ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
qrcode.h
00001 /*Copyright (C) 2008-2009 Timothy B. Terriberry (tterribe@xiph.org) 00002 You can redistribute this library and/or modify it under the terms of the 00003 GNU Lesser General Public License as published by the Free Software 00004 Foundation; either version 2.1 of the License, or (at your option) any later 00005 version.*/ 00006 #ifndef _QRCODE_H_ 00007 #define _QRCODE_H_ 00008 00009 #include <zbar.h> 00010 00011 typedef struct qr_reader qr_reader; 00012 00013 typedef int qr_point[2]; 00014 typedef struct qr_finder_line qr_finder_line; 00015 00016 /*The number of bits of subpel precision to store image coordinates in. 00017 This helps when estimating positions in low-resolution images, which may have 00018 a module pitch only a pixel or two wide, making rounding errors matter a 00019 great deal.*/ 00020 #define QR_FINDER_SUBPREC (2) 00021 00022 /*A line crossing a finder pattern. 00023 Whether the line is horizontal or vertical is determined by context. 00024 The offsts to various parts of the finder pattern are as follows: 00025 |*****| |*****|*****|*****| |*****| 00026 |*****| |*****|*****|*****| |*****| 00027 ^ ^ ^ ^ 00028 | | | | 00029 | | | pos[v]+len+eoffs 00030 | | pos[v]+len 00031 | pos[v] 00032 pos[v]-boffs 00033 Here v is 0 for horizontal and 1 for vertical lines.*/ 00034 struct qr_finder_line { 00035 /*The location of the upper/left endpoint of the line. 00036 The left/upper edge of the center section is used, since other lines must 00037 cross in this region.*/ 00038 qr_point pos; 00039 /*The length of the center section. 00040 This extends to the right/bottom of the center section, since other lines 00041 must cross in this region.*/ 00042 int len; 00043 /*The offset to the midpoint of the upper/left section (part of the outside 00044 ring), or 0 if we couldn't identify the edge of the beginning section. 00045 We use the midpoint instead of the edge because it can be located more 00046 reliably.*/ 00047 int boffs; 00048 /*The offset to the midpoint of the end section (part of the outside ring), 00049 or 0 if we couldn't identify the edge of the end section. 00050 We use the midpoint instead of the edge because it can be located more 00051 reliably.*/ 00052 int eoffs; 00053 }; 00054 00055 qr_reader *_zbar_qr_create(void); 00056 void _zbar_qr_destroy(qr_reader *reader); 00057 void _zbar_qr_reset(qr_reader *reader); 00058 00059 int _zbar_qr_found_line(qr_reader *reader, 00060 int direction, 00061 const qr_finder_line *line); 00062 int _zbar_qr_decode(qr_reader *reader, 00063 zbar_image_scanner_t *iscn, 00064 zbar_image_t *img); 00065 00066 #endif 00067
Generated on Tue Jul 12 2022 18:54:12 by
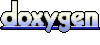