Simple interface for Mbed Cloud Client
pal_plat_rtos.h File Reference
PAL RTOS - platform. This file contains the real-time OS APIs that need to be implemented in the platform layer. More...
Go to the source code of this file.
Data Structures | |
struct | palThreadData |
PAL thread structure. More... | |
struct | palThreadServiceBridge |
PAL thread bridge structure - used by the platform thread to give control of the thread back to the service, see pal_plat_osThreadRun for more information. More... | |
Typedefs | |
typedef void * | palThreadPortData |
Type for holding platform specific (thread) data used exclusively by the platform, see pal_plat_osThreadDataInitialize for more information. | |
typedef struct palThreadData | palThreadData_t |
PAL thread structure. | |
typedef void(* | palThreadServiceBridgeFuncPtr )(palThreadData_t *threadData) |
PAL thread bridge function prototype. | |
typedef struct palThreadServiceBridge | palThreadServiceBridge_t |
PAL thread bridge structure - used by the platform thread to give control of the thread back to the service, see pal_plat_osThreadRun for more information. | |
Functions | |
void | pal_plat_osReboot (void) |
palStatus_t | pal_plat_RTOSInitialize (void *opaqueContext) |
palStatus_t | pal_plat_RTOSDestroy (void) |
uint64_t | pal_plat_osKernelSysTick (void) |
uint64_t | pal_plat_osKernelSysTickMicroSec (uint64_t microseconds) |
uint64_t | pal_plat_osKernelSysTickFrequency (void) |
int16_t | pal_plat_osThreadTranslatePriority (palThreadPriority_t priority) |
palStatus_t | pal_plat_osThreadDataInitialize (palThreadPortData *portData, int16_t priority, uint32_t stackSize) |
palStatus_t | pal_plat_osThreadRun (palThreadServiceBridge_t *bridge, palThreadID_t *osThreadID) |
palStatus_t | pal_plat_osThreadDataCleanup (palThreadData_t *threadData) |
palStatus_t | pal_plat_osThreadTerminate (palThreadData_t *threadData) |
palThreadID_t | pal_plat_osThreadGetId (void) |
palStatus_t | pal_plat_osDelay (uint32_t milliseconds) |
palStatus_t | pal_plat_osTimerCreate (palTimerFuncPtr function, void *funcArgument, palTimerType_t timerType, palTimerID_t *timerID) |
palStatus_t | pal_plat_osTimerStart (palTimerID_t timerID, uint32_t millisec) |
palStatus_t | pal_plat_osTimerStop (palTimerID_t timerID) |
palStatus_t | pal_plat_osTimerDelete (palTimerID_t *timerID) |
palStatus_t | pal_plat_osMutexCreate (palMutexID_t *mutexID) |
palStatus_t | pal_plat_osMutexWait (palMutexID_t mutexID, uint32_t millisec) |
palStatus_t | pal_plat_osMutexRelease (palMutexID_t mutexID) |
palStatus_t | pal_plat_osMutexDelete (palMutexID_t *mutexID) |
palStatus_t | pal_plat_osSemaphoreCreate (uint32_t count, palSemaphoreID_t *semaphoreID) |
palStatus_t | pal_plat_osSemaphoreWait (palSemaphoreID_t semaphoreID, uint32_t millisec, int32_t *countersAvailable) |
palStatus_t | pal_plat_osSemaphoreRelease (palSemaphoreID_t semaphoreID) |
palStatus_t | pal_plat_osSemaphoreDelete (palSemaphoreID_t *semaphoreID) |
int32_t | pal_plat_osAtomicIncrement (int32_t *valuePtr, int32_t increment) |
void * | pal_plat_malloc (size_t len) |
void | pal_plat_free (void *buffer) |
palStatus_t | pal_plat_osRandomBuffer (uint8_t *randomBuf, size_t bufSizeBytes, size_t *actualRandomSizeBytes) |
palStatus_t | pal_plat_osGetRoTFromHW (uint8_t *keyBuf, size_t keyLenBytes) |
palStatus_t | pal_plat_osSetRtcTime (uint64_t rtcSetTime) |
This function calls the platform layer and sets the new RTC to the H/W. | |
palStatus_t | pal_plat_osGetRtcTime (uint64_t *rtcGetTime) |
This function gets the RTC from the platform. | |
palStatus_t | pal_plat_rtcDeInit (void) |
This function DeInitialize the RTC module. | |
palStatus_t | pal_plat_rtcInit (void) |
This function initialize the RTC module. |
Detailed Description
PAL RTOS - platform. This file contains the real-time OS APIs that need to be implemented in the platform layer.
