libuav original
Dependents: UAVCAN UAVCAN_Subscriber
abstract_node.hpp
00001 /* 00002 * Copyright (C) 2014 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #ifndef UAVCAN_NODE_ABSTRACT_NODE_HPP_INCLUDED 00006 #define UAVCAN_NODE_ABSTRACT_NODE_HPP_INCLUDED 00007 00008 #include <uavcan/build_config.hpp> 00009 #include <uavcan/dynamic_memory.hpp> 00010 #include <uavcan/node/scheduler.hpp> 00011 00012 namespace uavcan 00013 { 00014 /** 00015 * Abstract node class. If you're going to implement your own node class for your application, 00016 * please inherit this class so it can be used with default publisher, subscriber, server, etc. classes. 00017 * Normally you don't need to use it directly though - please refer to the class Node<> instead. 00018 */ 00019 class UAVCAN_EXPORT INode 00020 { 00021 public: 00022 virtual ~INode() { } 00023 virtual IPoolAllocator& getAllocator() = 0; 00024 virtual Scheduler& getScheduler() = 0; 00025 virtual const Scheduler& getScheduler() const = 0; 00026 virtual void registerInternalFailure(const char* msg) = 0; 00027 00028 Dispatcher& getDispatcher() { return getScheduler().getDispatcher(); } 00029 const Dispatcher& getDispatcher() const { return getScheduler().getDispatcher(); } 00030 00031 ISystemClock& getSystemClock() { return getScheduler().getSystemClock(); } 00032 MonotonicTime getMonotonicTime() const { return getScheduler().getMonotonicTime(); } 00033 UtcTime getUtcTime() const { return getScheduler().getUtcTime(); } 00034 00035 /** 00036 * Returns the Node ID of this node. 00037 * If Node ID was not set yet, an invalid value will be returned. 00038 */ 00039 NodeID getNodeID() const { return getScheduler().getDispatcher().getNodeID(); } 00040 00041 /** 00042 * Sets the Node ID of this node. 00043 * Node ID can be assigned only once. This method returns true if the Node ID was successfully assigned, otherwise 00044 * it returns false. 00045 * As long as a valid Node ID is not set, the node will remain in passive mode. 00046 * Using a non-unicast Node ID puts the node into passive mode (as default). 00047 */ 00048 bool setNodeID(NodeID nid) 00049 { 00050 return getScheduler().getDispatcher().setNodeID(nid); 00051 } 00052 00053 /** 00054 * Whether the node is in passive mode, i.e. can't transmit anything to the bus. 00055 * Please read the specs to learn more. 00056 */ 00057 bool isPassiveMode() const { return getScheduler().getDispatcher().isPassiveMode(); } 00058 00059 /** 00060 * Same as @ref spin(MonotonicDuration), but the deadline is specified as an absolute time value 00061 * rather than duration. 00062 */ 00063 int spin(MonotonicTime deadline) 00064 { 00065 return getScheduler().spin(deadline); 00066 } 00067 00068 /** 00069 * Runs the node. 00070 * Normally your application should not block anywhere else. 00071 * Block inside this method forever or call it periodically. 00072 * This method returns 0 if no errors occurred, or a negative error code if something failed (see error.hpp). 00073 */ 00074 int spin(MonotonicDuration duration) 00075 { 00076 return getScheduler().spin(getMonotonicTime() + duration); 00077 } 00078 00079 /** 00080 * This method is designed for non-blocking applications. 00081 * Instead of blocking, it returns immediately once all available CAN frames and timer events are processed. 00082 * Note that this is unlike plain @ref spin(), which will strictly return when the deadline is reached, 00083 * even if there still are unprocessed events. 00084 * This method returns 0 if no errors occurred, or a negative error code if something failed (see error.hpp). 00085 */ 00086 int spinOnce() 00087 { 00088 return getScheduler().spinOnce(); 00089 } 00090 00091 /** 00092 * This method allows to directly transmit a raw CAN frame circumventing the whole UAVCAN stack. 00093 * Mandatory parameters: 00094 * 00095 * @param frame CAN frame to be transmitted. 00096 * 00097 * @param tx_deadline The frame will be discarded if it could not be transmitted by this time. 00098 * 00099 * @param iface_mask This bitmask allows to select what CAN interfaces this frame should go into. 00100 * Example: 00101 * - 1 - the frame will be sent only to iface 0. 00102 * - 4 - the frame will be sent only to iface 2. 00103 * - 3 - the frame will be sent to ifaces 0 and 1. 00104 * 00105 * Optional parameters: 00106 * 00107 * @param qos Quality of service. Please refer to the CAN IO manager for details. 00108 * 00109 * @param flags CAN IO flags. Please refer to the CAN driver API for details. 00110 */ 00111 int injectTxFrame(const CanFrame& frame, MonotonicTime tx_deadline, uint8_t iface_mask, 00112 CanTxQueue::Qos qos = CanTxQueue::Volatile, 00113 CanIOFlags flags = 0) 00114 { 00115 return getDispatcher().getCanIOManager().send(frame, tx_deadline, MonotonicTime(), iface_mask, qos, flags); 00116 } 00117 00118 #if !UAVCAN_TINY 00119 /** 00120 * The @ref IRxFrameListener interface allows one to monitor all incoming CAN frames. 00121 * This feature can be used to implement multithreaded nodes, or to add secondary protocol support. 00122 */ 00123 void removeRxFrameListener() { getDispatcher().removeRxFrameListener(); } 00124 void installRxFrameListener(IRxFrameListener* lst) { getDispatcher().installRxFrameListener(lst); } 00125 #endif 00126 }; 00127 00128 } 00129 00130 #endif // UAVCAN_NODE_ABSTRACT_NODE_HPP_INCLUDED
Generated on Tue Jul 12 2022 17:17:29 by
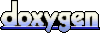