libuav original
Dependents: UAVCAN UAVCAN_Subscriber
INode Class Reference
Abstract node class. More...
#include <abstract_node.hpp>
Inherited by Node< NodeMemPoolSize >, SubNode< NodeMemPoolSize >, TestNode, Node< MemPoolSize >, and SubNode< MemPoolSize >.
Public Member Functions | |
NodeID | getNodeID () const |
Returns the Node ID of this node. | |
bool | setNodeID (NodeID nid) |
Sets the Node ID of this node. | |
bool | isPassiveMode () const |
Whether the node is in passive mode, i.e. | |
int | spin (MonotonicTime deadline) |
Same as spin(MonotonicDuration), but the deadline is specified as an absolute time value rather than duration. | |
int | spin (MonotonicDuration duration) |
Runs the node. | |
int | spinOnce () |
This method is designed for non-blocking applications. | |
int | injectTxFrame (const CanFrame &frame, MonotonicTime tx_deadline, uint8_t iface_mask, CanTxQueue::Qos qos=CanTxQueue::Volatile, CanIOFlags flags=0) |
This method allows to directly transmit a raw CAN frame circumventing the whole UAVCAN stack. | |
void | removeRxFrameListener () |
The IRxFrameListener interface allows one to monitor all incoming CAN frames. |
Detailed Description
Abstract node class.
If you're going to implement your own node class for your application, please inherit this class so it can be used with default publisher, subscriber, server, etc. classes. Normally you don't need to use it directly though - please refer to the class Node<> instead.
Definition at line 19 of file abstract_node.hpp.
Member Function Documentation
NodeID getNodeID | ( | ) | const |
Returns the Node ID of this node.
If Node ID was not set yet, an invalid value will be returned.
Definition at line 39 of file abstract_node.hpp.
int injectTxFrame | ( | const CanFrame & | frame, |
MonotonicTime | tx_deadline, | ||
uint8_t | iface_mask, | ||
CanTxQueue::Qos | qos = CanTxQueue::Volatile , |
||
CanIOFlags | flags = 0 |
||
) |
This method allows to directly transmit a raw CAN frame circumventing the whole UAVCAN stack.
Mandatory parameters:
- Parameters:
-
frame CAN frame to be transmitted. tx_deadline The frame will be discarded if it could not be transmitted by this time. iface_mask This bitmask allows to select what CAN interfaces this frame should go into. Example: - 1 - the frame will be sent only to iface 0.
- 4 - the frame will be sent only to iface 2.
- 3 - the frame will be sent to ifaces 0 and 1.
Optional parameters:
- Parameters:
-
qos Quality of service. Please refer to the CAN IO manager for details. flags CAN IO flags. Please refer to the CAN driver API for details.
Definition at line 111 of file abstract_node.hpp.
bool isPassiveMode | ( | ) | const |
Whether the node is in passive mode, i.e.
can't transmit anything to the bus. Please read the specs to learn more.
Definition at line 57 of file abstract_node.hpp.
void removeRxFrameListener | ( | ) |
The IRxFrameListener interface allows one to monitor all incoming CAN frames.
This feature can be used to implement multithreaded nodes, or to add secondary protocol support.
Definition at line 123 of file abstract_node.hpp.
bool setNodeID | ( | NodeID | nid ) |
Sets the Node ID of this node.
Node ID can be assigned only once. This method returns true if the Node ID was successfully assigned, otherwise it returns false. As long as a valid Node ID is not set, the node will remain in passive mode. Using a non-unicast Node ID puts the node into passive mode (as default).
Definition at line 48 of file abstract_node.hpp.
int spin | ( | MonotonicTime | deadline ) |
Same as spin(MonotonicDuration), but the deadline is specified as an absolute time value rather than duration.
Reimplemented in Node< MemPoolSize >, and Node< NodeMemPoolSize >.
Definition at line 63 of file abstract_node.hpp.
int spin | ( | MonotonicDuration | duration ) |
Runs the node.
Normally your application should not block anywhere else. Block inside this method forever or call it periodically. This method returns 0 if no errors occurred, or a negative error code if something failed (see error.hpp).
Reimplemented in Node< MemPoolSize >, and Node< NodeMemPoolSize >.
Definition at line 74 of file abstract_node.hpp.
int spinOnce | ( | ) |
This method is designed for non-blocking applications.
Instead of blocking, it returns immediately once all available CAN frames and timer events are processed. Note that this is unlike plain spin(), which will strictly return when the deadline is reached, even if there still are unprocessed events. This method returns 0 if no errors occurred, or a negative error code if something failed (see error.hpp).
Reimplemented in Node< MemPoolSize >, and Node< NodeMemPoolSize >.
Definition at line 86 of file abstract_node.hpp.
Generated on Tue Jul 12 2022 17:17:37 by
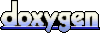