Definition in file pal_plat_rtos.h.
Typedef Documentation
typedef struct palThreadData palThreadData_t |
PAL thread structure.
typedef void* palThreadPortData |
Type for holding platform specific (thread) data used exclusively by the platform, see pal_plat_osThreadDataInitialize for more information.
Definition at line 34 of file pal_plat_rtos.h.
typedef struct palThreadServiceBridge palThreadServiceBridge_t |
PAL thread bridge structure - used by the platform thread to give control of the thread back to the service, see pal_plat_osThreadRun for more information.
typedef void(* palThreadServiceBridgeFuncPtr)(palThreadData_t *threadData) |
PAL thread bridge function prototype.
Definition at line 51 of file pal_plat_rtos.h.
Function Documentation
void pal_plat_free | ( | void * | buffer ) |
Free memory back to the OS heap.
- Parameters:
-
[in] *buffer A pointer to the buffer that should be free.
- Returns:
- `void`
Definition at line 724 of file FreeRTOS/RTOS/pal_plat_rtos.c.
void* pal_plat_malloc | ( | size_t | len ) |
Perform allocation from the heap according to the OS specification.
- Parameters:
-
[in] len The length of the buffer to be allocated.
- Returns:
- `void *`. The pointer of the malloc received from the OS if NULL error occurred
Definition at line 718 of file FreeRTOS/RTOS/pal_plat_rtos.c.
int32_t pal_plat_osAtomicIncrement | ( | int32_t * | valuePtr, |
int32_t | increment | ||
) |
Perform an atomic increment for a signed32 bit value.
- Parameters:
-
[in,out] valuePtr The address of the value to increment. [in] increment The number by which to increment.
- Returns:
- The value of the `valuePtr` after the increment operation.
Perform an atomic increment for a signed32 bit value.
- Parameters:
-
[in,out] valuePtr The address of the value to increment. [in] increment The number by which to increment.
- Returns:
- The value of the valuePtr after the increment operation.
Definition at line 1006 of file Linux/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osDelay | ( | uint32_t | milliseconds ) |
Wait for a specified period of time in milliseconds.
- Parameters:
-
[in] milliseconds The number of milliseconds to wait before proceeding.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
Wait for a specified period of time in milliseconds.
- Parameters:
-
[in] milliseconds The number of milliseconds to wait before proceeding.
- Returns:
- The status in the form of palStatus_t; PAL_SUCCESS(0) in case of success, a negative value indicating a specific error code in case of failure.
Definition at line 128 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osGetRoTFromHW | ( | uint8_t * | keyBuf, |
size_t | keyLenBytes | ||
) |
Retrieve platform Root of Trust certificate
- Parameters:
-
[in,out] *keyBuf A pointer to the buffer that holds the RoT. [in] keyLenBytes The size of the buffer to hold the 128 bit key, must be at least 16 bytes. The buffer needs to be able to hold 16 bytes of data.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
palStatus_t pal_plat_osGetRtcTime | ( | uint64_t * | rtcGetTime ) |
This function gets the RTC from the platform.
