ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
error.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _ERROR_H_ 00024 #define _ERROR_H_ 00025 00026 #include <config.h> 00027 #ifdef HAVE_INTTYPES_H 00028 # include <inttypes.h> 00029 #endif 00030 #include <stdlib.h> 00031 #include <stdio.h> 00032 #include <string.h> 00033 #include <errno.h> 00034 #include <assert.h> 00035 00036 #include <zbar.h> 00037 00038 #ifdef _WIN32 00039 # include <windows.h> 00040 #endif 00041 00042 #if __STDC_VERSION__ < 199901L 00043 # if __GNUC__ >= 2 00044 # define __func__ __FUNCTION__ 00045 # else 00046 # define __func__ "<unknown>" 00047 # endif 00048 #endif 00049 00050 #define ERRINFO_MAGIC (0x5252457a) /* "zERR" (LE) */ 00051 00052 typedef enum errsev_e { 00053 SEV_FATAL = -2, /* application must terminate */ 00054 SEV_ERROR = -1, /* might be able to recover and continue */ 00055 SEV_OK = 0, 00056 SEV_WARNING = 1, /* unexpected condition */ 00057 SEV_NOTE = 2, /* fyi */ 00058 } errsev_t; 00059 00060 typedef enum errmodule_e { 00061 ZBAR_MOD_PROCESSOR, 00062 ZBAR_MOD_VIDEO, 00063 ZBAR_MOD_WINDOW, 00064 ZBAR_MOD_IMAGE_SCANNER, 00065 ZBAR_MOD_UNKNOWN, 00066 } errmodule_t; 00067 00068 typedef struct errinfo_s { 00069 uint32_t magic; /* just in case */ 00070 errmodule_t module; /* reporting module */ 00071 char *buf; /* formatted and passed to application */ 00072 int errnum; /* errno for system errors */ 00073 00074 errsev_t sev; 00075 zbar_error_t type; 00076 const char *func; /* reporting function */ 00077 const char *detail; /* description */ 00078 char *arg_str; /* single string argument */ 00079 int arg_int; /* single integer argument */ 00080 } errinfo_t; 00081 00082 extern int _zbar_verbosity; 00083 00084 /* FIXME don't we need varargs hacks here? */ 00085 00086 #ifdef _WIN32 00087 # define ZFLUSH fflush(stderr); 00088 #else 00089 # define ZFLUSH 00090 #endif 00091 00092 #ifdef ZNO_MESSAGES 00093 00094 # ifdef __GNUC__ 00095 /* older versions of gcc (< 2.95) require a named varargs parameter */ 00096 # define zprintf(args...) 00097 # else 00098 /* unfortunately named vararg parameter is a gcc-specific extension */ 00099 # define zprintf(...) 00100 # endif 00101 00102 #else 00103 00104 # ifdef __GNUC__ 00105 # define zprintf(level, format, args...) do { \ 00106 if(_zbar_verbosity >= level) { \ 00107 fprintf(stderr, "%s: " format, __func__ , ##args); \ 00108 ZFLUSH \ 00109 } \ 00110 } while(0) 00111 # else 00112 # define zprintf(level, format, ...) do { \ 00113 if(_zbar_verbosity >= level) { \ 00114 fprintf(stderr, "%s: " format, __func__ , ##__VA_ARGS__); \ 00115 ZFLUSH \ 00116 } \ 00117 } while(0) 00118 # endif 00119 00120 #endif 00121 00122 static inline int err_copy (void *dst_c, 00123 void *src_c) 00124 { 00125 errinfo_t *dst = dst_c; 00126 errinfo_t *src = src_c; 00127 assert(dst->magic == ERRINFO_MAGIC); 00128 assert(src->magic == ERRINFO_MAGIC); 00129 00130 dst->errnum = src->errnum; 00131 dst->sev = src->sev; 00132 dst->type = src->type; 00133 dst->func = src->func; 00134 dst->detail = src->detail; 00135 dst->arg_str = src->arg_str; 00136 src->arg_str = NULL; /* unused at src, avoid double free */ 00137 dst->arg_int = src->arg_int; 00138 return(-1); 00139 } 00140 00141 static inline int err_capture (const void *container, 00142 errsev_t sev, 00143 zbar_error_t type, 00144 const char *func, 00145 const char *detail) 00146 { 00147 errinfo_t *err = (errinfo_t*)container; 00148 assert(err->magic == ERRINFO_MAGIC); 00149 if(type == ZBAR_ERR_SYSTEM) 00150 err->errnum = errno; 00151 #ifdef _WIN32 00152 else if(type == ZBAR_ERR_WINAPI) 00153 err->errnum = GetLastError(); 00154 #endif 00155 err->sev = sev; 00156 err->type = type; 00157 err->func = func; 00158 err->detail = detail; 00159 if(_zbar_verbosity >= 1) 00160 _zbar_error_spew(err, 0); 00161 return(-1); 00162 } 00163 00164 static inline int err_capture_str (const void *container, 00165 errsev_t sev, 00166 zbar_error_t type, 00167 const char *func, 00168 const char *detail, 00169 const char *arg) 00170 { 00171 errinfo_t *err = (errinfo_t*)container; 00172 assert(err->magic == ERRINFO_MAGIC); 00173 if(err->arg_str) 00174 free(err->arg_str); 00175 err->arg_str = strdup(arg); 00176 return(err_capture(container, sev, type, func, detail)); 00177 } 00178 00179 static inline int err_capture_int (const void *container, 00180 errsev_t sev, 00181 zbar_error_t type, 00182 const char *func, 00183 const char *detail, 00184 int arg) 00185 { 00186 errinfo_t *err = (errinfo_t*)container; 00187 assert(err->magic == ERRINFO_MAGIC); 00188 err->arg_int = arg; 00189 return(err_capture(container, sev, type, func, detail)); 00190 } 00191 00192 static inline int err_capture_num (const void *container, 00193 errsev_t sev, 00194 zbar_error_t type, 00195 const char *func, 00196 const char *detail, 00197 int num) 00198 { 00199 errinfo_t *err = (errinfo_t*)container; 00200 assert(err->magic == ERRINFO_MAGIC); 00201 err->errnum = num; 00202 return(err_capture(container, sev, type, func, detail)); 00203 } 00204 00205 static inline void err_init (errinfo_t *err, 00206 errmodule_t module) 00207 { 00208 err->magic = ERRINFO_MAGIC; 00209 err->module = module; 00210 } 00211 00212 static inline void err_cleanup (errinfo_t *err) 00213 { 00214 assert(err->magic == ERRINFO_MAGIC); 00215 if(err->buf) { 00216 free(err->buf); 00217 err->buf = NULL; 00218 } 00219 if(err->arg_str) { 00220 free(err->arg_str); 00221 err->arg_str = NULL; 00222 } 00223 } 00224 00225 #endif 00226
Generated on Tue Jul 12 2022 18:54:12 by
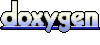