ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
scan_image.c
00001 #include <stdio.h> 00002 #include <stdlib.h> 00003 #if (0) // png is not used 00004 #include <png.h> 00005 #endif 00006 #include <zbar.h> 00007 00008 zbar_image_scanner_t *scanner = NULL; 00009 00010 /* to complete a runnable example, this abbreviated implementation of 00011 * get_data() will use libpng to read an image file. refer to libpng 00012 * documentation for details 00013 */ 00014 #if (0) // png is not used 00015 static void get_data (const char *name, 00016 int *width, int *height, 00017 void **raw) 00018 { 00019 FILE *file = fopen(name, "rb"); 00020 if(!file) exit(2); 00021 png_structp png = 00022 png_create_read_struct(PNG_LIBPNG_VER_STRING, 00023 NULL, NULL, NULL); 00024 if(!png) exit(3); 00025 if(setjmp(png_jmpbuf(png))) exit(4); 00026 png_infop info = png_create_info_struct(png); 00027 if(!info) exit(5); 00028 png_init_io(png, file); 00029 png_read_info(png, info); 00030 /* configure for 8bpp grayscale input */ 00031 int color = png_get_color_type(png, info); 00032 int bits = png_get_bit_depth(png, info); 00033 if(color & PNG_COLOR_TYPE_PALETTE) 00034 png_set_palette_to_rgb(png); 00035 if(color == PNG_COLOR_TYPE_GRAY && bits < 8) 00036 png_set_gray_1_2_4_to_8(png); 00037 if(bits == 16) 00038 png_set_strip_16(png); 00039 if(color & PNG_COLOR_MASK_ALPHA) 00040 png_set_strip_alpha(png); 00041 if(color & PNG_COLOR_MASK_COLOR) 00042 png_set_rgb_to_gray_fixed(png, 1, -1, -1); 00043 /* allocate image */ 00044 *width = png_get_image_width(png, info); 00045 *height = png_get_image_height(png, info); 00046 *raw = malloc(*width * *height); 00047 png_bytep rows[*height]; 00048 int i; 00049 for(i = 0; i < *height; i++) 00050 rows[i] = *raw + (*width * i); 00051 png_read_image(png, rows); 00052 } 00053 #endif // png is not used 00054 #if (1) // function name and arguments are changed. 00055 int zbar_main (void* image_buff, int width, int height) 00056 #else 00057 int main (int argc, char **argv) 00058 #endif 00059 { 00060 #if (1) // arguments are changed 00061 if((image_buff == NULL)||(width < 1)||(height < 1)) return(1); 00062 #else 00063 if(argc < 2) return(1); 00064 #endif 00065 00066 /* create a reader */ 00067 scanner = zbar_image_scanner_create(); 00068 00069 /* configure the reader */ 00070 zbar_image_scanner_set_config(scanner, 0, ZBAR_CFG_ENABLE, 1); 00071 00072 /* obtain image data */ 00073 #if (1) // width, height, raw data are fixed 00074 void *raw = image_buff; 00075 #else 00076 int width = 0, height = 0; 00077 void *raw = NULL; 00078 get_data(argv[1], &width, &height, &raw); 00079 #endif 00080 00081 /* wrap image data */ 00082 zbar_image_t *image = zbar_image_create(); 00083 zbar_image_set_format(image, *(int*)"Y800"); 00084 zbar_image_set_size(image, width, height); 00085 zbar_image_set_data(image, raw, width * height, zbar_image_free_data); 00086 00087 /* scan the image for barcodes */ 00088 int n = zbar_scan_image(scanner, image); 00089 00090 /* extract results */ 00091 const zbar_symbol_t *symbol = zbar_image_first_symbol(image); 00092 if ( symbol ) { 00093 for(; symbol; symbol = zbar_symbol_next(symbol)) { 00094 /* do something useful with results */ 00095 zbar_symbol_type_t typ = zbar_symbol_get_type(symbol); 00096 const char *data = zbar_symbol_get_data(symbol); 00097 printf("decoded %s symbol \"%s\"\n", 00098 zbar_get_symbol_name(typ), data); 00099 } 00100 } 00101 else { 00102 printf("No Code detected.\n"); 00103 } 00104 00105 /* clean up */ 00106 zbar_image_destroy(image); 00107 zbar_image_scanner_destroy(scanner); 00108 00109 return(0); 00110 } 00111
Generated on Tue Jul 12 2022 18:54:12 by
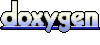