- Parameters:
-
[out] uint64_t * rtcGetTime - Holds the RTC value
- Returns:
- PAL_SUCCESS when the RTC return correctly
Definition at line 1065 of file Linux/RTOS/pal_plat_rtos.c.
uint64_t pal_plat_osKernelSysTick | ( | void | ) |
Get the RTOS kernel system timer counter.
- Returns:
- The RTOS kernel system timer counter.
- Note:
- The required tick counter is the OS (platform) kernel system tick counter.
- If the platform supports 64-bit tick counter, please implement it. If the platform supports only 32 bit, note that this counter wraps around very often (for example, once every 42 sec for 100Mhz).
Definition at line 135 of file FreeRTOS/RTOS/pal_plat_rtos.c.
uint64_t pal_plat_osKernelSysTickFrequency | ( | void | ) |
Get the system tick frequency.
- Returns:
- The system tick frequency.
- Note:
- The system tick frequency MUST be more than 1KHz (at least one tick per millisecond).
Get the system tick frequency.
- Returns:
- The system tick frequency.
Definition at line 156 of file FreeRTOS/RTOS/pal_plat_rtos.c.
uint64_t pal_plat_osKernelSysTickMicroSec | ( | uint64_t | microseconds ) |
Convert the value from microseconds to kernel sys ticks. This is the same as CMSIS macro `osKernelSysTickMicroSec`.
Definition at line 150 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osMutexCreate | ( | palMutexID_t * | mutexID ) |
Create and initialize a mutex object.
- Parameters:
-
[out] mutexID The created mutex ID handle, zero value indicates an error.
- Returns:
- PAL_SUCCESS when the mutex was created successfully, a specific error in case of failure.
PAL_ERR_NO_MEMORY when there is no memory resource available to create a mutex object.
- Note:
- The create function MUST NOT wait for the platform resources and it should return PAL_ERR_RTOS_RESOURCE, unless the platform API is blocking. By default, the mutex is created with a recursive flag set.
Create and initialize a mutex object.
- Parameters:
-
[out] mutexID The created mutex ID handle, zero value indicates an error.
- Returns:
- PAL_SUCCESS when the mutex was created successfully, a specific error in case of failure.
Definition at line 437 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osMutexDelete | ( | palMutexID_t * | mutexID ) |
Delete a mutex object.
- Parameters:
-
inout] mutexID The ID of the mutex to delete. In success, `*mutexID` = NULL.
- Returns:
- PAL_SUCCESS when the mutex was deleted successfully, one of the following error codes in case of failure:
- PAL_ERR_RTOS_RESOURCE - Mutex already released.
- PAL_ERR_RTOS_PARAMETER - Mutex ID is invalid.
- PAL_ERR_RTOS_ISR - Cannot be called from interrupt service routines.
- PAL_ERR_RTOS_RESOURCE - Mutex already released.
- Note:
- After this call, `mutex_id` is no longer valid and cannot be used.
Definition at line 540 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osMutexRelease | ( | palMutexID_t | mutexID ) |
Release a mutex that was obtained by `osMutexWait`.
- Parameters:
-
[in] mutexID The handle for the mutex.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
Definition at line 506 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osMutexWait | ( | palMutexID_t | mutexID, |
uint32_t | millisec | ||
) |
Wait until a mutex becomes available.
- Parameters:
-
[in] mutexID The handle for the mutex. [in] millisec The timeout for the waiting operation if the timeout expires before the semaphore is released and an error is returned from the function.
- Returns:
- PAL_SUCCESS(0) in case of success. One of the following error codes in case of failure:
- PAL_ERR_RTOS_RESOURCE - Mutex not available but no timeout set.
- PAL_ERR_RTOS_TIMEOUT - Mutex was not available until timeout expired.
- PAL_ERR_RTOS_PARAMETER - The mutex ID is invalid.
- PAL_ERR_RTOS_ISR - Cannot be called from interrupt service routines.
- PAL_ERR_RTOS_RESOURCE - Mutex not available but no timeout set.
