
the program have one sensor acs712 and moduleds1302
Dependencies: FATFileSystem mbed
Thread.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2012 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #include "Thread.h" 00023 00024 #include "mbed_error.h" 00025 #include "rtos_idle.h" 00026 00027 namespace rtos { 00028 00029 Thread::Thread(void (*task)(void const *argument), void *argument, 00030 osPriority priority, uint32_t stack_size, unsigned char *stack_pointer) { 00031 #ifdef CMSIS_OS_RTX 00032 _thread_def.pthread = task; 00033 _thread_def.tpriority = priority; 00034 _thread_def.stacksize = stack_size; 00035 if (stack_pointer != NULL) { 00036 _thread_def.stack_pointer = (uint32_t*)stack_pointer; 00037 _dynamic_stack = false; 00038 } else { 00039 _thread_def.stack_pointer = new uint32_t[stack_size/sizeof(uint32_t)]; 00040 if (_thread_def.stack_pointer == NULL) 00041 error("Error allocating the stack memory\n"); 00042 _dynamic_stack = true; 00043 } 00044 00045 //Fill the stack with a magic word for maximum usage checking 00046 for (uint32_t i = 0; i < (stack_size / sizeof(uint32_t)); i++) { 00047 _thread_def.stack_pointer[i] = 0xE25A2EA5; 00048 } 00049 #endif 00050 _tid = osThreadCreate(&_thread_def, argument); 00051 } 00052 00053 osStatus Thread::terminate() { 00054 return osThreadTerminate(_tid); 00055 } 00056 00057 osStatus Thread::set_priority(osPriority priority) { 00058 return osThreadSetPriority(_tid, priority); 00059 } 00060 00061 osPriority Thread::get_priority() { 00062 return osThreadGetPriority(_tid); 00063 } 00064 00065 int32_t Thread::signal_set(int32_t signals) { 00066 return osSignalSet(_tid, signals); 00067 } 00068 00069 int32_t Thread::signal_clr(int32_t signals) { 00070 return osSignalClear(_tid, signals); 00071 } 00072 00073 Thread::State Thread::get_state() { 00074 #ifndef __MBED_CMSIS_RTOS_CA9 00075 return ((State)_thread_def.tcb.state); 00076 #else 00077 uint8_t status; 00078 status = osThreadGetState(_tid); 00079 return ((State)status); 00080 #endif 00081 } 00082 00083 uint32_t Thread::stack_size() { 00084 #ifndef __MBED_CMSIS_RTOS_CA9 00085 return _thread_def.tcb.priv_stack; 00086 #else 00087 return 0; 00088 #endif 00089 } 00090 00091 uint32_t Thread::free_stack() { 00092 #ifndef __MBED_CMSIS_RTOS_CA9 00093 uint32_t bottom = (uint32_t)_thread_def.tcb.stack; 00094 return _thread_def.tcb.tsk_stack - bottom; 00095 #else 00096 return 0; 00097 #endif 00098 } 00099 00100 uint32_t Thread::used_stack() { 00101 #ifndef __MBED_CMSIS_RTOS_CA9 00102 uint32_t top = (uint32_t)_thread_def.tcb.stack + _thread_def.tcb.priv_stack; 00103 return top - _thread_def.tcb.tsk_stack; 00104 #else 00105 return 0; 00106 #endif 00107 } 00108 00109 uint32_t Thread::max_stack() { 00110 #ifndef __MBED_CMSIS_RTOS_CA9 00111 uint32_t high_mark = 0; 00112 while (_thread_def.tcb.stack[high_mark] == 0xE25A2EA5) 00113 high_mark++; 00114 return _thread_def.tcb.priv_stack - (high_mark * 4); 00115 #else 00116 return 0; 00117 #endif 00118 } 00119 00120 osEvent Thread::signal_wait(int32_t signals, uint32_t millisec) { 00121 return osSignalWait(signals, millisec); 00122 } 00123 00124 osStatus Thread::wait(uint32_t millisec) { 00125 return osDelay(millisec); 00126 } 00127 00128 osStatus Thread::yield() { 00129 return osThreadYield(); 00130 } 00131 00132 osThreadId Thread::gettid() { 00133 return osThreadGetId(); 00134 } 00135 00136 void Thread::attach_idle_hook(void (*fptr)(void)) { 00137 rtos_attach_idle_hook(fptr); 00138 } 00139 00140 Thread::~Thread() { 00141 terminate(); 00142 if (_dynamic_stack) { 00143 delete[] (_thread_def.stack_pointer); 00144 } 00145 } 00146 00147 }
Generated on Wed Jul 13 2022 05:59:47 by
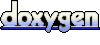