ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
decoder.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _DECODER_H_ 00024 #define _DECODER_H_ 00025 00026 #include <config.h> 00027 #include <stdlib.h> /* realloc */ 00028 00029 #include <zbar.h> 00030 00031 #define NUM_CFGS (ZBAR_CFG_MAX_LEN - ZBAR_CFG_MIN_LEN + 1) 00032 00033 #ifdef ENABLE_EAN 00034 # include "decoder/ean.h" 00035 #endif 00036 #ifdef ENABLE_I25 00037 # include "decoder/i25.h" 00038 #endif 00039 #ifdef ENABLE_CODE39 00040 # include "decoder/code39.h" 00041 #endif 00042 #ifdef ENABLE_CODE128 00043 # include "decoder/code128.h" 00044 #endif 00045 #ifdef ENABLE_PDF417 00046 # include "decoder/pdf417.h" 00047 #endif 00048 #ifdef ENABLE_QRCODE 00049 # include "decoder/qr_finder.h" 00050 #endif 00051 00052 /* size of bar width history (implementation assumes power of two) */ 00053 #ifndef DECODE_WINDOW 00054 # define DECODE_WINDOW 16 00055 #endif 00056 00057 /* initial data buffer allocation */ 00058 #ifndef BUFFER_MIN 00059 # define BUFFER_MIN 0x20 00060 #endif 00061 00062 /* maximum data buffer allocation 00063 * (longer symbols are rejected) 00064 */ 00065 #ifndef BUFFER_MAX 00066 # define BUFFER_MAX 0x100 00067 #endif 00068 00069 /* buffer allocation increment */ 00070 #ifndef BUFFER_INCR 00071 # define BUFFER_INCR 0x10 00072 #endif 00073 00074 #define CFG(dcode, cfg) ((dcode).configs[(cfg) - ZBAR_CFG_MIN_LEN]) 00075 #define TEST_CFG(config, cfg) (((config) >> (cfg)) & 1) 00076 00077 /* symbology independent decoder state */ 00078 struct zbar_decoder_s { 00079 unsigned char idx; /* current width index */ 00080 unsigned w[DECODE_WINDOW]; /* window of last N bar widths */ 00081 zbar_symbol_type_t type; /* type of last decoded data */ 00082 zbar_symbol_type_t lock; /* buffer lock */ 00083 00084 /* everything above here is automatically reset */ 00085 unsigned buf_alloc; /* dynamic buffer allocation */ 00086 unsigned buflen; /* binary data length */ 00087 unsigned char *buf; /* decoded characters */ 00088 void *userdata; /* application data */ 00089 zbar_decoder_handler_t *handler; /* application callback */ 00090 00091 /* symbology specific state */ 00092 #ifdef ENABLE_EAN 00093 ean_decoder_t ean; /* EAN/UPC parallel decode attempts */ 00094 #endif 00095 #ifdef ENABLE_I25 00096 i25_decoder_t i25; /* Interleaved 2 of 5 decode state */ 00097 #endif 00098 #ifdef ENABLE_CODE39 00099 code39_decoder_t code39; /* Code 39 decode state */ 00100 #endif 00101 #ifdef ENABLE_CODE128 00102 code128_decoder_t code128; /* Code 128 decode state */ 00103 #endif 00104 #ifdef ENABLE_PDF417 00105 pdf417_decoder_t pdf417; /* PDF417 decode state */ 00106 #endif 00107 #ifdef ENABLE_QRCODE 00108 qr_finder_t qrf; /* QR Code finder state */ 00109 #endif 00110 }; 00111 00112 /* return current element color */ 00113 static inline char get_color (const zbar_decoder_t *dcode) 00114 { 00115 return(dcode->idx & 1); 00116 } 00117 00118 /* retrieve i-th previous element width */ 00119 static inline unsigned get_width (const zbar_decoder_t *dcode, 00120 unsigned char offset) 00121 { 00122 return(dcode->w[(dcode->idx - offset) & (DECODE_WINDOW - 1)]); 00123 } 00124 00125 /* retrieve bar+space pair width starting at offset i */ 00126 static inline unsigned pair_width (const zbar_decoder_t *dcode, 00127 unsigned char offset) 00128 { 00129 return(get_width(dcode, offset) + get_width(dcode, offset + 1)); 00130 } 00131 00132 /* calculate total character width "s" 00133 * - start of character identified by context sensitive offset 00134 * (<= DECODE_WINDOW - n) 00135 * - size of character is n elements 00136 */ 00137 static inline unsigned calc_s (const zbar_decoder_t *dcode, 00138 unsigned char offset, 00139 unsigned char n) 00140 { 00141 /* FIXME check that this gets unrolled for constant n */ 00142 unsigned s = 0; 00143 while(n--) 00144 s += get_width(dcode, offset++); 00145 return(s); 00146 } 00147 00148 /* fixed character width decode assist 00149 * bar+space width are compared as a fraction of the reference dimension "x" 00150 * - +/- 1/2 x tolerance 00151 * - measured total character width (s) compared to symbology baseline (n) 00152 * (n = 7 for EAN/UPC, 11 for Code 128) 00153 * - bar+space *pair width* "e" is used to factor out bad "exposures" 00154 * ("blooming" or "swelling" of dark or light areas) 00155 * => using like-edge measurements avoids these issues 00156 * - n should be > 3 00157 */ 00158 static inline int decode_e (unsigned e, 00159 unsigned s, 00160 unsigned n) 00161 { 00162 /* result is encoded number of units - 2 00163 * (for use as zero based index) 00164 * or -1 if invalid 00165 */ 00166 unsigned char E = ((e * n * 2 + 1) / s - 3) / 2; 00167 return((E >= n - 3) ? -1 : E); 00168 } 00169 00170 /* acquire shared state lock */ 00171 static inline char get_lock (zbar_decoder_t *dcode, 00172 zbar_symbol_type_t req) 00173 { 00174 if(dcode->lock) 00175 return(1); 00176 dcode->lock = req; 00177 return(0); 00178 } 00179 00180 /* ensure output buffer has sufficient allocation for request */ 00181 static inline char size_buf (zbar_decoder_t *dcode, 00182 unsigned len) 00183 { 00184 if(len < dcode->buf_alloc) 00185 /* FIXME size reduction heuristic? */ 00186 return(0); 00187 if(len > BUFFER_MAX) 00188 return(1); 00189 if(len < dcode->buf_alloc + BUFFER_INCR) { 00190 len = dcode->buf_alloc + BUFFER_INCR; 00191 if(len > BUFFER_MAX) 00192 len = BUFFER_MAX; 00193 } 00194 unsigned char *buf = realloc(dcode->buf, len); 00195 if(!buf) 00196 return(1); 00197 dcode->buf = buf; 00198 dcode->buf_alloc = len; 00199 return(0); 00200 } 00201 00202 extern const char *_zbar_decoder_buf_dump (unsigned char *buf, 00203 unsigned int buflen); 00204 00205 #endif 00206
Generated on Tue Jul 12 2022 18:54:12 by
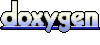