Definition at line 470 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osRandomBuffer | ( | uint8_t * | randomBuf, |
size_t | bufSizeBytes, | ||
size_t * | actualRandomSizeBytes | ||
) |
Generate a random number into the given buffer with the given size in bytes.
- Parameters:
-
[out] randomBuf A buffer to hold the generated number. [in] bufSizeBytes The size of the buffer and the size of the required random number to generate. [out] actualRandomSizeBytes The actual size of the written random data to the output buffer.
- Returns:
- PAL_SUCCESS on success. A negative value indicating a specific error code in case of failure.
- Note:
- In case the platform was able to provide random data with non-zero size and less than `bufSizeBytes`the function must return `PAL_ERR_RTOS_TRNG_PARTIAL_DATA`
Definition at line 730 of file FreeRTOS/RTOS/pal_plat_rtos.c.
void pal_plat_osReboot | ( | void | ) |
Initiate a system reboot.
Definition at line 89 of file Linux/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osSemaphoreCreate | ( | uint32_t | count, |
palSemaphoreID_t * | semaphoreID | ||
) |
Create and initialize a semaphore object.
- Parameters:
-
[in] count The number of available resources. [out] semaphoreID The ID of the created semaphore, zero value indicates an error.
- Returns:
- PAL_SUCCESS when the semaphore was created successfully, a specific error in case of failure.
PAL_ERR_NO_MEMORY: No memory resource available to create a semaphore object.
- Note:
- The create function MUST not wait for the platform resources and it should return PAL_ERR_RTOS_RESOURCE, unless the platform API is blocking.
Definition at line 566 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osSemaphoreDelete | ( | palSemaphoreID_t * | semaphoreID ) |
Delete a semaphore object.
- Parameters:
-
inout] semaphoreID: The ID of the semaphore to delete. In success, `*semaphoreID` = NULL.
- Returns:
- PAL_SUCCESS when the semaphore was deleted successfully. One of the following error codes in case of failure:
PAL_ERR_RTOS_RESOURCE - Semaphore already released.
PAL_ERR_RTOS_PARAMETER - Semaphore ID is invalid.
- Note:
- After this call, the `semaphore_id` is no longer valid and cannot be used.
Delete a semaphore object.
- Parameters:
-
inout] semaphoreID: The ID of the semaphore to delete. In success, *semaphoreID = NULL.
- Returns:
- PAL_SUCCESS when the semaphore was deleted successfully, one of the following error codes in case of failure: PAL_ERR_RTOS_RESOURCE - Semaphore already released. PAL_ERR_RTOS_PARAMETER - Semaphore ID is invalid.
- Note:
- After this call, the semaphore_id is no longer valid and cannot be used.
Definition at line 691 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osSemaphoreRelease | ( | palSemaphoreID_t | semaphoreID ) |
Release a semaphore token.
- Parameters:
-
[in] semaphoreID The handle for the semaphore.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
Release a semaphore token.
- Parameters:
-
[in] semaphoreID The handle for the semaphore.
- Returns:
- The status in the form of palStatus_t; PAL_SUCCESS(0) in case of success, a negative value indicating a specific error code in case of failure.
Definition at line 650 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osSemaphoreWait | ( | palSemaphoreID_t | semaphoreID, |
uint32_t | millisec, | ||
int32_t * | countersAvailable | ||
) |
Wait until a semaphore token becomes available.
- Parameters:
-
[in] semaphoreID The handle for the semaphore. [in] millisec The timeout for the waiting operation if the timeout expires before the semaphore is released and an error is returned from the function. [out] countersAvailable The number of semaphores available. If semaphores are not available (timeout/error) zero is returned.
- Returns:
- PAL_SUCCESS(0) in case of success. One of the following error codes in case of failure:
- PAL_ERR_RTOS_TIMEOUT - Semaphore was not available until timeout expired.
- PAL_ERR_RTOS_PARAMETER - Semaphore ID is invalid.
- PAL_ERR_RTOS_TIMEOUT - Semaphore was not available until timeout expired.
