
the program have one sensor acs712 and moduleds1302
Dependencies: FATFileSystem mbed
Thread Class Reference
The Thread class allow defining, creating, and controlling thread functions in the system. More...
#include <Thread.h>
Public Types | |
enum | State { Inactive, Ready, Running, WaitingDelay, WaitingInterval, WaitingOr, WaitingAnd, WaitingSemaphore, WaitingMailbox, WaitingMutex } |
State of the Thread. More... | |
Public Member Functions | |
Thread (void(*task)(void const *argument), void *argument=NULL, osPriority priority=osPriorityNormal, uint32_t stack_size=DEFAULT_STACK_SIZE, unsigned char *stack_pointer=NULL) | |
Create a new thread, and start it executing the specified function. | |
osStatus | terminate () |
Terminate execution of a thread and remove it from Active Threads. | |
osStatus | set_priority (osPriority priority) |
Set priority of an active thread. | |
osPriority | get_priority () |
Get priority of an active thread. | |
int32_t | signal_set (int32_t signals) |
Set the specified Signal Flags of an active thread. | |
int32_t | signal_clr (int32_t signals) |
Clears the specified Signal Flags of an active thread. | |
State | get_state () |
State of this Thread. | |
uint32_t | stack_size () |
Get the total stack memory size for this Thread. | |
uint32_t | free_stack () |
Get the currently unused stack memory for this Thread. | |
uint32_t | used_stack () |
Get the currently used stack memory for this Thread. | |
uint32_t | max_stack () |
Get the maximum stack memory usage to date for this Thread. | |
Static Public Member Functions | |
static osEvent | signal_wait (int32_t signals, uint32_t millisec=osWaitForever) |
Wait for one or more Signal Flags to become signaled for the current RUNNING thread. | |
static osStatus | wait (uint32_t millisec) |
Wait for a specified time period in millisec: | |
static osStatus | yield () |
Pass control to next thread that is in state READY. | |
static osThreadId | gettid () |
Get the thread id of the current running thread. | |
static void | attach_idle_hook (void(*fptr)(void)) |
Attach a function to be called by the RTOS idle task. |
Detailed Description
The Thread class allow defining, creating, and controlling thread functions in the system.
Definition at line 31 of file Thread.h.
Member Enumeration Documentation
enum State |
State of the Thread.
- Enumerator:
Constructor & Destructor Documentation
Thread | ( | void(*)(void const *argument) | task, |
void * | argument = NULL , |
||
osPriority | priority = osPriorityNormal , |
||
uint32_t | stack_size = DEFAULT_STACK_SIZE , |
||
unsigned char * | stack_pointer = NULL |
||
) |
Create a new thread, and start it executing the specified function.
- Parameters:
-
task function to be executed by this thread. argument pointer that is passed to the thread function as start argument. (default: NULL). priority initial priority of the thread function. (default: osPriorityNormal). stack_size stack size (in bytes) requirements for the thread function. (default: DEFAULT_STACK_SIZE). stack_pointer pointer to the stack area to be used by this thread (default: NULL).
Definition at line 29 of file Thread.cpp.
Member Function Documentation
void attach_idle_hook | ( | void(*)(void) | fptr ) | [static] |
Attach a function to be called by the RTOS idle task.
- Parameters:
-
fptr pointer to the function to be called
Definition at line 136 of file Thread.cpp.
uint32_t free_stack | ( | ) |
Get the currently unused stack memory for this Thread.
- Returns:
- the currently unused stack memory in bytes
Definition at line 91 of file Thread.cpp.
osPriority get_priority | ( | ) |
Get priority of an active thread.
- Returns:
- current priority value of the thread function.
Definition at line 61 of file Thread.cpp.
Thread::State get_state | ( | ) |
osThreadId gettid | ( | ) | [static] |
Get the thread id of the current running thread.
- Returns:
- thread ID for reference by other functions or NULL in case of error.
Definition at line 132 of file Thread.cpp.
uint32_t max_stack | ( | ) |
Get the maximum stack memory usage to date for this Thread.
- Returns:
- the maximum stack memory usage to date in bytes
Definition at line 109 of file Thread.cpp.
osStatus set_priority | ( | osPriority | priority ) |
Set priority of an active thread.
- Parameters:
-
priority new priority value for the thread function.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 57 of file Thread.cpp.
int32_t signal_clr | ( | int32_t | signals ) |
Clears the specified Signal Flags of an active thread.
- Parameters:
-
signals specifies the signal flags of the thread that should be cleared.
- Returns:
- resultant signal flags of the specified thread or 0x80000000 in case of incorrect parameters.
Definition at line 69 of file Thread.cpp.
int32_t signal_set | ( | int32_t | signals ) |
Set the specified Signal Flags of an active thread.
- Parameters:
-
signals specifies the signal flags of the thread that should be set.
- Returns:
- previous signal flags of the specified thread or 0x80000000 in case of incorrect parameters.
Definition at line 65 of file Thread.cpp.
osEvent signal_wait | ( | int32_t | signals, |
uint32_t | millisec = osWaitForever |
||
) | [static] |
Wait for one or more Signal Flags to become signaled for the current RUNNING thread.
- Parameters:
-
signals wait until all specified signal flags set or 0 for any single signal flag. millisec timeout value or 0 in case of no time-out. (default: osWaitForever).
- Returns:
- event flag information or error code.
Definition at line 120 of file Thread.cpp.
uint32_t stack_size | ( | ) |
Get the total stack memory size for this Thread.
- Returns:
- the total stack memory size in bytes
Definition at line 83 of file Thread.cpp.
osStatus terminate | ( | ) |
Terminate execution of a thread and remove it from Active Threads.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 53 of file Thread.cpp.
uint32_t used_stack | ( | ) |
Get the currently used stack memory for this Thread.
- Returns:
- the currently used stack memory in bytes
Definition at line 100 of file Thread.cpp.
osStatus wait | ( | uint32_t | millisec ) | [static] |
Wait for a specified time period in millisec:
- Parameters:
-
millisec time delay value
- Returns:
- status code that indicates the execution status of the function.
Definition at line 124 of file Thread.cpp.
osStatus yield | ( | ) | [static] |
Pass control to next thread that is in state READY.
- Returns:
- status code that indicates the execution status of the function.
Definition at line 128 of file Thread.cpp.
Generated on Wed Jul 13 2022 05:59:47 by
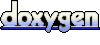