PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
CHIP: LPC11u6x DMA Controller driver common channel functions
[CHIP: LPC11u6x DMA Controller driver]
Functions | |
STATIC INLINE void | Chip_DMA_EnableChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Enables a single DMA channel. | |
STATIC INLINE void | Chip_DMA_DisableChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Disables a single DMA channel. | |
STATIC INLINE uint32_t | Chip_DMA_GetEnabledChannels (LPC_DMA_T *pDMA) |
Returns all enabled DMA channels. | |
STATIC INLINE uint32_t | Chip_DMA_GetActiveChannels (LPC_DMA_T *pDMA) |
Returns all active DMA channels. | |
STATIC INLINE uint32_t | Chip_DMA_GetBusyChannels (LPC_DMA_T *pDMA) |
Returns all busy DMA channels. | |
STATIC INLINE uint32_t | Chip_DMA_GetErrorIntChannels (LPC_DMA_T *pDMA) |
Returns pending error interrupt status for all DMA channels. | |
STATIC INLINE void | Chip_DMA_ClearErrorIntChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Clears a pending error interrupt status for a single DMA channel. | |
STATIC INLINE void | Chip_DMA_EnableIntChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Enables a single DMA channel's interrupt used in common DMA interrupt. | |
STATIC INLINE void | Chip_DMA_DisableIntChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Disables a single DMA channel's interrupt used in common DMA interrupt. | |
STATIC INLINE uint32_t | Chip_DMA_GetEnableIntChannels (LPC_DMA_T *pDMA) |
Returns all enabled interrupt channels. | |
STATIC INLINE uint32_t | Chip_DMA_GetActiveIntAChannels (LPC_DMA_T *pDMA) |
Returns active A interrupt status for all channels. | |
STATIC INLINE void | Chip_DMA_ClearActiveIntAChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Clears active A interrupt status for a single channel. | |
STATIC INLINE uint32_t | Chip_DMA_GetActiveIntBChannels (LPC_DMA_T *pDMA) |
Returns active B interrupt status for all channels. | |
STATIC INLINE void | Chip_DMA_ClearActiveIntBChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Clears active B interrupt status for a single channel. | |
STATIC INLINE void | Chip_DMA_SetValidChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Sets the VALIDPENDING control bit for a single channel. | |
STATIC INLINE void | Chip_DMA_SetTrigChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Sets the TRIG bit for a single channel. | |
STATIC INLINE void | Chip_DMA_AbortChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Aborts a DMA operation for a single channel. |
Function Documentation
STATIC INLINE void Chip_DMA_AbortChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Aborts a DMA operation for a single channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
- Note:
- To abort a channel, the channel should first be disabled. Then wait until the channel is no longer busy by checking the corresponding bit in BUSY. Finally, abort the channel operation. This prevents the channel from restarting an incomplete operation when it is enabled again.
Definition at line 423 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_ClearActiveIntAChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Clears active A interrupt status for a single channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 357 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_ClearActiveIntBChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Clears active B interrupt status for a single channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 382 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_ClearErrorIntChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Clears a pending error interrupt status for a single DMA channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 295 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_DisableChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Disables a single DMA channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 226 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_DisableIntChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Disables a single DMA channel's interrupt used in common DMA interrupt.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 317 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_EnableChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Enables a single DMA channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 215 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_EnableIntChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Enables a single DMA channel's interrupt used in common DMA interrupt.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 306 of file dma_11u6x.h.
STATIC INLINE uint32_t Chip_DMA_GetActiveChannels | ( | LPC_DMA_T * | pDMA ) |
Returns all active DMA channels.
- Parameters:
-
pDMA : The base of DMA controller on the chip
- Returns:
- An Or'ed value of all active DMA channels (0 - 15)
- Note:
- A high values in bits 0 .. 15 in the return values indicates that the channel for that bit (bit 0 = channel 0, bit 1 - channel 1, etc.) is active. A low state is inactive. A active channel indicates that a DMA operation has been started but not yet fully completed.