Definition at line 601 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osSetRtcTime | ( | uint64_t | rtcSetTime ) |
This function calls the platform layer and sets the new RTC to the H/W.
- Parameters:
-
[in] uint64_t rtcSetTime the new RTC time
- Returns:
- PAL_SUCCESS when the RTC return correctly
Definition at line 1102 of file Linux/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osThreadDataCleanup | ( | palThreadData_t * | threadData ) |
Free any allocated data for the thread.
- Parameters:
-
[in] threadData A pointer to a palThreadData_t structure containing information about the thread.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
- Note:
- If this function is called then pal_plat_osThreadTerminate will not be called, so clean up all allocated resources (if any).
- This function is called from within the thread's context after the user function has been invoked and only if the thread hasn't been terminated.
Definition at line 200 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osThreadDataInitialize | ( | palThreadPortData * | portData, |
int16_t | priority, | ||
uint32_t | stackSize | ||
) |
Allocate platform specific data for the thread.
- Parameters:
-
[out] portData A pointer to a palThreadPortData type containing (optional) platform specific data about the thread. [in] priority Platform specific thread priority (post-translation). [in] stackSize Thread stack size.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
- Note:
- portData is used exclusively by the platform, the service layer has no use for this and all it does is keep the pointer as part of the palThreadData_t structure. If the platform does not have any allocations to be done then just return PAL_SUCCESS(0)
Definition at line 166 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palThreadID_t pal_plat_osThreadGetId | ( | void | ) |
Get the ID of the current thread.
- Returns:
- The ID of the current thread. In case of error, returns PAL_MAX_UINT32.
- Note:
- For a thread with real time priority, the function always returns PAL_MAX_UINT32.
Definition at line 205 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osThreadRun | ( | palThreadServiceBridge_t * | bridge, |
palThreadID_t * | osThreadID | ||
) |
Create and run the thread.
- Parameters:
-
[in] bridge A pointer to a palThreadServiceBridge_t structure which contains the function pointer and data which must be invoked from the created thread function. [out] osThreadID Platform specific thread id associated with the created thread.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
- Note:
- From within the created thread function the plaform MUST execute "bridge->function(bridge->threadData);". This is the liaison between the platform and the service which gives the control back to the service. It is recommended (if possible) to pass the bridge pointer as an argument (or as part of an argument) to the platfrom specific thread function.
Definition at line 171 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osThreadTerminate | ( | palThreadData_t * | threadData ) |
Terminate and free allocated data for the thread.
- Parameters:
-
[in] threadData A pointer to a palThreadData_t structure containing information about the thread.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
- Note:
- If this function is called then pal_plat_osThreadDataCleanup will not be called, so clean up all allocated resources.
Definition at line 211 of file FreeRTOS/RTOS/pal_plat_rtos.c.
int16_t pal_plat_osThreadTranslatePriority | ( | palThreadPriority_t | priority ) |
Translate from palThreadPriority_t to platform specific priority.
- Parameters:
-
[in] priority PAL priority to be translated.
- Returns:
- value representing the platform specific thread priority.
Definition at line 161 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osTimerCreate | ( | palTimerFuncPtr | function, |
void * | funcArgument, | ||
palTimerType_t | timerType, | ||
palTimerID_t * | timerID | ||
) |
Create a timer.
- Parameters:
-
[in] function A function pointer to the timer callback function. [in] funcArgument An argument for the timer callback function. [in] timerType The timer type to be created, periodic or `oneShot`. [out] timerID The ID of the created timer. Zero value indicates an error.
- Returns:
- PAL_SUCCESS when the timer was created successfully. A specific error in case of failure.
PAL_ERR_NO_MEMORY: No memory resource available to create a timer object.
- Note:
- The timer callback function runs according to the platform resources of stack size and priority.
- The create function MUST not wait for platform resources and it should return PAL_ERR_RTOS_RESOURCE, unless the platform API is blocking.
