Simple interface for Mbed Cloud Client
Embed:
(wiki syntax)
Show/hide line numbers
pal_plat_rtos.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 /* PAL-RTOS porting for FreeRTOS-8.1.2 00018 * This is porting code for PAL RTOS APIS for 00019 * FreeRTOS-8.1.2 version. 00020 */ 00021 00022 #include "board.h" 00023 #include "FreeRTOS.h" 00024 #include "event_groups.h" 00025 #include "semphr.h" 00026 #include "task.h" 00027 00028 00029 #include "pal.h" 00030 #include "pal_plat_rtos.h" 00031 #include <stdlib.h> 00032 00033 00034 #define PAL_RTOS_TRANSLATE_CMSIS_ERROR_CODE(cmsisCode)\ 00035 ((int32_t)(cmsisCode + PAL_ERR_RTOS_ERROR_BASE)) 00036 00037 #define PAL_TICK_TO_MILLI_FACTOR 1000 00038 00039 PAL_PRIVATE int16_t g_threadPriorityMap[PAL_NUMBER_OF_THREAD_PRIORITIES] = 00040 { 00041 0, // PAL_osPriorityIdle 00042 1, // PAL_osPriorityLow 00043 2, // PAL_osPriorityReservedTRNG 00044 3, // PAL_osPriorityBelowNormal 00045 4, // PAL_osPriorityNormal 00046 5, // PAL_osPriorityAboveNormal 00047 6, // PAL_osPriorityReservedDNS 00048 7, // PAL_osPriorityReservedSockets 00049 8, // PAL_osPriorityHigh 00050 9, // PAL_osPriorityReservedHighResTimer 00051 10 // PAL_osPriorityRealtime 00052 }; 00053 00054 extern palStatus_t pal_plat_getRandomBufferFromHW(uint8_t *randomBuf, size_t bufSizeBytes, size_t* actualRandomSizeBytes); 00055 00056 /////////////////////////STATIC FUNCTION/////////////////////////// 00057 /*! Get IPSR Register 00058 * 00059 * @param[in] Void 00060 * \returns uint32 - the content of the IPSR Register. 00061 * 00062 */ 00063 PAL_PRIVATE PAL_INLINE uint32_t pal_plat_GetIPSR(void); 00064 /////////////////////////END STATIC FUNCTION/////////////////////////// 00065 00066 //! Timer structure 00067 typedef struct palTimer{ 00068 palTimerID_t timerID; 00069 // uint32_t internalTimerData[PAL_TIMER_DATA_SIZE]; ///< pointer to internal data 00070 TimerCallbackFunction_t function; 00071 void* functionArgs; 00072 uint32_t timerType; 00073 } palTimer_t; 00074 00075 //! Mutex structure 00076 typedef struct palMutex{ 00077 palMutexID_t mutexID; 00078 }palMutex_t; 00079 00080 //! Semaphore structure 00081 typedef struct palSemaphore{ 00082 palSemaphoreID_t semaphoreID; 00083 uint32_t maxCount; 00084 }palSemaphore_t; 00085 00086 00087 PAL_PRIVATE PAL_INLINE uint32_t pal_plat_GetIPSR(void) 00088 { 00089 uint32_t result; 00090 00091 #if defined (__CC_ARM) 00092 __asm volatile 00093 { 00094 MRS result, ipsr 00095 } 00096 #elif defined (__GNUC__) 00097 __asm volatile ("MRS %0, ipsr" : "=r" (result) ); 00098 #endif 00099 00100 return(result); 00101 } 00102 00103 PAL_PRIVATE void threadFunction(void const* arg) 00104 { 00105 palThreadServiceBridge_t* bridge = (palThreadServiceBridge_t*)arg; 00106 bridge->function(bridge->threadData ); 00107 vTaskDelete(NULL); 00108 } 00109 00110 palStatus_t pal_plat_RTOSInitialize (void* opaqueContext) 00111 { 00112 palStatus_t status = PAL_SUCCESS; 00113 #if (PAL_USE_HW_RTC) 00114 status = pal_plat_rtcInit(); 00115 #endif 00116 return status; 00117 } 00118 00119 palStatus_t pal_plat_RTOSDestroy (void) 00120 { 00121 palStatus_t status = PAL_SUCCESS; 