Definition at line 254 of file dma_11u6x.h.
STATIC INLINE uint32_t Chip_DMA_GetActiveIntAChannels | ( | LPC_DMA_T * | pDMA ) |
Returns active A interrupt status for all channels.
- Parameters:
-
pDMA : The base of DMA controller on the chip
- Returns:
- Nothing
- Note:
- A high values in bits 0 .. 15 in the return values indicates that the channel for that bit (bit 0 = channel 0, bit 1 - channel 1, etc.) has an active A interrupt for the channel. A low state indicates that the A interrupt is not active.
Definition at line 346 of file dma_11u6x.h.
STATIC INLINE uint32_t Chip_DMA_GetActiveIntBChannels | ( | LPC_DMA_T * | pDMA ) |
Returns active B interrupt status for all channels.
- Parameters:
-
pDMA : The base of DMA controller on the chip
- Returns:
- Nothing
- Note:
- A high values in bits 0 .. 15 in the return values indicates that the channel for that bit (bit 0 = channel 0, bit 1 - channel 1, etc.) has an active B interrupt for the channel. A low state indicates that the B interrupt is not active.
Definition at line 371 of file dma_11u6x.h.
STATIC INLINE uint32_t Chip_DMA_GetBusyChannels | ( | LPC_DMA_T * | pDMA ) |
Returns all busy DMA channels.
- Parameters:
-
pDMA : The base of DMA controller on the chip
- Returns:
- An Or'ed value of all busy DMA channels (0 - 15)
- Note:
- A high values in bits 0 .. 15 in the return values indicates that the channel for that bit (bit 0 = channel 0, bit 1 - channel 1, etc.) is busy. A low state is not busy. A DMA channel is considered busy when there is any operation related to that channel in the DMA controller�s internal pipeline.
Definition at line 270 of file dma_11u6x.h.
STATIC INLINE uint32_t Chip_DMA_GetEnabledChannels | ( | LPC_DMA_T * | pDMA ) |
Returns all enabled DMA channels.
- Parameters:
-
pDMA : The base of DMA controller on the chip
- Returns:
- An Or'ed value of all enabled DMA channels (0 - 15)
- Note:
- A high values in bits 0 .. 15 in the return values indicates that the channel for that bit (bit 0 = channel 0, bit 1 - channel 1, etc.) is enabled. A low state is disabled.
Definition at line 239 of file dma_11u6x.h.
STATIC INLINE uint32_t Chip_DMA_GetEnableIntChannels | ( | LPC_DMA_T * | pDMA ) |
Returns all enabled interrupt channels.
- Parameters:
-
pDMA : The base of DMA controller on the chip
- Returns:
- Nothing
- Note:
- A high values in bits 0 .. 15 in the return values indicates that the channel for that bit (bit 0 = channel 0, bit 1 - channel 1, etc.) has an enabled interrupt for the channel. A low state indicates that the DMA channel will not contribute to the common DMA interrupt status.
Definition at line 332 of file dma_11u6x.h.
STATIC INLINE uint32_t Chip_DMA_GetErrorIntChannels | ( | LPC_DMA_T * | pDMA ) |
Returns pending error interrupt status for all DMA channels.
- Parameters:
-
pDMA : The base of DMA controller on the chip
- Returns:
- An Or'ed value of all channels (0 - 15) error interrupt status
- Note:
- A high values in bits 0 .. 15 in the return values indicates that the channel for that bit (bit 0 = channel 0, bit 1 - channel 1, etc.) has a pending error interrupt. A low state indicates no error interrupt.
Definition at line 284 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_SetTrigChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Sets the TRIG bit for a single channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
- Note:
- See the User Manual for more information for what this bit does.
Definition at line 407 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_SetValidChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Sets the VALIDPENDING control bit for a single channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
- Note:
- See the User Manual for more information for what this bit does.
Definition at line 395 of file dma_11u6x.h.
Generated on Tue Jul 12 2022 11:20:46 by
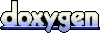