ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
config.c
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2008-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 00024 #include <config.h> 00025 #include <stdlib.h> /* strtol */ 00026 #include <string.h> /* strchr, strncmp, strlen */ 00027 #include <errno.h> 00028 #include <assert.h> 00029 00030 #include <zbar.h> 00031 00032 int zbar_parse_config (const char *cfgstr, 00033 zbar_symbol_type_t *sym, 00034 zbar_config_t *cfg, 00035 int *val) 00036 { 00037 if(!cfgstr) 00038 return(1); 00039 00040 const char *dot = strchr(cfgstr, '.'); 00041 if(dot) { 00042 int len = dot - cfgstr; 00043 if(!len || (len == 1 && !strncmp(cfgstr, "*", len))) 00044 *sym = 0; 00045 else if(len < 2) 00046 return(1); 00047 else if(!strncmp(cfgstr, "qrcode", len)) 00048 *sym = ZBAR_QRCODE; 00049 else if(len < 3) 00050 return(1); 00051 else if(!strncmp(cfgstr, "upca", len)) 00052 *sym = ZBAR_UPCA; 00053 else if(!strncmp(cfgstr, "upce", len)) 00054 *sym = ZBAR_UPCE; 00055 else if(!strncmp(cfgstr, "ean13", len)) 00056 *sym = ZBAR_EAN13; 00057 else if(!strncmp(cfgstr, "ean8", len)) 00058 *sym = ZBAR_EAN8; 00059 else if(!strncmp(cfgstr, "i25", len)) 00060 *sym = ZBAR_I25; 00061 else if(len < 4) 00062 return(1); 00063 else if(!strncmp(cfgstr, "scanner", len)) 00064 *sym = ZBAR_PARTIAL; /* FIXME lame */ 00065 else if(!strncmp(cfgstr, "isbn13", len)) 00066 *sym = ZBAR_ISBN13; 00067 else if(!strncmp(cfgstr, "isbn10", len)) 00068 *sym = ZBAR_ISBN10; 00069 #if 0 00070 /* FIXME addons are configured per-main symbol type */ 00071 else if(!strncmp(cfgstr, "addon2", len)) 00072 *sym = ZBAR_ADDON2; 00073 else if(!strncmp(cfgstr, "addon5", len)) 00074 *sym = ZBAR_ADDON5; 00075 #endif 00076 else if(len < 6) 00077 return(1); 00078 else if(!strncmp(cfgstr, "code39", len)) 00079 *sym = ZBAR_CODE39; 00080 else if(!strncmp(cfgstr, "pdf417", len)) 00081 *sym = ZBAR_PDF417; 00082 else if(len < 7) 00083 return(1); 00084 else if(!strncmp(cfgstr, "code128", len)) 00085 *sym = ZBAR_CODE128; 00086 else 00087 return(1); 00088 cfgstr = dot + 1; 00089 } 00090 else 00091 *sym = 0; 00092 00093 int len = strlen(cfgstr); 00094 const char *eq = strchr(cfgstr, '='); 00095 if(eq) 00096 len = eq - cfgstr; 00097 else 00098 *val = 1; /* handle this here so we can override later */ 00099 char negate = 0; 00100 00101 if(len > 3 && !strncmp(cfgstr, "no-", 3)) { 00102 negate = 1; 00103 cfgstr += 3; 00104 len -= 3; 00105 } 00106 00107 if(len < 1) 00108 return(1); 00109 else if(!strncmp(cfgstr, "y-density", len)) 00110 *cfg = ZBAR_CFG_Y_DENSITY; 00111 else if(!strncmp(cfgstr, "x-density", len)) 00112 *cfg = ZBAR_CFG_X_DENSITY; 00113 else if(len < 2) 00114 return(1); 00115 else if(!strncmp(cfgstr, "enable", len)) 00116 *cfg = ZBAR_CFG_ENABLE; 00117 else if(len < 3) 00118 return(1); 00119 else if(!strncmp(cfgstr, "disable", len)) { 00120 *cfg = ZBAR_CFG_ENABLE; 00121 negate = !negate; /* no-disable ?!? */ 00122 } 00123 else if(!strncmp(cfgstr, "min-length", len)) 00124 *cfg = ZBAR_CFG_MIN_LEN; 00125 else if(!strncmp(cfgstr, "max-length", len)) 00126 *cfg = ZBAR_CFG_MAX_LEN; 00127 else if(!strncmp(cfgstr, "ascii", len)) 00128 *cfg = ZBAR_CFG_ASCII; 00129 else if(!strncmp(cfgstr, "add-check", len)) 00130 *cfg = ZBAR_CFG_ADD_CHECK; 00131 else if(!strncmp(cfgstr, "emit-check", len)) 00132 *cfg = ZBAR_CFG_EMIT_CHECK; 00133 else if(!strncmp(cfgstr, "position", len)) 00134 *cfg = ZBAR_CFG_POSITION; 00135 else 00136 return(1); 00137 00138 if(eq) { 00139 errno = 0; 00140 *val = strtol(eq + 1, NULL, 0); 00141 if(errno) 00142 return(1); 00143 } 00144 if(negate) 00145 *val = !*val; 00146 00147 return(0); 00148 } 00149
Generated on Tue Jul 12 2022 18:54:12 by
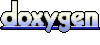