00122 #if (PAL_USE_HW_RTC) 00123 status = pal_plat_rtcDeInit(); 00124 #endif 00125 return status; 00126 } 00127 00128 palStatus_t pal_plat_osDelay (uint32_t milliseconds) 00129 { 00130 vTaskDelay(milliseconds / portTICK_PERIOD_MS); 00131 return PAL_SUCCESS; 00132 } 00133 00134 00135 uint64_t pal_plat_osKernelSysTick () 00136 { 00137 00138 uint64_t result; 00139 if (pal_plat_GetIPSR() != 0) 00140 { 00141 result = xTaskGetTickCountFromISR(); 00142 } 00143 else 00144 { 00145 result = xTaskGetTickCount(); 00146 } 00147 return result; 00148 } 00149 00150 uint64_t pal_plat_osKernelSysTickMicroSec (uint64_t microseconds) 00151 { 00152 uint64_t sysTicks = microseconds * configTICK_RATE_HZ / (PAL_TICK_TO_MILLI_FACTOR * PAL_TICK_TO_MILLI_FACTOR); 00153 return sysTicks; 00154 } 00155 00156 uint64_t pal_plat_osKernelSysTickFrequency () 00157 { 00158 return configTICK_RATE_HZ; 00159 } 00160 00161 int16_t pal_plat_osThreadTranslatePriority (palThreadPriority_t priority) 00162 { 00163 return g_threadPriorityMap[priority]; 00164 } 00165 00166 palStatus_t pal_plat_osThreadDataInitialize (palThreadPortData* portData, int16_t priority, uint32_t stackSize) 00167 { 00168 return PAL_SUCCESS; 00169 } 00170 00171 palStatus_t pal_plat_osThreadRun (palThreadServiceBridge_t* bridge, palThreadID_t* osThreadID) 00172 { 00173 palStatus_t status = PAL_SUCCESS; 00174 TaskHandle_t threadID = NULLPTR; 00175 00176 //Note: the stack in this API is handled as an array of "StackType_t" which can be of different sizes for different ports. 00177 // in this specific port of (8.1.2) the "StackType_t" is defined to 4-bytes this is why we divide the "stackSize" parameter by "sizeof(uint32_t)". 00178 // inside freeRTOS code, the stack size is calculated according to the following formula: "((size_t)usStackDepth) * sizeof(StackType_t)" 00179 // where "usStackDepth" is equal to "stackSize / sizeof(uint32_t)" 00180 BaseType_t result = xTaskGenericCreate((TaskFunction_t)threadFunction, 00181 "palTask", 00182 (bridge->threadData ->stackSize / sizeof(uint32_t)), 00183 bridge, 00184 bridge->threadData ->osPriority , 00185 &threadID, 00186 NULL, //if stack pointer is NULL then allocate the stack according to stack size 00187 NULL); 00188 00189 if (pdPASS == result) 00190 { 00191 *osThreadID = (palThreadID_t)threadID; 00192 } 00193 else 00194 { 00195 status = PAL_ERR_GENERIC_FAILURE; 00196 } 00197 return status; 00198 } 00199 00200 palStatus_t pal_plat_osThreadDataCleanup (palThreadData_t* threadData) 00201 { 00202 return PAL_SUCCESS; 00203 } 00204 00205 palThreadID_t pal_plat_osThreadGetId (void) 00206 { 00207 palThreadID_t osThreadID = (palThreadID_t)xTaskGetCurrentTaskHandle(); 00208 return osThreadID; 00209 } 00210 00211 palStatus_t pal_plat_osThreadTerminate (palThreadData_t* threadData) 00212 { 00213 palStatus_t status = PAL_ERR_RTOS_TASK ; 00214 TaskHandle_t threadID = (TaskHandle_t)(threadData->osThreadID ); 00215 if (xTaskGetCurrentTaskHandle() != threadID) // terminate only if not trying to terminate from self 00216 { 00217 vTaskDelete(threadID); 00218 status = PAL_SUCCESS; 00219 } 00220 return status; 00221 } 00222 