Create a timer.
- Parameters:
-
[in] function A function pointer to the timer callback function. [in] funcArgument An argument for the timer callback function. [in] timerType The timer type to be created, periodic or oneShot. [out] timerID The ID of the created timer, zero value indicates an error.
- Returns:
- PAL_SUCCESS when the timer was created successfully. A specific error in case of failure.
Definition at line 240 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osTimerDelete | ( | palTimerID_t * | timerID ) |
Delete the timer object.
- Parameters:
-
inout] timerID The handle for the timer to delete. In success, `*timerID` = NULL.
- Returns:
- PAL_SUCCESS when the timer was deleted successfully. PAL_ERR_RTOS_PARAMETER when the `timerID` is incorrect.
- Note:
- In case of a running timer, `pal_platosTimerDelete()` MUST stop the timer before deletion.
Delete the timer object
- Parameters:
-
inout] timerID The handle for the timer to delete. In success, *timerID = NULL.
- Returns:
- PAL_SUCCESS when the timer was deleted successfully, PAL_ERR_RTOS_PARAMETER when the timerID is incorrect.
Definition at line 392 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osTimerStart | ( | palTimerID_t | timerID, |
uint32_t | millisec | ||
) |
Start or restart a timer.
- Parameters:
-
[in] timerID The handle for the timer to start. [in] millisec,: The time in milliseconds to set the timer to, MUST be larger than 0. In case the value is 0, the error PAL_ERR_RTOS_VALUE will be returned.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
Start or restart a timer.
- Parameters:
-
[in] timerID The handle for the timer to start. [in] millisec The time in milliseconds to set the timer to.
- Returns:
- The status in the form of palStatus_t; PAL_SUCCESS(0) in case of success, a negative value indicating a specific error code in case of failure.
Definition at line 304 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_osTimerStop | ( | palTimerID_t | timerID ) |
Stop a timer.
- Parameters:
-
[in] timerID The handle for the timer to stop.
- Returns:
- PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure.
Stop a timer.
- Parameters:
-
[in] timerID The handle for the timer to stop.
- Returns:
- The status in the form of palStatus_t; PAL_SUCCESS(0) in case of success, a negative value indicating a specific error code in case of failure.
Definition at line 358 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_rtcDeInit | ( | void | ) |
This function DeInitialize the RTC module.
- Returns:
- PAL_SUCCESS when the success or error upon failing
Definition at line 1143 of file Linux/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_rtcInit | ( | void | ) |
This function initialize the RTC module.
- Returns:
- PAL_SUCCESS when the success or error upon failing
Definition at line 1133 of file Linux/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_RTOSDestroy | ( | void | ) |
De-initialize thread objects.
De-Initialize thread objects.
Definition at line 119 of file FreeRTOS/RTOS/pal_plat_rtos.c.
palStatus_t pal_plat_RTOSInitialize | ( | void * | opaqueContext ) |
Initialize all data structures (semaphores, mutexes, memory pools, message queues) at system initialization. In case of a failure in any of the initializations, the function returns an error and stops the rest of the initializations.
- Parameters:
-
[in] opaqueContext The context passed to the initialization (not required for generic CMSIS, pass NULL in this case).
- Returns:
- PAL_SUCCESS(0) in case of success, PAL_ERR_CREATION_FAILED in case of failure.
Initialize all data structures (semaphores, mutexs, memory pools, message queues) at system initialization. In case of a failure in any of the initializations, the function returns with an error and stops the rest of the initializations.
- Parameters:
-
[in] opaqueContext The context passed to the initialization (not required for generic CMSIS, pass NULL in this case).
- Returns:
- PAL_SUCCESS(0) in case of success, PAL_ERR_CREATION_FAILED in case of failure.
Definition at line 110 of file FreeRTOS/RTOS/pal_plat_rtos.c.
Generated on Tue Jul 12 2022 19:01:38 by
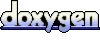