ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
image.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _IMAGE_H_ 00024 #define _IMAGE_H_ 00025 00026 #include <config.h> 00027 #ifdef HAVE_INTTYPES_H 00028 # include <inttypes.h> 00029 #endif 00030 #include <stdlib.h> 00031 #include <assert.h> 00032 00033 #include <zbar.h> 00034 #include "error.h" 00035 #include "symbol.h" 00036 #include "refcnt.h" 00037 00038 /* adapted from v4l2 spec */ 00039 #define fourcc(a, b, c, d) \ 00040 ((uint32_t)(a) | ((uint32_t)(b) << 8) | \ 00041 ((uint32_t)(c) << 16) | ((uint32_t)(d) << 24)) 00042 00043 /* unpack size/location of component */ 00044 #define RGB_SIZE(c) ((c) >> 5) 00045 #define RGB_OFFSET(c) ((c) & 0x1f) 00046 00047 /* coarse image format categorization. 00048 * to limit conversion variations 00049 */ 00050 typedef enum zbar_format_group_e { 00051 ZBAR_FMT_GRAY, 00052 ZBAR_FMT_YUV_PLANAR, 00053 ZBAR_FMT_YUV_PACKED, 00054 ZBAR_FMT_RGB_PACKED, 00055 ZBAR_FMT_YUV_NV, 00056 ZBAR_FMT_JPEG, 00057 00058 /* enum size */ 00059 ZBAR_FMT_NUM 00060 } zbar_format_group_t; 00061 00062 00063 struct zbar_image_s { 00064 uint32_t format; /* fourcc image format code */ 00065 unsigned width, height; /* image size */ 00066 const void *data; /* image sample data */ 00067 unsigned long datalen; /* allocated/mapped size of data */ 00068 void *userdata; /* user specified data associated w/image */ 00069 00070 /* cleanup handler */ 00071 zbar_image_cleanup_handler_t *cleanup; 00072 refcnt_t refcnt; /* reference count */ 00073 zbar_video_t *src; /* originator */ 00074 int srcidx; /* index used by originator */ 00075 zbar_image_t *next; /* internal image lists */ 00076 00077 unsigned seq; /* page/frame sequence number */ 00078 zbar_symbol_set_t *syms; /* decoded result set */ 00079 }; 00080 00081 /* description of an image format */ 00082 typedef struct zbar_format_def_s { 00083 uint32_t format; /* fourcc */ 00084 zbar_format_group_t group; /* coarse categorization */ 00085 union { 00086 uint8_t gen[4]; /* raw bytes */ 00087 struct { 00088 uint8_t bpp; /* bits per pixel */ 00089 uint8_t red, green, blue; /* size/location a la RGB_BITS() */ 00090 } rgb; 00091 struct { 00092 uint8_t xsub2, ysub2; /* chroma subsampling in each axis */ 00093 uint8_t packorder; /* channel ordering flags 00094 * bit0: 0=UV, 1=VU 00095 * bit1: 0=Y/chroma, 1=chroma/Y 00096 */ 00097 } yuv; 00098 uint32_t cmp; /* quick compare equivalent formats */ 00099 } p; 00100 } zbar_format_def_t; 00101 00102 00103 extern int _zbar_best_format(uint32_t, uint32_t*, const uint32_t*); 00104 extern const zbar_format_def_t *_zbar_format_lookup(uint32_t); 00105 extern void _zbar_image_free(zbar_image_t*); 00106 00107 #ifdef DEBUG_SVG 00108 extern int zbar_image_write_png(const zbar_image_t*, const char*); 00109 #else 00110 # define zbar_image_write_png(...) 00111 #endif 00112 00113 static inline void _zbar_image_refcnt (zbar_image_t *img, 00114 int delta) 00115 { 00116 if(!_zbar_refcnt(&img->refcnt, delta) && delta <= 0) { 00117 if(img->cleanup) 00118 img->cleanup(img); 00119 if(!img->src) 00120 _zbar_image_free(img); 00121 } 00122 } 00123 00124 static inline void _zbar_image_swap_symbols (zbar_image_t *a, 00125 zbar_image_t *b) 00126 { 00127 zbar_symbol_set_t *tmp = a->syms; 00128 a->syms = b->syms; 00129 b->syms = tmp; 00130 } 00131 00132 #endif 00133
Generated on Tue Jul 12 2022 18:54:12 by
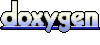