00223 PAL_PRIVATE palTimer_t* s_timerArrays[PAL_MAX_NUM_OF_TIMERS] = {0}; 00224 00225 PAL_PRIVATE void pal_plat_osTimerWarpperFunction( TimerHandle_t xTimer ) 00226 { 00227 int i; 00228 palTimer_t* timer = NULL; 00229 for(i=0 ; i< PAL_MAX_NUM_OF_TIMERS ; i++) 00230 { 00231 if (s_timerArrays[i]->timerID == (palTimerID_t)xTimer) 00232 { 00233 timer = s_timerArrays[i]; 00234 timer->function(timer->functionArgs); 00235 00236 } 00237 } 00238 } 00239 00240 palStatus_t pal_plat_osTimerCreate (palTimerFuncPtr function, void* funcArgument, palTimerType_t timerType, palTimerID_t* timerID) 00241 { 00242 palStatus_t status = PAL_SUCCESS; 00243 palTimer_t* timer = NULL; 00244 int i; 00245 if(NULL == timerID || NULL == function) 00246 { 00247 return PAL_ERR_INVALID_ARGUMENT ; 00248 } 00249 00250 timer = (palTimer_t*)malloc(sizeof(palTimer_t)); 00251 00252 if (NULL == timer) 00253 { 00254 status = PAL_ERR_NO_MEMORY ; 00255 } 00256 else 00257 { 00258 memset(timer,0,sizeof(palTimer_t)); 00259 } 00260 00261 if (PAL_SUCCESS == status) 00262 { 00263 for (i=0; i< PAL_MAX_NUM_OF_TIMERS; i++) 00264 { 00265 if (s_timerArrays[i] == NULL) 00266 { 00267 s_timerArrays[i] = timer; 00268 break; 00269 } 00270 } 00271 if (PAL_MAX_NUM_OF_TIMERS == i) 00272 { 00273 status = PAL_ERR_NO_MEMORY ; 00274 } 00275 if (PAL_SUCCESS == status) 00276 { 00277 timer->function = (TimerCallbackFunction_t)function; 00278 timer->functionArgs = funcArgument; 00279 timer->timerType = timerType; 00280 00281 timer->timerID = (palTimerID_t)xTimerCreate( 00282 "timer", 00283 1, // xTimerPeriod - cannot be '0' 00284 (const TickType_t)timerType, // 0 = osTimerOnce, 1 = osTimerPeriodic 00285 NULL, 00286 (TimerCallbackFunction_t)pal_plat_osTimerWarpperFunction 00287 ); 00288 } 00289 if (NULLPTR == timer->timerID) 00290 { 00291 free(timer); 00292 timer = NULLPTR; 00293 PAL_LOG(ERR, "Rtos timer create failure"); 00294 status = PAL_ERR_GENERIC_FAILURE; 00295 } 00296 else 00297 { 00298 *timerID = (palTimerID_t)timer; 00299 } 00300 } 00301 return status; 00302 } 00303 00304 palStatus_t pal_plat_osTimerStart (palTimerID_t timerID, uint32_t millisec) 00305 { 00306 palStatus_t status = PAL_SUCCESS; 00307 palTimer_t* timer = NULL; 00308 00309 if (NULLPTR == timerID) 00310 { 00311 return PAL_ERR_INVALID_ARGUMENT ; 00312 } 00313 00314 timer = (palTimer_t*)timerID; 00315 00316 if (pal_plat_GetIPSR() != 0) 00317 { 00318 BaseType_t pxHigherPriorityTaskWoken; 00319 status = xTimerChangePeriodFromISR( 00320 (TimerHandle_t)(timer->timerID), 00321 (millisec / portTICK_PERIOD_MS), 00322 &pxHigherPriorityTaskWoken 00323 ); 00324 } 00325 else 00326 { 00327 status = xTimerChangePeriod((TimerHandle_t)(timer->timerID), (millisec / portTICK_PERIOD_MS), 0); 00328 } 00329 00330 if (pdPASS != status) 00331 { 00332 status = PAL_ERR_RTOS_PARAMETER ; 00333 } 00334 if (pdPASS == status) 00335 { 00336 if (pal_plat_GetIPSR() != 0) 00337 { 00338 BaseType_t pxHigherPriorityTaskWoken; 00339 status = xTimerStartFromISR((TimerHandle_t)(timer->timerID), &pxHigherPriorityTaskWoken); 00340 } 00341 else 00342 { 00343 status = xTimerStart((TimerHandle_t)(timer->timerID), 