
blablabla
Dependencies: MAG3110 MMA8451Q SLCD- TSI USBDevice mbed
USBMouse Class Reference
#include <USBMouse.h>
Inherits USBHID.
Public Member Functions | |
USBMouse (MOUSE_TYPE mouse_type=REL_MOUSE, uint16_t vendor_id=0x1234, uint16_t product_id=0x0001, uint16_t product_release=0x0001) | |
Constructor. | |
bool | update (int16_t x, int16_t y, uint8_t buttons, int8_t z) |
Write a state of the mouse. | |
bool | move (int16_t x, int16_t y) |
Move the cursor to (x, y) | |
bool | press (uint8_t button) |
Press one or several buttons. | |
bool | release (uint8_t button) |
Release one or several buttons. | |
bool | doubleClick () |
Double click (MOUSE_LEFT) | |
bool | click (uint8_t button) |
Click. | |
bool | scroll (int8_t z) |
Scrolling. | |
bool | send (HID_REPORT *report) |
Send a Report. | |
bool | sendNB (HID_REPORT *report) |
Send a Report. | |
bool | read (HID_REPORT *report) |
Read a report: blocking. | |
bool | readNB (HID_REPORT *report) |
Read a report: non blocking. |
Detailed Description
USBMouse example.
#include "mbed.h" #include "USBMouse.h" USBMouse mouse; int main(void) { while (1) { mouse.move(20, 0); wait(0.5); } }
#include "mbed.h" #include "USBMouse.h" #include <math.h> USBMouse mouse(ABS_MOUSE); int main(void) { uint16_t x_center = (X_MAX_ABS - X_MIN_ABS)/2; uint16_t y_center = (Y_MAX_ABS - Y_MIN_ABS)/2; uint16_t x_screen = 0; uint16_t y_screen = 0; uint32_t x_origin = x_center; uint32_t y_origin = y_center; uint32_t radius = 5000; uint32_t angle = 0; while (1) { x_screen = x_origin + cos((double)angle*3.14/180.0)*radius; y_screen = y_origin + sin((double)angle*3.14/180.0)*radius; mouse.move(x_screen, y_screen); angle += 3; wait(0.01); } }
Definition at line 107 of file USBMouse.h.
Constructor & Destructor Documentation
USBMouse | ( | MOUSE_TYPE | mouse_type = REL_MOUSE , |
uint16_t | vendor_id = 0x1234 , |
||
uint16_t | product_id = 0x0001 , |
||
uint16_t | product_release = 0x0001 |
||
) |
Constructor.
- Parameters:
-
mouse_type Mouse type: ABS_MOUSE (absolute mouse) or REL_MOUSE (relative mouse) (default: REL_MOUSE) vendor_id Your vendor_id (default: 0x1234) product_id Your product_id (default: 0x0001) product_release Your preoduct_release (default: 0x0001)
Definition at line 120 of file USBMouse.h.
Member Function Documentation
bool click | ( | uint8_t | button ) |
Click.
- Parameters:
-
button state of the buttons ( ex: clic(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 88 of file USBMouse.cpp.
bool doubleClick | ( | ) |
Double click (MOUSE_LEFT)
- Returns:
- true if there is no error, false otherwise
Definition at line 81 of file USBMouse.cpp.
bool move | ( | int16_t | x, |
int16_t | y | ||
) |
Move the cursor to (x, y)
- Parameters:
-
x-axis position y-axis position
- Returns:
- true if there is no error, false otherwise
Definition at line 72 of file USBMouse.cpp.
bool press | ( | uint8_t | button ) |
Press one or several buttons.
- Parameters:
-
button button state (ex: press(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 95 of file USBMouse.cpp.
bool read | ( | HID_REPORT * | report ) | [inherited] |
Read a report: blocking.
- Parameters:
-
report pointer to the report to fill
- Returns:
- true if successful
Definition at line 45 of file USBHID.cpp.
bool readNB | ( | HID_REPORT * | report ) | [inherited] |
Read a report: non blocking.
- Parameters:
-
report pointer to the report to fill
- Returns:
- true if successful
Definition at line 57 of file USBHID.cpp.
bool release | ( | uint8_t | button ) |
Release one or several buttons.
- Parameters:
-
button button state (ex: release(MOUSE_LEFT))
- Returns:
- true if there is no error, false otherwise
Definition at line 100 of file USBMouse.cpp.
bool scroll | ( | int8_t | z ) |
Scrolling.
- Parameters:
-
z value of the wheel (>0 to go down, <0 to go up)
- Returns:
- true if there is no error, false otherwise
Definition at line 76 of file USBMouse.cpp.
bool send | ( | HID_REPORT * | report ) | [inherited] |
Send a Report.
warning: blocking
- Parameters:
-
report Report which will be sent (a report is defined by all data and the length)
- Returns:
- true if successful
Definition at line 34 of file USBHID.cpp.
bool sendNB | ( | HID_REPORT * | report ) | [inherited] |
Send a Report.
warning: non blocking
- Parameters:
-
report Report which will be sent (a report is defined by all data and the length)
- Returns:
- true if successful
Definition at line 39 of file USBHID.cpp.
bool update | ( | int16_t | x, |
int16_t | y, | ||
uint8_t | buttons, | ||
int8_t | z | ||
) |
Write a state of the mouse.
- Parameters:
-
x x-axis position y y-axis position buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) z wheel state (>0 to scroll down, <0 to scroll up)
- Returns:
- true if there is no error, false otherwise
Definition at line 22 of file USBMouse.cpp.
Generated on Tue Jul 12 2022 16:20:44 by
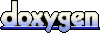