libuav original
Dependents: UAVCAN UAVCAN_Subscriber
panic_broadcaster.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_PROTOCOL_PANIC_BROADCASTER_HPP_INCLUDED 00006 #define UAVCAN_PROTOCOL_PANIC_BROADCASTER_HPP_INCLUDED 00007 00008 #include <uavcan/debug.hpp> 00009 #include <uavcan/node/publisher.hpp> 00010 #include <uavcan/node/timer.hpp> 00011 #include <uavcan/protocol/Panic.hpp> 00012 00013 namespace uavcan 00014 { 00015 /** 00016 * Helper for broadcasting the message uavcan.protocol.Panic. 00017 */ 00018 class UAVCAN_EXPORT PanicBroadcaster : private TimerBase 00019 { 00020 Publisher<protocol::Panic> pub_; 00021 protocol::Panic msg_; 00022 00023 void publishOnce() 00024 { 00025 const int res = pub_.broadcast(msg_); 00026 if (res < 0) 00027 { 00028 pub_.getNode().registerInternalFailure("Panic pub failed"); 00029 } 00030 } 00031 00032 virtual void handleTimerEvent(const TimerEvent&) 00033 { 00034 publishOnce(); 00035 } 00036 00037 public: 00038 explicit PanicBroadcaster(INode& node) 00039 : TimerBase(node) 00040 , pub_(node) 00041 { } 00042 00043 /** 00044 * This method does not block and can't fail. 00045 * @param short_reason Short ASCII string that describes the reason of the panic, 7 characters max. 00046 * If the string exceeds 7 characters, it will be truncated. 00047 * @param broadcasting_period Broadcasting period. Optional. 00048 * @param priority Transfer priority. Optional. 00049 */ 00050 void panic(const char* short_reason_description, 00051 MonotonicDuration broadcasting_period = MonotonicDuration::fromMSec(100), 00052 const TransferPriority priority = TransferPriority::Default) 00053 { 00054 msg_.reason_text.clear(); 00055 const char* p = short_reason_description; 00056 while (p && *p) 00057 { 00058 if (msg_.reason_text.size() == msg_.reason_text.capacity()) 00059 { 00060 break; 00061 } 00062 msg_.reason_text.push_back(protocol::Panic::FieldTypes::reason_text::ValueType(*p)); 00063 p++; 00064 } 00065 00066 UAVCAN_TRACE("PanicBroadcaster", "Panicking with reason '%s'", getReason().c_str()); 00067 00068 pub_.setTxTimeout(broadcasting_period); 00069 pub_.setPriority(priority); 00070 00071 publishOnce(); 00072 startPeriodic(broadcasting_period); 00073 } 00074 00075 /** 00076 * Stop broadcasting immediately. 00077 */ 00078 void dontPanic() // Where's my towel 00079 { 00080 stop(); 00081 msg_.reason_text.clear(); 00082 } 00083 00084 bool isPanicking() const { return isRunning(); } 00085 00086 const typename protocol::Panic::FieldTypes::reason_text& getReason() const 00087 { 00088 return msg_.reason_text; 00089 } 00090 }; 00091 00092 } 00093 00094 #endif // UAVCAN_PROTOCOL_PANIC_BROADCASTER_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:33 by
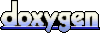