0); 00344 } 00345 00346 if (pdPASS != status) 00347 { 00348 status = PAL_ERR_RTOS_PARAMETER ; 00349 } 00350 else 00351 { 00352 status = PAL_SUCCESS; 00353 } 00354 } 00355 return status; 00356 } 00357 00358 palStatus_t pal_plat_osTimerStop (palTimerID_t timerID) 00359 { 00360 palStatus_t status = PAL_SUCCESS; 00361 palTimer_t* timer = NULL; 00362 00363 if(NULLPTR == timerID) 00364 { 00365 return PAL_ERR_INVALID_ARGUMENT ; 00366 } 00367 00368 timer = (palTimer_t*)timerID; 00369 00370 if (pal_plat_GetIPSR() != 0) 00371 { 00372 BaseType_t pxHigherPriorityTaskWoken; 00373 status = xTimerStopFromISR((TimerHandle_t)(timer->timerID), &pxHigherPriorityTaskWoken); 00374 } 00375 else 00376 { 00377 status = xTimerStop((TimerHandle_t)(timer->timerID), 0); 00378 } 00379 00380 00381 if (pdPASS != status) 00382 { 00383 status = PAL_ERR_RTOS_PARAMETER ; 00384 } 00385 else 00386 { 00387 status = PAL_SUCCESS; 00388 } 00389 return status; 00390 } 00391 00392 palStatus_t pal_plat_osTimerDelete (palTimerID_t* timerID) 00393 { 00394 palStatus_t status = PAL_ERR_RTOS_PARAMETER ; 00395 palTimer_t* timer = NULL; 00396 int i; 00397 00398 if(NULL == timerID || NULLPTR == *timerID) 00399 { 00400 return PAL_ERR_INVALID_ARGUMENT ; 00401 } 00402 00403 timer = (palTimer_t*)*timerID; 00404 00405 if (timer->timerID) 00406 { 00407 for(i=0 ; i< PAL_MAX_NUM_OF_TIMERS ; i++) 00408 { 00409 if (s_timerArrays[i] == timer) 00410 { 00411 status = xTimerDelete((TimerHandle_t)(timer->timerID), 0); 00412 free(timer); 00413 s_timerArrays[i] = NULL; 00414 *timerID = NULLPTR; 00415 break; 00416 } 00417 } 00418 00419 if (pdPASS == status) 00420 { 00421 status = PAL_SUCCESS; 00422 } 00423 else 00424 { 00425 status = PAL_ERR_RTOS_PARAMETER ; 00426 } 00427 } 00428 else 00429 { 00430 status = PAL_ERR_RTOS_PARAMETER ; 00431 } 00432 00433 return status; 00434 } 00435 00436 00437 palStatus_t pal_plat_osMutexCreate (palMutexID_t* mutexID) 00438 { 00439 00440 palStatus_t status = PAL_SUCCESS; 00441 palMutex_t* mutex = NULL; 00442 if(NULL == mutexID) 00443 { 00444 return PAL_ERR_INVALID_ARGUMENT ; 00445 } 00446 00447 mutex = (palMutex_t*)malloc(sizeof(palMutex_t)); 00448 if (NULL == mutex) 00449 { 00450 status = PAL_ERR_NO_MEMORY ; 00451 } 00452 00453 if (PAL_SUCCESS == status) 00454 { 00455 00456 mutex->mutexID = (uintptr_t) xSemaphoreCreateRecursiveMutex(); 00457 if (NULLPTR == mutex->mutexID) 00458 { 00459 free(mutex); 00460 mutex = NULL; 00461 PAL_LOG(ERR, "Rtos mutex create failure"); 00462 status = PAL_ERR_GENERIC_FAILURE; 00463 } 00464 *mutexID = (palMutexID_t)mutex; 00465 } 00466 return status; 00467 } 00468 00469 00470 palStatus_t pal_plat_osMutexWait (palMutexID_t mutexID, uint32_t millisec) 00471 { 00472 00473 palStatus_t status = PAL_SUCCESS; 00474 palMutex_t* mutex = NULL; 00475 BaseType_t res = pdTRUE; 00476 00477 if(NULLPTR == mutexID) 00478 { 00479 return PAL_ERR_INVALID_ARGUMENT ; 00480 } 00481 00482 mutex = (palMutex_t*)mutexID; 00483 if (pal_plat_GetIPSR() != 0) 00484 { 00485 BaseType_t pxHigherPriorityTaskWoken; 00486 res = xSemaphoreTakeFromISR(mutex->mutexID, &pxHigherPriorityTaskWoken); 00487 } 00488 else 00489 { 00490 res = xSemaphoreTakeRecursive((QueueHandle_t)(mutex->mutexID), (millisec / portTICK_PERIOD_MS) ); 00491 } 00492 00493 if (pdTRUE == res) 00494 { 00495 status = PAL_SUCCESS; 00496 } 00497 else 00498 { 00499 status = PAL_ERR_RTOS_TIMEOUT ; 00500 } 00501 00502 return status; 00503 } 00504 00505 00506 palStatus_t pal_plat_osMutexRelease (palMutexID_t mutexID) 00507 { 00508 palStatus_t status = PAL_SUCCESS; 00509 palMutex_t* mutex = NULL; 00510 BaseType_t res = pdTRUE; 00511 00512 if(NULLPTR == mutexID) 00513 { 00514 return PAL_ERR_INVALID_ARGUMENT ; 00515 } 00516 00517 mutex = (palMutex_t*)mutexID; 00518 if (pal_plat_GetIPSR() != 0) 00519 { 00520 BaseType_t pxHigherPriorityTaskWoken; 00521 res = xSemaphoreGiveFromISR(mutex->mutexID, &pxHigherPriorityTaskWoken); 00522 } 00523 else 00524 { 00525 res = xSemaphoreGiveRecursive((QueueHandle_t)(mutex->mutexID)); 00526 } 00527 00528 if (pdTRUE == res) 00529 { 00530 status = PAL_SUCCESS; 00531 } 00532 else 00533 { 00534 PAL_LOG(ERR, "Rtos mutex release failure %ld", res); 00535 status = PAL_ERR_GENERIC_FAILURE; 00536 } 00537 return status; 00538 } 00539 00540 palStatus_t pal_plat_osMutexDelete (palMutexID_t* mutexID) 00541 { 00542 palStatus_t status = PAL_SUCCESS; 00543 palMutex_t* mutex = NULL; 00544 00545 if(NULL == mutexID || NULLPTR == *mutexID) 00546 { 00547 return PAL_ERR_INVALID_ARGUMENT ; 00548 } 00549 00550 mutex = (palMutex_t*)*mutexID; 00551 if (NULLPTR != mutex->mutexID) 00552 { 00553 vSemaphoreDelete(mutex->mutexID); 00554 free(mutex); 00555 *mutexID = NULLPTR; 00556 status = PAL_SUCCESS; 00557 } 00558 else 00559 { 00560 PAL_LOG(ERR, "Rtos mutex delete failure"); 00561 status = PAL_ERR_GENERIC_FAILURE; 00562 } 00563 return status; 00564 } 00565 00566 palStatus_t pal_plat_osSemaphoreCreate (uint32_t count, palSemaphoreID_t* semaphoreID) 00567 { 00568 palStatus_t status = PAL_SUCCESS; 00569 palSemaphore_t* semaphore = NULL; 00570 00571 if(NULL == semaphoreID) 00572 { 00573 return PAL_ERR_INVALID_ARGUMENT ; 00574 } 00575 00576 semaphore = (palSemaphore_t*)malloc(sizeof(palSemaphore_t)); 00577 if (NULL == semaphore) 00578 { 00579 status = PAL_ERR_NO_MEMORY ; 00580 } 00581 00582 if(PAL_SUCCESS == status) 00583 { 00584 semaphore->semaphoreID = (uintptr_t)xSemaphoreCreateCounting(PAL_SEMAPHORE_MAX_COUNT, count); 00585 semaphore->maxCount = PAL_SEMAPHORE_MAX_COUNT; 00586 if (NULLPTR == semaphore->semaphoreID) 00587 { 00588 free(semaphore); 00589 semaphore = NULLPTR; 00590 PAL_LOG(ERR, "Rtos semaphore create error"); 00591 status = PAL_ERR_GENERIC_FAILURE; 00592 } 00593 else 00594 { 00595 *semaphoreID = (palSemaphoreID_t)semaphore; 00596 } 00597 } 00598 return status; 00599 } 00600 00601 palStatus_t pal_plat_osSemaphoreWait (palSemaphoreID_t semaphoreID, uint32_t millisec, int32_t* countersAvailable) 00602 { 00603 palStatus_t status = PAL_SUCCESS; 00604 palSemaphore_t* semaphore = NULL; 00605 int32_t tmpCounters = 0; 00606 BaseType_t res = pdTRUE; 00607 00608 if(NULLPTR == semaphoreID) 00609 { 00610 return PAL_ERR_INVALID_ARGUMENT ; 00611 } 00612 00613 semaphore = (palSemaphore_t*)semaphoreID; 00614 if (pal_plat_GetIPSR() != 