
blablabla
Dependencies: MAG3110 MMA8451Q SLCD- TSI USBDevice mbed
USBMouse.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBMOUSE_H 00020 #define USBMOUSE_H 00021 00022 #include "USBHID.h" 00023 00024 #define REPORT_ID_MOUSE 2 00025 00026 /* Common usage */ 00027 00028 enum MOUSE_BUTTON 00029 { 00030 MOUSE_LEFT = 1, 00031 MOUSE_RIGHT = 2, 00032 MOUSE_MIDDLE = 4, 00033 }; 00034 00035 /* X and Y limits */ 00036 /* These values do not directly map to screen pixels */ 00037 /* Zero may be interpreted as meaning 'no movement' */ 00038 #define X_MIN_ABS (1) /*!< Minimum value on x-axis */ 00039 #define Y_MIN_ABS (1) /*!< Minimum value on y-axis */ 00040 #define X_MAX_ABS (0x7fff) /*!< Maximum value on x-axis */ 00041 #define Y_MAX_ABS (0x7fff) /*!< Maximum value on y-axis */ 00042 00043 #define X_MIN_REL (-127) /*!< The maximum value that we can move to the left on the x-axis */ 00044 #define Y_MIN_REL (-127) /*!< The maximum value that we can move up on the y-axis */ 00045 #define X_MAX_REL (127) /*!< The maximum value that we can move to the right on the x-axis */ 00046 #define Y_MAX_REL (127) /*!< The maximum value that we can move down on the y-axis */ 00047 00048 enum MOUSE_TYPE 00049 { 00050 ABS_MOUSE, 00051 REL_MOUSE, 00052 }; 00053 00054 /** 00055 * 00056 * USBMouse example 00057 * @code 00058 * #include "mbed.h" 00059 * #include "USBMouse.h" 00060 * 00061 * USBMouse mouse; 00062 * 00063 * int main(void) 00064 * { 00065 * while (1) 00066 * { 00067 * mouse.move(20, 0); 00068 * wait(0.5); 00069 * } 00070 * } 00071 * 00072 * @endcode 00073 * 00074 * 00075 * @code 00076 * #include "mbed.h" 00077 * #include "USBMouse.h" 00078 * #include <math.h> 00079 * 00080 * USBMouse mouse(ABS_MOUSE); 00081 * 00082 * int main(void) 00083 * { 00084 * uint16_t x_center = (X_MAX_ABS - X_MIN_ABS)/2; 00085 * uint16_t y_center = (Y_MAX_ABS - Y_MIN_ABS)/2; 00086 * uint16_t x_screen = 0; 00087 * uint16_t y_screen = 0; 00088 * 00089 * uint32_t x_origin = x_center; 00090 * uint32_t y_origin = y_center; 00091 * uint32_t radius = 5000; 00092 * uint32_t angle = 0; 00093 * 00094 * while (1) 00095 * { 00096 * x_screen = x_origin + cos((double)angle*3.14/180.0)*radius; 00097 * y_screen = y_origin + sin((double)angle*3.14/180.0)*radius; 00098 * 00099 * mouse.move(x_screen, y_screen); 00100 * angle += 3; 00101 * wait(0.01); 00102 * } 00103 * } 00104 * 00105 * @endcode 00106 */ 00107 class USBMouse: public USBHID 00108 { 00109 public: 00110 00111 /** 00112 * Constructor 00113 * 00114 * @param mouse_type Mouse type: ABS_MOUSE (absolute mouse) or REL_MOUSE (relative mouse) (default: REL_MOUSE) 00115 * @param vendor_id Your vendor_id (default: 0x1234) 00116 * @param product_id Your product_id (default: 0x0001) 00117 * @param product_release Your preoduct_release (default: 0x0001) 00118 * 00119 */ 00120 USBMouse(MOUSE_TYPE mouse_type = REL_MOUSE, uint16_t vendor_id = 0x1234, uint16_t product_id = 0x0001, uint16_t product_release = 0x0001): 00121 USBHID(0, 0, vendor_id, product_id, product_release, false) 00122 { 00123 button = 0; 00124 this->mouse_type = mouse_type; 00125 connect(); 00126 }; 00127 00128 /** 00129 * Write a state of the mouse 00130 * 00131 * @param x x-axis position 00132 * @param y y-axis position 00133 * @param buttons buttons state (first bit represents MOUSE_LEFT, second bit MOUSE_RIGHT and third bit MOUSE_MIDDLE) 00134 * @param z wheel state (>0 to scroll down, <0 to scroll up) 00135 * @returns true if there is no error, false otherwise 00136 */ 00137 bool update(int16_t x, int16_t y, uint8_t buttons, int8_t z); 00138 00139 00140 /** 00141 * Move the cursor to (x, y) 00142 * 00143 * @param x-axis position 00144 * @param y-axis position 00145 * @returns true if there is no error, false otherwise 00146 */ 00147 bool move(int16_t x, int16_t y); 00148 00149 /** 00150 * Press one or several buttons 00151 * 00152 * @param button button state (ex: press(MOUSE_LEFT)) 00153 * @returns true if there is no error, false otherwise 00154 */ 00155 bool press(uint8_t button); 00156 00157 /** 00158 * Release one or several buttons 00159 * 00160 * @param button button state (ex: release(MOUSE_LEFT)) 00161 * @returns true if there is no error, false otherwise 00162 */ 00163 bool release(uint8_t button); 00164 00165 /** 00166 * Double click (MOUSE_LEFT) 00167 * 00168 * @returns true if there is no error, false otherwise 00169 */ 00170 bool doubleClick(); 00171 00172 /** 00173 * Click 00174 * 00175 * @param button state of the buttons ( ex: clic(MOUSE_LEFT)) 00176 * @returns true if there is no error, false otherwise 00177 */ 00178 bool click(uint8_t button); 00179 00180 /** 00181 * Scrolling 00182 * 00183 * @param z value of the wheel (>0 to go down, <0 to go up) 00184 * @returns true if there is no error, false otherwise 00185 */ 00186 bool scroll(int8_t z); 00187 00188 /* 00189 * To define the report descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00190 * 00191 * @returns pointer to the report descriptor 00192 */ 00193 virtual uint8_t * reportDesc(); 00194 00195 protected: 00196 /* 00197 * Get configuration descriptor 00198 * 00199 * @returns pointer to the configuration descriptor 00200 */ 00201 virtual uint8_t * configurationDesc(); 00202 00203 private: 00204 MOUSE_TYPE mouse_type; 00205 uint8_t button; 00206 bool mouseSend(int8_t x, int8_t y, uint8_t buttons, int8_t z); 00207 }; 00208 00209 #endif
Generated on Tue Jul 12 2022 16:20:44 by
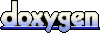