; 00221 free(syms); 00222 } 00223 00224 void zbar_symbol_set_ref (const zbar_symbol_set_t *syms, 00225 int delta) 00226 { 00227 zbar_symbol_set_t *ncsyms = (zbar_symbol_set_t*)syms; 00228 if(!_zbar_refcnt(&ncsyms->refcnt, delta) && delta <= 0) 00229 _zbar_symbol_set_free(ncsyms); 00230 } 00231 00232 int zbar_symbol_set_get_size (const zbar_symbol_set_t *syms) 00233 { 00234 return(syms->nsyms); 00235 } 00236 00237 const zbar_symbol_t* 00238 zbar_symbol_set_first_symbol (const zbar_symbol_set_t *syms) 00239 { 00240 zbar_symbol_t *sym = syms->tail; 00241 if(sym) 00242 return(sym->next); 00243 return(syms->head); 00244 } 00245
Generated on Tue Jul 12 2022 18:54:12 by
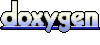