0) 00615 { 00616 BaseType_t pxHigherPriorityTaskWoken; 00617 res = xSemaphoreTakeFromISR(semaphore->semaphoreID, &pxHigherPriorityTaskWoken); 00618 } 00619 else 00620 { 00621 if (millisec == PAL_RTOS_WAIT_FOREVER) 00622 { 00623 res = xSemaphoreTake(semaphore->semaphoreID, portMAX_DELAY); 00624 } 00625 else 00626 { 00627 res = xSemaphoreTake(semaphore->semaphoreID, millisec / portTICK_PERIOD_MS); 00628 } 00629 } 00630 00631 if (pdTRUE == res) 00632 { 00633 00634 tmpCounters = uxQueueMessagesWaiting((QueueHandle_t)(semaphore->semaphoreID)); 00635 } 00636 else 00637 { 00638 tmpCounters = 0; 00639 status = PAL_ERR_RTOS_TIMEOUT ; 00640 } 00641 00642 if (NULL != countersAvailable) 00643 { 00644 //because mbedOS returns the number available BEFORE the current take, we have to add 1 here. 00645 *countersAvailable = tmpCounters; 00646 } 00647 return status; 00648 } 00649 00650 palStatus_t pal_plat_osSemaphoreRelease (palSemaphoreID_t semaphoreID) 00651 { 00652 palStatus_t status = PAL_SUCCESS; 00653 palSemaphore_t* semaphore = NULL; 00654 BaseType_t res = pdTRUE; 00655 int32_t tmpCounters = 0; 00656 00657 if(NULLPTR == semaphoreID) 00658 { 00659 return PAL_ERR_INVALID_ARGUMENT ; 00660 } 00661 00662 semaphore = (palSemaphore_t*)semaphoreID; 00663 00664 tmpCounters = uxQueueMessagesWaiting((QueueHandle_t)(semaphore->semaphoreID)); 00665 00666 if(tmpCounters < semaphore->maxCount) 00667 { 00668 if (pal_plat_GetIPSR() != 0) 00669 { 00670 BaseType_t pxHigherPriorityTaskWoken; 00671 res = xSemaphoreGiveFromISR(semaphore->semaphoreID, &pxHigherPriorityTaskWoken); 00672 } 00673 else 00674 { 00675 res = xSemaphoreGive(semaphore->semaphoreID); 00676 } 00677 00678 if (pdTRUE != res) 00679 { 00680 status = PAL_ERR_RTOS_PARAMETER ; 00681 } 00682 } 00683 else 00684 { 00685 status = PAL_ERR_RTOS_RESOURCE ; 00686 } 00687 00688 return status; 00689 } 00690 00691 palStatus_t pal_plat_osSemaphoreDelete (palSemaphoreID_t* semaphoreID) 00692 { 00693 palStatus_t status = PAL_SUCCESS; 00694 palSemaphore_t* semaphore = NULL; 00695 00696 if(NULL == semaphoreID || NULLPTR == *semaphoreID) 00697 { 00698 return PAL_ERR_INVALID_ARGUMENT ; 00699 } 00700 00701 semaphore = (palSemaphore_t*)*semaphoreID; 00702 if (NULLPTR != semaphore->semaphoreID) 00703 { 00704 vSemaphoreDelete(semaphore->semaphoreID); 00705 free(semaphore); 00706 *semaphoreID = NULLPTR; 00707 status = PAL_SUCCESS; 00708 } 00709 else 00710 { 00711 PAL_LOG(ERR, "Rtos semaphore destroy error"); 00712 status = PAL_ERR_GENERIC_FAILURE; 00713 } 00714 return status; 00715 } 00716 00717 00718 void *pal_plat_malloc (size_t len) 00719 { 00720 return malloc(len); 00721 } 00722 00723 00724 void pal_plat_free (void * buffer) 00725 { 00726 free(buffer); 00727 } 00728 00729 00730 palStatus_t pal_plat_osRandomBuffer (uint8_t *randomBuf, size_t bufSizeBytes, size_t* actualRandomSizeBytes) 00731 { 00732 palStatus_t status = PAL_SUCCESS; 00733 00734 status = pal_plat_getRandomBufferFromHW(randomBuf, bufSizeBytes, actualRandomSizeBytes); 00735 return status; 00736 } 00737 00738
Generated on Tue Jul 12 2022 19:01:36 by
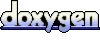